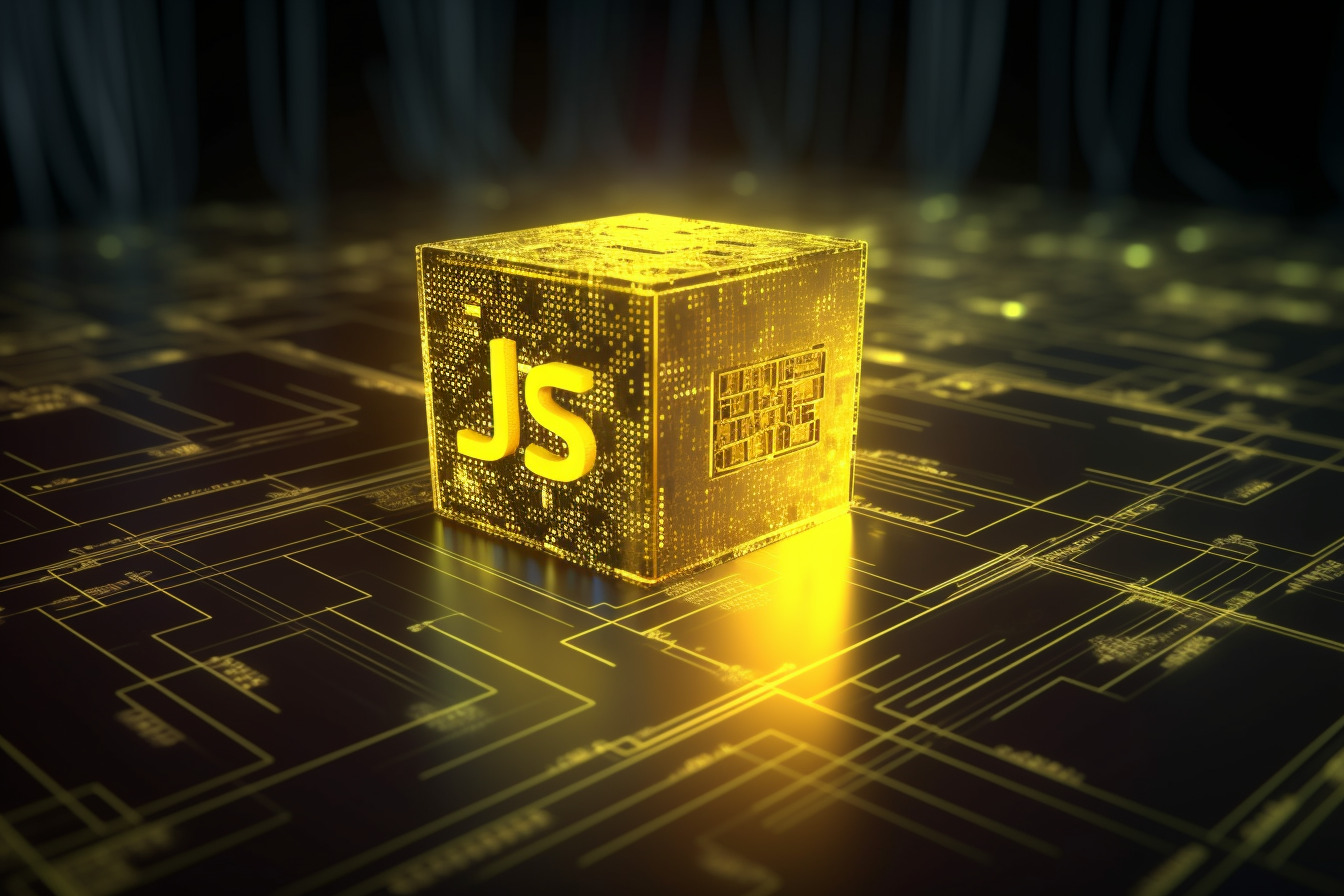
- What are the Spread and Rest Operators?
- The Spread Operator
- The Rest Operator
- Using the Spread Operator to Copy Arrays
- Using the Spread Operator to Concatenate Arrays
- Using the Spread Operator with Function Arguments
- Using the Spread Operator to Merge Objects
- Using the Rest Operator to Gather Function Arguments
- Using the Rest Operator to Destructure Arrays
- Using the Rest Operator to Destructure Objects
- Applying the Spread and Rest Operators in Real World Examples
- 1. Combining Arrays
- 2. Copying Arrays and Objects
- 3. Function Arguments
- 4. Function Parameters
- 5. Object Property Shorthand
- Advanced Techniques: Combining Spread and Rest Operators
- Combining Arrays with the Spread Operator
- Using Rest Parameters to Collect Arguments
- Combining Arrays and Rest Parameters
- Advanced Techniques: Partial Application with the Rest Operator
- Advanced Techniques: Using the Spread Operator with Strings
- Common Mistakes and Pitfalls to Avoid
- 1. Forgetting to use the Spread Operator
- 2. Misusing the Rest Operator
- 3. Modifying Original Arrays
- 4. Using Spread Operator on Non-Iterable Objects
What are the Spread and Rest Operators?
The spread and rest operators are two powerful features introduced in ECMAScript 6 (ES6) that provide a concise and flexible way to work with arrays and objects in JavaScript. Both operators use the three-dot syntax (...
) but serve different purposes.
Related Article: How To Generate Random String Characters In Javascript
The Spread Operator
The spread operator allows us to “spread” or unpack elements from an iterable (like an array or a string) into another iterable or as arguments to a function. It provides a convenient way to create copies, merge or combine multiple arrays, and pass multiple arguments to a function.
Here’s an example of using the spread operator to create a copy of an array:
const numbers = [1, 2, 3]; const copyNumbers = [...numbers]; console.log(copyNumbers); // Output: [1, 2, 3]
We can also use the spread operator to merge multiple arrays into a single array:
const array1 = [1, 2, 3]; const array2 = [4, 5, 6]; const mergedArray = [...array1, ...array2]; console.log(mergedArray); // Output: [1, 2, 3, 4, 5, 6]
In addition, the spread operator can be used to pass multiple arguments to a function:
function addNumbers(a, b, c) { return a + b + c; } const numbers = [1, 2, 3]; const sum = addNumbers(...numbers); console.log(sum); // Output: 6
The Rest Operator
The rest operator allows us to represent an indefinite number of arguments as an array. It allows functions to accept any number of arguments, making them more flexible and versatile.
Here’s an example of using the rest operator to accept multiple arguments in a function:
function sumNumbers(...numbers) { return numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0); } const sum = sumNumbers(1, 2, 3, 4, 5); console.log(sum); // Output: 15
The rest operator can also be used to capture the remaining elements of an array when destructuring:
const [first, second, ...rest] = [1, 2, 3, 4, 5]; console.log(first); // Output: 1 console.log(second); // Output: 2 console.log(rest); // Output: [3, 4, 5]
The spread and rest operators are powerful tools that enhance the capabilities of JavaScript when working with arrays and objects. They provide concise syntax and enable us to write more expressive and flexible code. Understanding these operators is essential for any JavaScript developer looking to leverage the full potential of ES6.
For more information, you can refer to the official ECMAScript documentation on the spread operator and rest operator.
Using the Spread Operator to Copy Arrays
To copy an array using the spread operator, we simply place three dots (…) before the array we want to copy. Here’s an example:
const originalArray = [1, 2, 3]; const copiedArray = [...originalArray]; console.log(copiedArray); // Output: [1, 2, 3]
In the above example, we create a new variable copiedArray
and assign it the value of originalArray
using the spread operator. The result is a new array with the same elements as the original array.
It’s important to note that the copied array is a shallow copy. This means that if the elements of the original array are objects or arrays themselves, the copied array will still reference the same objects or arrays. Any changes made to the objects or arrays within the copied array will also affect the original array.
Let’s take a look at an example that demonstrates this:
const originalArray = [{ name: 'John' }, { name: 'Jane' }]; const copiedArray = [...originalArray]; copiedArray[0].name = 'Mike'; console.log(originalArray); // Output: [{ name: 'Mike' }, { name: 'Jane' }]
In the above example, we have an array of objects originalArray
. We create a copy of this array using the spread operator and assign it to copiedArray
. Then, we modify the name
property of the first object in copiedArray
. As a result, the name
property of the first object in originalArray
is also updated.
To avoid this behavior and create a deep copy of an array, we can use other methods like Array.from()
or JSON.parse(JSON.stringify())
. However, these methods have their own limitations and may not work as expected in all scenarios.
Related Article: How to Work with Async Calls in JavaScript
Using the Spread Operator to Concatenate Arrays
To use the spread operator to concatenate arrays, we simply place three dots (…) in front of the array we want to spread. Let’s see some examples to understand how it works.
const array1 = [1, 2, 3]; const array2 = [4, 5, 6]; const concatenatedArray = [...array1, ...array2]; console.log(concatenatedArray); // Output: [1, 2, 3, 4, 5, 6]
In the above example, we have two arrays, array1
and array2
. By using the spread operator, we can easily concatenate them into a new array called concatenatedArray
. The resulting array contains all the elements from array1
followed by all the elements from array2
.
The spread operator can also be used to concatenate multiple arrays at once. Let’s see an example:
const array1 = [1, 2, 3]; const array2 = [4, 5, 6]; const array3 = [7, 8, 9]; const concatenatedArray = [...array1, ...array2, ...array3]; console.log(concatenatedArray); // Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
In this example, we have three arrays, array1
, array2
, and array3
. By using the spread operator, we can concatenate all three arrays into a new array called concatenatedArray
. The resulting array contains all the elements from each individual array.
The spread operator is not limited to just arrays. It can also be used to concatenate other iterable objects like strings or even the arguments object in a function call. Let’s see an example with strings:
const string1 = "Hello"; const string2 = "World"; const concatenatedString = [...string1, ...string2]; console.log(concatenatedString); // Output: ['H', 'e', 'l', 'l', 'o', 'W', 'o', 'r', 'l', 'd']
In this example, we have two strings, string1
and string2
. By using the spread operator, we can concatenate them into a new array called concatenatedString
. The resulting array contains all the characters from both strings.
The spread operator is a handy tool in JavaScript ES6 for quickly concatenating arrays, strings, or any other iterable objects. It provides a clean and concise syntax, making our code more readable and efficient.
To learn more about the spread operator and its other use cases, you can refer to the Mozilla Developer Network (MDN) documentation.
Using the Spread Operator with Function Arguments
When used with function arguments, the spread operator allows us to pass an array as individual arguments to a function. This can be especially useful when the function expects a specific number of arguments or when we want to combine multiple arrays into a single argument. Let’s look at some examples to understand this better.
Suppose we have a function called sum
that takes in three arguments and returns their sum:
function sum(a, b, c) { return a + b + c; }
If we want to call this function with an array of three numbers, we can use the spread operator to pass the array elements as individual arguments:
const numbers = [1, 2, 3]; const result = sum(...numbers); console.log(result); // Output: 6
In this example, the spread operator ...
before the numbers
array expands it into three individual arguments: 1
, 2
, and 3
. The sum
function receives these arguments and returns their sum, which is 6
.
The spread operator can also be used to combine multiple arrays into a single argument. Let’s say we have two arrays of numbers, array1
and array2
, and we want to pass their combined elements as arguments to a function:
const array1 = [1, 2, 3]; const array2 = [4, 5, 6]; const combinedArray = [...array1, ...array2]; console.log(combinedArray); // Output: [1, 2, 3, 4, 5, 6]
In this example, the spread operator is used to concatenate the elements of array1
and array2
into a new array called combinedArray
. The resulting array contains all the elements from both arrays.
The spread operator is not limited to arrays. It can also be used with other iterable objects like strings, maps, and sets. For example, we can use the spread operator to split a string into individual characters:
const string = "hello"; const characters = [...string]; console.log(characters); // Output: ['h', 'e', 'l', 'l', 'o']
In this example, the spread operator is used to split the string "hello"
into individual characters and store them in the characters
array.
The spread operator with function arguments provides a more concise and flexible way to pass arrays or iterable objects as individual arguments. It allows us to work with arrays more easily and perform operations like concatenation or splitting with minimal code.
Using the Spread Operator to Merge Objects
To use the spread operator to merge objects, we simply place the spread operator in front of each object that we want to merge. Let’s take a look at an example:
const obj1 = { name: 'John' }; const obj2 = { age: 25 }; const mergedObj = { ...obj1, ...obj2 }; console.log(mergedObj);
In the above code snippet, we have two objects obj1
and obj2
. We then create a new object mergedObj
by using the spread operator to merge the properties of obj1
and obj2
. The result is a new object with properties from both objects:
{ name: 'John', age: 25 }
The spread operator can also be used to merge multiple objects together. Let’s see an example:
const obj1 = { name: 'John' }; const obj2 = { age: 25 }; const obj3 = { occupation: 'Developer' }; const mergedObj = { ...obj1, ...obj2, ...obj3 }; console.log(mergedObj);
In this example, we have three objects obj1
, obj2
, and obj3
. We use the spread operator to merge all three objects into a new object mergedObj
. The resulting object will contain properties from all three objects:
{ name: 'John', age: 25, occupation: 'Developer' }
It’s important to note that if there are duplicate properties in the objects being merged, the property from the last object will overwrite the previous ones. For example:
const obj1 = { name: 'John', age: 25 }; const obj2 = { age: 30 }; const mergedObj = { ...obj1, ...obj2 }; console.log(mergedObj);
In this case, the resulting object will have the age property overwritten with the value from obj2
:
{ name: 'John', age: 30 }
The spread operator is a handy tool when it comes to merging objects in JavaScript. It allows us to easily combine properties from multiple objects into a new object. Keep in mind that the spread operator is only available in ES6 and newer versions of JavaScript.
To learn more about the spread operator and other features of JavaScript ES6, you can refer to the official documentation on the Mozilla Developer Network.
Related Article: Understanding JavaScript Execution Context and Hoisting
Using the Rest Operator to Gather Function Arguments
The rest operator is particularly useful when you want to pass multiple arguments to a function without knowing how many arguments will be passed. Instead of using the arguments object, which is an array-like object, you can use the rest operator to gather the arguments into a real array.
Here’s an example that demonstrates how the rest operator can be used to gather function arguments:
function sum(...numbers) { let total = 0; for (let number of numbers) { total += number; } return total; } console.log(sum(1, 2, 3, 4, 5)); // Output: 15
In the above example, the sum
function accepts a variable number of arguments using the rest operator. The numbers
parameter gathers all the arguments passed to the function into an array. Inside the function, we can treat numbers
as a regular array and perform operations on it.
By using the rest operator, we can pass any number of arguments to the sum
function, and they will be automatically gathered into the numbers
array. In this case, the function adds all the numbers together and returns the sum, which is then logged to the console.
It’s important to note that the rest operator only collects the remaining arguments into an array. If you need to pass additional parameters before the rest parameter, you still need to define them explicitly.
function multiply(multiplier, ...numbers) { let total = multiplier; for (let number of numbers) { total *= number; } return total; } console.log(multiply(2, 3, 4, 5)); // Output: 120
In the above example, the multiply
function accepts a multiplier
parameter followed by any number of additional arguments. The rest operator gathers the additional arguments into the numbers
array. The function multiplies the multiplier
with all the numbers in the array and returns the result.
The rest operator allows us to work with a variable number of function arguments. It provides a more convenient and intuitive way to handle multiple arguments compared to the traditional arguments
object. Whether you need to calculate a sum, concatenate strings, or perform any other operation on multiple values, the rest operator can simplify your code and make it more readable.
Using the Rest Operator to Destructure Arrays
To understand how the rest operator works, let’s consider an example. Suppose we have an array of numbers and we want to extract the first number and assign the remaining numbers to a new array. We can achieve this using the rest operator as follows:
const numbers = [1, 2, 3, 4, 5]; const [firstNumber, ...remainingNumbers] = numbers; console.log(firstNumber); // Output: 1 console.log(remainingNumbers); // Output: [2, 3, 4, 5]
In the code snippet above, we use array destructuring to assign the first element of the numbers
array to the firstNumber
variable. The rest of the elements are assigned to the remainingNumbers
variable using the rest operator (...
).
We can also use the rest operator to destructure arrays with a varying number of elements. For example, if we have an array with an unknown number of elements, we can use the rest operator to extract all the elements into a new array. Here’s an example:
const colors = ['red', 'green', 'blue']; const [firstColor, ...remainingColors] = colors; console.log(firstColor); // Output: 'red' console.log(remainingColors); // Output: ['green', 'blue']
In the code snippet above, we extract the first color from the colors
array using array destructuring and assign it to the firstColor
variable. The rest of the colors are assigned to the remainingColors
variable using the rest operator.
The rest operator can also be used to gather function arguments into an array. For example, if we have a function that takes a variable number of arguments, we can use the rest operator to gather all the arguments into an array. Here’s an example:
function sum(...numbers) { return numbers.reduce((total, current) => total + current, 0); } console.log(sum(1, 2, 3, 4, 5)); // Output: 15 console.log(sum(10, 20)); // Output: 30
In the code snippet above, the sum
function takes a variable number of arguments using the rest operator (...numbers
). The rest operator gathers all the arguments passed to the function into an array named numbers
. We can then use array methods like reduce
to perform operations on the gathered arguments.
The rest operator allows us to destructure arrays and gather function arguments with ease. It provides a concise and flexible way to work with arrays and handle varying numbers of elements.
Using the Rest Operator to Destructure Objects
In this section, we will explore how to use the rest operator to destructure objects. When destructuring an object with the rest operator, we can extract specific properties and assign the remaining properties to a new variable.
Let’s consider the following example:
const person = { name: 'John', age: 30, city: 'New York', country: 'USA' }; const { name, age, ...address } = person; console.log(name); // Output: John console.log(age); // Output: 30 console.log(address); // Output: { city: 'New York', country: 'USA' }
In the code snippet above, we have an object called person
with properties such as name
, age
, city
, and country
. By using the rest operator, we can extract the name
and age
properties and assign the remaining properties (city
and country
) to the address
variable.
By using the rest operator, we can easily extract specific properties from an object while keeping the remaining properties intact.
It’s important to note that the rest operator collects the remaining properties into a new object. In our example, the address
variable is an object containing the properties city
and country
. In addition to object destructuring, the rest operator can also be used with array destructuring. Let’s take a look at an example:
const numbers = [1, 2, 3, 4, 5]; const [first, second, ...rest] = numbers; console.log(first); // Output: 1 console.log(second); // Output: 2 console.log(rest); // Output: [3, 4, 5]
In the code snippet above, we have an array called numbers
with elements 1, 2, 3, 4, and 5. Using the rest operator, we can extract the first and second elements and assign the remaining elements to the rest
variable.
Similar to object destructuring, the rest operator collects the remaining elements into a new array. In our example, the rest
variable is an array containing the elements 3, 4, and 5.
The rest operator is a handy tool that simplifies the process of extracting specific elements from objects and arrays while preserving the remaining elements. It allows us to write cleaner and more concise code.
In the next section, we will explore how to use the spread operator in JavaScript.
Related Article: How to Use Closures with JavaScript
Applying the Spread and Rest Operators in Real World Examples
In this chapter, we will explore some real-world examples of how these operators can be applied to solve common programming problems.
1. Combining Arrays
One common use case for the spread operator is to combine multiple arrays into a single array. This can be done easily by using the spread operator followed by the arrays you want to combine.
const arr1 = [1, 2, 3]; const arr2 = [4, 5, 6]; const combinedArray = [...arr1, ...arr2]; console.log(combinedArray); // Output: [1, 2, 3, 4, 5, 6]
By using the spread operator, we can avoid the need for traditional methods like concat()
or manual iteration to combine arrays.
2. Copying Arrays and Objects
The spread operator is also useful for creating copies of arrays and objects. This allows us to modify the copied array or object without affecting the original.
const originalArray = [1, 2, 3]; const copiedArray = [...originalArray]; copiedArray.push(4); console.log(originalArray); // Output: [1, 2, 3] console.log(copiedArray); // Output: [1, 2, 3, 4]
Similarly, we can use the spread operator to create a shallow copy of an object.
const originalObject = { name: 'John', age: 30 }; const copiedObject = { ...originalObject, gender: 'Male' }; console.log(originalObject); // Output: { name: 'John', age: 30 } console.log(copiedObject); // Output: { name: 'John', age: 30, gender: 'Male' }
Related Article: How to Implement Functional Programming in JavaScript: A Practical Guide
3. Function Arguments
The rest operator allows us to accept a variable number of arguments in a function. It collects all the remaining arguments into an array, making it easier to work with dynamic inputs.
function sum(...numbers) { return numbers.reduce((total, num) => total + num, 0); } console.log(sum(1, 2, 3)); // Output: 6 console.log(sum(4, 5, 6, 7)); // Output: 22
In the above example, the rest operator ...numbers
collects all the arguments passed to the sum()
function into an array called numbers
. We can then use array methods like reduce()
to perform operations on the arguments.
4. Function Parameters
The spread operator can also be used to spread an array into individual arguments when calling a function. This can be useful when working with functions that expect individual arguments instead of an array.
function greet(name, age) { console.log(`Hello, ${name}! You are ${age} years old.`); } const person = ['John', 30]; greet(...person); // Output: Hello, John! You are 30 years old.
In the example above, the spread operator ...person
spreads the elements of the person
array into individual arguments for the greet()
function.
5. Object Property Shorthand
The spread operator can be used to extract specific properties from an object and create a new object with those properties.
const person = { name: 'John', age: 30, gender: 'Male', occupation: 'Developer' }; const { name, age, ...details } = person; console.log(name); // Output: John console.log(age); // Output: 30 console.log(details); // Output: { gender: 'Male', occupation: 'Developer' }
In the example above, the spread operator ...details
collects all the remaining properties of the person
object into a new object called details
, excluding the name
and age
properties.
These are just a few examples of how the spread and rest operators can be applied in real-world scenarios. By mastering these operators, you can write more concise and efficient code in JavaScript.
Related Article: How to Work with Big Data using JavaScript
Advanced Techniques: Combining Spread and Rest Operators
In this chapter, we will explore advanced techniques for combining these operators to perform complex operations.
Combining Arrays with the Spread Operator
The spread operator can be used to combine multiple arrays into a single array. Let’s consider an example:
const arr1 = [1, 2, 3]; const arr2 = [4, 5, 6]; const combined = [...arr1, ...arr2]; console.log(combined); // Output: [1, 2, 3, 4, 5, 6]
In the above code snippet, the spread operator (...
) is used to expand each array into individual elements, which are then combined into a new array called combined
. This technique is particularly useful when you need to concatenate arrays or create a shallow copy of an array.
Using Rest Parameters to Collect Arguments
The rest operator can be used to collect multiple arguments into an array. This is especially useful when you have a function that can accept a variable number of arguments. Let’s see an example:
function sum(...numbers) { return numbers.reduce((acc, curr) => acc + curr, 0); } console.log(sum(1, 2, 3)); // Output: 6 console.log(sum(4, 5, 6, 7)); // Output: 22
In the above code snippet, the rest operator (...
) is used in the function declaration to collect all the arguments passed to the sum
function into an array called numbers
. This allows us to handle any number of arguments dynamically.
Related Article: How to Use Classes in JavaScript
Combining Arrays and Rest Parameters
One interesting technique is combining the spread and rest operators to manipulate arrays. Let’s consider an example:
const arr = [1, 2, 3, 4, 5]; const [first, second, ...rest] = arr; console.log(first); // Output: 1 console.log(second); // Output: 2 console.log(rest); // Output: [3, 4, 5]
In the above code snippet, the spread operator (...
) is used to assign the first two elements of the arr
array to the variables first
and second
, while the rest operator (...
) is used to assign the remaining elements to the rest
array. This technique allows us to extract specific elements from an array and collect the remaining elements into a new array.
Advanced Techniques: Partial Application with the Rest Operator
In JavaScript, partial application is a technique that allows us to fix a number of arguments of a function, generating a new function with the remaining arguments. This technique is particularly useful when dealing with functions that accept a large number of arguments or when we want to create reusable functions.
Let’s see an example of how we can use the rest operator to implement partial application:
function multiply(...args) { return args.reduce((total, current) => total * current); } function partial(fn, ...fixedArgs) { return function(...remainingArgs) { return fn(...fixedArgs, ...remainingArgs); }; } const double = partial(multiply, 2); console.log(double(4)); // Output: 8 console.log(double(6)); // Output: 12
In the above example, we have a multiply
function that takes any number of arguments and returns their product. We also have a partial
function that accepts a function fn
and a list of fixed arguments fixedArgs
. It returns a new function that, when called, combines the fixed arguments with the remaining arguments passed to it.
We then use the partial
function to create a new function double
that multiplies a given number by 2. When we call double(4)
, it internally calls multiply(2, 4)
, resulting in the output 8. Similarly, double(6)
calls multiply(2, 6)
, resulting in the output 12.
By using the rest operator and partial application, we can easily create reusable functions with fixed arguments, which can be very handy in scenarios where we need to perform repetitive operations.
To learn more about the rest operator and its usage in JavaScript, refer to the official documentation on MDN.
Advanced Techniques: Using the Spread Operator with Strings
While it is commonly used with arrays and objects, the spread operator can also be used with strings to perform advanced operations. In this section, we will explore some of the techniques for using the spread operator with strings.
1. Concatenating Strings
One of the most common use cases for the spread operator with strings is concatenating two or more strings together. Instead of using the traditional concatenation operator (+), we can use the spread operator to achieve the same result in a more concise and efficient way. Here’s an example:
const string1 = "Hello"; const string2 = "World"; const concatenatedString = [...string1, ...string2].join(''); console.log(concatenatedString); // Output: HelloWorld
In the code above, we create two string variables, string1
and string2
. By spreading each string into an array, we can then use the join()
method to concatenate them together and obtain the desired result.
2. Splitting Strings
Another useful technique is splitting a string into an array of individual characters. This can be done easily using the spread operator along with the split()
method. Here’s an example:
const string = "Hello"; const splitString = [...string]; console.log(splitString); // Output: ['H', 'e', 'l', 'l', 'o']
In the code above, we use the spread operator to spread the characters of the string
variable into an array. As a result, each character becomes an element in the new array.
3. Reversing Strings
We can also use the spread operator to reverse a string by spreading it into an array, then using the reverse()
method. Here’s an example:
const string = "Hello"; const reversedString = [...string].reverse().join(''); console.log(reversedString); // Output: olleH
In the code above, we first spread the characters of the string
variable into an array. Then, we use the reverse()
method to reverse the order of the elements in the array. Finally, we use the join()
method to convert the reversed array back into a string.
4. Applying String Methods
The spread operator can also be used to apply string methods to each character in a string. By spreading the string into an array, we can then use array methods like map()
to apply a function to each character. Here’s an example:
const string = "Hello"; const uppercasedString = [...string].map(char => char.toUpperCase()).join(''); console.log(uppercasedString); // Output: HELLO
In the code above, we spread the characters of the string
variable into an array. Then, we use the map()
method to apply the toUpperCase()
method to each character, converting them to uppercase. Finally, we use the join()
method to convert the modified array back into a string.
These are just a few examples of the advanced techniques that can be achieved using the spread operator with strings.
Related Article: Javascript Template Literals: A String Interpolation Guide
Common Mistakes and Pitfalls to Avoid
When working with the JavaScript ES6 Spread and Rest operators, it’s important to be aware of some common mistakes and pitfalls that developers often encounter. By understanding these issues, you can avoid potential bugs and improve the quality of your code.
1. Forgetting to use the Spread Operator
One common mistake is forgetting to use the Spread operator when it’s necessary. The Spread operator allows you to expand an iterable (like an array or a string) into individual elements. This can be useful when you need to pass multiple arguments to a function or when you want to concatenate arrays.
For example, consider the following code snippet:
const numbers = [1, 2, 3]; const sum = (a, b, c) => a + b + c; // Incorrect usage without the Spread operator const result = sum(numbers); // Error: sum expects 3 arguments // Correct usage with the Spread operator const result = sum(...numbers); // Output: 6
In the incorrect usage, the sum
function expects three arguments, but we only pass the numbers
array. By using the Spread operator, we can expand the numbers
array into individual elements, allowing the function to work as expected.
2. Misusing the Rest Operator
The Rest operator, denoted by the ellipsis (...
), allows you to gather multiple function arguments into an array. However, misusing the Rest operator can lead to unexpected behavior.
One common mistake is using the Rest operator in combination with other parameters. The Rest parameter should always be the last parameter in a function declaration. For example:
// Incorrect usage const sum = (a, ...rest, b) => { console.log(a); console.log(rest); console.log(b); }; // Correct usage const sum = (a, ...rest) => { console.log(a); console.log(rest); };
In the incorrect usage, we try to use the Rest operator in combination with the parameter b
. This will result in a syntax error. To avoid this mistake, always make sure that the Rest parameter is the last parameter in your function declaration.
Related Article: High Performance JavaScript with Generators and Iterators
3. Modifying Original Arrays
Another common pitfall is modifying the original arrays when using the Spread operator. The Spread operator creates a shallow copy of the original array, which means that any modifications made to the copied array will also affect the original array.
Consider the following code snippet:
const originalArray = [1, 2, 3]; const copiedArray = [...originalArray]; copiedArray.push(4); console.log(originalArray); // Output: [1, 2, 3, 4] console.log(copiedArray); // Output: [1, 2, 3, 4]
In this example, we create a copy of the originalArray
using the Spread operator. However, when we push a new element into the copiedArray
, the originalArray
is also modified. To avoid this issue, make sure to create a deep copy of the array if you need to modify it independently.
4. Using Spread Operator on Non-Iterable Objects
It’s important to note that the Spread operator can only be used with iterable objects, such as arrays and strings. Using it on non-iterable objects, such as numbers or booleans, will result in an error.
For example:
const number = 42; const spreadNumber = [...number]; // Error: number is not iterable
In this example, we try to use the Spread operator on the number
variable, which is not iterable. This will result in a runtime error. To avoid this mistake, always ensure that you’re using the Spread operator on the correct types of objects.
By being aware of these common mistakes and pitfalls, you can effectively use the JavaScript ES6 Spread and Rest operators in your code and avoid potential bugs.