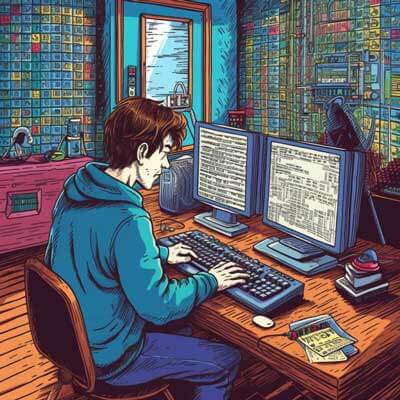
To retrieve values from GET parameters in JavaScript, you can use the URLSearchParams
object or parse the query string manually. Here are two possible approaches:
Using the URLSearchParams Object
The URLSearchParams
object provides a straightforward way to extract query parameters from a URL. It is available in modern browsers and can be used as follows:
const urlParams = new URLSearchParams(window.location.search); const myParam = urlParams.get('paramName');
In the code snippet above:
– window.location.search
returns the query string portion of the URL.
– URLSearchParams
is used to create a new instance of the URLSearchParams
object, passing the query string as the argument.
– get('paramName')
retrieves the value of the specified parameter (paramName
in this case).
If the URL contains multiple occurrences of the same parameter, get()
will return the first occurrence. To retrieve all occurrences, you can use the getAll()
method instead:
const urlParams = new URLSearchParams(window.location.search); const myParams = urlParams.getAll('paramName');
The getAll()
method returns an array with all the values of the specified parameter.
Related Article: Utilizing Debugger Inside GetInitialProps in Next.js
Manual Parsing of the Query String
If you need to support older browsers that do not have the URLSearchParams
object, you can manually parse the query string using JavaScript string manipulation methods. Here’s an example:
function getQueryParam(paramName) { const queryString = window.location.search.substr(1); const queryParams = queryString.split('&'); for (let i = 0; i < queryParams.length; i++) { const param = queryParams[i].split('='); if (param[0] === paramName) { return decodeURIComponent(param[1]); } } return null; } const myParam = getQueryParam('paramName');
In the code snippet above:
– window.location.search
is used to get the query string portion of the URL.
– substr(1)
is used to remove the leading '?' character from the query string.
– split('&')
splits the query string into an array of key-value pairs.
– The for
loop iterates over each key-value pair.
– split('=')
splits each key-value pair into separate parts.
– The if
condition checks if the parameter name matches the desired parameter.
– decodeURIComponent()
is used to decode the parameter value, handling special characters properly.
If the parameter is found, the corresponding value is returned. If the parameter is not found, null
is returned.
Best Practices and Considerations
When retrieving values from GET parameters in JavaScript, consider the following best practices:
– Always sanitize and validate the retrieved values to prevent security vulnerabilities and unexpected behavior. Avoid using the retrieved values directly in SQL queries or outputting them without proper escaping.
– If the parameter value is expected to be a number, use parseInt()
or parseFloat()
to convert it to the appropriate data type.
– If the parameter value is expected to be a boolean, use strict equality (===
) to check for specific values, such as 'true'
or 'false'
.
– If the parameter value can have multiple occurrences, use the getAll()
method or manually handle multiple values as an array.
Alternative Ideas
Apart from the approaches mentioned above, there are alternative libraries and frameworks available that can simplify working with URL parameters in JavaScript. Some popular options include:
– URI.js: A library for working with URLs and query strings, offering a more comprehensive set of features than the native URLSearchParams
object.
– query-string: A lightweight library for parsing and stringifying URL query strings, compatible with both Node.js and browsers.
Consider these alternatives if you need more advanced functionality or if you are already using these libraries in your project.
Overall, whether you choose to use the URLSearchParams
object or manually parse the query string, both approaches will allow you to retrieve values from GET parameters in JavaScript effectively.
Related Article: Using the JavaScript Fetch API for Data Retrieval