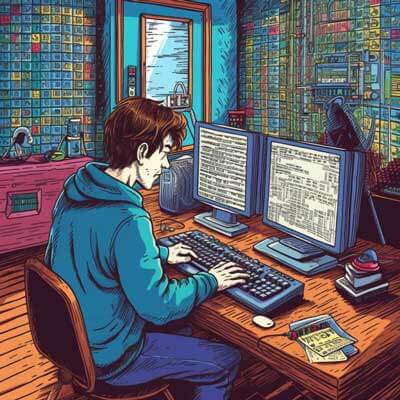
Converting an array to an object in JavaScript can be achieved using various methods. In this answer, we will explore two common approaches to convert an array to an object. Let’s dive in.
Method 1: Using the Object.fromEntries() method
The Object.fromEntries() method was introduced in ECMAScript 2019 and provides an easy way to convert an array of key-value pairs into an object. Here’s how you can use it:
const array = [['key1', 'value1'], ['key2', 'value2'], ['key3', 'value3']]; const object = Object.fromEntries(array); console.log(object);
Output:
{ key1: 'value1', key2: 'value2', key3: 'value3' }
This method takes an array of key-value pair arrays as input and returns an object with the keys and values from the array. It is important to note that the array should have an even number of elements, with the first element of each pair representing the key and the second element representing the value.
Related Article: How To Merge Arrays And Remove Duplicates In Javascript
Method 2: Using a loop to iterate over the array
Another approach to convert an array to an object is by using a loop to iterate over the array and manually constructing the object. Here’s an example:
const array = [['key1', 'value1'], ['key2', 'value2'], ['key3', 'value3']]; const object = {}; for (let [key, value] of array) { object[key] = value; } console.log(object);
Output:
{ key1: 'value1', key2: 'value2', key3: 'value3' }
In this method, we initialize an empty object and then use a loop to iterate over each element in the array. For each key-value pair, we assign the key as the object property and the value as the corresponding value.
Why the question was asked and potential reasons
The question of how to convert an array to an object in JavaScript is commonly asked because there are scenarios where it can be useful to work with data in object format rather than an array. Objects provide key-value pairs, which can be beneficial for accessing and manipulating data in a more structured manner.
One potential reason for converting an array to an object is when working with APIs that return data in array format but need to be transformed into objects for easier data manipulation. Additionally, objects provide the ability to access values using keys, which can be more intuitive and efficient than searching for values in an array.
Suggestions and alternative ideas
When converting an array to an object, it is important to consider the structure and format of the original array and how it maps to the desired object format. Depending on the specific requirements, you may need to modify the code snippets provided above to suit your needs.
If the array contains duplicate keys, it is important to consider how to handle these duplicates. One approach is to overwrite the values with the last occurrence, as demonstrated in the examples above. Alternatively, you may choose to concatenate or merge the values if duplicates are encountered.
Another alternative idea is to use the reduce() method in combination with the spread operator to convert the array to an object:
const array = [['key1', 'value1'], ['key2', 'value2'], ['key3', 'value3']]; const object = array.reduce((acc, [key, value]) => ({ ...acc, [key]: value }), {}); console.log(object);
Output:
{ key1: 'value1', key2: 'value2', key3: 'value3' }
This approach uses the reduce() method to iterate over the array and build the object by spreading the accumulated object with the new key-value pair.
Related Article: JavaScript HashMap: A Complete Guide
Best practices
When converting an array to an object, it is important to ensure that the array is in the correct format. Each element in the array should represent a key-value pair, with the key and value appropriately matched. Failure to do so may result in unexpected behavior or errors.
If you are working with large arrays, consider the performance implications of the conversion process. The Object.fromEntries() method and the reduce() method are generally more efficient than using a loop, especially when dealing with a large number of key-value pairs.
Additionally, it is good practice to handle potential errors or edge cases when converting an array to an object. For example, you may want to handle scenarios where the array is empty or contains invalid data. Adding appropriate error handling can help prevent unexpected behavior in your code.