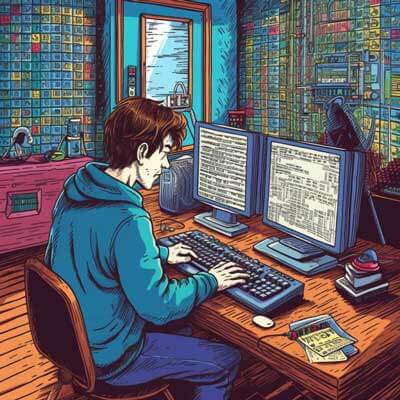
- Configuring TypeScript in a Next.js project
- Setting up TypeScript in Next.js
- Adding TypeScript to an existing Next.js project
- Benefits of using TypeScript in Next.js
- Migrating an existing Next.js project to TypeScript
- Compatibility of Next.js and TypeScript
- Using TypeScript with Next.js: Best practices
- TypeScript-specific features in Next.js
- Optimizations for TypeScript in Next.js
- Common pitfalls when using TypeScript in Next.js
- Making the most of TypeScript in Next.js
- Improving performance in Next.js with TypeScript
- Using code snippets with TypeScript in Next.js
- Testing strategies for TypeScript in Next.js
- Debugging TypeScript code in Next.js
- TypeScript and serverless functions in Next.js
- Deploying a Next.js app with TypeScript
- Tooling and resources for TypeScript in Next.js
- FAQ: Using TypeScript in Next.js
Configuring TypeScript in a Next.js project
To use TypeScript in a Next.js project, you need to configure the project to support TypeScript files (.ts and .tsx). Next.js has built-in support for TypeScript, making it easy to set up.
1. Start by creating a new Next.js project using the following command:
npx create-next-app my-app
2. Once the project is created, navigate to the project directory:
cd my-app
3. Next, install the necessary dependencies for TypeScript:
npm install --save-dev typescript @types/react @types/node
4. After the dependencies are installed, create a tsconfig.json
file in the root of your project:
touch tsconfig.json
5. Open the tsconfig.json
file and add the following configuration:
{ "compilerOptions": { "target": "es5", "module": "commonjs", "jsx": "preserve", "lib": ["dom", "dom.iterable", "esnext"], "allowJs": true, "skipLibCheck": true, "esModuleInterop": true, "allowSyntheticDefaultImports": true, "strict": true, "forceConsistentCasingInFileNames": true, "noEmit": true, "resolveJsonModule": true, "isolatedModules": true }, "include": ["next-env.d.ts", "<strong>/*.ts", "</strong>/*.tsx"], "exclude": ["node_modules"] }
6. Now, you can start using TypeScript in your Next.js project by renaming your JavaScript files to TypeScript files (.js
to .ts
or .jsx
to .tsx
).
Related Article: 25 Handy Javascript Code Snippets for Everyday Use
Setting up TypeScript in Next.js
Once you have configured TypeScript in your Next.js project, you can start using TypeScript features in your code.
1. Start by renaming your JavaScript files to TypeScript files. For example, if you have a file named index.js
, rename it to index.ts
.
2. Update the file’s content to use TypeScript syntax. For example, if you have a functional component in index.js
, you can update it to use TypeScript as follows:
import React from 'react'; const IndexPage: React.FC = () => { return ( <div> <h1>Hello, Next.js with TypeScript!</h1> </div> ); } export default IndexPage;
3. Save the file and run your Next.js project using the following command:
npm run dev
4. Now, you should see your Next.js application running with TypeScript support.
Adding TypeScript to an existing Next.js project
If you have an existing Next.js project and want to add TypeScript support, you can follow these steps:
1. Install the necessary dependencies for TypeScript:
npm install --save-dev typescript @types/react @types/node
2. Create a tsconfig.json
file in the root of your project:
touch tsconfig.json
3. Open the tsconfig.json
file and add the following configuration:
{ "compilerOptions": { "target": "es5", "module": "commonjs", "jsx": "preserve", "lib": ["dom", "dom.iterable", "esnext"], "allowJs": true, "skipLibCheck": true, "esModuleInterop": true, "allowSyntheticDefaultImports": true, "strict": true, "forceConsistentCasingInFileNames": true, "noEmit": true, "resolveJsonModule": true, "isolatedModules": true }, "include": ["next-env.d.ts", "<strong>/*.ts", "</strong>/*.tsx"], "exclude": ["node_modules"] }
4. Rename your JavaScript files to TypeScript files by changing their extensions to .ts
or .tsx
.
5. Update the content of your files to use TypeScript syntax.
6. Run your Next.js project using the following command:
npm run dev
7. Your existing Next.js project should now have TypeScript support.
Benefits of using TypeScript in Next.js
Using TypeScript in a Next.js project offers several benefits:
1. Type Safety: TypeScript adds static typing to JavaScript, allowing you to catch type-related errors at compile-time rather than runtime. This helps in reducing bugs and increasing the overall reliability of your code.
2. Better Tooling: TypeScript provides better tooling support, including autocompletion, type inference, and refactoring capabilities. This improves developer productivity and makes code maintenance easier.
3. Enhanced Collaboration: TypeScript’s type annotations act as documentation for your code, making it easier for other developers to understand and collaborate on your Next.js project.
4. Improved Code Quality: TypeScript encourages writing clean and maintainable code by enforcing strict type checks. This leads to better code quality and reduces the likelihood of introducing common programming errors.
5. Easier Refactoring: With TypeScript, refactoring becomes easier and safer. The compiler provides feedback on potential issues during refactoring, allowing you to make changes with confidence.
Related Article: Accessing Parent State from Child Components in Next.js
Migrating an existing Next.js project to TypeScript
If you have an existing Next.js project written in JavaScript and want to migrate it to TypeScript, you can follow these steps:
1. Install the necessary dependencies for TypeScript:
npm install --save-dev typescript @types/react @types/node
2. Create a tsconfig.json
file in the root of your project:
touch tsconfig.json
3. Open the tsconfig.json
file and add the following configuration:
{ "compilerOptions": { "target": "es5", "module": "commonjs", "jsx": "preserve", "lib": ["dom", "dom.iterable", "esnext"], "allowJs": true, "skipLibCheck": true, "esModuleInterop": true, "allowSyntheticDefaultImports": true, "strict": true, "forceConsistentCasingInFileNames": true, "noEmit": true, "resolveJsonModule": true, "isolatedModules": true }, "include": ["next-env.d.ts", "<strong>/*.ts", "</strong>/*.tsx"], "exclude": ["node_modules"] }
4. Rename your JavaScript files to TypeScript files by changing their extensions to .ts
or .tsx
.
5. Update the content of your files to use TypeScript syntax.
6. Run your Next.js project using the following command:
npm run dev
7. Your existing Next.js project should now be migrated to TypeScript.
Compatibility of Next.js and TypeScript
Next.js has excellent compatibility with TypeScript, making it a popular choice for developers who prefer using TypeScript in their projects. Next.js provides built-in support for TypeScript, allowing you to seamlessly integrate TypeScript into your Next.js applications.
Next.js’s compatibility with TypeScript extends to various features and functionality, including:
– Server-side rendering (SSR)
– Static site generation (SSG)
– API routes
– React components
– Routing
– Data fetching
– CSS-in-JS libraries (e.g., styled-components, Emotion)
This compatibility ensures that you can leverage the power of TypeScript while developing Next.js applications without any significant limitations or compatibility issues.
Using TypeScript with Next.js: Best practices
When using TypeScript with Next.js, it’s essential to follow best practices to ensure clean and maintainable code. Here are some best practices to consider:
1. Enable strict mode: Enable strict mode in your tsconfig.json
file ("strict": true
). This enforces strict type checking and helps catch potential errors at compile-time.
2. Use interfaces and types: Use interfaces and types to define the shape of your data and provide better type safety. This allows for better code readability and maintainability.
3. Avoid using the any
type: The any
type bypasses TypeScript’s type checking, defeating the purpose of using TypeScript. Instead, strive to specify the types explicitly to ensure type safety.
4. Leverage TypeScript’s type inference: TypeScript has excellent type inference capabilities. Take advantage of this by allowing TypeScript to infer types whenever possible, reducing the need for explicit type annotations.
5. Use generics for reusable components: Generics allow you to create reusable components that work with different types. By designing components with generics, you can ensure type safety while maintaining flexibility.
6. Utilize utility types: TypeScript provides utility types like Partial
, Pick
, and Omit
, which can simplify your code and make it more concise. Familiarize yourself with these utility types and use them when appropriate.
7. Use explicit returns in functions: TypeScript’s strict mode requires explicit return types for functions. Always specify the return type of your functions explicitly to ensure type safety.
8. Enable strict null checks: Enable strict null checks in your tsconfig.json
file ("strictNullChecks": true
). This prevents common null and undefined-related errors and promotes safer coding practices.
9. Utilize enums and discriminated unions: Enums and discriminated unions can provide better type safety and improve code readability. Use them when appropriate to represent a finite set of values or handle different variants of a type.
10. Leverage TypeScript in IDEs: Take advantage of TypeScript’s useful tooling and editor support in popular IDEs like Visual Studio Code. This includes autocompletion, type inference, and refactoring capabilities, enhancing your development experience.
Related Article: Advanced DB Queries with Nodejs, Sequelize & Knex.js
TypeScript-specific features in Next.js
Next.js provides several features specifically designed to enhance the development experience when using TypeScript:
1. Automatic TypeScript configuration: Next.js automatically configures TypeScript when you create a new Next.js project using the create-next-app
command. This saves time and reduces the setup effort.
2. TypeScript-based error reporting: Next.js uses TypeScript’s language server to provide enhanced error reporting and diagnostics. This helps catch type-related errors and provides more detailed error messages during development.
3. TypeScript support for API routes: Next.js allows you to define API routes using TypeScript, ensuring type safety for your API endpoints. You can take advantage of TypeScript’s type system to validate input and output data in your API routes.
4. TypeScript support for serverless functions: Next.js’s serverless functions can be written in TypeScript, allowing you to leverage TypeScript’s type safety and tooling when building serverless APIs.
5. TypeScript support for dynamic imports: Next.js supports dynamic imports with TypeScript, enabling you to load components and modules dynamically based on runtime conditions. TypeScript ensures type safety even with dynamically imported modules.
Optimizations for TypeScript in Next.js
Next.js provides optimizations for TypeScript-based projects to improve performance and reduce bundle sizes:
1. Incremental compilation: Next.js uses TypeScript’s incremental compilation feature to speed up subsequent builds. Only modified files are recompiled, resulting in faster build times during development.
2. Babel macros: Next.js supports Babel macros, which allow you to use TypeScript macros to generate optimized code during the build process. This can help reduce bundle sizes and improve performance.
3. Tree shaking: Next.js leverages TypeScript’s type information during the build process to perform tree shaking. This eliminates dead code and reduces the size of the final bundle.
4. Code splitting: Next.js supports code splitting, which allows you to split your code into smaller chunks. TypeScript’s type system helps identify dependencies, enabling efficient code splitting and lazy loading.
5. Static optimization: Next.js’s static optimization feature, combined with TypeScript, allows for efficient pre-rendering of static pages. This improves performance and reduces the time to first meaningful paint (TTMP).
Common pitfalls when using TypeScript in Next.js
When using TypeScript in Next.js, it’s essential to be aware of common pitfalls to avoid potential issues:
1. Incorrect type annotations: Make sure to provide accurate type annotations for your variables, functions, and components. Incorrect type annotations can lead to unexpected runtime errors.
2. Incompatible type definitions: When using third-party libraries, ensure that the type definitions are compatible with the version of the library you are using. Outdated or incorrect type definitions can cause compilation errors.
3. Overusing the any
type: Avoid overusing the any
type, as it bypasses TypeScript’s type checking. Strive to specify types explicitly to ensure type safety.
4. Ignoring TypeScript compiler errors: Don’t ignore TypeScript compiler errors. Address and fix them promptly to maintain type safety and prevent potential bugs.
5. Insufficient type coverage: Ensure that your code has sufficient type coverage. Leaving sections of your code untyped reduces the benefits of using TypeScript and can lead to unexpected errors.
Related Article: Advanced Node.js: Event Loop, Async, Buffer, Stream & More
Making the most of TypeScript in Next.js
To make the most of TypeScript in Next.js, consider the following tips:
1. Leverage TypeScript’s type inference: TypeScript has excellent type inference capabilities. Utilize them to reduce the need for explicit type annotations and make your code more concise.
2. Utilize advanced TypeScript features: Familiarize yourself with advanced TypeScript features like conditional types, mapped types, and intersection types. These features can help you write more expressive and useful code.
3. Use generics for reusable components and functions: Generics allow you to create reusable components and functions that work with different types. Take advantage of generics to write flexible and type-safe code.
4. Use TypeScript’s non-null assertion operator (!
) sparingly: The non-null assertion operator (!
) tells TypeScript that a value is not null or undefined. Use it sparingly and only when you are certain about the value’s non-nullability.
5. Take advantage of TypeScript’s tooling: TypeScript provides useful tooling and editor support. Familiarize yourself with features like autocompletion, type inference, and refactoring capabilities to enhance your development experience.
Improving performance in Next.js with TypeScript
To improve performance in a Next.js project with TypeScript, consider the following tips:
1. Enable strict mode in TypeScript: Enabling strict mode ("strict": true
) in your tsconfig.json
file helps catch potential errors at compile-time and promotes better code quality.
2. Optimize your TypeScript code: Write efficient and performant TypeScript code by following best practices, like minimizing unnecessary type checks, avoiding excessive object creation, and optimizing algorithms.
3. Utilize Next.js optimizations: Leverage Next.js’s built-in optimizations, such as incremental compilation, Babel macros, tree shaking, and code splitting. These optimizations can help reduce bundle sizes and improve performance.
4. Profile and optimize performance bottlenecks: Use performance profiling tools like the Chrome DevTools Performance tab to identify and optimize performance bottlenecks in your Next.js application.
5. Implement server-side rendering (SSR) and static site generation (SSG) where appropriate: SSR and SSG can significantly improve the performance of your Next.js application by pre-rendering pages on the server or at build time.
Using code snippets with TypeScript in Next.js
When working with TypeScript in Next.js, you can use code snippets to enhance your productivity. Here are a few examples of code snippets that you can use:
1. React component with TypeScript:
import React from 'react'; interface Props { name: string; } const MyComponent: React.FC = ({ name }) => { return <h1>Hello, {name}!</h1>; }; export default MyComponent;
2. API route with TypeScript:
import { NextApiRequest, NextApiResponse } from 'next'; interface Data { message: string; } export default function handler( req: NextApiRequest, res: NextApiResponse ) { res.status(200).json({ message: 'Hello, World!' }); }
Related Article: AI Implementations in Node.js with TensorFlow.js and NLP
Testing strategies for TypeScript in Next.js
When it comes to testing TypeScript code in Next.js, you can follow the same testing strategies as you would for regular TypeScript projects. Here are some common testing strategies:
1. Unit Testing: Write unit tests for individual functions, components, and modules using a testing framework like Jest. Utilize TypeScript’s type system to provide type-safe mocks and assertions.
2. Integration Testing: Perform integration tests to ensure that different parts of your Next.js application work together correctly. Use tools like Jest, Testing Library, or Cypress to write integration tests that cover multiple components, API routes, or pages.
3. Snapshot Testing: Use Jest’s snapshot testing feature to capture the output of a component or a data structure and compare it against a stored snapshot. This helps catch unexpected changes in the output and ensures consistent behavior.
4. End-to-End Testing: Conduct end-to-end tests to simulate user interactions and test the entire application flow. Tools like Cypress or Selenium can be used to write end-to-end tests for your Next.js application.
5. Mocking APIs: When testing components or modules that interact with external APIs, use mocking libraries like msw
or nock
to mock API responses. TypeScript’s type system can help ensure that the mocked responses match the expected types.
Debugging TypeScript code in Next.js
When debugging TypeScript code in Next.js, you can use the built-in debugging capabilities of your IDE or browser developer tools. Here are some debugging tips:
1. Use breakpoints: Set breakpoints in your TypeScript code to pause the execution at specific lines. This allows you to inspect variables, step through the code, and identify issues.
2. Utilize console.log: Insert console.log statements in your code to log values and debug information. TypeScript’s type system helps ensure that you log the correct types, reducing the likelihood of type-related errors.
3. Take advantage of TypeScript’s type inference: TypeScript’s type inference can help you identify potential issues during debugging. Pay attention to the inferred types and check if they match your expectations.
4. Inspect TypeScript compiler errors: When encountering TypeScript compiler errors, use your IDE’s error panel or the command line output to understand the error messages. TypeScript’s error messages often provide valuable insights into the root causes of issues.
TypeScript and serverless functions in Next.js
Next.js provides excellent support for serverless functions written in TypeScript. Serverless functions allow you to create API endpoints that run in a serverless environment, enabling scalable and cost-effective backend functionality.
To create a serverless function in Next.js with TypeScript:
1. Create a TypeScript file with a .ts
or .tsx
extension in the pages/api
directory.
2. In the file, export a default function that takes two arguments: req
(NextApiRequest) and res
(NextApiResponse).
3. Use TypeScript’s type annotations to specify the request and response types.
4. Write the logic for your serverless function inside the exported function.
Here’s an example of a serverless function written in TypeScript:
import { NextApiRequest, NextApiResponse } from 'next'; interface Data { message: string; } export default function handler( req: NextApiRequest, res: NextApiResponse ) { const { name } = req.query; res.status(200).json({ message: `Hello, ${name}!` }); }
This serverless function expects a name
query parameter and returns a JSON response with a greeting message.
Related Article: AngularJS: Check if a Field Contains a MatFormFieldControl
Deploying a Next.js app with TypeScript
Deploying a Next.js app with TypeScript is similar to deploying a regular Next.js app. Here are the general steps to deploy a Next.js app with TypeScript:
1. Build your Next.js app for production using the following command:
npm run build
2. Next, start the server in production mode:
npm start
3. Depending on your deployment environment, you can use various hosting platforms or services to deploy your Next.js app with TypeScript. Some popular options include:
– Vercel: Vercel is the creator of Next.js and provides an excellent platform for deploying Next.js apps. It has built-in support for TypeScript and offers seamless deployment workflows.
– AWS Amplify: AWS Amplify is a cloud-based service that simplifies the deployment of web applications. It supports Next.js apps with TypeScript and provides various deployment options.
– Heroku: Heroku is a platform as a service (PaaS) that supports Next.js apps. It allows you to deploy your Next.js app with TypeScript using a straightforward deployment process.
– Netlify: Netlify is a popular hosting platform for static websites and JAMstack applications. It supports Next.js apps with TypeScript and provides easy deployment and continuous integration workflows.
Tooling and resources for TypeScript in Next.js
When working with TypeScript in Next.js, you can leverage various tools and resources to enhance your development experience. Here are some useful tools and resources:
1. TypeScript: TypeScript is the primary tool for working with TypeScript in Next.js. It provides static typing and other language features that improve code quality and maintainability.
2. TypeScript ESLint: Use TypeScript ESLint to lint your TypeScript code and enforce coding standards. It provides rules specific to TypeScript and integrates well with Next.js projects.
3. Prettier: Prettier is a code formatter that helps maintain consistent code style. Use it in combination with TypeScript to format your code automatically.
4. DefinitelyTyped: DefinitelyTyped is a repository of TypeScript type definitions for popular JavaScript libraries. It provides type definitions for many Next.js dependencies, allowing you to use them with TypeScript.
5. TypeScript Handbook: The TypeScript Handbook is an official guide that covers all aspects of TypeScript. It provides detailed explanations, examples, and best practices for using TypeScript effectively.
6. Next.js TypeScript example: Next.js provides an official TypeScript example that demonstrates how to use TypeScript in a Next.js project. You can refer to this example for guidance and inspiration.
FAQ: Using TypeScript in Next.js
Q: Can I use JavaScript libraries with TypeScript in Next.js?
A: Yes, you can use JavaScript libraries with TypeScript in Next.js. TypeScript supports type definitions for popular JavaScript libraries, allowing you to use them seamlessly in your Next.js projects.
Q: Do I need to use TypeScript in Next.js?
A: No, using TypeScript in Next.js is optional. Next.js supports both JavaScript and TypeScript, allowing you to choose the language that best suits your needs.
Q: Can I mix JavaScript and TypeScript files in a Next.js project?
A: Yes, you can mix JavaScript and TypeScript files in a Next.js project. Next.js supports both languages and can handle mixed projects without any issues.
Q: Are there any performance differences between using JavaScript and TypeScript in Next.js?
A: When properly configured, there should be no significant performance differences between using JavaScript and TypeScript in Next.js. TypeScript’s type checking is done during the build process and does not impact runtime performance.
Q: Can I use Next.js-specific features with TypeScript?
A: Yes, Next.js provides excellent TypeScript support for its features, including serverless functions, API routes, static site generation, and more. You can leverage TypeScript’s type system and tooling to enhance your Next.js development experience.
Q: Does Next.js support hot reloading with TypeScript?
A: Yes, Next.js supports hot reloading with TypeScript. When you make changes to your TypeScript code, Next.js will automatically rebuild and reload the application to reflect the changes.
Q: Can I use TypeScript decorators with Next.js?
A: Yes, you can use TypeScript decorators with Next.js. TypeScript decorators provide a way to modify the behavior of classes, methods, or properties. However, decorators are an experimental feature in TypeScript, so use them with caution.
Q: Can I use TypeScript in the client-side code of my Next.js app?
A: Yes, you can use TypeScript in the client-side code of your Next.js app. TypeScript provides excellent support for writing browser-compatible code and can be used for both server-side and client-side development in Next.js.
Q: Can I use TypeScript namespaces in Next.js?
A: TypeScript namespaces are an older way of organizing code and have been largely replaced by modules. While TypeScript still supports namespaces, it is recommended to use modules for code organization in Next.js.
Q: How does TypeScript impact the build size of a Next.js app?
A: TypeScript type information is removed during the build process, so it does not significantly impact the build size of a Next.js app. However, if you use complex type annotations or excessive type declarations, it may slightly increase the build size.
Q: Can I use Next.js with TypeScript in a monorepo setup?
A: Yes, you can use Next.js with TypeScript in a monorepo setup. Next.js provides built-in support for monorepo setups, allowing you to share TypeScript code between multiple Next.js projects or packages.
Q: Does Next.js have a TypeScript template for creating new projects?
A: Yes, Next.js provides a TypeScript template for creating new projects. You can use the create-next-app
command with the --typescript
flag to create a new Next.js project with TypeScript support.
Q: Does Next.js support type checking during development with TypeScript?
A: Yes, Next.js supports type checking during development with TypeScript. When you run the Next.js development server (npm run dev
), TypeScript performs type checking and displays any type errors or warnings in the console.
Q: Can I use TypeScript in Next.js without using JSX?
A: Yes, you can use TypeScript in Next.js without using JSX. TypeScript supports both JSX and regular TypeScript syntax, allowing you to choose the one that best fits your project’s requirements.
Q: Does Next.js support TypeScript’s experimental features?
A: Next.js supports TypeScript’s experimental features as long as they are enabled in your tsconfig.json
file. However, it is recommended to use experimental features with caution and be aware of their potential limitations or instability.
Q: Can I use TypeScript’s strict mode with Next.js?
A: Yes, you can use TypeScript’s strict mode with Next.js. Enabling strict mode ("strict": true
) in your tsconfig.json
file helps catch potential errors at compile-time and promotes better code quality.