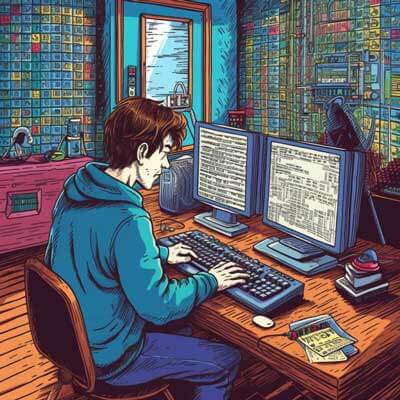
- How to Wait for an Async Call in JavaScript
- Using async/await
- Using Promises
- Using Callbacks
- Additional Questions
- What is the difference between async/await and Promises?
- How can I handle errors in async/await?
- What is the purpose of a callback function in JavaScript?
- How can I make an async call using the fetch API?
- What is the difference between fetch and axios in JavaScript?
- How can I delay execution in JavaScript?
- What is XMLHttpRequest in JavaScript?
- What is AJAX and how is it related to async calls?
- What is the event loop in JavaScript?
- How does JavaScript handle concurrency in async calls?
- Additional Resources
How to Wait for an Async Call in JavaScript
In JavaScript, asynchronous calls are a common occurrence when working with APIs, databases, or performing any non-blocking operations. These calls allow the program to continue execution while waiting for the response from the asynchronous operation. However, there are situations where you need to wait for the async call to complete before proceeding with the next steps in your code. In this article, we will explore various methods to wait for an async call in JavaScript.
Related Article: How To Generate Random String Characters In Javascript
Using async/await
One of the most straightforward ways to wait for an async call in JavaScript is by using the async/await syntax. Async/await is a feature introduced in ECMAScript 2017 that allows you to write asynchronous code in a more synchronous manner.
Here’s how you can use async/await to wait for an async call:
async function fetchData() { const response = await fetch('https://api.example.com/data'); const data = await response.json(); return data; } async function processAsyncCall() { try { const result = await fetchData(); console.log(result); // continue with other code that depends on the async call } catch (error) { console.error(error); } } processAsyncCall();
In the example above, the fetchData
function makes an async call to an API using the fetch
function. The await
keyword is used to pause the execution of the function until the async call is complete and the result is returned. Once the result is obtained, you can continue with the rest of your code.
Using async/await not only allows you to wait for the async call to complete but also provides a convenient way to handle errors using a try-catch block.
Using Promises
Another way to wait for an async call in JavaScript is by using Promises. Promises are objects that represent the eventual completion or failure of an asynchronous operation and provide a way to handle the result asynchronously.
Here’s an example of how you can use Promises to wait for an async call:
function fetchData() { return new Promise((resolve, reject) => { fetch('https://api.example.com/data') .then(response => response.json()) .then(data => resolve(data)) .catch(error => reject(error)); }); } fetchData() .then(result => { console.log(result); // continue with other code that depends on the async call }) .catch(error => { console.error(error); });
In the example above, the fetchData
function returns a Promise that wraps the async call to the API. The resolve
function is called when the async call is successful, and the reject
function is called when there is an error. By chaining the then
method, you can handle the result of the async call and continue with the rest of your code.
Using Promises is a more traditional way to handle async calls in JavaScript and is widely supported in older versions of the language.
Using Callbacks
Before the advent of Promises and async/await, callbacks were the primary way to handle asynchronous operations in JavaScript. A callback is a function that is passed as an argument to another function and is executed once the async operation is complete.
Here’s an example of how you can use callbacks to wait for an async call:
function fetchData(callback) { fetch('https://api.example.com/data') .then(response => response.json()) .then(data => callback(null, data)) .catch(error => callback(error, null)); } fetchData((error, result) => { if (error) { console.error(error); } else { console.log(result); // continue with other code that depends on the async call } });
In the example above, the fetchData
function accepts a callback function as an argument. Inside the function, the async call to the API is made using the fetch
function. If the call is successful, the callback is invoked with the result. If there is an error, the callback is invoked with the error.
Using callbacks can be cumbersome, especially when dealing with multiple async calls or complex error handling. However, it is still widely used in older JavaScript codebases and libraries.
Related Article: Understanding JavaScript Execution Context and Hoisting
Additional Questions
What is the difference between async/await and Promises?
Async/await and Promises are both mechanisms for handling asynchronous operations in JavaScript. However, there are some key differences between the two:
– Syntax: Async/await provides a more synchronous-like syntax, allowing you to write asynchronous code that looks similar to synchronous code. Promises, on the other hand, use a chain of then
and catch
methods to handle the result of an asynchronous operation.
– Error Handling: With async/await, you can use a try-catch block to handle errors, making the code more readable and easier to debug. Promises handle errors using the catch
method, which requires you to chain it after each then
method.
– Readability: Async/await code tends to be more readable and easier to understand, especially when dealing with complex async operations and multiple async calls. Promises can become nested and harder to follow, leading to what is commonly known as “callback hell”.
– Compatibility: Async/await was introduced in ECMAScript 2017 and may not be supported in older browsers or environments that do not have modern JavaScript features. Promises, on the other hand, have been around for longer and are widely supported.
How can I handle errors in async/await?
Handling errors in async/await is similar to handling errors in synchronous code. You can use a try-catch block to catch any errors that occur during the execution of the async call.
Here’s an example:
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); return data; } catch (error) { console.error(error); throw error; } } try { const result = await fetchData(); console.log(result); // continue with other code that depends on the async call } catch (error) { console.error(error); }
In the example above, the async function fetchData
uses a try-catch block to catch any errors that occur during the async call. If an error is caught, it is logged to the console and re-thrown to be caught by the outer try-catch block.
It is important to handle errors appropriately when using async/await to prevent uncaught errors and ensure that your code behaves as expected.
Related Article: How to Use Closures with JavaScript
What is the purpose of a callback function in JavaScript?
A callback function in JavaScript is a function that is passed as an argument to another function and is executed once a certain condition is met, usually after an asynchronous operation has completed.
The purpose of a callback function is to allow you to define what should happen after an async operation is complete. It provides a way to handle the result or error of the async operation without blocking the execution of the program.
Callback functions are commonly used in JavaScript to handle async operations such as API calls, database queries, or file system operations. They allow you to perform actions like updating the UI, processing the data, or triggering other functions once the async operation is complete.
Here’s an example of a callback function:
function fetchData(callback) { // simulate an async operation setTimeout(() => { const data = { name: 'John', age: 30 }; callback(data); }, 1000); } function processResult(result) { console.log(result); } fetchData(processResult);
In the example above, the fetchData
function simulates an async operation using setTimeout
. Once the operation is complete, it calls the callback function processResult
with the result.
Callbacks can be useful when used correctly, but they can also lead to callback hell and make code harder to read and maintain. Promises and async/await are newer and more elegant ways to handle async operations in JavaScript.
How can I make an async call using the fetch API?
The fetch API is a modern JavaScript API that provides a built-in way to make HTTP requests. It supports both synchronous and asynchronous operations and returns a Promise that resolves to the response from the server.
Here’s an example of how you can make an async call using the fetch API:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { console.log(data); // continue with other code that depends on the async call }) .catch(error => { console.error(error); });
In the example above, the fetch
function is called with the URL of the API as an argument. The returned Promise is then chained with the then
method to handle the response and parse it as JSON. Finally, the result is logged to the console, and any errors are caught using the catch
method.
The fetch API provides a useful and flexible way to make async calls in JavaScript and is supported in all modern browsers.
What is the difference between fetch and axios in JavaScript?
Fetch and Axios are both popular libraries used for making HTTP requests in JavaScript. While they serve the same purpose, there are some differences between the two:
– Syntax: Fetch is a built-in browser API and uses a chain of then
methods to handle the response and parse it. Axios, on the other hand, uses a more traditional approach with a callback-based syntax and supports both browser and Node.js environments.
– Browser Support: Fetch is a newer API and may not be supported in older browsers or environments that do not have modern JavaScript features. Axios, on the other hand, is a standalone library that can be used in any browser or environment.
– Error Handling: Fetch handles errors using the catch
method, while Axios provides a built-in way to handle errors using the catch
method as well as interceptors for global error handling.
– Flexibility: Axios provides additional features such as request cancellation, automatic JSON parsing, and support for sending data in different formats like URL-encoded or multipart form data. Fetch, on the other hand, requires manual handling of these features.
Both Fetch and Axios have their own strengths and weaknesses, and the choice between the two depends on the specific needs of your project. If you are targeting modern browsers or environments that support Fetch, it can be a lightweight and efficient option. If you need more features and flexibility, Axios might be a better choice.
Related Article: How to Implement Functional Programming in JavaScript: A Practical Guide
How can I delay execution in JavaScript?
There are several ways to delay the execution of code in JavaScript. Here are a few examples:
– Using setTimeout: The setTimeout function allows you to delay the execution of a function by a specified number of milliseconds. Here’s an example:
console.log('Before delay'); setTimeout(() => { console.log('Delayed execution'); }, 2000); console.log('After delay');
In this example, the function inside setTimeout is executed after a delay of 2000 milliseconds (2 seconds), while the code outside setTimeout continues to execute immediately.
– Using setInterval: The setInterval function is similar to setTimeout but repeatedly executes the specified function with a fixed time delay between each execution. Here’s an example:
let count = 0; const intervalId = setInterval(() => { console.log('Execution', count); count++; if (count === 5) { clearInterval(intervalId); } }, 1000);
In this example, the function inside setInterval is executed every 1000 milliseconds (1 second) until the count reaches 5. clearInterval is used to stop the execution of the interval.
– Using async/await and setTimeout: If you are using async/await, you can delay the execution of code by wrapping the setTimeout function in a Promise. Here’s an example:
function delay(ms) { return new Promise(resolve => setTimeout(resolve, ms)); } async function delayedExecution() { console.log('Before delay'); await delay(2000); console.log('Delayed execution'); } delayedExecution();
In this example, the delay function returns a Promise that resolves after a specified number of milliseconds. The await keyword is used to pause the execution of the delayedExecution function until the delay is complete.
These are just a few examples of how you can delay the execution of code in JavaScript. The method you choose depends on the specific requirements of your code and the context in which it is used.
What is XMLHttpRequest in JavaScript?
XMLHttpRequest is a built-in JavaScript object that provides an easy way to make HTTP requests from the browser. It was the primary method for making AJAX requests before the fetch API was introduced.
Here’s an example of how you can use XMLHttpRequest to make an async call:
const xhr = new XMLHttpRequest(); xhr.open('GET', 'https://api.example.com/data'); xhr.onreadystatechange = function() { if (xhr.readyState === 4 && xhr.status === 200) { const data = JSON.parse(xhr.responseText); console.log(data); // continue with other code that depends on the async call } }; xhr.send();
In this example, the XMLHttpRequest object is created using the new XMLHttpRequest()
constructor. The open
method is used to specify the HTTP method and the URL of the API. The onreadystatechange
event handler is set to handle the response when the readyState of the request changes to 4 (which means the request is complete) and the status is 200 (which means the request was successful). Finally, the send
method is called to send the request.
XMLHttpRequest provides a lower-level API compared to fetch and allows for more fine-grained control over the request and response. However, it requires more boilerplate code and can be less intuitive to use.
What is AJAX and how is it related to async calls?
AJAX stands for Asynchronous JavaScript and XML and is a technique used to make async requests from a web page without reloading the entire page. It allows for the retrieval of data from a server in the background and the update of parts of the web page without interrupting the user’s interaction.
AJAX is closely related to async calls as it is the foundation of making async requests in JavaScript. It uses techniques such as XMLHttpRequest or the fetch API to make async calls to a server and retrieve data.
Here’s an example of how you can use AJAX to make an async call:
function fetchData() { const xhr = new XMLHttpRequest(); xhr.open('GET', 'https://api.example.com/data'); xhr.onreadystatechange = function() { if (xhr.readyState === 4 && xhr.status === 200) { const data = JSON.parse(xhr.responseText); console.log(data); // continue with other code that depends on the async call } }; xhr.send(); } fetchData();
In this example, the fetchData
function uses XMLHttpRequest to make an async call to an API and retrieve data. The onreadystatechange
event handler is set to handle the response when the readyState of the request changes to 4 and the status is 200. The retrieved data is then logged to the console.
AJAX is commonly used in web development to create dynamic and interactive web pages that can update data without requiring a page reload. It has been widely adopted and has become an essential part of modern web development.
Related Article: How to Work with Big Data using JavaScript
What is the event loop in JavaScript?
The event loop is a fundamental concept in JavaScript that handles the execution of asynchronous code. It is responsible for managing the execution of tasks in a non-blocking manner and ensuring that the program remains responsive.
In JavaScript, the event loop works by continuously checking for tasks in the event queue and executing them one by one. When an async operation, such as a setTimeout or an AJAX request, is encountered, it is added to the event queue along with a callback function. Once the current task is complete, the event loop picks the next task from the event queue and executes it.
Here’s a simplified example of how the event loop works:
console.log('First'); setTimeout(() => { console.log('Second'); }, 0); console.log('Third');
In this example, the first console.log statement is executed immediately. The setTimeout function is then called with a delay of 0 milliseconds, which means it will be added to the event queue immediately. Finally, the third console.log statement is executed.
The event loop ensures that the setTimeout callback is not executed immediately, even though the delay is set to 0. Instead, it waits for the current task to complete before picking the next task from the event queue, allowing the program to remain responsive.
Understanding the event loop is crucial for writing efficient and responsive JavaScript code, especially when dealing with async operations. It helps prevent blocking the main thread and allows the program to handle multiple tasks concurrently.
How does JavaScript handle concurrency in async calls?
JavaScript is a single-threaded language, which means it can only execute one task at a time. However, when it comes to async calls, JavaScript handles concurrency using an event-driven model and the event loop.
When an async call is made, such as a setTimeout or an AJAX request, JavaScript registers the callback function associated with the call and continues executing the next task. The async operation is then performed in the background by the browser or the JavaScript runtime, and once it is complete, the callback function is added to the event queue.
The event loop continuously checks for tasks in the event queue and executes them one by one. This allows multiple async calls to be processed concurrently, even though JavaScript itself is single-threaded.
Here’s an example to illustrate how JavaScript handles concurrency:
setTimeout(() => { console.log('Async task 1'); }, 1000); setTimeout(() => { console.log('Async task 2'); }, 500); console.log('Synchronous task');
In this example, two setTimeout calls are made with different delays. The synchronous task is executed immediately, while the async tasks are added to the event queue with their respective callback functions. Once the delays are complete, the callback functions are executed, even though they were registered after the synchronous task.
JavaScript achieves concurrency in async calls by leveraging the event loop and the non-blocking nature of async operations. This allows for efficient execution of multiple tasks and ensures that the program remains responsive even when performing time-consuming operations.
Additional Resources
– Difference between async/await and Promises
– Handling errors in async/await
– Purpose of a callback function in JavaScript
– Making an async call using the fetch API