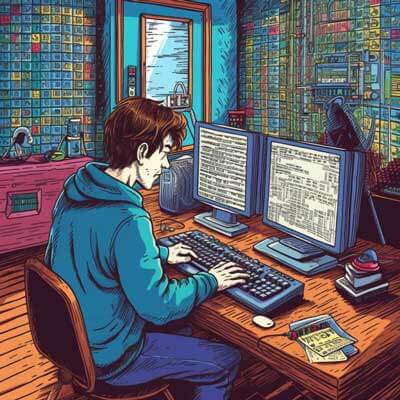
To set the checked state of a checkbox using jQuery, you can use the prop()
method or the attr()
method. Here are two possible approaches:
Using the prop() method
The prop()
method allows you to manipulate properties of elements. To set the checked state of a checkbox, you can use the prop()
method with the checked
property. Here’s an example:
$('#checkboxId').prop('checked', true);
In this example, #checkboxId
is the ID of the checkbox element you want to set as checked. The prop()
method is called on the checkbox element and the checked
property is set to true
.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Using the attr() method
Alternatively, you can use the attr()
method to set the checked state of a checkbox. Here’s an example:
$('#checkboxId').attr('checked', 'checked');
In this example, #checkboxId
is the ID of the checkbox element you want to set as checked. The attr()
method is called on the checkbox element and the checked
attribute is set to 'checked'
.
It’s worth noting that the attr()
method sets the attribute value directly, while the prop()
method sets the property value. In most cases, using the prop()
method is recommended for setting the checked state of a checkbox.
Best practices
When setting the checked state of a checkbox with jQuery, it’s important to follow best practices. Here are a few suggestions:
1. Use unique IDs: Ensure that each checkbox element has a unique ID. This allows you to target specific checkboxes using jQuery selectors without conflicts.
2. Use proper event handling: If you’re setting the checked state in response to a user action, such as a button click, make sure to handle the event appropriately. You can use event delegation or attach event handlers to the checkbox element directly.
3. Consider using a CSS class: If you have multiple checkboxes that need to be set as checked, you can add a CSS class to those checkboxes and use a single jQuery selector to set the checked state for all of them.
4. Use the change event: If you’re setting the checked state based on user input, consider using the change
event to handle the changes. This allows you to respond to changes in the checkbox state in a more reliable and consistent manner.
Here’s an example that demonstrates setting the checked state of multiple checkboxes using a CSS class and the change event:
$('.checkboxClass').on('change', function() { if ($(this).is(':checked')) { $(this).prop('checked', true); } else { $(this).prop('checked', false); } });
In this example, .checkboxClass
is the CSS class added to the checkboxes that need to be set as checked. The change
event is attached to all checkboxes with the .checkboxClass
. When a checkbox’s state changes, the event handler checks if the checkbox is checked and sets the checked
property accordingly.
Overall, setting the checked state of a checkbox with jQuery is straightforward using either the prop()
method or the attr()
method. Following best practices ensures reliable and consistent behavior across different scenarios.
Related Article: How to Use the forEach Loop with JavaScript