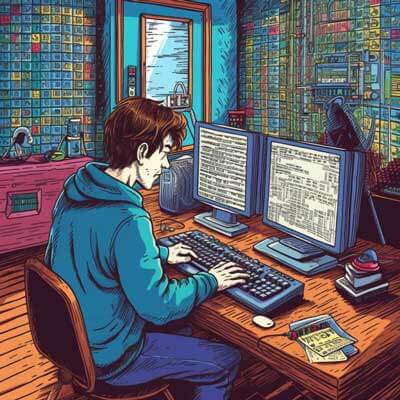
- Setting up a Basic Next.js Project
- Understanding React Components
- Creating a Basic Navbar Component
- Styling a Navbar with CSS
- Integrating Bootstrap into Next.js
- Adding HTML Markup to the Navbar
- Making the Navbar Responsive
- Implementing a Mobile Navigation Menu
- Customizing the Appearance of the Navbar
- Applying UI Design Principles to the Navbar
- Adding Dropdown Menus to the Navbar
- Best Practices for Designing a Navigation Menu
- Creating a Sticky Navbar in Next.js
- Animating the Navbar with JavaScript
- Additional Resources
Setting up a Basic Next.js Project
To create a navbar in Next.js, we first need to set up a basic Next.js project. Next.js is a popular JavaScript framework for building server-rendered React applications. It provides a simple and intuitive way to create dynamic web pages with server-side rendering capabilities.
To get started, make sure you have Node.js and npm installed on your machine. Then, open your terminal and run the following command to create a new Next.js project:
npx create-next-app my-navbar-app
This command will create a new directory called my-navbar-app
and set up a basic Next.js project inside it. Once the command finishes running, navigate to the project directory by running:
cd my-navbar-app
Now, you can start the development server by running the following command:
npm run dev
This will start the server and make your Next.js application accessible at http://localhost:3000
. You should see a basic Next.js page with the Next.js logo.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Understanding React Components
Before we dive into creating a navbar component in Next.js, it’s important to understand the basics of React components. React components are the building blocks of a React application. They are reusable and self-contained pieces of code that encapsulate the UI and behavior of a specific part of the application.
In Next.js, components are typically defined as JavaScript functions or classes. Function components are the simplest form of components and are recommended for most use cases. Class components are more useful and offer additional features like lifecycle methods and access to the component’s state.
Let’s create a simple function component called Navbar
that will serve as the foundation for our navbar component. Open the components
directory in your Next.js project and create a new file called Navbar.js
. In this file, add the following code:
import React from 'react'; const Navbar = () => { return ( <nav> <ul> <li>Home</li> <li>About</li> <li>Contact</li> </ul> </nav> ); }; export default Navbar;
In this code, we define a function component called Navbar
that returns a JSX element representing the navbar. The navbar is structured as an unordered list (<ul>
) with three list items (<li>
) representing the different navigation links. We’ll style this navbar later on.
Creating a Basic Navbar Component
Now that we have a basic understanding of React components, let’s create a basic navbar component in Next.js. We’ll start by importing the Navbar
component we just created into the pages/index.js
file.
Open the pages/index.js
file in your Next.js project and replace its contents with the following code:
import React from 'react'; import Navbar from '../components/Navbar'; const Home = () => { return ( <div> <h1>Welcome to my Next.js Navbar Tutorial</h1> <p>This is a basic example of how to create a navbar in Next.js using JavaScript.</p> </div> ); }; export default Home;
In this code, we import the Navbar
component from the components/Navbar
file and render it inside the Home
component. We also add a heading and a paragraph to give some context to the page.
If you save the file and refresh your browser, you should now see the navbar rendered at the top of the page, followed by the heading and paragraph.
Styling a Navbar with CSS
Now that we have a basic navbar component, let’s add some CSS styles to make it visually appealing. Next.js allows us to import CSS files directly into our components, making it easy to apply styles.
Inside the components
directory, create a new file called Navbar.module.css
. In this file, add the following CSS code:
.navbar { background-color: #333; color: #fff; padding: 10px; } .navbar ul { list-style-type: none; display: flex; justify-content: space-between; margin: 0; padding: 0; } .navbar li { margin-right: 10px; } .navbar li:last-child { margin-right: 0; } .navbar a { color: #fff; text-decoration: none; } .navbar a:hover { text-decoration: underline; }
In this CSS code, we define styles for the navbar component. The .navbar
class sets the background color, text color, and padding of the navbar. The .navbar ul
class styles the unordered list inside the navbar, making it a flex container with space between the items. The .navbar li
class styles the list items, adding some margin between them. The .navbar a
class styles the anchor tags inside the list items, setting the color and removing the default underline. The .navbar a:hover
class styles the anchor tags when hovered, adding an underline.
Now, go back to the Navbar.js
file and import the CSS file by adding the following line at the top of the file:
import styles from './Navbar.module.css';
Then, update the JSX code inside the Navbar
component to use the CSS classes:
const Navbar = () => { return ( <nav> <ul> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Contact</a></li> </ul> </nav> ); };
In this code, we use the className
prop to assign the navbar
class from the imported CSS module to the <nav>
element. This will apply the styles defined in the CSS file to the navbar component.
If you save the file and refresh your browser, you should now see the navbar with the applied styles.
Related Article: How to Use the forEach Loop with JavaScript
Integrating Bootstrap into Next.js
Bootstrap is a popular CSS framework that provides a set of pre-styled components and utilities for building responsive web pages. Integrating Bootstrap into Next.js is straightforward and can be done by installing the bootstrap
package and importing the required CSS files.
To get started, open your terminal and navigate to your Next.js project directory. Then, run the following command to install Bootstrap as a dependency:
npm install bootstrap
Once the installation is complete, open the pages/_app.js
file in your Next.js project. This file is responsible for initializing the Next.js application and is a great place to import global CSS files.
Add the following line at the top of the file to import the Bootstrap CSS:
import 'bootstrap/dist/css/bootstrap.min.css';
This will import the compiled Bootstrap CSS file into your Next.js application, allowing you to use Bootstrap classes and styles.
Now, you can leverage Bootstrap’s classes and components to enhance your navbar. For example, you can replace the unordered list in the Navbar
component with a Bootstrap navbar component:
import React from 'react'; import Link from 'next/link'; const Navbar = () => { return ( <nav> <a>My Navbar</a> <button type="button" data-toggle="collapse" data-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation"> <span></span> </button> <div id="navbarNav"> <ul> <li> <a>Home</a> </li> <li> <a>About</a> </li> <li> <a>Contact</a> </li> </ul> </div> </nav> ); }; export default Navbar;
In this code, we import the Link
component from the next/link
module to create client-side navigation links. We replace the <ul>
element with a Bootstrap navbar-nav
component and use the navbar-brand
class for the navbar brand. We also add a collapsible navbar functionality using Bootstrap’s navbar-toggler
component and the collapse
class.
If you save the file and refresh your browser, you should now see an enhanced navbar with Bootstrap styles and functionality.
Adding HTML Markup to the Navbar
In addition to navigation links, a navbar often contains other HTML elements like logos, buttons, or search bars. In this section, we’ll add a search bar to our navbar component.
First, create a new file called SearchBar.js
inside the components
directory. In this file, add the following code:
import React from 'react'; const SearchBar = () => { return ( <button type="submit"> Search </button> ); }; export default SearchBar;
In this code, we define a function component called SearchBar
that renders a Bootstrap form with an input field and a submit button.
Next, go back to the Navbar.js
file and import the SearchBar
component by adding the following line at the top of the file:
import SearchBar from './SearchBar';
Then, update the JSX code inside the Navbar
component to include the SearchBar
component:
const Navbar = () => { return ( <nav> <a>My Navbar</a> <button type="button" data-toggle="collapse" data-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation"> <span></span> </button> <div id="navbarNav"> <ul> <li> <a>Home</a> </li> <li> <a>About</a> </li> <li> <a>Contact</a> </li> </ul> </div> </nav> ); };
In this code, we import the SearchBar
component and render it inside the navbar-collapse
div. We also update the navbar-nav
class to mr-auto
to align the navigation links to the left.
If you save the file and refresh your browser, you should now see the search bar added to the right side of the navbar.
Making the Navbar Responsive
A responsive navbar is crucial for providing a seamless user experience across different devices and screen sizes. In this section, we’ll make our navbar responsive using Bootstrap’s built-in responsive classes and components.
To make the navbar responsive, we need to add a few additional Bootstrap classes to our existing code.
First, update the navbar-expand-lg
class in the nav
element to navbar-expand-md
:
<nav>
This will make the navbar expand to a full-width layout on medium-sized screens and larger.
Next, update the navbar-toggler
button to include the navbar-light
class:
<button type="button" data-toggle="collapse" data-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
This will change the color of the toggle button to a lighter color.
Finally, update the navbar-nav
class to include the ml-auto
class:
<ul>
This will align the navigation links to the right side of the navbar.
If you save the file and refresh your browser, you should now see that the navbar collapses into a mobile-friendly menu when viewed on smaller screens.
Related Article: How to Use Javascript Substring, Splice, and Slice
Implementing a Mobile Navigation Menu
In the previous section, we made our navbar responsive by collapsing it into a mobile-friendly menu on smaller screens. However, the menu is currently not functional. In this section, we’ll add the necessary JavaScript code to toggle the menu when the toggle button is clicked.
To implement the mobile navigation menu, we’ll leverage Bootstrap’s built-in JavaScript functionality.
First, open the Navbar.js
file and add the following lines at the top of the file to import the required Bootstrap JavaScript libraries:
import 'bootstrap/dist/js/bootstrap.bundle.min.js';
Next, update the navbar-toggler
button to include the data-toggle
and data-target
attributes:
<button type="button" data-toggle="collapse" data-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
The data-toggle
attribute specifies that the button should toggle the collapsible menu, and the data-target
attribute specifies the ID of the collapsible element.
If you save the file and refresh your browser, you should now be able to click the toggle button to expand and collapse the mobile navigation menu.
Customizing the Appearance of the Navbar
While Bootstrap provides a set of default styles for the navbar, you may want to customize its appearance to match your application’s design. In this section, we’ll explore some customization options for the navbar component.
Bootstrap provides a wide range of classes that can be used to customize the appearance of the navbar. Here are a few examples:
– Changing the background color:
<nav>
This will change the background color of the navbar to the primary color defined by Bootstrap.
– Changing the text color:
<nav>
This will change the text color of the navbar to white.
– Changing the font size:
<nav>
This will increase the font size of the navbar text.
– Adding a logo or brand image:
<a> <img src="/logo.png" alt="Logo" /> </a>
This will add a logo or brand image to the navbar.
– Adding a custom CSS class:
<nav>
This will add a custom CSS class called custom-navbar
to the navbar.
These are just a few examples of how you can customize the appearance of the navbar. Feel free to experiment with different Bootstrap classes and CSS styles to achieve the desired look and feel.
Applying UI Design Principles to the Navbar
When designing a navbar, it’s important to consider the principles of user interface (UI) design to ensure a seamless and intuitive user experience. Here are a few UI design principles to keep in mind when designing your navbar:
– Keep it simple: A cluttered navbar can overwhelm users. Stick to the essential navigation links and avoid unnecessary elements.
– Use clear and concise labels: Use descriptive labels for your navigation links to make it clear to users what each link represents.
– Provide visual feedback: Use visual cues such as hover effects or active states to provide feedback to users when they interact with the navbar.
– Ensure accessibility: Make sure the navbar is accessible to all users, including those with disabilities. Use appropriate HTML tags and attributes, and ensure that the navbar can be navigated using keyboard controls.
– Optimize for mobile: With the increasing use of mobile devices, it’s important to design your navbar to be mobile-friendly. Consider using a responsive design that collapses into a mobile menu on smaller screens.
Related Article: JavaScript Arrays: Learn Array Slice, Array Reduce, and String to Array Conversion
Adding Dropdown Menus to the Navbar
In addition to basic navigation links, you may want to include dropdown menus in your navbar to provide more options to users. In this section, we’ll add a dropdown menu to our navbar component.
To add a dropdown menu, we’ll use Bootstrap’s dropdown
component and the Link
component from the next/link
module.
First, open the Navbar.js
file and import the Link
component by adding the following line at the top of the file:
import Link from 'next/link';
Next, update the JSX code inside the Navbar
component to include a dropdown menu:
const Navbar = () => { return ( <nav> <a>My Navbar</a> <button type="button" data-toggle="collapse" data-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation"> <span></span> </button> <div id="navbarNav"> <ul> <li> <a>Home</a> </li> <li> <a>About</a> </li> <li> <a href="#" id="navbarDropdown" role="button" data-toggle="dropdown" aria-expanded="false"> Dropdown </a> <div aria-labelledby="navbarDropdown"> <a>Dropdown Item 1</a> <a>Dropdown Item 2</a> <div></div> <a>Dropdown Item 3</a> </div> </li> <li> <a>Contact</a> </li> </ul> </div> </nav> ); };
In this code, we add a new list item with the nav-item dropdown
classes to create the dropdown menu. The dropdown toggle link has the nav-link dropdown-toggle
classes and includes the necessary data-toggle
and data-target
attributes to enable the dropdown functionality. The dropdown menu itself is defined inside a <div>
element with the dropdown-menu
class.
Inside the dropdown menu, we use the Link
component to create navigation links for the dropdown items. Each dropdown item is wrapped in a component and has the
dropdown-item
class.
If you save the file and refresh your browser, you should now see a dropdown menu in the navbar. Clicking on the dropdown toggle will reveal the dropdown menu with the specified items.
Best Practices for Designing a Navigation Menu
Designing a navigation menu is an important part of creating a user-friendly website or application. Here are some best practices to consider when designing a navigation menu:
– Keep it simple: A cluttered menu can confuse users and make it difficult for them to find what they’re looking for. Stick to the essential navigation links and avoid unnecessary complexity.
– Use clear and concise labels: Make sure the labels for your navigation links are clear and easy to understand. Avoid jargon or technical terms that may not be familiar to all users.
– Group related links together: Grouping related links together can make it easier for users to find what they’re looking for. Consider using dropdown menus or submenus to organize your navigation links.
– Provide visual feedback: Use visual cues to indicate the current page or active link in your navigation menu. This can help users understand their current location within the website or application.
– Optimize for mobile: With the increasing use of mobile devices, it’s important to design your navigation menu to be mobile-friendly. Consider using a responsive design that adapts to different screen sizes and provides a seamless user experience on mobile devices.
– Test and iterate: Don’t be afraid to test different designs and gather feedback from users. Use analytics to track user behavior and identify areas for improvement. Continuously iterate and refine your navigation menu based on user feedback and data.
Creating a Sticky Navbar in Next.js
A sticky navbar remains fixed at the top of the page, even when the user scrolls down. This can be useful for providing easy access to navigation links at all times. In this section, we’ll create a sticky navbar in Next.js using CSS.
To create a sticky navbar, we’ll use a combination of CSS and JavaScript. First, open the Navbar.module.css
file and add the following CSS code:
.navbar { position: sticky; top: 0; z-index: 100; }
In this code, we set the position
property of the navbar to sticky
, which will make it stick to the top of the page. We also set the top
property to 0
to ensure that the navbar remains at the top of the page even when scrolling. The z-index
property is used to control the stacking order of elements on the page, and a higher value ensures that the navbar appears on top of other elements.
Next, open the Navbar.js
file and import the styles
object from the Navbar.module.css
file by adding the following line at the top of the file:
import styles from './Navbar.module.css';
Then, update the JSX code inside the Navbar
component to use the navbar
class from the imported CSS module:
const Navbar = () => { return ( <nav> {/* Navbar content */} </nav> ); };
If you save the file and refresh your browser, you should now see that the navbar remains fixed at the top of the page as you scroll.
Related Article: JavaScript HashMap: A Complete Guide
Animating the Navbar with JavaScript
Adding subtle animations to the navbar can enhance the user experience and make the navigation feel more interactive. In this section, we’ll add a simple animation to our navbar using JavaScript.
To animate the navbar, we’ll use the scroll
event and the classList
property in JavaScript. First, open the Navbar.js
file and add the following JavaScript code inside the Navbar
component:
import React, { useEffect } from 'react'; import styles from './Navbar.module.css'; const Navbar = () => { useEffect(() => { const navbar = document.querySelector(`.${styles.navbar}`); window.addEventListener('scroll', () => { if (window.scrollY > 0) { navbar.classList.add(styles.scrolled); } else { navbar.classList.remove(styles.scrolled); } }); }, []); return ( <nav> {/* Navbar content */} </nav> ); }; export default Navbar;
In this code, we use the useEffect
hook to add an event listener to the scroll
event. Inside the event listener, we check the window.scrollY
property to determine the current scroll position. If the scroll position is greater than 0
, we add a scrolled
class to the navbar using the classList
property. Otherwise, we remove the scrolled
class.
Next, open the Navbar.module.css
file and add the following CSS code:
.navbar { transition: background-color 0.3s; } .navbar.scrolled { background-color: #f8f9fa; box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1); }
In this code, we add a transition effect to the background-color
property of the navbar using the transition
property. We also define a new .navbar.scrolled
class that changes the background color and adds a subtle box shadow when the navbar has the scrolled
class.
If you save the files and refresh your browser, you should now see that the navbar animates its background color and adds a box shadow as you scroll down the page.
We hope this tutorial has been helpful in building your own navbar in Next.js. Feel free to experiment with different styles and functionality to create a unique and user-friendly navigation experience for your Next.js applications.
Additional Resources
– CSS – MDN Web Docs
– CSS Basics – W3Schools
– HTML – MDN Web Docs