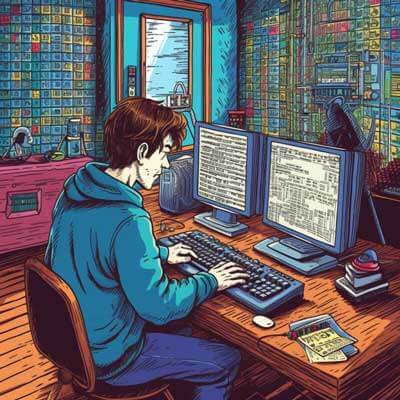
Table of Contents
React component execution in a JavaScript file
React is a popular JavaScript library used for building user interfaces. It allows developers to create reusable UI components that can be used across different parts of an application. In order to use a React component in a JavaScript file, there are a few steps that need to be followed.
Related Article: How To Append To A Javascript Array
Step 1: Install React
Before we can start using React components in a JavaScript file, we need to install React and its dependencies. This can be done using npm, the package manager for JavaScript.
npm install react react-dom
Step 2: Create a React component
In order to use a React component in a JavaScript file, we first need to create the component itself. A React component is a JavaScript function or class that returns JSX, which is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript files.
Here's an example of a simple React component that displays a "Hello, World!" message:
// HelloWorld.js import React from 'react'; function HelloWorld() { return <div>Hello, World!</div>; } export default HelloWorld;
In this example, we define a function called HelloWorld
that returns a JSX element containing the message "Hello, World!". We then export this component using the export default
syntax so that it can be used in other JavaScript files.
Calling a React component in JavaScript
Once we have created a React component, we can call it in a JavaScript file by importing the component and rendering it within the desired DOM element.
Related Article: Conditional Flow in JavaScript: Understand the 'if else' and 'else if' Syntax and More
Step 1: Import the React component
To use a React component in a JavaScript file, we first need to import it. This can be done using the import
keyword followed by the name of the component and the file path.
import HelloWorld from './HelloWorld';
In this example, we import the HelloWorld
component from the HelloWorld.js
file located in the same directory as our JavaScript file.
Step 2: Render the React component
Once we have imported the React component, we can render it in our JavaScript file by using the ReactDOM.render()
function. This function takes two arguments: the component to render and the DOM element where the component should be rendered.
import ReactDOM from 'react-dom'; ReactDOM.render(, document.getElementById('root'));
In this example, we use the ReactDOM.render()
function to render the HelloWorld
component and mount it to the DOM element with the id
of "root".
Importing a React component into a JavaScript file
To use a React component in a JavaScript file, we need to import it using the import
keyword followed by the component name and the file path.
Here's an example of how to import a React component called HelloWorld
from a file called HelloWorld.js
:
import HelloWorld from './HelloWorld';
In this example, we import the HelloWorld
component from the HelloWorld.js
file located in the same directory as our JavaScript file.
Rendering a React component in a JavaScript file
To render a React component in a JavaScript file, we use the ReactDOM.render()
function. This function takes two arguments: the component to render and the DOM element where the component should be rendered.
Here's an example of how to render a React component called HelloWorld
:
import ReactDOM from 'react-dom'; import HelloWorld from './HelloWorld'; ReactDOM.render(, document.getElementById('root'));
In this example, we import the HelloWorld
component and render it in the DOM element with the id
of "root".
Related Article: Implementing Global CSS Imports in Next.js
Invoking a React component in JavaScript
Invoking a React component in JavaScript involves creating an instance of the component and calling its render method.
Here's an example of how to invoke a React component called HelloWorld
:
import React from 'react'; class HelloWorld extends React.Component { render() { return <div>Hello, World!</div>; } } const helloWorld = new HelloWorld(); console.log(helloWorld.render());
In this example, we create a new instance of the HelloWorld
component using the new
keyword. We then call the render()
method of the component, which returns the JSX element representing the component. Finally, we log the result to the console.
Integrating a React component into a JavaScript file
To integrate a React component into a JavaScript file, we need to import the component and render it within the desired DOM element.
Here's an example of how to integrate a React component called HelloWorld
:
import React from 'react'; import ReactDOM from 'react-dom'; class HelloWorld extends React.Component { render() { return <div>Hello, World!</div>; } } ReactDOM.render(, document.getElementById('root'));
In this example, we import the React
and ReactDOM
libraries, as well as the HelloWorld
component. We then use the ReactDOM.render()
function to render the HelloWorld
component and mount it to the DOM element with the id
of "root".
Using a React component in JavaScript
To use a React component in JavaScript, we need to import the component and render it within the desired DOM element.
Here's an example of how to use a React component called HelloWorld
:
import React from 'react'; import ReactDOM from 'react-dom'; class HelloWorld extends React.Component { render() { return <div>Hello, World!</div>; } } ReactDOM.render(, document.getElementById('root'));
In this example, we import the React
and ReactDOM
libraries, as well as the HelloWorld
component. We then use the ReactDOM.render()
function to render the HelloWorld
component and mount it to the DOM element with the id
of "root".
Calling a React component using a JavaScript function
To call a React component using a JavaScript function, we need to define a function that renders the component and invoke that function.
Here's an example of how to call a React component called HelloWorld
using a JavaScript function:
import React from 'react'; import ReactDOM from 'react-dom'; function renderHelloWorld() { ReactDOM.render(, document.getElementById('root')); } class HelloWorld extends React.Component { render() { return <div>Hello, World!</div>; } } renderHelloWorld();
In this example, we define a function called renderHelloWorld()
that uses the ReactDOM.render()
function to render the HelloWorld
component and mount it to the DOM element with the id
of "root". We then define the HelloWorld
component and invoke the renderHelloWorld()
function.
Related Article: How to Use Angular Reactive Forms
Calling a class component in a JavaScript file
To call a class component in a JavaScript file, we need to create an instance of the component and render it using the ReactDOM.render()
function.
Here's an example of how to call a class component called HelloWorld
:
import React from 'react'; import ReactDOM from 'react-dom'; class HelloWorld extends React.Component { render() { return <div>Hello, World!</div>; } } const helloWorld = new HelloWorld(); ReactDOM.render(helloWorld.render(), document.getElementById('root'));
In this example, we create a new instance of the HelloWorld
component using the new
keyword. We then render the component by calling its render()
method and passing the result to the ReactDOM.render()
function. The component is mounted to the DOM element with the id
of "root".
Including a React component in a JavaScript file
To include a React component in a JavaScript file, we need to import the component and render it within the desired DOM element.
Here's an example of how to include a React component called HelloWorld
:
import React from 'react'; import ReactDOM from 'react-dom'; class HelloWorld extends React.Component { render() { return <div>Hello, World!</div>; } } ReactDOM.render(, document.getElementById('root'));
In this example, we import the React
and ReactDOM
libraries, as well as the HelloWorld
component. We then use the ReactDOM.render()
function to render the HelloWorld
component and mount it to the DOM element with the id
of "root".
Additional Resources
- Syntax for Calling a React Component in JavaScript