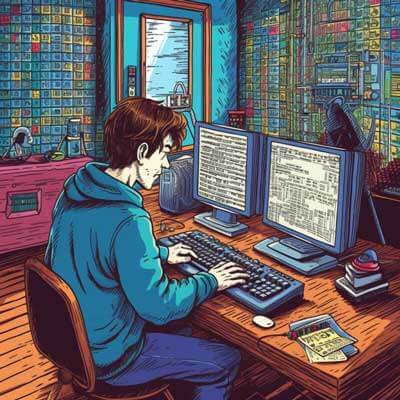
Table of Contents
To check if a string matches a regex in JavaScript, you can use the test
method of the regular expression object. This method returns true
if the string matches the regex pattern, and false
otherwise.
Here are two possible ways to check if a string matches a regex in JavaScript:
Using the test
method
You can create a regular expression object using the RegExp
constructor, passing the regex pattern as a string. Then, you can use the test
method of the regex object to check if a string matches the pattern. Here's an example:
const regex = new RegExp('pattern'); const string = 'example string'; const isMatch = regex.test(string); console.log(isMatch); // true or false
In this example, replace 'pattern'
with your desired regex pattern, and 'example string'
with the string you want to test.
Related Article: How to Remove a Specific Item from an Array in JavaScript
Using the match
method
Another way to check if a string matches a regex in JavaScript is by using the match
method of the string object. This method returns an array of matches if the string matches the regex pattern, and null
otherwise. Here's an example:
const regex = /pattern/; const string = 'example string'; const matches = string.match(regex); const isMatch = matches !== null; console.log(isMatch); // true or false
In this example, replace /pattern/
with your desired regex pattern, and 'example string'
with the string you want to test.
It's important to note that the match
method returns an array of matches, so you can access the matched portions of the string if needed. If you only want to check if the string matches the regex pattern, you can simply check if the matches
variable is not null
.
Best Practices
Related Article: How to Convert an Object to a String in JavaScript
When checking if a string matches a regex in JavaScript, consider the following best practices:
1. Use regular expression literals (/pattern/
) instead of the RegExp
constructor when the regex pattern is known in advance. This provides better readability and avoids unnecessary overhead.
2. Include the necessary flags in the regex literal if needed. For example, /pattern/i
matches the pattern case-insensitively, and /pattern/g
matches all occurrences of the pattern.
3. Test your regex patterns thoroughly with different input strings to ensure they match the desired criteria. You can use online regex testers or JavaScript's test
and match
methods for this purpose.
4. Consider using regex quantifiers (such as *
, +
, ?
, {n}
, {n,}
, or {n,m}
) to specify the number of occurrences a pattern should match. This helps to make your regex more precise and avoid false positives.
5. Escape special characters in your regex pattern using a backslash (\
) if you want to match them literally. For example, to match a dot character (.
), use \.
in your regex pattern.
6. Take advantage of regex character classes ([...]
) to match specific sets of characters. For example, [a-z]
matches any lowercase letter, [0-9]
matches any digit, and [^0-9]
matches any character that is not a digit.