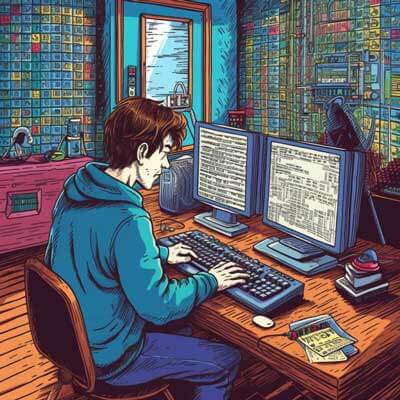
Copying text to the clipboard in JavaScript can be achieved using the Clipboard API or by using the document.execCommand('copy')
method. Let’s explore both approaches in detail:
Using the Clipboard API
The Clipboard API provides a modern and standardized way to interact with the clipboard in JavaScript. It allows you to read from and write to the clipboard using the navigator.clipboard
object. Here’s how you can copy text to the clipboard using the Clipboard API:
navigator.clipboard.writeText('Text to be copied') .then(() => { console.log('Text copied to clipboard'); }) .catch((error) => { console.error('Failed to copy text: ', error); });
In the code snippet above, we call the writeText()
method of the navigator.clipboard
object and pass the text that we want to copy as a parameter. This returns a promise that resolves when the text is successfully copied to the clipboard. If there is an error, the promise is rejected, and the error can be handled in the catch()
block.
It’s important to note that the Clipboard API has some limitations. For security reasons, browsers require user interaction (such as a click event) to access the clipboard. Additionally, this API might not be supported in older browsers. Therefore, it’s recommended to check for browser support before using the Clipboard API by using the navigator.clipboard
property:
if (navigator.clipboard) { // Clipboard API is supported } else { // Fallback to alternative method }
Related Article: How to Compare Arrays in Javascript
Using the document.execCommand(‘copy’) method
If you need to support older browsers that do not have support for the Clipboard API, you can use the document.execCommand('copy')
method. This method relies on the browser’s built-in copy functionality and can be used as a fallback option. Here’s an example of how to use it:
const copyText = document.createElement('textarea'); copyText.value = 'Text to be copied'; document.body.appendChild(copyText); copyText.select(); document.execCommand('copy'); document.body.removeChild(copyText); console.log('Text copied to clipboard');
In the code snippet above, we create a temporary textarea element, set its value to the text that we want to copy, append it to the document body, select its contents, execute the copy
command, and finally remove the temporary element from the document body.
It’s worth mentioning that the document.execCommand('copy')
method is deprecated and no longer recommended for general use. However, it can still be used as a fallback for older browsers that lack support for the Clipboard API.
Best Practices
When copying text to the clipboard in JavaScript, it’s important to consider the following best practices:
1. Provide feedback to the user: After copying text to the clipboard, it’s a good practice to provide visual feedback to the user, such as displaying a success message or changing the appearance of the copy button.
2. Handle errors gracefully: When using the Clipboard API, it’s possible that copying text to the clipboard may fail due to various reasons, such as browser restrictions or user denial. Make sure to handle these errors gracefully and provide appropriate error messages to the user.
3. Consider accessibility: Ensure that your copy functionality is accessible to all users, including those who rely on assistive technologies. Use appropriate ARIA attributes and provide alternative methods for copying text if needed.
4. Test across different browsers: Test your copy functionality across different browsers and browser versions to ensure consistent behavior and compatibility.
Alternative Ideas
Apart from the Clipboard API and the document.execCommand('copy')
method, there are also other libraries and techniques available for copying text to the clipboard in JavaScript. Some popular libraries include Clipboard.js, clipboard-polyfill, and react-copy-to-clipboard. These libraries provide additional features and cross-browser compatibility.
If you’re working with a framework like React, you can also make use of the useClipboard
hook provided by libraries like React-Query or Chakra UI. These hooks abstract away the complexities of copying text to the clipboard and provide a simple and declarative API.
Additionally, if you’re building a web application that requires more advanced copy functionality, such as copying rich text or images, you can explore using third-party libraries or implementing custom solutions.
Related Article: How to Create a Countdown Timer with Javascript