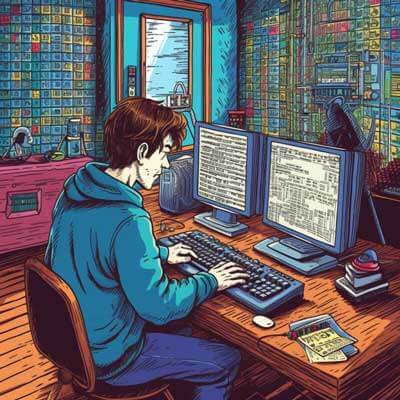
Table of Contents
What are Template Literals?
Template literals, also known as template strings, are a new feature introduced in ECMAScript 6 (ES6) that provide an easier and more readable way to interpolate variables within strings in JavaScript. Before template literals, developers had to use concatenation or string interpolation methods like string concatenation with the +
operator or the concat()
method, as well as string interpolation with the +
operator or the replace()
method.
Template literals make it possible to embed expressions inside a string, which can be variables, function calls, or even other template literals. They are defined using backticks instead of single or double quotes. By using template literals, you can create strings that span multiple lines without the need for escape characters, making your code more concise and readable.
Here's a simple example that demonstrates the syntax of template literals:
const name = 'John'; const age = 30; const message = `My name is ${name} and I am ${age} years old.`; console.log(message);
In this example, we define two variables, name
and age
, and then use them within a template literal to create the message
string. The variables are enclosed in ${}
within the template literal, allowing them to be interpolated directly into the string. When we log the message
variable to the console, it will display the following output:
My name is John and I am 30 years old.
Template literals also support multiline strings without the need for concatenation:
const multilineMessage = `This is a multiline string.`; console.log(multilineMessage);
The output of this code will be:
This is a multiline string.
Template literals can also be nested within each other:
const nestedMessage = `Hello, ${`World`}!`; console.log(nestedMessage);
The output will be:
Hello, World!
Template literals can also be used with tagged templates, which allow for more advanced string manipulation. Tagged templates are a way to process template literals with a function, called a tag function, which can modify the output.
function tagFunction(strings, ...values) { // Process the template strings and interpolated values // ... } const name = 'John'; const age = 30; const taggedMessage = tagFunction`My name is ${name} and I am ${age} years old.`; console.log(taggedMessage);
In this example, the tagFunction
is called with the template literal as its argument. The strings
parameter is an array containing the static parts of the template literal, while the values
parameter is an array containing the interpolated values. The tagFunction
can then process these parts and values as needed.
Template literals provide a more concise and readable way to interpolate variables within strings in JavaScript. They make it easier to create dynamic strings without the need for complex concatenation or interpolation methods. With their support for multiline strings and nested expressions, template literals are a powerful addition to the JavaScript language.
Template literals can also contain expressions and function calls. This allows us to perform calculations or execute functions within the string itself. Here's an example:
const a = 5; const b = 10; const result = `The sum of ${a} and ${b} is ${a + b}.`; console.log(result);
In the above code, we define two variables a
and b
, and calculate their sum inside the template literal. When the code is executed, the sum is substituted into the string, resulting in the following output:
The sum of 5 and 10 is 15.
Template literals can also be tagged with a function, known as a tagged template. This allows us to modify the behavior of the template literal by processing its contents before it is output. Tagged templates are especially useful for tasks like internationalization or sanitizing user input. Here's an example:
function tag(strings, ...values) { return strings.reduce((result, string, index) => { result += string; if (index < values.length) { result += values[index]; } return result; }, ''); } const name = 'John'; const message = tag`Hello, ${name}!`; console.log(message);
In the above code, we define a function tag
that takes an array of strings (strings
) and an array of values (values
). The function then combines the strings and values to form the final output. In this example, we simply concatenate the strings and values together, but you can customize the behavior of the tag
function according to your needs.
Overall, ES6 template literals provide a powerful and flexible way to work with strings in JavaScript. They simplify string interpolation, make code more readable, and offer additional features like multi-line strings and tagged templates.
Related Article: How to Use ForEach in JavaScript
Interpolating Variables
ES6 template literals provide a simplified way to interpolate variables into strings in JavaScript. With template literals, you can easily include variables and expressions within a string without having to concatenate or escape characters.
To interpolate a variable, you simply enclose it within ${}
within the template literal. Let's take a look at an example:
const name = 'John'; const age = 30; const message = `My name is ${name} and I am ${age} years old.`; console.log(message);
In the example above, we have defined two variables, name
and age
. We use these variables within the template literal to create the message
string. The variables are enclosed within ${}
and are automatically evaluated and replaced with their respective values when the template literal is evaluated.
The output of the above code will be:
My name is John and I am 30 years old.
Template literals also support expressions, allowing you to perform calculations or execute functions within the template. Here's an example:
const price = 10; const quantity = 5; const total = `The total cost is ${price * quantity} dollars.`; console.log(total);
In this example, we calculate the total cost by multiplying the price
and quantity
variables within the template literal. The output will be:
The total cost is 50 dollars.
Template literals can also be used with multiline strings, making it easier to work with multiline strings without having to use escape characters. Here's an example:
const message = ` This is a multiline string. It can span multiple lines without the need for escape characters. It also supports variable interpolation, like this: ${name}. `; console.log(message);
In this example, the message
variable contains a multiline string. The variable name
is interpolated within the template literal. The output will be:
This is a multiline string. It can span multiple lines without the need for escape characters. It also supports variable interpolation, like this: John.
ES6 template literals provide a more concise and readable way to interpolate variables and expressions into strings. They eliminate the need for concatenation and make it easier to work with multiline strings.
String Manipulation
Template literals can be used to perform various string manipulation operations. Here are some examples:
String Concatenation:
const firstName = 'John'; const lastName = 'Doe'; const fullName = `${firstName} ${lastName}`; console.log(fullName);
The output will be:
John Doe
Arithmetic Operations:
const x = 10; const y = 5; const sum = `${x} + ${y} = ${x + y}`; console.log(sum);
The output will be:
10 + 5 = 15
Function Calls:
function capitalize(str) { return str.charAt(0).toUpperCase() + str.slice(1); } const name = 'john'; const capitalized = `Capitalized name: ${capitalize(name)}`; console.log(capitalized);
The output will be:
Capitalized name: John
Tagged Templates
Template literals can also be used with tagged templates, allowing you to modify the output of a template literal using a function. The function is called a tag function and it receives the interpolated values as arguments. Here's an example:
function highlight(strings, ...values) { let result = ''; strings.forEach((string, i) => { result += string; if (i < values.length) { result += `<strong>${values[i]}</strong>`; } }); return result; } const name = 'John'; const age = 30; const message = highlight`Hello, my name is ${name} and I am ${age} years old.`; console.log(message);
The output will be:
Hello, my name is <strong>John</strong> and I am <strong>30</strong> years old.
In this example, the highlight
function receives the string parts and interpolated values as arguments. It concatenates the string parts and wraps the interpolated values with <strong>
tags.
Template literals provide a concise and powerful way to manipulate strings in JavaScript. They make it easier to concatenate variables, create multi-line strings, and perform string manipulation operations.
Related Article: How to Implement Inline Style Best Practices in React Js
Multiline Strings
In JavaScript, creating multiline strings used to be a cumbersome task. Developers had to manually concatenate multiple strings with the newline character ("\n") or use string concatenation operators. This approach was not only error-prone but also made the code harder to read and maintain.
ES6 template literals provide an elegant solution to this problem by allowing us to create multiline strings without any additional effort. To create a multiline string, we simply enclose the content within backticks.
const multilineString = ` This is a multiline string. It allows us to write multiple lines of text without any hassle. We can even include variables like ${name} inside the string. `;
As shown in the example above, we can easily write multiple lines of text without worrying about escaping characters or concatenation. The resulting string will preserve the indentation, making it easier to read and write.
Template literals also support interpolation, which means we can embed variables or expressions directly into the string. To interpolate a variable, we wrap it with ${}
. Let's see an example:
const name = "John"; const age = 25; const greeting = `Hello, my name is ${name} and I am ${age} years old.`;
In the example above, the variables name
and age
are interpolated within the string. The resulting string will be "Hello, my name is John and I am 25 years old."
Template literals also support expressions inside the ${}
placeholders. This allows us to perform calculations or execute functions within the interpolated values. Here's an example:
const a = 10; const b = 5; const sum = `The sum of ${a} and ${b} is ${a + b}.`;
In this example, the expression ${a + b}
is evaluated and the result is interpolated within the string. The resulting string will be "The sum of 10 and 5 is 15."
In summary, multiline strings can be easily created with ES6 template literals by enclosing the content within backticks. Template literals also support interpolation, making it convenient to embed variables or expressions directly into the string. This feature simplifies string manipulation in JavaScript and improves code readability.
Tagged Templates: Customizing String Output
Tagged templates are a powerful feature introduced in ES6 that allow you to customize the output of template literals in JavaScript. By using a function called a tag, you can process the template literal and modify its output in any way you want.
To create a tagged template, you need to prefix the template literal with the name of the tag function followed by a pair of parentheses. The tag function receives two arguments: an array of string values and an array of the expressions within the template literal.
Here's an example of a tagged template:
function customTag(strings, ...values) { // Process the template literal here } const name = 'John'; const age = 25; const result = customTag`My name is ${name} and I'm ${age} years old.`;
In this example, the customTag
function is used as the tag for the template literal. The strings
array contains the string values of the template literal, and the values
array contains the values of the expressions within the template literal.
The tag function can then process the template literal and return a modified string. It can perform any kind of manipulation on the strings and values, such as formatting, filtering, or even generating completely new output based on the input.
Here's a simple implementation of the customTag
function that uppercase the string values and concatenates them with the expressions:
function customTag(strings, ...values) { let result = ''; strings.forEach((string, index) => { result += string.toUpperCase(); if (index < values.length) { result += values[index]; } }); return result; } const name = 'John'; const age = 25; const result = customTag`My name is ${name} and I'm ${age} years old.`; console.log(result); // Output: "MY NAME IS JOHN AND I'M 25 YEARS OLD."
In this example, the customTag
function loops over the strings
array, uppercase each string, and appends the corresponding value from the values
array. The resulting string is then returned.
Tagged templates can be useful in various scenarios, such as internationalization (i18n), syntax highlighting, or creating domain-specific languages (DSLs). They provide a flexible and customizable way to manipulate string output in JavaScript.
To learn more about tagged templates and their usage, you can refer to the official documentation on MDN.
That's it for this chapter on tagged templates. In the next chapter, we will explore another powerful feature of ES6 template literals: multiline strings.
Using Template Literals with Functions
Template literals in ES6 not only allow us to interpolate variables and expressions directly into strings, but they also provide a convenient way to use functions within the template. This feature opens up new possibilities for generating dynamic content and manipulating strings in JavaScript.
To use a function within a template literal, we simply enclose the function call or expression inside the ${}
syntax. The function can be any valid JavaScript function, including built-in functions or custom functions.
Let's consider a simple example where we have a function that returns the current date:
function getCurrentDate() { return new Date().toDateString(); } const message = `Today's date is ${getCurrentDate()}.`; console.log(message);
In the code above, we define a getCurrentDate
function that returns the current date using the Date
object. We then use this function within the template literal by calling it inside ${}
. The resulting string will include the current date when the template literal is evaluated.
The output of the above code will be something like:
Today's date is Wed Jan 01 2022.
We can also use template literals with functions that accept arguments. Let's see an example where we have a function that calculates the sum of two numbers:
function sum(a, b) { return a + b; } const num1 = 5; const num2 = 10; const result = `The sum of ${num1} and ${num2} is ${sum(num1, num2)}.`; console.log(result);
In this case, we define a sum
function that takes two arguments and returns their sum. Inside the template literal, we interpolate the values of num1
, num2
, and the result of the sum
function.
The output of the above code will be:
The sum of 5 and 10 is 15.
Template literals provide a concise and readable way to incorporate dynamic values and function calls into strings. They eliminate the need for concatenation or string manipulation, making our code cleaner and more maintainable.
In addition to using functions directly within template literals, we can also use tagged template literals to further customize the behavior of string interpolation. Tagged template literals allow us to preprocess the template literal with a function before it is evaluated. This can be useful for tasks like localization, escaping characters, or formatting.
To learn more about tagged template literals, you can refer to the [MDN web docs](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Template_literals#tagged_templates).
In the next chapter, we will explore more advanced features of ES6 template literals and how they can simplify string interpolation in JavaScript.
Escaping Characters in Template Literals
In ES6, template literals provide a convenient way to interpolate variables and expressions within strings. However, there may be cases where you need to include special characters or escape sequences within template literals. This chapter will discuss how to escape characters in template literals to ensure they are treated as literal characters and not as part of the string interpolation.
To escape a character in a template literal, you simply use the backslash (\) before the character you want to escape. This tells JavaScript to treat the character as a literal instead of interpreting it as part of the template literal syntax. Here's an example:
const name = 'John'; console.log(`Hello, \${name}!`);
In this example, the backslash before the dollar sign (\$) escapes it, so it is treated as a literal character and not as the start of a template expression. The output of this code will be:
Hello, ${name}!
Similarly, you can escape other special characters such as backticks, backslashes (\\), and newlines (\n) within template literals. Here's an example that demonstrates escaping these characters:
console.log(`This is a backtick: \` This is a backslash: \\ This is a newline: \n`);
The output of this code will be:
This is a backtick: ` This is a backslash: \ This is a newline:
It's important to note that escaping characters in template literals is only necessary if you want to include them as literal characters within the string. If you want to use them as part of the template literal syntax, there's no need to escape them.
In some cases, you may want to include a backslash character itself as a literal within a template literal. To do this, you need to escape the backslash by using two backslashes (\\). Here's an example:
console.log(`This is a backslash: \\\\`);
The output of this code will be:
This is a backslash: \\
In conclusion, escaping characters in template literals is a straightforward process that allows you to include special characters or escape sequences as literal characters within the string. By using the backslash (\) before the character you want to escape, you can ensure that it is treated as a literal and not as part of the string interpolation.
Related Article: How to Convert Date to Timestamp in Javascript
Template Literals in Conditional Statements
When working with conditional statements in JavaScript, template literals can be incredibly useful for simplifying string interpolation. They allow you to embed expressions directly within a string, making it easier to create dynamic content based on certain conditions.
Let's take a look at some examples to see how template literals can be used in conditional statements.
Example 1:
Suppose we have a variable called age
that represents a person's age. We want to display a different message depending on whether the person is a minor or an adult. We can achieve this using a template literal in an if
statement:
const age = 20; if (age >= 18) { console.log(`You are an adult.`); } else { console.log(`You are a minor.`); }
In this example, the template literal is used to dynamically generate the output message based on the value of the age
variable. If the age
is 18 or greater, the output will be "You are an adult." Otherwise, it will be "You are a minor."
Example 2:
Template literals can also be used in a ternary operator to conditionally assign a value to a variable. Let's say we have a variable called isAuthenticated
that represents the user's authentication status, and we want to assign a different greeting message based on whether the user is authenticated or not:
const isAuthenticated = true; const greeting = isAuthenticated ? `Welcome back, user!` : `Please log in.`; console.log(greeting);
In this example, the template literal is used within the ternary operator to dynamically assign a value to the greeting
variable. If isAuthenticated
is true, the greeting will be "Welcome back, user!" Otherwise, it will be "Please log in."
Example 3:
Template literals can also be used within a loop to generate dynamic content. Let's say we have an array of names and we want to display a custom greeting for each name:
const names = ['Alice', 'Bob', 'Charlie']; names.forEach(name => { console.log(`Hello, ${name}!`); });
In this example, the template literal is used within the forEach
loop to generate a custom greeting for each name in the names
array. The output will be:
Hello, Alice! Hello, Bob! Hello, Charlie!
As you can see, template literals make it easy to interpolate expressions within strings, allowing for more flexible and concise code when working with conditional statements.
Template literals can be a powerful tool when it comes to simplifying string interpolation in JavaScript. They allow you to embed expressions within strings, making it easier to create dynamic content based on certain conditions. Whether you are building a simple if statement, using a ternary operator, or iterating over an array, template literals can help you write cleaner and more readable code.
Template Literals in Loops
Template literals can be particularly useful when working with loops in JavaScript. They allow for dynamic string interpolation, making it easier to create and manipulate strings within a loop.
One common use case is when iterating over an array and generating a string based on the array elements. With template literals, we can easily concatenate the array elements into a single string without the need for complex string concatenation or array join operations.
Let's consider an example where we have an array of names and we want to generate a greeting message for each name using a loop:
const names = ['John', 'Jane', 'Mike']; for (let i = 0; i { const message = `Hello, ${name}!`; console.log(message); });
The above code achieves the same result as the previous example, but with a more concise syntax. The arrow function passed to forEach
takes each element of the names
array as an argument and generates the corresponding greeting message using a template literal.
Using template literals in loops can greatly improve the readability and maintainability of your code when working with dynamic string generation. They eliminate the need for manual string concatenation and make it easier to incorporate variables or expressions within strings.
In the next chapter, we will explore more advanced features of ES6 template literals, including multiline strings and tagged templates.
Template Literals for HTML Templates
ES6 template literals provide a powerful way to create HTML templates in JavaScript. They simplify the process of string interpolation and make it easier to generate dynamic HTML content. In this chapter, we will explore how to use template literals for HTML templates.
Template literals allow you to embed expressions inside a string using the ${}
syntax. This makes it easy to insert dynamic values into your HTML templates. Let's take a look at a simple example:
const name = "John"; const age = 30; const html = ` <div> <h1>Welcome, ${name}!</h1> <p>You are ${age} years old.</p> </div> `; document.body.innerHTML = html;
In the above example, we have a template literal that contains HTML markup. Inside the template literal, we use ${}
to insert the values of the name
and age
variables. The resulting HTML is then assigned to the document.body.innerHTML
property, which updates the content of the body element.
Template literals also support multi-line strings, which is useful for creating more complex HTML templates. You can include line breaks and indentation directly inside the template literal, making your code more readable:
const items = ["Apple", "Banana", "Orange"]; const html = ` <ul> ${items.map(item => `<li>${item}</li>`).join("")} </ul> `; document.body.innerHTML = html;
In this example, we have an array of items that we want to display as a list. We use the map
method to generate an array of <li>
elements, and then use the join
method to concatenate them into a single string. The resulting HTML is then assigned to the document.body.innerHTML
property.
Template literals also support tagged templates, which allow you to apply custom logic to the template values before they are interpolated into the string. This can be useful for sanitizing user input or applying formatting to the values. Here's an example:
function sanitize(strings, ...values) { const sanitizedValues = values.map(value => { // Sanitize the value here return value; }); // Combine the sanitized values with the strings return strings.reduce((acc, string, index) => { return acc + string + sanitizedValues[index]; }, ""); } const name = "alert('XSS');"; const html = sanitize` <p>Hello, ${name}!</p> `; document.body.innerHTML = html;
In this example, we define a sanitize
function that takes the template strings and values as arguments. Inside the function, we can apply any custom logic to sanitize the values. The sanitized values are then combined with the template strings to generate the final HTML.
Using ES6 template literals for HTML templates simplifies the process of string interpolation and makes it easier to generate dynamic HTML content. Whether you're creating simple or complex HTML templates, template literals provide a clean and efficient way to work with strings and expressions.
Using Template Literals for API Calls
Template literals in ES6 provide a convenient way to simplify string interpolation in JavaScript. One of the areas where template literals can be particularly useful is when making API calls.
Traditionally, when making API calls, we would construct the URL by concatenating strings and variables. This can quickly become cumbersome and error-prone, especially when dealing with complex query parameters or dynamic values. With template literals, we can make API calls much more readable and maintainable.
Let's take a look at an example. Suppose we want to make a GET request to the GitHub API to fetch information about a user. In the traditional approach, we might construct the URL like this:
const username = 'octocat'; const apiUrl = 'https://api.github.com/users/' + username;
With template literals, we can achieve the same result in a more concise way:
const username = 'octocat'; const apiUrl = `https://api.github.com/users/${username}`;
Using template literals, we can directly embed the username
variable within the URL string using the ${}
syntax. This makes it easier to read and understand the code, especially when dealing with longer URLs or multiple variables.
Template literals also allow us to easily handle complex query parameters. Let's say we want to fetch a list of repositories for a user, sorted by the number of stars in descending order. In the traditional approach, we would need to construct the URL like this:
const username = 'octocat'; const sort = 'stars'; const order = 'desc'; const apiUrl = 'https://api.github.com/users/' + username + '/repos' + '?sort=' + sort + '&order=' + order;
With template literals, we can achieve the same result in a more readable way:
const username = 'octocat'; const sort = 'stars'; const order = 'desc'; const apiUrl = `https://api.github.com/users/${username}/repos?sort=${sort}&order=${order}`;
By directly embedding the variables within the URL string, we can easily construct the query parameters without the need for complex string concatenation.
Template literals also support multiline strings, which can be useful for constructing complex API requests. Here's an example:
const username = 'octocat'; const apiUrl = `https://api.github.com/users/${username}/repos ?sort=${sort} &order=${order}`;
In this example, we can easily format the URL string across multiple lines for better readability. The resulting string will still be concatenated into a single line when sent as the API request.
In summary, using template literals for API calls simplifies string interpolation in JavaScript. It allows us to construct URLs and handle complex query parameters in a more readable and maintainable way. By directly embedding variables within the string, we can avoid complex string concatenation and make our code more concise.
Related Article: How To Convert Array To Object In Javascript
Template Literals in Regular Expressions
Regular expressions are powerful tools for pattern matching and manipulation in JavaScript. With the introduction of ES6 template literals, working with regular expressions has become even easier and more readable.
Template literals allow us to embed expressions inside strings using the ${}
syntax. This makes it convenient to include variables, function calls, and even other template literals within a regular expression.
Let's take a look at some examples to see how template literals can simplify working with regular expressions:
Example 1: Matching URLs
Suppose we want to match URLs that start with "http://" or "https://". We can use a regular expression like this:
const protocolRegex = /^(http|https):\/\//;
While this works, it can be hard to read and modify. With template literals, we can write the same regular expression in a more readable way:
const protocolRegex = /^(http|https):\/\//;
The template literal allows us to interpolate the http
and https
directly into the regular expression, making it clearer what the pattern is.
Example 2: Generating Dynamic Patterns
Template literals can also be used to generate dynamic regular expression patterns. Let's say we have an array of keywords and we want to match any of them in a string. We can use a regular expression like this:
const keywords = ['apple', 'banana', 'orange']; const keywordRegex = new RegExp(`(${keywords.join('|')})`, 'gi');
In this example, the template literal is used to dynamically generate the pattern by joining the keywords with the |
(OR) operator. The gi
flags are also included to make the regular expression case-insensitive and match multiple occurrences.
Example 3: Multi-line Matching
Template literals also make it easier to work with multi-line strings in regular expressions. In the past, we had to use the dot-all flag (s
) or manually include newlines in the pattern. With template literals, we can simply include newlines directly in the string:
const multilineRegex = /First line\nSecond line/;
The template literal allows us to write the regular expression in a more readable way, making it clear that we are matching multiple lines.
Template literals provide a convenient way to work with regular expressions in JavaScript. They make it easier to interpolate variables, generate dynamic patterns, and work with multi-line strings. Consider using template literals when working with regular expressions to simplify your code and improve readability.
Template Literals in Error Handling
Template literals provide a convenient way to handle error messages and improve code readability in JavaScript. By using template literals, you can easily interpolate variables and expressions into error messages, making them more descriptive and informative.
Traditionally, error messages were constructed by concatenating strings and variables, which made the code harder to read and maintain. With template literals, you can create error messages in a more concise and intuitive way.
Let's consider a simple example where we want to display an error message when a user enters an invalid email address.
const email = 'invalid'; throw new Error(`Invalid email address: ${email}`);
In the example above, we use a template literal to construct the error message. The ${email}
part is the placeholder for the variable email
. When the code is executed, the value of the email
variable will be interpolated into the error message.
Template literals also allow us to include expressions inside the placeholders. This can be useful when we want to provide additional context or perform some calculations in the error message.
const num1 = 5; const num2 = 0; if (num2 === 0) { throw new Error(`Cannot divide ${num1} by ${num2}. Result is undefined.`); }
In the example above, we use a template literal to construct an error message when attempting to divide num1
by num2
. By including the expressions ${num1}
and ${num2}
inside the error message, we can provide the values of these variables in the error message.
Template literals can also be used to handle multiline error messages in a more readable way. Instead of using string concatenation or escape characters, you can simply use multiline template literals.
const errorCode = 404; const errorMessage = `Error: Page not found. Error code: ${errorCode} Please check the URL and try again.`; throw new Error(errorMessage);
In the example above, we use a multiline template literal to construct an error message for a 404 page not found error. The template literal allows us to easily create a multiline error message without the need for concatenation or escape characters.
In summary, template literals simplify error handling in JavaScript by allowing for easy interpolation of variables and expressions into error messages. They improve code readability and maintainability by providing a more concise and intuitive way to construct error messages. With template literals, you can create more descriptive and informative error messages, making it easier to debug and fix issues in your code.
Using Template Literals with Promises
ES6 template literals not only simplify string interpolation but also work seamlessly with JavaScript promises. Promises are a powerful abstraction for handling asynchronous operations, and combining them with template literals can make your code more concise and readable.
When using template literals with promises, you can easily incorporate the values of resolved promises directly into your string templates. This allows you to dynamically construct strings based on the results of asynchronous operations.
Let's take a look at an example to see how this works:
const getUser = () => { return new Promise((resolve, reject) => { setTimeout(() => { resolve({ name: 'John', age: 30 }); }, 2000); }); }; const getPosts = () => { return new Promise((resolve, reject) => { setTimeout(() => { resolve(['Post 1', 'Post 2', 'Post 3']); }, 1500); }); }; Promise.all([getUser(), getPosts()]) .then(([user, posts]) => { console.log(`User: ${user.name}, Age: ${user.age}`); console.log(`Posts: ${posts.join(', ')}`); }) .catch((error) => { console.error(error); });
In this example, we have two asynchronous functions, getUser
and getPosts
, which return promises that resolve after a certain period of time. We use Promise.all
to wait for both promises to resolve before executing the callback.
Inside the callback, we can use template literals to construct strings that incorporate the values of the resolved promises. We access the properties of the user
object, such as name
and age
, and join the posts
array elements using the join
method.
By using template literals in this way, we can easily create dynamic strings that depend on the results of asynchronous operations. This can be especially useful when working with APIs or performing database queries, where the values may only be available after an asynchronous request.
In addition to Promise.all
, you can also use template literals with other promise methods such as Promise.race
or Promise.allSettled
. The flexibility of template literals allows you to easily customize your string templates based on the results of different promise scenarios.
Overall, using template literals with promises can greatly simplify string interpolation in JavaScript. It provides a more elegant and concise way to incorporate dynamic values into your strings, especially when dealing with asynchronous operations.
Template Literals in Object Literals
ES6 template literals are not limited to just simple string interpolation. They can also be used effectively within object literals to create more dynamic and readable code. In this chapter, we will explore how template literals can simplify the process of defining and manipulating object literals in JavaScript.
Related Article: How to Autofill a Textarea Element with VueJS
Basic Object Literal Syntax
Before we dive into using template literals, let's quickly review the basic syntax for creating object literals in JavaScript. Object literals are a convenient way to define and initialize objects without the need for a constructor function.
const name = 'John'; const age = 30; const person = { name: name, age: age, }; console.log(person); // { name: 'John', age: 30 }
In the example above, we define a person object with two properties: name and age. The values of these properties are assigned using variables. This syntax works fine, but it can become cumbersome when dealing with longer and more complex object literals.
Using Template Literals in Object Literals
Template literals can make the process of defining object literals more concise and readable. Instead of repeating the property name twice, we can use the shorthand syntax provided by template literals.
const name = 'John'; const age = 30; const person = { name, age, }; console.log(person); // { name: 'John', age: 30 }
By using template literals, we can directly assign the value of a variable to a property without explicitly specifying the property name. This shorthand syntax is especially useful when the variable name matches the property name.
Dynamic Property Names
In addition to simplifying the syntax for defining object literals, template literals can also be used to dynamically generate property names. This can be helpful when working with computed properties or when dealing with external data sources.
const prefix = 'user'; const userId = 123; const user = { [`${prefix}-${userId}`]: 'John', }; console.log(user); // { user-123: 'John' }
In the example above, we use a template literal inside square brackets to dynamically generate the property name. The resulting property name is a combination of the prefix and the userId variable.
Using Template Literals in Object Methods
Template literals can also be used inside object methods to dynamically generate strings. This can be useful when constructing dynamic URLs or generating formatted output.
const person = { name: 'John', age: 30, sayHello() { console.log(`Hello, my name is ${this.name} and I'm ${this.age} years old.`); }, }; person.sayHello(); // Hello, my name is John and I'm 30 years old.
In the example above, we define a sayHello method that uses a template literal to construct a dynamic string. The values of the name and age properties are interpolated into the string using the ${}
syntax.
Related Article: Integrating HTMX with Javascript Frameworks
Template Literals in ES6 Modules
ES6 introduced a powerful feature called template literals, which simplifies string interpolation in JavaScript. In this chapter, we will explore how template literals can be used in ES6 modules.
ES6 modules provide a way to organize and separate our code into reusable modules. With template literals, we can easily construct dynamic strings within these modules.
Template literals are surrounded by backticks. Inside a template literal, we can embed expressions using the ${expression}
syntax. These expressions are evaluated and their values are inserted into the resulting string.
Let's take a look at an example to understand how template literals can be used in ES6 modules:
// module.js export function greet(name) { return `Hello, ${name}!`; }
In the above code snippet, we have a module called module.js
. It exports a function called greet
, which takes a name
parameter. Inside the function, we use a template literal to construct a greeting message with the name
parameter.
To consume this module in another file, we can use the import
statement:
// main.js import { greet } from './module.js'; console.log(greet('John')); // Output: Hello, John!
In the main.js
file, we import the greet
function from the module.js
file. We then call the greet
function and pass it the name 'John'
. The resulting greeting message is logged to the console.
Template literals can also be used for multiline strings. We can directly include line breaks and indentation within the template literal.
// module.js export function formatMessage(name, message) { return ` Dear ${name}, ${message} Regards, Your Friend `; }
In the above example, the formatMessage
function takes a name
and a message
parameter. It constructs a multiline message using template literals, including line breaks and indentation for better readability.
Template literals also support tagged templates, which allow us to modify the output of the template literal by passing it through a function. This can be useful for escaping characters or implementing custom formatting.
// module.js export function highlight(strings, ...values) { let result = ''; strings.forEach((string, i) => { result += string; if (values[i]) { result += `<span class="highlight">${values[i]}</span>`; } }); return result; }
In the above example, we define a highlight
function that takes an array of strings
and an arbitrary number of values
. The strings
array represents the literal parts of the template literal, while the values
array represents the interpolated expressions.
Inside the highlight
function, we iterate over the strings
array and append each string to the result
variable. If a corresponding value exists in the values
array, we wrap it in a <span>
element with a class of "highlight".
Template literals in ES6 modules provide a more concise and expressive way to construct dynamic strings. They enhance the readability and maintainability of our code by simplifying string interpolation. By combining template literals with ES6 modules, we can create reusable and modular code that is easier to work with.
In the next chapter, we will explore more advanced features of template literals in JavaScript. Stay tuned!
Template Literals in Node.js
Node.js is a popular runtime environment for executing JavaScript code outside of a web browser. It allows developers to build server-side applications using JavaScript. With the introduction of ES6, Node.js also gained support for template literals, making string interpolation in Node.js more convenient and readable.
Template literals in Node.js are enclosed within backticks. They allow you to embed expressions and variables directly into a string without the need for concatenation or escaping characters. This simplifies the process of creating dynamic strings.
Let's take a look at a simple example:
const name = 'John'; const greeting = `Hello, ${name}!`; console.log(greeting);
In this example, we define a variable name
with the value "John". We then use a template literal to create a string greeting
that includes the value of the name
variable. The result is "Hello, John!".
Template literals also support multiline strings, making it easier to work with larger blocks of text. Here's an example:
const message = ` This is a multiline string. It can span across multiple lines without the need for special characters. `; console.log(message);
In this case, the message
variable contains a multiline string that spans across multiple lines. The resulting output will preserve the line breaks and indentation, making it easier to format and read.
Template literals can also be used to execute functions or expressions within the string. This is achieved by using the ${}
syntax. Here's an example:
function add(a, b) { return a + b; } const a = 5; const b = 10; const result = `The sum of ${a} and ${b} is ${add(a, b)}.`; console.log(result);
In this example, we define a function add
that takes two parameters and returns their sum. We then use template literals to create a string result
that includes the values of a
and b
, as well as the result of calling the add
function. The output will be "The sum of 5 and 10 is 15.".
Template literals in Node.js provide a more concise and readable way to perform string interpolation. They simplify the process of creating dynamic strings and make it easier to work with multiline text. Whether you're building a server-side application or a command-line tool with Node.js, template literals are a powerful feature that can enhance your code readability and maintainability.
Template Literals in Popular Libraries
Many popular JavaScript libraries and frameworks have also embraced the use of template literals to simplify string interpolation. Let's explore how some of these libraries make use of template literals.
React
React, a widely used JavaScript library for building user interfaces, leverages template literals in several ways. One common use case is in defining dynamic JSX elements. JSX is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript files.
Here's an example of how template literals can be used in a React component to dynamically render a list of items:
import React from 'react'; const MyComponent = ({ items }) => ( <ul> {items.map(item => ( <li>{`${item.name} - ${item.price}`}</li> ))} </ul> ); export default MyComponent;
In the above code, the template literal ${item.name} - ${item.price}
is used to interpolate the values of item.name
and item.price
within the JSX element.
Related Article: How to Navigate Using React Router Programmatically
Vue.js
Vue.js, another popular JavaScript framework for building user interfaces, also supports template literals. In Vue.js, you can use template literals in the template syntax to dynamically generate content.
Here's an example of how template literals can be used in a Vue.js component template:
<div> <p>{{ `${message} ${name}` }}</p> <button>{{ `Increment (${count})` }}</button> </div> export default { data() { return { message: 'Hello', name: 'World', count: 0 }; }, methods: { increment() { this.count++; } } };
In the above code, the template literals {{
${message} ${name} }}
and {{
Increment (${count}) }}
are used to dynamically display the values of message
, name
, and count
within the template.
Angular
Angular, a comprehensive JavaScript framework for building web applications, also supports template literals. In Angular, you can use template literals within the template syntax to interpolate values and generate dynamic content.
Here's an example of how template literals can be used in an Angular component template:
<p>{{ `${item.name} - ${item.price}` }}</p>
In the above code, the template literal {{
${item.name} - ${item.price} }}
is used to interpolate the values of item.name
and item.price
within the template.
jQuery
Even jQuery, a popular JavaScript library for DOM manipulation, can benefit from the use of template literals. With jQuery, you can use template literals to dynamically generate HTML content and insert it into the DOM.
Here's an example of how template literals can be used with jQuery to create and append an HTML element:
const name = 'John'; const age = 30; const html = `<div>Name: ${name}</div><div>Age: ${age}</div>`; $('body').append(html);
In the above code, the template literals <div>Name: ${name}</div>
and <div>Age: ${age}</div>
are used to interpolate the values of name
and age
within the HTML content.
Template literals have become an essential tool in modern JavaScript development, and their adoption by popular libraries and frameworks further demonstrates their usefulness in simplifying string interpolation.
Advanced Techniques: Creating Dynamic Templates
ES6 template literals provide a powerful way to create dynamic templates in JavaScript. In addition to simple variable substitution, template literals support more advanced techniques that can greatly enhance the flexibility and readability of your code.
1. Expressions within Template Literals
Template literals allow you to embed expressions within the string, using the ${}
syntax. This enables you to perform calculations, call functions, or even embed conditional logic directly within the template.
const name = 'John'; const age = 30; const message = `My name is ${name} and I am ${age > 18 ? 'an adult' : 'a minor'}.`; console.log(message); // Output: My name is John and I am an adult.
By using expressions within template literals, you can easily build dynamic templates based on the values of variables or the result of calculations.
2. Multi-line Templates
Another powerful feature of template literals is the ability to create multi-line templates without the need for manual line breaks or concatenation.
const message = ` Hello, This is a multi-line template literal. It allows you to easily create formatted and readable text. `; console.log(message); // Output: // Hello, // This is a multi-line template literal. // It allows you to easily create // formatted and readable text.
The ability to create multi-line templates can be especially useful when dealing with large chunks of text, such as HTML templates or email bodies.
3. Tagged Templates
Tagged templates allow you to customize the behavior of template literals by prefixing the template literal with a function name, known as a "tag function". The tag function receives the template literal as an argument, along with the interpolated values as separate arguments.
function highlight(strings, ...values) { let result = ''; strings.forEach((string, i) => { result += string; if (values[i]) { result += `<span class="highlight">${values[i]}</span>`; } }); return result; } const name = 'John'; const age = 30; const message = highlight`My name is ${name} and I am ${age} years old.`; console.log(message); // Output: My name is <span class="highlight">John</span> and I am <span class="highlight">30</span> years old.
In the above example, the highlight
function is used as a tag function to wrap the interpolated values with an HTML <span>
element. This allows you to apply custom formatting or styling to the interpolated values.
4. Nested Templates
Template literals can be nested within each other, allowing for more complex and dynamic templates. This can be useful when creating reusable templates or when building complex data structures.
const name = 'John'; const age = 30; function personTemplate(strings, ...values) { const [nameString, ageString] = strings; const formattedName = nameString.replace('${name}', values[0]); const formattedAge = ageString.replace('${age}', values[1]); return `${formattedName}, ${formattedAge}`; } const message = personTemplate`Name: ${name}, Age: ${age}`; console.log(message); // Output: Name: John, Age: 30
In the above example, the personTemplate
function is used to format a person's name and age. The nested template literals allow for dynamic substitution of values within the template.
These advanced techniques demonstrate the flexibility and power of ES6 template literals in JavaScript. By leveraging expressions, multi-line templates, tagged templates, and nested templates, you can create dynamic and readable templates that enhance the clarity and maintainability of your code.
Related Article: How to Apply Ngstyle Conditions in Angular
Advanced Techniques: Template Literals and Internationalization
ES6 template literals provide a powerful tool for simplifying string interpolation in JavaScript. They allow us to embed expressions inside backticks and directly insert their values into the string. However, template literals can do much more than simple variable substitution. In this chapter, we will explore some advanced techniques that leverage template literals to handle internationalization in JavaScript.
One of the main challenges in internationalization is formatting numbers, dates, and currencies according to different locales. JavaScript provides the Intl
object, which offers a set of constructors and methods to handle these tasks. By combining Intl
with template literals, we can easily create localized strings.
Let's start by formatting numbers. The Intl.NumberFormat
constructor allows us to specify the desired locale and options for formatting numbers. We can then use template literals to insert the formatted value into a string. Here's an example:
const number = 1234.56; const formattedNumber = new Intl.NumberFormat('en-US', { style: 'currency', currency: 'USD' }).format(number); console.log(`The total amount is ${formattedNumber}`);
In the example above, we create a Intl.NumberFormat
object with the locale set to 'en-US' and the style set to 'currency'. We then format the number
variable using the format
method and store the result in formattedNumber
. Finally, we use a template literal to insert formattedNumber
into the string.
Next, let's see how we can format dates. The Intl.DateTimeFormat
constructor allows us to specify the locale and options for formatting dates. We can then use template literals to insert the formatted date into a string. Here's an example:
const date = new Date(); const formattedDate = new Intl.DateTimeFormat('en-US', { dateStyle: 'full' }).format(date); console.log(`Today is ${formattedDate}`);
In the example above, we create a Intl.DateTimeFormat
object with the locale set to 'en-US' and the dateStyle
set to 'full'. We then format the current date using the format
method and store the result in formattedDate
. Finally, we use a template literal to insert formattedDate
into the string.
Finally, let's explore how to handle pluralization. The Intl.PluralRules
constructor allows us to determine the plural form of a number based on the locale. We can then use template literals to insert the correct plural form into a string. Here's an example:
const quantity = 5; const pluralRule = new Intl.PluralRules('en-US').select(quantity); console.log(`You have ${quantity} ${pluralRule} item${quantity !== 1 ? 's' : ''}`);
In the example above, we create an Intl.PluralRules
object with the locale set to 'en-US'. We then use the select
method to determine the plural form of quantity
. Finally, we use a template literal to insert quantity
, pluralRule
, and the correct plural suffix into the string.
By combining ES6 template literals with the Intl
object, we can greatly simplify the process of handling internationalization in JavaScript. Whether it's formatting numbers, dates, or handling pluralization, template literals provide a clean and concise way to create localized strings.
Advanced Techniques: Template Literals and Performance
Template literals in JavaScript provide a powerful and convenient way to interpolate variables into strings. In addition to their simplicity and readability, template literals also offer some advanced techniques that can help improve performance in certain scenarios.
1. Raw Strings: Template literals have a special property called "raw", which allows you to access the raw string form without any escaping. This can be useful when working with strings that contain backslashes or other special characters. To access the raw string, you can use the
String.raw
method with the template literal as its argument.
const path = String.raw`C:\Program Files\Node.js`; console.log(path); // Output: C:\Program Files\Node.js
2. Tagged Templates: Template literals can be "tagged" with a function that processes the template. This is achieved by placing the function name immediately before the template literal. The tagged function receives the interpolated values as arguments, along with the raw strings. This technique is especially useful when you need to perform some custom logic or manipulation on the interpolated values.
function highlight(strings, ...values) { let result = ''; strings.forEach((string, index) => { result += string; if (index < values.length) { result += `<mark>${values[index]}</mark>`; } }); return result; } const name = 'John'; const age = 30; const message = highlight`Hello ${name}, you are ${age} years old.`; console.log(message); // Output: Hello <mark>John</mark>, you are <mark>30</mark> years old.
3. Performance Considerations: While template literals are generally efficient, there are a few performance considerations to keep in mind.
- String Concatenation: When using template literals with multiple interpolations, it is more efficient to concatenate the individual parts instead of using nested template literals. This avoids unnecessary string allocations and improves performance.
// Bad performance const fullName = `${firstName} ${lastName}`; // Better performance const fullName = firstName + ' ' + lastName;
- Nested Template Literals: Nesting template literals within each other can result in decreased performance, especially when dealing with a large number of nested interpolations. In such cases, it is recommended to extract the nested template literal into a separate variable for better performance.
// Bad performance const message = `Hello ${name}, your order is ${`$${price}`}.`; // Better performance const nestedTemplate = `$${price}`; const message = `Hello ${name}, your order is ${nestedTemplate}.`;
- Literal Syntax: In certain scenarios, using the regular string literal syntax ('single quotes'
or "double quotes"
) might be more performant than using template literals. This is especially true when there are no interpolations or special characters involved.
// Template literal const name = 'John'; const message = `Hello ${name}!`; // Regular string literal const name = 'John'; const message = 'Hello ' + name + '!';
In summary, template literals provide a concise and powerful way to interpolate variables into strings in JavaScript. By leveraging advanced techniques such as raw strings and tagged templates, you can further enhance your string manipulation capabilities. However, it's important to consider performance implications and use the appropriate syntax and optimizations when necessary.
Best Practices and Common Pitfalls
Template literals in ES6 provide a convenient way to handle string interpolation in JavaScript. However, like any other feature, there are best practices to follow and common pitfalls to avoid when using template literals. In this chapter, we will explore some of these best practices and pitfalls.
1. Properly Escaping Characters: When using template literals, it's important to properly escape characters to avoid syntax errors. Backticks are used to define template literals, and if you need to include a backtick inside the template literal, you must use the backslash (\) to escape it. For example:
const message = `This is a backtick: \` `;
2. Avoiding Injection Attacks: Template literals should not be used to directly inject user input into the template. Doing so can lead to security vulnerabilities like cross-site scripting (XSS) attacks. Always sanitize and validate user input before using it in template literals. For example:
const userInput = 'alert("XSS Attack");'; const sanitizedInput = sanitize(userInput); const message = `Hello, ${sanitizedInput}!`;
3. Using Tagged Templates: Tagged templates are a powerful feature of template literals that allow you to process the template using a function. This can be useful for tasks like internationalization or custom string formatting. Here's an example:
function formatCurrency(strings, ...values) { return values.map((value, index) => { return `${strings[index]}$${value.toFixed(2)}`; }).join(''); } const price = 9.99; const quantity = 3; const total = formatCurrency`The total is ${price * quantity}.`; console.log(total); // Output: The total is $29.97.
4. Avoiding Complex Logic: While template literals can contain expressions, it's generally best to keep the logic simple. Complex logic inside a template can make the code harder to read and maintain. Instead, consider moving the logic to separate functions or variables. For example:
function calculateTotal(price, quantity) { return price * quantity; } const price = 9.99; const quantity = 3; const total = calculateTotal(price, quantity); const message = `The total is ${total}.`; console.log(message); // Output: The total is 29.97.
5. Using Template Literal Tags: Template literal tags allow you to customize the behavior of template literals by specifying a function that will process the template. This can be useful for tasks like escaping HTML or adding custom behavior. Here's an example:
function escapeHtml(strings, ...values) { return strings.reduce((result, string, index) => { const value = values[index] || ''; return result + string + value.replace(/[&"']/g, (char) => { switch (char) { case '&': return '&'; case '': return '>'; case '"': return '"'; case "'": return '''; default: return char; } }); }, ''); } const userInput = 'alert("XSS Attack");'; const message = escapeHtml`Hello, ${userInput}!`; console.log(message); // Output: Hello, <script>alert("XSS Attack");</script>!
By following these best practices and being aware of common pitfalls, you can leverage the power of template literals in ES6 and simplify your string interpolation in JavaScript.
Troubleshooting Template Literals
Template literals in JavaScript provide a concise and powerful way to work with strings. However, like any other feature, they can sometimes cause issues or errors. In this section, we will explore some common troubleshooting scenarios when working with template literals.
Related Article: JavaScript Objects & Managing Complex Data Structures
1. Syntax Errors
One of the most common issues when using template literals is encountering syntax errors. These errors occur when the syntax of the template literal is incorrect, such as missing backticks or improperly placed placeholders. Let's take a look at an example:
const name = 'John'; console.log(`Hello, ${name}; // SyntaxError: Unterminated template literal
In this example, we forgot to add the closing backtick at the end of the template literal, which results in a syntax error. To fix this, we simply need to add the missing backtick:
console.log(`Hello, ${name}`); // Hello, John
Remember to always double-check the syntax of your template literals to avoid these kinds of errors.
2. Escaping Characters
Another common issue when working with template literals is the need to include special characters within the string. In JavaScript, you can escape characters using the backslash (\) to ensure they are treated as part of the string and not as part of the template literal syntax. For example:
const message = `This is a backtick: \``; console.log(message); // This is a backtick: `
In this case, we used the backslash to escape the backtick character, so it is treated as a regular character within the template literal.
3. Mixing Quotes
Sometimes, you may want to include single or double quotes within your template literals. However, if you do not handle them correctly, it can lead to syntax errors. To include quotes within a template literal, you can use either single or double quotes, depending on the context. Here's an example:
const message = `I'm using single quotes inside a template literal`; console.log(message); // I'm using single quotes inside a template literal
In this example, we used single quotes to wrap the template literal, allowing us to use double quotes within the string without any issues.
4. Function Calls and Expressions
Template literals can also include function calls and expressions within placeholders. However, it's important to ensure that the function calls and expressions are valid and return the expected values. Let's see an example:
function getName() { return 'John'; } console.log(`Hello, ${getName()}`); // Hello, John
In this case, the getName
function is called within the template literal, and its return value is interpolated into the string. It's crucial to make sure the function returns the correct value to avoid unexpected results.
Related Article: How To Get A Timestamp In Javascript
5. Mixing Template Literals with HTML
Template literals are commonly used in web development to generate dynamic HTML content. However, when mixing template literals with HTML, you may encounter issues with special characters or the structure of the HTML code. To avoid this, you can use JavaScript libraries or frameworks that handle HTML templating, such as React or Vue.js.
6. Browser Compatibility
Although template literals are widely supported in modern browsers, older browsers may not fully support this ES6 feature. If you need to support older browsers, you can use transpilers like Babel to convert your code to compatible syntax.
In this chapter, we explored some common troubleshooting scenarios when working with template literals in JavaScript. Understanding and addressing these issues will help you utilize template literals effectively in your code.