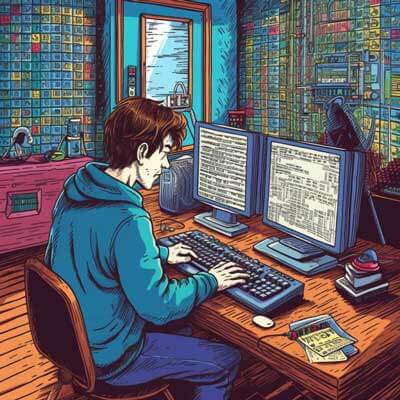
- Redirecting the Output of a Bash Script
- Redirecting Standard Output to a File
- Appending Standard Output to a File
- Suppressing Printing in a Bash Script
- Silencing a Command with the Null Device
- Redirecting Standard Output and Error to the Null Device
- Disabling Printing to the Terminal from a Bash Script
- Redirecting Standard Output and Error to /dev/null
- Using the “exec” Command to Disable Printing
- Discarding the Output of a Command in a Bash Script
- Using the “>/dev/null” Syntax
- Using the “2>/dev/null” Syntax
- Running a Bash Script in Silent Mode
- Redirecting Standard Output and Error to /dev/null
- Using the “-q” or “–quiet” Option
Redirecting the Output of a Bash Script
In Linux, when running a bash script, the output of the script is typically displayed in the terminal. However, there may be situations where you want to redirect the output of a bash script to a file or another location instead of displaying it on the terminal. This can be useful for logging purposes or when you want to save the output for later analysis.
There are several ways to redirect the output of a bash script in Linux. Let’s explore some of the common methods:
Related Article: Adding Color to Bash Scripts in Linux
Redirecting Standard Output to a File
One way to redirect the output of a bash script is by using the “>” operator to redirect the standard output to a file. Here’s an example:
#!/bin/bash echo "This is a sample output" > output.txt
In this example, the echo
command is used to print the text “This is a sample output” to the standard output. The “>” operator is then used to redirect the standard output to a file named “output.txt”. If the file doesn’t exist, it will be created. If the file already exists, its contents will be overwritten.
After running the script, you can check the contents of the “output.txt” file using a text editor or the cat
command:
cat output.txt
This will display the contents of the “output.txt” file, which should be “This is a sample output”.
Appending Standard Output to a File
If you want to append the output of a bash script to an existing file instead of overwriting it, you can use the “>>” operator. Here’s an example:
#!/bin/bash echo "This is another sample output" >> output.txt
In this example, the “>>” operator is used to append the standard output to the “output.txt” file. If the file doesn’t exist, it will be created. If the file already exists, the output will be appended to the end of the file.
After running the script, you can check the contents of the “output.txt” file again:
cat output.txt
This time, the file should contain both the previous output (“This is a sample output”) and the new output (“This is another sample output”).
Suppressing Printing in a Bash Script
Sometimes, you may want to suppress the printing of certain commands or output in a bash script. This can be useful when you have debugging statements or verbose output that you don’t want to display to the user. There are several ways to achieve this in Linux. Let’s explore some of the common methods:
Related Article: How to Calculate the Sum of Inputs in Bash Scripts
Silencing a Command with the Null Device
One way to suppress the printing of a command in a bash script is by redirecting its output to the null device (“/dev/null”). The null device is a special device file in Linux that discards all data written to it and provides no data when read from.
Here’s an example:
#!/bin/bash echo "This will be printed" echo "This will be silenced" > /dev/null echo "This will be printed again"
In this example, the second echo
command is redirected to the null device using the “>” operator. As a result, the output of the command will not be displayed in the terminal.
After running the script, you should see the following output:
This will be printed This will be printed again
The second echo
command, which outputs “This will be silenced”, is not displayed.
Redirecting Standard Output and Error to the Null Device
In addition to suppressing the printing of a command, you may also want to silence any error messages that the command produces. You can achieve this by redirecting both the standard output and error streams to the null device. Here’s an example:
#!/bin/bash echo "This will be printed" command_that_may_produce_error > /dev/null 2>&1 echo "This will be printed again"
In this example, the command_that_may_produce_error
is executed, and both its standard output and error streams are redirected to the null device using the “> /dev/null 2>&1” syntax. The “2>&1” part redirects the error stream (file descriptor 2) to the same location as the standard output (file descriptor 1).
After running the script, you should see the following output:
This will be printed This will be printed again
Any error messages produced by the command_that_may_produce_error
command will not be displayed in the terminal.
Disabling Printing to the Terminal from a Bash Script
In some cases, you may want to completely disable the printing of any output to the terminal from a bash script. This can be useful when you want to run a script silently without any visible output. There are a few ways to achieve this in Linux. Let’s explore some of the common methods:
Related Article: Locating Largest Memory in Bash Script on Linux
Redirecting Standard Output and Error to /dev/null
One way to disable printing to the terminal from a bash script is by redirecting both the standard output and error streams to the null device (“/dev/null”). This is similar to the previous method of suppressing printing, but in this case, all output is discarded instead of being redirected to a file.
Here’s an example:
#!/bin/bash echo "This will not be printed" command_that_may_produce_output_or_error > /dev/null 2>&1
In this example, the standard output and error streams of the command_that_may_produce_output_or_error
command are redirected to the null device using the “> /dev/null 2>&1” syntax. This effectively disables any printing to the terminal from the script.
After running the script, you should not see any output in the terminal.
Using the “exec” Command to Disable Printing
Another way to disable printing to the terminal from a bash script is by using the “exec” command to redirect the standard output and error streams to the null device. This method is particularly useful when you want to disable printing for the entire script.
Here’s an example:
#!/bin/bash echo "This will not be printed" exec > /dev/null 2>&1 # Any commands below this line will not produce any visible output command_that_may_produce_output_or_error
In this example, the “exec” command is used to redirect the standard output and error streams to the null device. The “> /dev/null 2>&1” syntax is used to redirect both streams to the null device.
After running the script, you should not see any output in the terminal.
Discarding the Output of a Command in a Bash Script
Sometimes, you may want to execute a command in a bash script but discard its output, without redirecting it to a file or suppressing it completely. This can be useful when you’re only interested in the return status of the command and don’t care about its output. There are a few ways to achieve this in Linux. Let’s explore some of the common methods:
Related Article: Terminate Bash Script Loop via Keyboard Interrupt in Linux
Using the “>/dev/null” Syntax
One way to discard the output of a command in a bash script is by redirecting its standard output to the null device (“/dev/null”). This is similar to the previous methods of redirecting output to the null device, but in this case, the output is discarded instead of being redirected to a file.
Here’s an example:
#!/bin/bash command_that_may_produce_output > /dev/null
In this example, the standard output of the command_that_may_produce_output
command is redirected to the null device using the “> /dev/null” syntax. This effectively discards the output of the command.
After running the script, you should not see any output in the terminal.
Using the “2>/dev/null” Syntax
If you only want to discard the error output of a command, you can use the “2>/dev/null” syntax to redirect the error stream to the null device. This allows you to still see the standard output of the command, but discard any error messages it produces.
Here’s an example:
#!/bin/bash command_that_may_produce_error 2>/dev/null
In this example, the error stream of the command_that_may_produce_error
command is redirected to the null device using the “2>/dev/null” syntax. This effectively discards any error messages produced by the command.
After running the script, you should not see any error messages in the terminal.
Running a Bash Script in Silent Mode
Running a bash script in silent mode means executing the script without displaying any output or error messages in the terminal. This can be useful when you want to run a script quietly or in the background without any visible output. There are several ways to achieve this in Linux. Let’s explore some of the common methods:
Related Article: Displaying Memory Usage in Bash Scripts on Linux
Redirecting Standard Output and Error to /dev/null
One way to run a bash script in silent mode is by redirecting both the standard output and error streams to the null device (“/dev/null”). This effectively disables any printing to the terminal from the script.
Here’s an example:
#!/bin/bash exec > /dev/null 2>&1 # Any commands below this line will not produce any visible output or error messages command1 command2 command3
In this example, the “exec” command is used to redirect the standard output and error streams to the null device. The “> /dev/null 2>&1” syntax is used to redirect both streams to the null device.
After running the script, you should not see any output or error messages in the terminal.
Using the “-q” or “–quiet” Option
Some commands or utilities provide a built-in option to run in silent or quiet mode. This option typically suppresses any unnecessary output or error messages. You can use this option when invoking the command in your bash script to run it silently.
Here’s an example:
#!/bin/bash command --quiet
In this example, the “command” is executed with the “–quiet” option. This option tells the command to run in silent mode and suppress any unnecessary output or error messages.
After running the script, you should not see any output or error messages in the terminal.