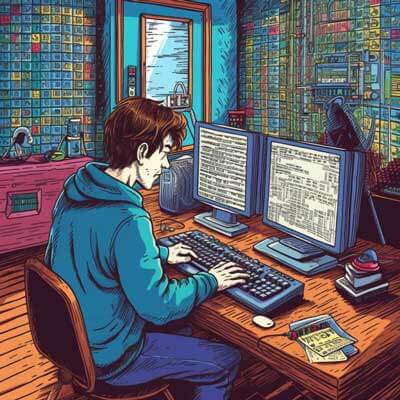
- Ignoring Quotes in String Comparison
- Comparing Strings without Considering Quotes
- Handling Quoted Strings in String Comparison
- Techniques for String Comparison without Quotes
- Comparing Strings with Double Brackets
- Using the Test Command for String Comparison
- Comparing Strings with the [[ Operator
- Performing Case-Insensitive String Comparison
- Checking if a String is Empty or Null
- Comparing Strings with the == Operator
- Comparing Strings with the != Operator
- Using Pattern Matching for String Comparison
- Performing String Comparison with Regular Expressions
- Best Practices for String Comparison in Bash
When working with strings in a Bash script, you may come across situations where you need to compare strings regardless of whether they are enclosed in quotes or not. This can be particularly useful when dealing with user input or handling data from external sources. In this article, we will explore various techniques and operators in Bash that allow us to compare strings without considering the presence of quotes.
Ignoring Quotes in String Comparison
To compare strings without considering the presence of quotes, you can remove the quotes before comparison. This can be done using parameter substitution with the //
operator.
Here’s an example:
string1="hello 'world'" string2="hello world" string1_without_quotes="${string1//\'/}" string2_without_quotes="$string2" if [[ $string1_without_quotes == $string2_without_quotes ]]; then echo "Strings are equal" else echo "Strings are not equal" fi
In this example, the single quotes are removed from string1
using parameter substitution, and then the two strings, string1_without_quotes
and string2_without_quotes
, are compared. Since the strings are equal, the output will be “Strings are equal”.
Related Article: Adding Color to Bash Scripts in Linux
Comparing Strings without Considering Quotes
If you need to compare strings without considering the presence of quotes, you can use the same approach as before, but apply it to both strings.
Here’s an example:
string1="'hello'" string2="hello" string1_without_quotes="${string1//\'/}" string2_without_quotes="${string2//\'/}" if [[ $string1_without_quotes == $string2_without_quotes ]]; then echo "Strings are equal" else echo "Strings are not equal" fi
In this example, both string1
and string2
are processed to remove the single quotes, and then the resulting strings are compared. Since the strings are equal, the output will be “Strings are equal”.
Handling Quoted Strings in String Comparison
When comparing strings in Bash, it’s important to handle quoted strings correctly. Quoted strings may include special characters that need to be escaped before comparison.
Here’s an example:
string1="hello 'world'" string2="hello world" if [[ $string1 == $string2 ]]; then echo "Strings are equal" else echo "Strings are not equal" fi
In this example, the two strings, string1
and string2
, are not equal because the first string includes single quotes that are not present in the second string. The output will be “Strings are not equal”.
Techniques for String Comparison without Quotes
When comparing strings without considering quotes, there are multiple techniques you can use. Some of these techniques include:
1. Removing quotes using parameter substitution, as shown in the previous examples.
2. Using regular expressions to match patterns within the strings.
3. Converting the strings to a common format, such as lowercase or uppercase, before comparison.
The choice of technique depends on your specific requirements and the nature of the strings you are comparing.
Related Article: How to Calculate the Sum of Inputs in Bash Scripts
Comparing Strings with Double Brackets
One of the most common ways to compare strings in Bash is by using the double brackets syntax. The double brackets, [[ ]]
, provide advanced string comparison capabilities and are recommended over the single brackets, [ ]
, for string comparison.
The double brackets syntax allows you to use operators such as ==
and !=
for string comparison. Here’s an example:
string1="hello" string2="HELLO" if [[ $string1 == $string2 ]]; then echo "Strings are equal" else echo "Strings are not equal" fi
In this example, the ==
operator is used to compare the two strings, string1
and string2
. Since the comparison is case-sensitive, the output will be “Strings are not equal”.
Using the Test Command for String Comparison
Another way to compare strings in Bash is by using the test
command with the -z
and -n
options. The -z
option checks if a string is empty, while the -n
option checks if a string is not empty.
Here’s an example:
string="hello" if test -z "$string"; then echo "String is empty" else echo "String is not empty" fi
In this example, the -z
option is used to check if the string is empty. Since the string is not empty, the output will be “String is not empty”.
Comparing Strings with the [[ Operator
The double brackets syntax, [[ ]]
, also provides additional operators for string comparison, such as <
, >
, <=
, and >=
. These operators can be used to compare strings lexicographically.
Here’s an example:
string1="apple" string2="banana" if [[ $string1 < $string2 ]]; then echo "$string1 comes before $string2" else echo "$string1 comes after $string2" fi
In this example, the <
operator is used to compare the two strings, string1
and string2
. Since “apple” comes before “banana” lexicographically, the output will be “apple comes before banana”.
Related Article: Locating Largest Memory in Bash Script on Linux
Performing Case-Insensitive String Comparison
Here’s an example:
shopt -s nocasematch string1="Hello" string2="hello" if [[ $string1 == $string2 ]]; then echo "Strings are equal" else echo "Strings are not equal" fi shopt -u nocasematch
In this example, the nocasematch
shell option is enabled using the shopt -s
command. This allows the ==
operator to perform case-insensitive comparison. Since “Hello” is equal to “hello” regardless of case, the output will be “Strings are equal”.
Checking if a String is Empty or Null
Before comparing strings, you may need to check if a string is empty or null. This can be done using the -z
option with the test
command, as mentioned earlier.
Here’s an example:
string="" if test -z "$string"; then echo "String is empty or null" else echo "String is not empty or null" fi
In this example, the -z
option is used to check if the string is empty or null. Since the string is empty, the output will be “String is empty or null”.
Comparing Strings with the == Operator
The ==
operator is used to compare strings for equality in Bash. This operator performs a pattern matching comparison and can handle quoted strings.
Here’s an example:
string1="hello" string2="hello" if [[ $string1 == $string2 ]]; then echo "Strings are equal" else echo "Strings are not equal" fi
In this example, the ==
operator is used to compare the two strings, string1
and string2
. Since the strings are equal, the output will be “Strings are equal”.
Related Article: Terminate Bash Script Loop via Keyboard Interrupt in Linux
Comparing Strings with the != Operator
The !=
operator is used to compare strings for inequality in Bash. This operator performs a pattern matching comparison and can handle quoted strings.
Here’s an example:
string1="hello" string2="world" if [[ $string1 != $string2 ]]; then echo "Strings are not equal" else echo "Strings are equal" fi
In this example, the !=
operator is used to compare the two strings, string1
and string2
. Since the strings are not equal, the output will be “Strings are not equal”.
Using Pattern Matching for String Comparison
Pattern matching can be used for more complex string comparison in Bash. The case
statement can be used with pattern matching to compare strings based on specific patterns.
Here’s an example:
string="apple" case $string in a*) echo "String starts with 'a'" ;; *e) echo "String ends with 'e'" ;; *) echo "String does not match any pattern" ;; esac
In this example, the case
statement is used to compare the string, string
, based on different patterns. Since the string starts with ‘a’, the output will be “String starts with ‘a'”.
Performing String Comparison with Regular Expressions
Regular expressions can also be used for string comparison in Bash. The =~
operator is used with regular expressions to compare strings.
Here’s an example:
string="hello123" if [[ $string =~ [0-9]+ ]]; then echo "String contains one or more digits" else echo "String does not contain any digits" fi
In this example, the =~
operator is used to compare the string, string
, with the regular expression [0-9]+
. Since the string contains one or more digits, the output will be “String contains one or more digits”.
Related Article: Displaying Memory Usage in Bash Scripts on Linux
Best Practices for String Comparison in Bash
When comparing strings in Bash, it’s important to follow some best practices to ensure accurate and reliable comparisons:
1. Use the double brackets, [[ ]]
, for string comparison instead of the single brackets, [ ]
.
2. Consider the case-sensitivity of the comparison and use the appropriate operators or options accordingly.
3. Be mindful of special characters and quotes in the strings and handle them correctly.
4. Test your string comparison code with various scenarios to ensure it behaves as expected.
5. Document your string comparison code to make it easier for others to understand and maintain.