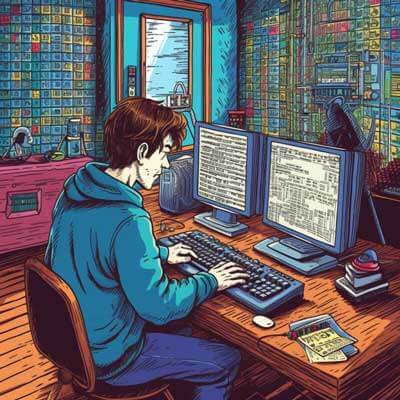
Table of Contents
Parallel Processing in Bash Scripts
Parallel processing is a technique that allows multiple tasks to be executed simultaneously, improving performance and efficiency. While traditionally associated with languages like C++ or Java, it is also possible to achieve parallelism in Bash scripts on Linux. This can be particularly useful in scenarios where a script needs to perform computationally intensive tasks or handle multiple I/O operations simultaneously.
One way to achieve parallel processing in Bash scripts is by making use of background processes. By running commands or functions in the background, they can execute concurrently with the main script, allowing for parallel execution.
Here's an example of how you can use background processes to achieve parallel processing in a Bash script:
#!/bin/bash # Function to perform a computationally intensive task function compute { echo "Computing..." sleep 5 echo "Done!" } # Run the compute function in the background compute & # Perform other tasks in the main script echo "Performing other tasks..." # Wait for the background process to finish wait echo "All tasks completed."
In this example, the compute
function performs a computationally intensive task, simulated by the sleep
command. By running it in the background with the &
symbol, it executes concurrently with the rest of the script. The wait
command is then used to wait for the background process to finish before proceeding with the rest of the script.
Related Article: Using SSH to Connect to a Remote Server in Linux
Concurrent Execution in Bash Scripts
While parallel processing allows multiple tasks to be executed simultaneously, concurrent execution refers to the ability to handle multiple tasks in an interleaved manner. This can be achieved in Bash scripts through the use of job control and process substitution.
Job control allows for the manipulation of multiple processes within a script, including starting, stopping, and managing their execution. Process substitution, on the other hand, enables processes to communicate with each other through pipes.
Here's an example of how you can achieve concurrent execution in a Bash script:
#!/bin/bash # Function to perform a task function task { echo "Executing task $1" sleep 2 echo "Task $1 completed" } # Execute tasks concurrently using job control and process substitution task 1 & task 2 & # Wait for all tasks to finish wait echo "All tasks completed."
In this example, the task
function performs a simple task, simulated by the sleep
command. By running the tasks in the background with the &
symbol, they execute concurrently. The wait
command is then used to wait for all background processes to finish before proceeding with the rest of the script.
Threading in Bash Scripts
While Bash is primarily a scripting language and not designed for multi-threading, it is still possible to achieve threading-like behavior through the use of background processes and job control.
One approach to simulate threading in Bash scripts is by dividing the workload into smaller tasks and assigning each task to a separate background process. By carefully managing the communication and synchronization between these processes, you can achieve a similar effect to multi-threading.
Here's an example of how you can simulate threading in a Bash script:
#!/bin/bash # Function to perform a task function task { echo "Executing task $1" sleep 2 echo "Task $1 completed" } # Number of threads NUM_THREADS=4 # Array to hold background process IDs PIDS=() # Execute tasks in separate threads for ((i=0; i<NUM_THREADS; i++)); do task $i & PIDS+=($!) done # Wait for all threads to finish for pid in "${PIDS[@]}"; do wait $pid done echo "All threads completed."
In this example, the task
function performs a task, simulated by the sleep
command. The script divides the workload into four separate tasks and assigns each task to a separate background process. The process IDs of the background processes are stored in the PIDS
array. The script then waits for each background process to finish using the wait
command and the process ID. Once all background processes have finished, the script proceeds with the rest of the execution.
Multi-threading in Linux
Multi-threading is a technique that allows multiple threads of execution to run concurrently within a single process. Each thread has its own set of registers and stack, but shares the same memory space as other threads within the process. This enables efficient communication and synchronization between threads.
While Bash scripts do not natively support multi-threading, Linux provides various mechanisms to achieve multi-threading in other programming languages, such as C or C++. These mechanisms include libraries like pthreads and OpenMP, which provide APIs for creating and managing threads.
Here's an example of how you can achieve multi-threading in C using the pthreads library:
#include <stdio.h> #include <pthread.h> // Function to be executed by each thread void *thread_function(void *arg) { int thread_id = *((int *)arg); printf("Thread %d executing\n", thread_id); // Perform thread-specific tasks return NULL; } int main() { pthread_t threads[4]; int thread_args[4]; // Create threads for (int i = 0; i < 4; i++) { thread_args[i] = i; pthread_create(&threads[i], NULL, thread_function, &thread_args[i]); } // Wait for threads to finish for (int i = 0; i < 4; i++) { pthread_join(threads[i], NULL); } printf("All threads completed\n"); return 0; }
In this example, the thread_function
is the function to be executed by each thread. The pthread_create
function is used to create threads, passing the thread function and the thread-specific arguments. The pthread_join
function is then used to wait for each thread to finish before proceeding with the rest of the execution.
While this example is written in C, similar multi-threading concepts can be applied in other languages that support the pthreads library, such as C++.
Related Article: How to Use Mkdir Only If a Directory Doesn't Exist in Linux
Background Processes in Bash
Background processes in Bash allow commands or functions to be executed concurrently with the main script. They are denoted by the &
symbol, which can be appended to a command or function call to run it in the background.
Here's an example of how you can run a command in the background in a Bash script:
#!/bin/bash # Run a command in the background command & echo "Continue with the rest of the script."
In this example, the command
is executed in the background by appending the &
symbol after it. The script then continues with the rest of the execution without waiting for the background process to finish.
Background processes are useful when you want to execute tasks concurrently or when you want to run long-running tasks in the background while performing other operations in the script.
Process Synchronization in Bash
Process synchronization is the coordination of multiple processes to ensure proper execution and avoid conflicts. In Bash scripts, process synchronization can be achieved through various mechanisms, such as signals, locks, or inter-process communication (IPC) methods like pipes or shared memory.
Here's an example of how you can use a lock file for process synchronization in a Bash script:
#!/bin/bash # Lock file path LOCK_FILE="/tmp/lockfile" # Acquire the lock if mkdir "$LOCK_FILE"; then echo "Lock acquired" else echo "Failed to acquire lock" exit 1 fi # Critical section echo "Executing critical section" sleep 5 # Release the lock rmdir "$LOCK_FILE" echo "Lock released"
In this example, a lock file is used to ensure that only one instance of the script can execute the critical section at a time. The script tries to create a directory with the mkdir
command, which will succeed only if the directory does not exist. If the mkdir
command succeeds, it means that the lock has been acquired and the critical section can be executed. Once the critical section is completed, the lock is released by removing the directory with the rmdir
command.
Process synchronization is crucial in scenarios where multiple processes or threads need to access shared resources or when a certain order of execution needs to be maintained.
Bash Script Performance Optimization
Optimizing the performance of Bash scripts can significantly improve their execution time and efficiency. While Bash is not as performant as low-level languages like C or C++, there are several techniques and best practices that can be applied to optimize the performance of Bash scripts.
Here are some tips for optimizing the performance of Bash scripts:
1. Use built-in Bash commands and operators: Bash provides a wide range of built-in commands and operators that are optimized for performance. Whenever possible, use built-in commands instead of external commands or scripts.
2. Minimize I/O operations: I/O operations, such as reading from or writing to files, can be costly in terms of performance. Minimize the number of I/O operations by buffering or combining multiple operations into a single operation.
3. Use efficient data structures: Bash supports arrays and associative arrays, which can be used to store and manipulate data efficiently. Choose the appropriate data structure for your script's needs to optimize memory usage and access times.
4. Limit the use of external commands: External commands executed by Bash scripts can introduce overhead due to process creation and communication. Minimize the use of external commands by utilizing built-in Bash functionality whenever possible.
5. Use efficient loops and iterations: Bash provides various loops and iterations, such as for
and while
loops. Use the most efficient loop construct for your script's requirements to minimize overhead and improve performance.
6. Optimize string manipulation: String manipulation operations, such as concatenation or substitution, can be resource-intensive. Use efficient string manipulation techniques, such as parameter expansion or command substitution, to optimize performance.
7. Avoid unnecessary variable expansion: Unnecessary variable expansion can introduce overhead and impact performance. Use single quotes instead of double quotes when variable expansion is not required.
8. Profile and benchmark your script: Use tools like time
or strace
to profile and benchmark your script's performance. Identify performance bottlenecks and areas for improvement.
Implementing Multi-threading in a Bash Script
While Bash does not natively support multi-threading, it is still possible to implement multi-threading behavior in Bash scripts using background processes and job control.
Here's an example of how you can implement multi-threading in a Bash script:
#!/bin/bash # Function to be executed by each thread function thread_function { local thread_id=$1 echo "Thread $thread_id executing" # Perform thread-specific tasks } # Number of threads NUM_THREADS=4 # Array to hold background process IDs PIDS=() # Execute threads for ((i=0; i<NUM_THREADS; i++)); do thread_function $i & PIDS+=($!) done # Wait for all threads to finish for pid in "${PIDS[@]}"; do wait $pid done echo "All threads completed"
In this example, the thread_function
is the function to be executed by each thread. The script creates a specified number of threads by running the thread_function
in the background using the &
symbol. The process IDs of the background processes are stored in the PIDS
array. The script then waits for each background process to finish using the wait
command and the process ID. Once all background processes have finished, the script proceeds with the rest of the execution.
While this approach simulates multi-threading behavior, it's important to note that Bash scripts are best suited for sequential, single-threaded tasks. For complex multi-threaded applications, it is recommended to use languages specifically designed for multi-threading, such as C or C++.
Related Article: Locating and Moving Files in Bash Scripting on Linux
Simultaneous Execution of Multiple Tasks in a Bash Script
Simultaneous execution of multiple tasks in a Bash script can be achieved through the use of background processes and job control. By running tasks in the background, they can execute concurrently with the main script, allowing for simultaneous execution.
Here's an example of how you can achieve simultaneous execution of multiple tasks in a Bash script:
#!/bin/bash # Function to perform a task function task { echo "Executing task $1" sleep 2 echo "Task $1 completed" } # Execute tasks simultaneously using background processes task 1 & task 2 & # Wait for all tasks to finish wait echo "All tasks completed"
In this example, the task
function performs a task, simulated by the sleep
command. The script runs multiple tasks in the background by appending the &
symbol after each task. The script then waits for all background processes to finish using the wait
command. Once all tasks have completed, the script proceeds with the rest of the execution.
Simultaneous execution of multiple tasks can be beneficial in scenarios where tasks are independent of each other and can be executed in parallel, improving overall performance and efficiency.
Advantages of Multi-threading in a Bash Script
Multi-threading in a Bash script offers several advantages, including:
1. Improved performance: Multi-threading allows multiple threads to execute concurrently, enabling faster execution of computationally intensive tasks or handling multiple I/O operations simultaneously.
2. Efficient resource utilization: Multi-threading enables efficient utilization of system resources, such as CPU cores, by distributing the workload across multiple threads. This can lead to better overall system performance and resource utilization.
3. Enhanced responsiveness: By leveraging multi-threading, a Bash script can be more responsive and interactive, as it can continue executing tasks in the background while still accepting user input or responding to external events.
4. Simplified code organization: Multi-threading allows for better code organization by dividing complex tasks into smaller, more manageable threads. This can improve code readability and maintainability.
5. Simplified task coordination: Multi-threading enables easy coordination and synchronization between threads, allowing for efficient sharing of data and resources. This simplifies the implementation of complex algorithms or workflows that require coordination between multiple tasks.
Overall, multi-threading in a Bash script can significantly improve the performance, responsiveness, and efficiency of the script, making it a valuable technique in certain scenarios.
Synchronization between Threads in a Bash Script
Synchronization between threads is essential to ensure proper coordination and avoid conflicts when multiple threads are executing concurrently. While Bash scripts do not natively support multi-threading, you can still achieve synchronization between threads using various synchronization primitives available in Linux.
One such synchronization primitive is the use of locks. Locks allow threads to gain exclusive access to a shared resource, ensuring that only one thread can modify it at a time. Bash scripts can utilize locks through the use of lock files.
Here's an example of how you can implement synchronization between threads using locks in a Bash script:
#!/bin/bash # Lock file path LOCK_FILE="/tmp/lockfile" # Acquire the lock if mkdir "$LOCK_FILE"; then echo "Lock acquired" else echo "Failed to acquire lock" exit 1 fi # Critical section echo "Executing critical section" sleep 5 # Release the lock rmdir "$LOCK_FILE" echo "Lock released"
In this example, a lock file is used to ensure that only one instance of the script can execute the critical section at a time. The script tries to create a directory with the mkdir
command, which will succeed only if the directory does not exist. If the mkdir
command succeeds, it means that the lock has been acquired and the critical section can be executed. Once the critical section is completed, the lock is released by removing the directory with the rmdir
command.
Synchronization between threads is crucial to prevent race conditions and ensure the integrity of shared resources. By utilizing synchronization primitives like locks, Bash scripts can achieve thread synchronization and coordination.
Limitations of Multi-threading in Bash
While multi-threading in Bash scripts can offer improved performance and efficiency, there are certain limitations to be aware of. These limitations stem from the nature of Bash as a scripting language and its lack of native support for multi-threading.
Here are some limitations of multi-threading in Bash:
1. Limited control over thread execution: Bash does not provide granular control over thread execution, such as thread priorities or scheduling policies. The operating system handles the scheduling and execution of threads, which can limit the level of control and customization.
2. Lack of thread isolation: Bash threads share the same memory space, making it challenging to achieve complete isolation between threads. This can lead to potential data race conditions or conflicts when multiple threads access or modify shared resources simultaneously.
3. Limited scalability: Bash scripts are primarily designed for sequential, single-threaded execution. While background processes and job control can simulate multi-threading behavior, they may not scale well for complex multi-threaded applications with a large number of threads.
4. Lack of built-in thread synchronization mechanisms: Bash does not provide built-in mechanisms for thread synchronization, such as mutexes or condition variables. Achieving thread synchronization in Bash often requires the use of external tools or synchronization primitives, such as lock files or signals.
5. Performance overhead: The use of background processes and job control in Bash scripts can introduce performance overhead due to process creation and communication. This overhead may limit the scalability and performance benefits of multi-threading in Bash.
Despite these limitations, multi-threading in Bash can still be useful in certain scenarios where the advantages outweigh the limitations. However, for more complex multi-threaded applications, it is recommended to use languages specifically designed for multi-threading, such as C or C++.
Related Article: Creating a Bash Script for a MySQL Database Backup
Impact of Multi-threading on Bash Script Performance
Multi-threading can have a significant impact on the performance of Bash scripts, both positive and negative. The impact of multi-threading on Bash script performance depends on various factors, such as the nature of the tasks being performed, the number of threads, and the system's hardware capabilities.
Here are some potential impacts of multi-threading on Bash script performance:
1. Improved performance: Multi-threading can improve performance by allowing multiple threads to execute concurrently, effectively utilizing available CPU resources. This can lead to faster execution of computationally intensive tasks or handling multiple I/O operations simultaneously.
2. Increased resource utilization: Multi-threading can increase resource utilization, particularly CPU and memory resources. By distributing the workload across multiple threads, multi-threading can make better use of available resources, leading to improved overall system performance.
3. Scalability limitations: While multi-threading can improve performance, it may not scale well for complex multi-threaded applications with a large number of threads. The overhead associated with thread creation, synchronization, and communication can limit the scalability and performance benefits of multi-threading in Bash.
4. Potential synchronization overhead: Achieving proper synchronization between threads can introduce overhead and impact performance. Synchronization primitives, such as locks or semaphores, can introduce delays and contention when multiple threads try to access shared resources simultaneously.
5. Interference and contention: In certain scenarios, multi-threading can lead to interference and contention between threads, resulting in decreased performance. This can occur when multiple threads compete for the same shared resources or when frequent context switching between threads imposes overhead.
6. Overhead of background processes: Multi-threading in Bash is achieved through the use of background processes and job control. The creation and management of these background processes can introduce overhead in terms of process creation, context switching, and communication, potentially impacting performance.
To fully understand the impact of multi-threading on Bash script performance, it is important to consider the specific characteristics of the script, the tasks being performed, and the hardware capabilities of the system.
Best Practices for Writing Multi-threaded Bash Scripts
When writing multi-threaded Bash scripts, it is important to follow best practices to ensure proper execution, synchronization, and maintainability. While Bash is not designed for multi-threading, these best practices can help you achieve multi-threaded behavior in a more reliable and efficient manner.
Here are some best practices for writing multi-threaded Bash scripts:
1. Use background processes and job control: Background processes and job control are the primary mechanisms for achieving multi-threading in Bash scripts. Use the &
symbol to run tasks in the background and the wait
command to wait for background processes to finish.
2. Implement proper thread synchronization: Proper synchronization between threads is crucial to avoid race conditions and conflicts. Utilize synchronization primitives such as locks or semaphores to coordinate access to shared resources.
3. Avoid global variables: Global variables can introduce unintended side effects and make it difficult to reason about thread behavior. Instead, pass thread-specific data as function arguments or use local variables.
4. Minimize shared resource access: Minimize the access to shared resources to avoid contention and potential conflicts. Identify critical sections that require exclusive access and implement proper synchronization mechanisms.
5. Limit the number of threads: While multi-threading can improve performance, too many threads can introduce overhead and impact overall performance. Determine the optimal number of threads based on the specific characteristics of the script and the system's hardware capabilities.
6. Implement error handling and graceful termination: Proper error handling and graceful termination are essential in multi-threaded scripts. Implement mechanisms to handle errors, propagate them to the main thread, and ensure that all threads are terminated properly.
7. Profile and benchmark: Profile and benchmark your multi-threaded Bash scripts to identify performance bottlenecks and areas for improvement. Use tools like time
or strace
to analyze the script's performance and identify areas for optimization.
8. Consider alternatives: If your multi-threaded script becomes too complex or performance-critical, consider using languages specifically designed for multi-threading, such as C or C++. These languages provide more granular control over thread execution and better performance optimization.
Utilizing Multiple Processor Cores in a Bash Script
Utilizing multiple processor cores in a Bash script can significantly improve performance and efficiency by distributing the workload across multiple threads. While Bash scripts do not natively support multi-threading, you can achieve multi-threading behavior by utilizing background processes and job control.
Here's an example of how you can utilize multiple processor cores in a Bash script:
#!/bin/bash # Function to be executed by each thread function thread_function { local thread_id=$1 echo "Thread $thread_id executing" # Perform thread-specific tasks } # Number of threads NUM_THREADS=$(nproc) # Array to hold background process IDs PIDS=() # Execute threads for ((i=0; i<NUM_THREADS; i++)); do thread_function $i & PIDS+=($!) done # Wait for all threads to finish for pid in "${PIDS[@]}"; do wait $pid done echo "All threads completed"
In this example, the thread_function
is the function to be executed by each thread. The script determines the number of available processor cores using the nproc
command and assigns it to the NUM_THREADS
variable. The script then creates a thread for each processor core by running the thread_function
in the background using the &
symbol. The process IDs of the background processes are stored in the PIDS
array. The script waits for each background process to finish using the wait
command and the process ID. Once all background processes have finished, the script proceeds with the rest of the execution.
Tools and Libraries for Multi-threading in Bash
While Bash does not natively support multi-threading, there are several tools and libraries available that can help achieve multi-threading behavior in Bash scripts. These tools and libraries provide additional functionality and synchronization mechanisms that can enhance the multi-threading capabilities of Bash.
Here are some tools and libraries for multi-threading in Bash:
1. GNU Parallel: GNU Parallel is a command-line tool that allows for the parallel execution of commands or scripts. It provides an easy-to-use interface for executing tasks in parallel, utilizing multiple processor cores. GNU Parallel can be installed on Linux systems using package managers like apt or yum.
2. Bash Threader: Bash Threader is a lightweight library that provides thread-like behavior in Bash scripts. It abstracts the complexities of background processes and job control, allowing for easier implementation of multi-threading. Bash Threader can be sourced in Bash scripts and used to create and manage threads.
3. flock: The flock command is a built-in utility in Bash that provides file-based locking. It can be used to synchronize access to shared resources between threads or processes by acquiring and releasing locks on files. The flock command is part of the util-linux package, which is typically pre-installed on Linux systems.
4. Named pipes: Named pipes, or FIFOs, can be used to facilitate communication between threads or processes. By creating named pipes, threads can write data to the pipe for consumption by other threads. Named pipes can be created using the mkfifo command and read from or written to using standard file I/O operations.
5. Signals: Signals can be used for inter-process communication and synchronization in Bash scripts. By sending and handling signals, threads or processes can coordinate actions, communicate status, or trigger specific behaviors. Signals can be sent using the kill command and handled using the trap command.
These tools and libraries provide additional functionality and synchronization mechanisms that can enhance the multi-threading capabilities of Bash scripts. Depending on the specific requirements of your script, one or more of these tools or libraries may be suitable for your needs.
Related Article: Tutorial: Using Unzip Command in Linux
Debugging Multi-threaded Bash Scripts
Debugging multi-threaded Bash scripts can be challenging due to the nature of background processes and job control. However, there are several techniques and tools available that can help in debugging and troubleshooting multi-threaded Bash scripts.
Here are some techniques and tools for debugging multi-threaded Bash scripts:
1. Logging and debugging statements: Insert logging statements throughout your script to track the execution flow and identify potential issues. These statements can be simple echo
commands that output debug information to the console or a log file. By carefully placing logging statements, you can gain insight into the execution of different threads and identify potential synchronization or race condition issues.
2. Signal handling: Utilize signal handling to catch and handle specific signals that can indicate issues or trigger debugging actions. For example, you can use the trap
command to catch the SIGUSR1 signal and execute a specific debugging function or print debug information.
3. Debugging tools: Make use of debugging tools like strace
or gdb
to track system calls and analyze the behavior of your script. These tools can provide valuable insights into the execution of background processes, file operations, or process communication.
4. Shellcheck: Use Shellcheck, a static analysis tool for shell scripts, to identify potential issues, syntax errors, or common mistakes in your multi-threaded Bash script. Shellcheck can help you catch potential bugs or best practices violations before running your script.
5. Reproducible test cases: Create reproducible test cases that trigger the issue you are trying to debug. By isolating the problematic scenario and creating a minimal test case, you can focus on the specific issue and eliminate unnecessary complexity.
6. Code review and pair programming: Seek assistance from colleagues or fellow developers to review your multi-threaded Bash script. Another pair of eyes can help identify potential issues or suggest alternative approaches for your script.
Debugging multi-threaded Bash scripts can be challenging, but by utilizing these techniques and tools, you can effectively identify and resolve issues in your script.