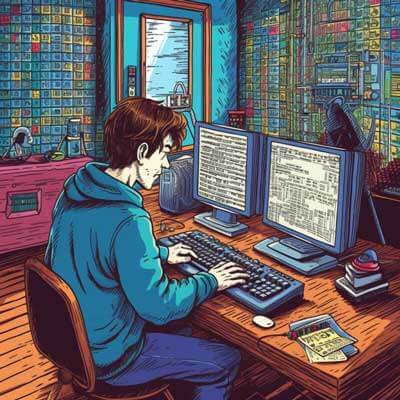
Table of Contents
How to source a Bash script into a Makefile?
To source a Bash script into a Makefile, you can use the include
directive in your Makefile. The include
directive reads and processes the specified file as if its contents were included directly in the Makefile.
Here's an example of how to source a Bash script into a Makefile:
Makefile:
include script.sh build: @echo "Building..." @$(call my_function) .PHONY: build
script.sh:
#!/bin/bash my_function() { echo "This is a sourced function" }
In the above example, the include
directive is used to source the script.sh
Bash script into the Makefile. The build
target in the Makefile then calls the my_function
sourced from the script.
When the Makefile is executed, it will include the contents of the script.sh
file and make the my_function
available for use in the build
target.
Related Article: Using Variables in If Statements in Bash Scripts
Advantages of sourcing a Bash script into a Makefile
Sourcing a Bash script into a Makefile offers several advantages:
1. Code reuse: Sourcing allows you to reuse common Bash code across multiple Makefiles. This promotes code modularity and reduces duplication.
2. Maintainability: By separating functionality into individual Bash scripts, Makefiles become easier to read, understand, and maintain. Changes made to the sourced script will be reflected in all Makefiles that include it.
3. Flexibility: Sourcing Bash scripts gives you the flexibility to extend and customize the build process without modifying the Makefile directly. You can add or modify functions and variables in the sourced script to suit your specific needs.
4. Integration: Sourcing Bash scripts enables seamless integration with external tools and utilities. You can encapsulate complex commands or configurations in the script and include it in your Makefile, making it easier to incorporate external dependencies.
Limitations and caveats of sourcing a Bash script into a Makefile
While sourcing a Bash script into a Makefile offers many benefits, there are some limitations and caveats to be aware of:
1. Variable scoping: Sourced scripts have access to the variables and environment of the calling Makefile or shell session. However, changes made to variables in the sourced script will not be reflected in the calling Makefile or shell session. This is because each script runs in its own process.
2. Makefile syntax: Sourced scripts should not contain Makefile-specific syntax. Makefiles use a different syntax and set of rules compared to Bash scripts. Mixing the two can lead to syntax errors and unexpected behavior.
3. Order of sourcing: The order in which scripts are sourced can affect the behavior of the Makefile. Make sure to carefully consider the order in which scripts are included to avoid conflicts or unintended consequences.
4. File paths: Sourced scripts should use absolute file paths or paths relative to the Makefile location. Relative paths may not work as expected, especially when the Makefile is executed from a different directory.
5. Dependencies: If a sourced script has dependencies on other scripts or tools, those dependencies must be satisfied either by including the dependencies in the Makefile or by ensuring they are available in the system environment.
Can you source multiple Bash scripts into a Makefile?
Yes, you can source multiple Bash scripts into a Makefile. This can be useful when you have different scripts for different purposes or when you want to modularize your build process further.
To source multiple Bash scripts into a Makefile, you can use multiple include
directives, each specifying the path to a different script. Here's an example:
Makefile:
include script1.sh include script2.sh build: @echo "Building..." @$(call function1) @$(call function2) .PHONY: build
script1.sh:
#!/bin/bash function1() { echo "This is function 1" }
script2.sh:
#!/bin/bash function2() { echo "This is function 2" }
In the above example, the Makefile includes both script1.sh
and script2.sh
using separate include
directives. The build
target then calls function1
from script1.sh
and function2
from script2.sh
.
When the Makefile is executed, it will include the contents of both scripts and make the functions available for use in the build
target.
Related Article: Executing Bash Scripts with Chef Apply in Linux
Passing arguments to a sourced Bash script in a Makefile
To pass arguments to a sourced Bash script in a Makefile, you can use variables defined in the Makefile and pass them as arguments when calling the sourced functions.
Here's an example:
Makefile:
include script.sh build: @echo "Building..." @$(call my_function,$(ARG)) .PHONY: build
script.sh:
#!/bin/bash my_function() { echo "This is a sourced function" echo "Argument passed: $1" }
In the above example, the Makefile includes the script.sh
Bash script and defines a variable ARG
. The build
target then calls the my_function
function from the sourced script, passing $(ARG)
as the argument.
When executing the Makefile, you can pass the argument using the ARG
variable:
make build ARG="example argument"
The output will be:
Building... This is a sourced function Argument passed: example argument
Best practices for sourcing Bash scripts into Makefiles
When sourcing Bash scripts into Makefiles, it's important to follow some best practices to ensure maintainability and avoid potential issues:
1. Use clear naming conventions: Choose descriptive names for your Bash scripts and Makefile targets to make it easier to understand their purpose and functionality.
2. Define functions and variables in the sourced script: Encapsulate reusable code and configurations within functions and variables defined in the sourced script. This promotes code modularity and reusability.
3. Document the usage and dependencies: Include comments in your sourced script and Makefile to document the usage, dependencies, and any specific requirements for the sourced script.
4. Separate Makefile-specific logic from the sourced script: Makefiles have their own syntax and rules. Keep Makefile-specific logic in the Makefile itself and use the sourced script for generic Bash code and configurations.
5. Test and validate the sourced script separately: Before sourcing a script into a Makefile, test and validate it independently to ensure it works as expected. This can help avoid issues when the script is used in different contexts.
Debugging issues when sourcing a Bash script into a Makefile
When encountering issues when sourcing a Bash script into a Makefile, there are several steps you can take to debug and identify the problem:
1. Check file paths: Ensure that the paths to the sourced script and any referenced files or dependencies are correct and accessible from the Makefile. Use absolute file paths or paths relative to the Makefile location to avoid path resolution issues.
2. Verify script syntax: Make sure the sourced script has the correct Bash syntax and does not contain any errors or typos. You can manually execute the script from the command line to check for syntax errors or use a linter or syntax checker for Bash scripts.
3. Debugging output: Add debugging statements or echo commands to the sourced script and Makefile to print relevant information or variables. This can help you identify where the issue occurs and what values are being used.
4. Test with a minimal example: If the issue persists, create a minimal example with only the necessary code and dependencies to isolate the problem. This can help identify specific lines or sections causing the issue.
5. Check for conflicting variables or functions: Ensure that there are no variable or function name conflicts between the sourced script and the Makefile. Conflicting names can lead to unexpected behavior or errors.
6. Debugging tools: Make use of debugging tools and utilities available for Bash scripts and Makefiles. These tools can help trace the execution flow, inspect variables, and identify potential issues.
Alternatives to sourcing Bash scripts into Makefiles
While sourcing Bash scripts into Makefiles is a common approach, there are alternative methods you can consider depending on your specific requirements and preferences:
1. Inline commands: Instead of sourcing a separate Bash script, you can directly include the necessary commands in the Makefile itself. This can be useful for small, one-off tasks or when the functionality is straightforward and does not require external scripts.
2. External shell scripts: Rather than sourcing Bash scripts, you can execute them as external shell commands using the shell
function in Makefiles. This allows you to leverage the power of Bash scripting without the need for sourcing.
3. Build automation tools: If your build process requires more advanced features or complex logic, you may consider using build automation tools specifically designed for that purpose. Tools like CMake, Gradle, or Maven provide comprehensive build management capabilities and can handle more complex scenarios.
4. Configuration management tools: For larger projects or infrastructure management, using configuration management tools like Ansible, Chef, or Puppet may be more appropriate. These tools provide a higher-level abstraction for managing system configurations and can also execute Bash scripts as part of their workflows.
Related Article: Executing Bash Scripts at Startup in Ubuntu Linux
Sourcing a Makefile into a Bash script
In addition to sourcing Bash scripts into Makefiles, it is also possible to source a Makefile into a Bash script. This can be useful when you want to reuse Makefile targets or use Makefile variables within a Bash script.
To source a Makefile into a Bash script, you can use the source
or .
command followed by the path to the Makefile. Here's an example:
#!/bin/bash source Makefile # Use Makefile variables echo "Variable value: $VARIABLE" # Call Makefile targets build
In the above example, the Bash script sources the Makefile using the source
command and then uses Makefile variables and calls Makefile targets within the Bash script.
When executing the Bash script, it will include the contents of the Makefile and make the variables and targets available for use.
Note that sourcing a Makefile into a Bash script may not always be necessary or the most appropriate approach, as Makefiles and Bash scripts have different purposes and functionality. Consider the specific requirements and context of your project before deciding to source a Makefile into a Bash script.
What is a Makefile?
A Makefile is a special file used by the make utility to automate the build process of software projects. It contains a set of rules that define how to compile and link source code files to create executable programs or libraries. Make is a build automation tool that determines which files need to be recompiled and then invokes the necessary compiler commands.
Makefiles are commonly used in software development projects to manage complex build processes. They can specify dependencies between source files, include compiler flags and options, define targets and prerequisites, and perform various other build-related tasks.
Makefiles are written in a simple and concise domain-specific language. They consist of a series of rules, each consisting of a target, prerequisites, and commands. The target is the file or action that needs to be built, the prerequisites are the files or actions required to build the target, and the commands are the actions to be executed.
Here's an example of a simple Makefile that compiles a C program:
CC = gcc CFLAGS = -Wall -Werror hello: hello.c $(CC) $(CFLAGS) -o hello hello.c
What is sourcing in Bash?
In Bash scripting, sourcing refers to executing the contents of one script within another script. When a script is sourced, its commands and variables become part of the current shell session. This allows the sourced script to modify the environment and variables of the calling script.
Sourcing a script is different from executing it as a standalone script. When a script is executed as a standalone script, it runs in its own separate shell process and any changes it makes to the environment or variables do not affect the calling script.
Sourcing is often used to share common functions, variables, or configurations between multiple scripts. It allows scripts to be modular and reusable, as different scripts can include and use the same sourced script.
Sourcing a script in Bash is done using the dot (.) or source command followed by the path to the script. Here's an example:
. /path/to/script.sh
Why should you source a Bash script into a Makefile?
Sourcing a Bash script into a Makefile can be useful in several scenarios:
1. Reusing Bash code: If you have a Bash script that contains useful functions or configuration settings, you can source it into a Makefile to reuse that code. This allows you to avoid duplicating code and ensures consistency across scripts.
2. Modularity and maintainability: By sourcing Bash scripts, you can split your Makefile into smaller, more manageable parts. Each sourced script can handle a specific aspect of the build process, making the Makefile easier to understand and maintain.
3. Environment setup: Sourcing a Bash script into a Makefile allows you to set up the environment for the build process. This can include setting environment variables, initializing paths, or configuring other system-specific settings.
4. Integration with external tools: If you need to integrate external tools or utilities into your build process, you can use a Bash script to encapsulate the necessary commands and configurations. Sourcing this script into your Makefile allows you to seamlessly incorporate these tools into your build workflow.