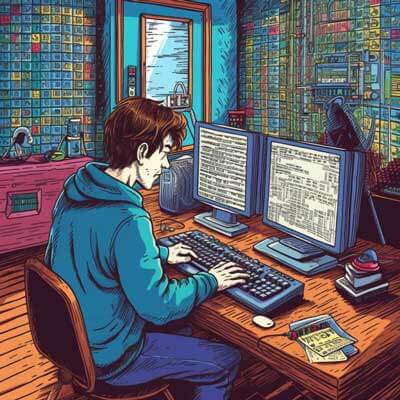
- Introduction to Loops in Bash
- Understanding the While Loop in Bash
- Exploring the Do While Loop in Bash
- Comparing Do While Loop and While Loop in Bash
- Writing a For Loop in Bash Script
- Using If Statements in Bash Scripting
- Common Bash Commands for Linux Terminal
- Syntax for a Do While Loop in Bash
- Step-by-Step Guide to Creating a Do While Loop in Bash
Introduction to Loops in Bash
Loops are essential when it comes to automating repetitive tasks or iterating over a collection of items. Bash provides several loop constructs to accomplish this, including the while loop, do-while loop, and for loop. In this article, we will focus on the do-while loop.
Related Article: How To Echo a Newline In Bash
Understanding the While Loop in Bash
The while loop in Bash executes a block of code as long as a specified condition is true. It follows this general syntax:
while [ condition ]; do # Code to be executed done
Here’s an example that prints the numbers 1 to 5 using a while loop:
counter=1 while [ $counter -le 5 ]; do echo $counter counter=$((counter + 1)) done
In this example, the counter variable is initialized to 1. The while loop continues executing as long as the counter is less than or equal to 5. Inside the loop, the current value of the counter is printed, and then the counter is incremented by 1.
Exploring the Do While Loop in Bash
The do-while loop in Bash is similar to the while loop, but with one key difference: the code block is executed at least once before checking the condition. It follows this general syntax:
do # Code to be executed done while [ condition ]
Let’s modify the previous example to use a do-while loop instead:
counter=1 do echo $counter counter=$((counter + 1)) done while [ $counter -le 5 ]
In this example, the code block inside the do-while loop is executed first, regardless of the condition. Then, the condition is checked. If the condition is true, the loop continues executing, and if the condition is false, the loop terminates.
Comparing Do While Loop and While Loop in Bash
The key difference between the do-while loop and the while loop in Bash is the order in which the condition is checked. In a while loop, the condition is checked before executing the code block, while in a do-while loop, the code block is executed at least once before checking the condition.
The choice between the two loop constructs depends on the specific requirements of your script. If you need to ensure that a code block executes at least once, even if the condition is initially false, then a do-while loop is appropriate. On the other hand, if you want to skip the code block entirely when the condition is false, then a while loop is more suitable.
Related Article: How to Use If-Else Statements in Shell Scripts
Writing a For Loop in Bash Script
The for loop in Bash is used to iterate over a sequence of values, such as an array or a range of numbers. It follows this general syntax:
for variable in value1 value2 ... valuen; do # Code to be executed done
Here’s an example that prints the numbers 1 to 5 using a for loop:
for ((i=1; i<=5; i++)); do echo $i done
In this example, the for loop initializes the variable “i” to 1 and continues executing as long as “i” is less than or equal to 5. After each iteration, the value of “i” is incremented by 1.
Using If Statements in Bash Scripting
If statements are used to perform different actions based on certain conditions. In Bash scripting, the if statement is written as follows:
if [ condition ]; then # Code to be executed if the condition is true fi
Here’s an example that checks if a number is positive:
number=10 if [ $number -gt 0 ]; then echo "The number is positive" fi
In this example, the if statement checks if the value of the “number” variable is greater than 0. If it is, the message “The number is positive” is displayed.
Common Bash Commands for Linux Terminal
The Linux terminal provides a wide range of useful commands that can be used in Bash scripts. Here are some common Bash commands:
– echo
: Prints a message or variable value.
– read
: Reads input from the user.
– if
: Executes code based on a condition.
– for
: Iterates over a sequence of values.
– while
: Executes code as long as a condition is true.
– case
: Executes code based on multiple conditions.
– function
: Defines a reusable block of code.
– grep
: Searches for a pattern in a file or output.
– sed
: Edits text using regular expressions.
– awk
: Processes text files using pattern scanning and processing language.
– chmod
: Changes the permissions of a file or directory.
– chown
: Changes the owner and group of a file or directory.
– rm
: Removes files or directories.
– cp
: Copies files or directories.
– mv
: Moves or renames files or directories.
– ls
: Lists files and directories.
– cd
: Changes the current directory.
– pwd
: Prints the current working directory.
These are just a few examples of the many Bash commands available in the Linux terminal. Each command has its own set of options and arguments, which can be explored further in the respective command’s documentation.
Related Article: How to Manipulate Quotes & Strings in Bash Scripts
Syntax for a Do While Loop in Bash
The syntax for a do-while loop in Bash is as follows:
do # Code to be executed done while [ condition ]
In this syntax, the code block inside the do
keyword is executed first, regardless of the condition. Then, the condition is checked. If the condition is true, the loop continues executing, and if the condition is false, the loop terminates.
Here’s an example of a do-while loop that prints the numbers 1 to 5:
counter=1 do echo $counter counter=$((counter + 1)) done while [ $counter -le 5 ]
In this example, the do
keyword signifies the start of the loop, and the condition [ $counter -le 5 ]
is checked after each iteration.
Step-by-Step Guide to Creating a Do While Loop in Bash
To create a do-while loop in Bash, follow these steps:
1. Open a text editor of your choice.
2. Start the script with the shebang line #!/bin/bash
.
3. Write the code block that you want to execute inside the loop.
4. Add the do
keyword to signify the start of the loop.
5. Write the condition that will be checked after each iteration.
6. Add the done
keyword to signify the end of the loop.
Here’s an example of a do-while loop that prompts the user for input until a valid number is entered:
#!/bin/bash number=0 do read -p "Enter a number: " number done while ! [[ $number =~ ^[0-9]+$ ]] echo "Valid number entered: $number"
In this example, the script prompts the user to enter a number using the read
command. The loop continues until a valid number (consisting of digits only) is entered. The condition ! [[ $number =~ ^[0-9]+$ ]]
checks if the value of the number
variable does not match the regular expression pattern [0-9]+
, which represents one or more digits.