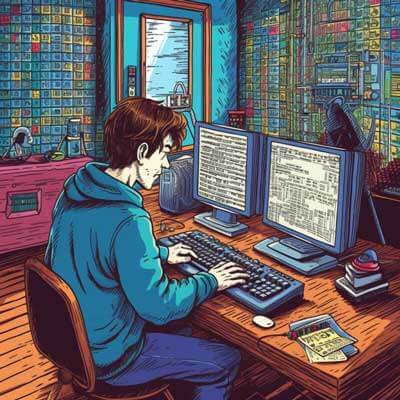
Table of Contents
To write multiple if statements in a bash script, you can use the if
keyword followed by a condition, and then specify the commands to be executed if the condition is true. You can also use the elif
keyword to specify additional conditions to be checked, and the else
keyword to specify commands to be executed if none of the conditions are true.
Here's an example of a bash script with multiple if statements:
#!/bin/bash # Check if the current user is root if [[ $EUID -eq 0 ]]; then echo "You are running this script as root." elif [[ $EUID -gt 1000 ]]; then echo "You are running this script as a regular user." else echo "You are running this script as a system user." fi # Check if a file exists if [[ -f "myfile.txt" ]]; then echo "The file myfile.txt exists." else echo "The file myfile.txt does not exist." fi
In this example, the script first checks if the current user is root using the if
statement. If the condition $EUID -eq 0
is true, it prints "You are running this script as root." If not, it proceeds to check the next condition using the elif
statement. If none of the conditions are true, it executes the commands specified in the else
statement.
The script then checks if a file named "myfile.txt" exists using the if
statement. If the condition -f "myfile.txt"
is true, it prints "The file myfile.txt exists." Otherwise, it prints "The file myfile.txt does not exist."
Can you have nested if statements in a bash script?
Yes, nested if statements are allowed in a bash script. A nested if statement is an if statement that is contained within another if statement. This allows for more complex conditional logic and the ability to check multiple conditions.
Here's an example of a bash script with nested if statements:
#!/bin/bash # Check if the current user is root if [[ $EUID -eq 0 ]]; then echo "You are running this script as root." else # Check if the current user is in the sudo group if [[ $(groups) == *sudo* ]]; then echo "You are running this script as a user with sudo privileges." else echo "You are running this script as a regular user." fi fi
In this example, the script first checks if the current user is root using the outer if statement. If the condition $EUID -eq 0
is true, it prints "You are running this script as root." If not, it proceeds to the else block and checks if the current user is in the sudo group using the inner if statement. If the condition $(groups) == *sudo*
is true, it prints "You are running this script as a user with sudo privileges." Otherwise, it prints "You are running this script as a regular user."
Related Article: Interactions between Bash Scripts and Linux Command History
What are some examples of conditional statements in bash scripting?
Conditional statements in bash scripting allow you to perform different actions based on the evaluation of a condition. Here are some examples of conditional statements in bash scripting:
Example 1: Check if a number is even or odd
#!/bin/bash read -p "Enter a number: " num if [[ $((num % 2)) -eq 0 ]]; then echo "$num is an even number." else echo "$num is an odd number." fi
In this example, the script prompts the user to enter a number and then uses the if statement to check if the number is even or odd. It does this by evaluating the condition $((num % 2)) -eq 0
. If the condition is true, it prints "$num is an even number." Otherwise, it prints "$num is an odd number."
Example 2: Check if a file exists and is readable
#!/bin/bash read -p "Enter a file path: " file if [[ -f "$file" && -r "$file" ]]; then echo "The file $file exists and is readable." else echo "The file $file either does not exist or is not readable." fi
In this example, the script prompts the user to enter a file path and then uses the if statement to check if the file exists and is readable. It does this by evaluating the condition -f "$file" && -r "$file"
. If the condition is true, it prints "The file $file exists and is readable." Otherwise, it prints "The file $file either does not exist or is not readable."
These examples demonstrate how conditional statements in bash scripting can be used to make decisions based on different conditions and perform specific actions accordingly.
Are if statements the only way to perform conditional execution in bash?
No, if statements are not the only way to perform conditional execution in bash. Bash provides several other constructs for conditional execution, including the case
statement and the test
command.
The case
statement allows for multiple conditional branches based on a single value. It is often used as an alternative to multiple if
statements when there are many possible conditions to check.
Here's an example of using the case
statement:
#!/bin/bash read -p "Enter a fruit: " fruit case $fruit in apple) echo "You entered an apple." ;; banana) echo "You entered a banana." ;; *) echo "You entered an unknown fruit." ;; esac
In this example, the script uses the case
statement to check the value of the variable $fruit
and execute different commands based on the value. If the value is "apple", it prints "You entered an apple." If the value is "banana", it prints "You entered a banana." If the value is anything else, it prints "You entered an unknown fruit."
The test
command, also known as the [
command, allows for conditional checks within a single command or as part of a larger command.
Here's an example of using the test
command:
#!/bin/bash read -p "Enter a number: " num if [ $num -eq 0 ]; then echo "The number is zero." elif [ $num -gt 0 ]; then echo "The number is positive." else echo "The number is negative." fi
In this example, the script uses the test
command to check if the value of the variable $num
is zero, positive, or negative. The condition [ $num -eq 0 ]
checks if the number is zero, [ $num -gt 0 ]
checks if the number is greater than zero, and the else
block handles all other cases.
So, while if statements are a common and widely used construct for conditional execution in bash, they are not the only way to perform such operations. The choice between if statements, case statements, and the test command depends on the specific requirements and complexity of the script.
What are some best practices for writing if statements in bash scripts?
When writing if statements in bash scripts, it is important to follow certain best practices to ensure readability, maintainability, and efficiency. Here are some best practices for writing if statements in bash scripts:
1. Use double square brackets: It is recommended to use double square brackets ([[ ]]
) instead of single square brackets ([ ]
) for conditional checks. Double square brackets provide additional features and improvements over single square brackets, such as pattern matching and support for logical operators.
2. Quote variables: When using variables in conditions, it is best practice to quote them to handle cases where the variable may contain spaces or special characters. For example, use "$var"
instead of $var
.
3. Indent code: Indent the code within if statements to improve readability. Use tabs or spaces consistently to align the commands within the if statement block.
4. Provide comments: Add comments to explain the purpose and logic of the if statements. This helps other developers understand the code and makes it easier to maintain and debug.
5. Avoid unnecessary nesting: Try to avoid excessive nesting of if statements, as it can make the code harder to read and understand. If possible, refactor the code to eliminate nested if statements and use logical operators (&&
, ||
) to combine conditions.
6. Use the exit status: Take advantage of the exit status of commands or conditions to simplify if statements. For example, instead of writing if [ -f "$file" ] && [ -r "$file" ]
, you can write if [ -f "$file" -a -r "$file" ]
.
Related Article: Executing Bash Scripts at Startup in Ubuntu Linux
What is the syntax for if statements in bash?
The syntax for if statements in bash is as follows:
if condition then # commands to be executed if the condition is true elif condition then # commands to be executed if the previous condition is false and this condition is true else # commands to be executed if none of the conditions are true fi
The if
keyword is used to start the if statement, followed by a condition enclosed in double square brackets ([[ ]]
). The condition can be any expression that evaluates to true or false. If the condition is true, the commands between then
and elif
(if present) are executed. If the condition is false, the script proceeds to the elif
statement and checks the next condition. If none of the conditions are true, the commands between else
and fi
are executed.
It is important to note that the then
, elif
, and else
keywords must be placed on separate lines and indented for readability. The fi
keyword is used to end the if statement.
Here's an example of the syntax for if statements in bash:
if [[ condition1 ]] then # commands to be executed if condition1 is true elif [[ condition2 ]] then # commands to be executed if condition1 is false and condition2 is true else # commands to be executed if neither condition1 nor condition2 is true fi
In this example, the script checks two conditions, condition1
and condition2
, using the if
and elif
statements respectively. The commands between then
and elif
are executed if condition1
is true, and the commands between elif
and else
are executed if condition1
is false and condition2
is true. If neither condition is true, the commands between else
and fi
are executed.
Additional Resources
- Bash Scripting Tutorial for Beginners