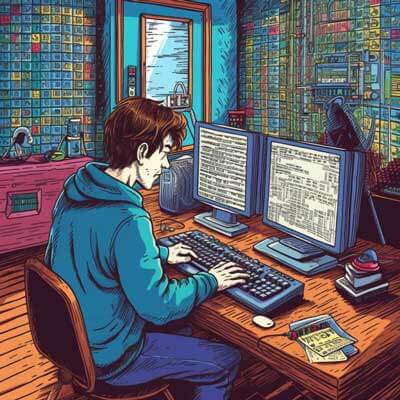
- Practical Tips for MongoDB Performance Optimization
- 1. Use Indexes
- 2. Avoid Large Result Sets
- Query Optimization Techniques for MongoDB
- 1. Use Query Selectivity
- 2. Utilize Query Projection
- Improving MongoDB Query Speed
- 1. Avoid Regular Expressions
- 2. Use Covered Queries
- Indexing in MongoDB for Better Query Performance
- 1. Single Field Index
- 2. Compound Index
- Caching Mechanisms in MongoDB to Speed Up Queries
- 1. MongoDB Query Cache
- 2. External Caching Solutions
- Utilizing Sharding in MongoDB to Improve Query Performance
- 1. Enable Sharding
- 2. Choose the Right Shard Key
- Best Practices to Avoid Nested Queries in MongoDB
- 1. Use {{EJS51}} with {{EJS52}} Instead of Nested Queries
- 2. Denormalize Data
- Using the Aggregation Framework in MongoDB to Optimize Queries
- 1. Use Indexes in Aggregation Pipelines
- 2. Utilize Aggregation Pipeline Stages
- Optimizing Read/Write Operations in MongoDB
- 1. Use Bulk Write Operations
- 2. Use Write Concerns
- Leveraging Covered Queries in MongoDB for Improved Performance
- 1. Indexes and Projection
- 2. Avoid Sort Operations
- Monitoring and Analyzing MongoDB Query Performance
- 1. Use the MongoDB Profiler
- 2. Utilize Database Profiling Tools
Practical Tips for MongoDB Performance Optimization
When working with MongoDB, it’s important to optimize your queries to ensure efficient and fast performance. In this section, we will discuss some practical tips for MongoDB performance optimization.
Related Article: Tutorial: Using Python to Interact with MongoDB Collections
1. Use Indexes
Indexes play a crucial role in improving query performance in MongoDB. They allow the database to quickly locate and retrieve the requested data. By creating appropriate indexes on the fields commonly used in queries, you can significantly speed up your MongoDB queries.
Here’s an example of creating an index on a collection using the MongoDB shell:
db.collection.createIndex({ field: 1 });
In the example above, collection
is the name of your collection and field
is the name of the field you want to create an index on. The value 1
indicates an ascending index, while -1
indicates a descending index.
2. Avoid Large Result Sets
Fetching large result sets from MongoDB can negatively impact query performance. It’s important to limit the number of documents returned by your queries by using the limit()
method. This ensures that only the necessary data is fetched, reducing the query execution time.
Here’s an example of using the limit()
method:
db.collection.find().limit(10);
The example above limits the result set to 10 documents.
Query Optimization Techniques for MongoDB
In this section, we will explore some query optimization techniques to further improve the performance of your MongoDB queries.
Related Article: Exploring MongoDB: Does it Load Documents When Querying?
1. Use Query Selectivity
Query selectivity refers to the proportion of documents in a collection that match a query. By designing your queries to be more selective, you can reduce the amount of data that needs to be scanned, resulting in faster query execution.
For example, instead of using a query like this:
db.collection.find({ status: "active" });
You can make it more selective by adding additional criteria:
db.collection.find({ status: "active", category: "electronics" });
2. Utilize Query Projection
Query projection allows you to specify which fields to include or exclude from the query results. By retrieving only the necessary fields, you can reduce the amount of data transferred from the database to the client, improving query performance.
Here’s an example of using query projection to include only specific fields:
db.collection.find({}, { name: 1, age: 1 });
The example above retrieves only the name
and age
fields from the documents.
Improving MongoDB Query Speed
In this section, we will discuss techniques to further improve the speed of your MongoDB queries.
Related Article: How to Add a Field with a Blank Value in MongoDB
1. Avoid Regular Expressions
Regular expressions can be resource-intensive and slow down your queries. If possible, avoid using regular expressions in your MongoDB queries or use them sparingly.
For example, instead of using a regular expression to match a string:
db.collection.find({ name: /john/ });
You can use the $regex
operator with an index-friendly query:
db.collection.find({ name: { $regex: "^john" } });
2. Use Covered Queries
A covered query is a query that can be satisfied entirely using the index and does not need to access the actual documents. This can significantly improve query performance by reducing disk I/O and network traffic.
To create a covered query, you need to ensure that all the fields in the query and projection are covered by an index. Here’s an example:
db.collection.find({ status: "active" }, { _id: 0, name: 1, age: 1 }).hint({ status: 1 });
In the example above, the index on the status
field is used to satisfy the query and projection.
Indexing in MongoDB for Better Query Performance
Indexes play a crucial role in improving query performance in MongoDB. In this section, we will explore different indexing techniques to optimize your MongoDB queries.
Related Article: How to Use Range Queries in MongoDB
1. Single Field Index
A single field index is the most basic type of index in MongoDB. It indexes a single field and allows for efficient retrieval of data based on that field.
Here’s an example of creating a single field index:
db.collection.createIndex({ field: 1 });
In the example above, collection
is the name of your collection and field
is the name of the field you want to create an index on. The value 1
indicates an ascending index, while -1
indicates a descending index.
2. Compound Index
A compound index is an index that includes multiple fields. It allows for efficient retrieval of data based on multiple fields.
Here’s an example of creating a compound index:
db.collection.createIndex({ field1: 1, field2: -1 });
In the example above, field1
and field2
are the names of the fields you want to create a compound index on. The value 1
indicates an ascending index for field1
, while -1
indicates a descending index for field2
.
Caching Mechanisms in MongoDB to Speed Up Queries
Caching can greatly improve the performance of your MongoDB queries by reducing the amount of time spent on retrieving data from the database. In this section, we will explore caching mechanisms that you can utilize in MongoDB.
Related Article: Crafting Query Operators in MongoDB
1. MongoDB Query Cache
MongoDB has an internal query cache that can cache the results of frequently performed read queries. By enabling the query cache, MongoDB can quickly retrieve the results from memory instead of executing the query again.
To enable the query cache, you can set the queryCacheEnabled
option to true
in your MongoDB configuration file.
2. External Caching Solutions
In addition to the internal query cache, you can also utilize external caching solutions such as Redis or Memcached to cache the results of your MongoDB queries. These caching solutions can provide even faster access to frequently accessed data.
Here’s an example of using Redis to cache the results of a MongoDB query:
const redis = require('redis'); const client = redis.createClient(); function getCachedData(callback) { client.get('cachedData', (err, reply) => { if (reply) { callback(JSON.parse(reply)); } else { db.collection.find().toArray((err, data) => { client.setex('cachedData', 3600, JSON.stringify(data)); callback(data); }); } }); }
In the example above, the getCachedData
function first checks if the data is available in the Redis cache. If it is, it retrieves the data from the cache. Otherwise, it retrieves the data from MongoDB and stores it in the cache for future use.
Utilizing Sharding in MongoDB to Improve Query Performance
Sharding is a technique used in MongoDB to distribute data across multiple servers. It can improve query performance by allowing for parallel processing and reducing the load on individual servers. In this section, we will explore how to utilize sharding in MongoDB.
Related Article: Using Multi-Indexes with MongoDB Queries
1. Enable Sharding
To enable sharding, you need to connect to your MongoDB deployment and run the following commands:
sh.enableSharding("database"); sh.shardCollection("database.collection", { "_id": "hashed" });
In the example above, database
is the name of your database and collection
is the name of your collection. The { "_id": "hashed" }
option specifies that documents should be distributed based on the hashed value of the _id
field.
2. Choose the Right Shard Key
The shard key determines how data is distributed across the shards in a MongoDB cluster. It’s important to choose a shard key that evenly distributes the data and allows for efficient querying.
For example, if you have a collection of user documents and frequently query by the email
field, you can use the email
field as the shard key:
sh.shardCollection("database.collection", { "email": 1 });
In the example above, the email
field is used as the shard key, and the value 1
indicates an ascending index.
Best Practices to Avoid Nested Queries in MongoDB
Nested queries can be resource-intensive and slow down your MongoDB queries. In this section, we will discuss some best practices to avoid nested queries and improve query performance.
Related Article: MongoDB Queries Tutorial
1. Use $lookup
with $match
Instead of Nested Queries
Instead of using nested queries, you can utilize the $lookup
aggregation stage with the $match
operator to perform a join operation between collections.
Here’s an example of using $lookup
with $match
:
db.orders.aggregate([ { $lookup: { from: "customers", localField: "customerId", foreignField: "_id", as: "customer" } }, { $match: { "customer.country": "USA" } } ]);
In the example above, the $lookup
stage performs a join between the orders
collection and the customers
collection based on the customerId
field. The $match
stage filters the results based on the country of the customer.
2. Denormalize Data
Denormalization involves combining related data into a single document. By denormalizing your data, you can avoid the need for nested queries and improve the performance of your MongoDB queries.
For example, instead of storing customer information in a separate collection and performing a nested query to retrieve the customer details, you can denormalize the data and store the customer information directly in the order documents.
Using the Aggregation Framework in MongoDB to Optimize Queries
The aggregation framework in MongoDB provides useful tools for processing and transforming data. In this section, we will explore how to use the aggregation framework to optimize your MongoDB queries.
Related Article: Tutorial: MongoDB Aggregate Query Analysis
1. Use Indexes in Aggregation Pipelines
Just like regular queries, indexes can greatly improve the performance of aggregation pipelines. By creating appropriate indexes on the fields used in the aggregation stages, you can significantly speed up your MongoDB queries.
Here’s an example of using an index in an aggregation pipeline:
db.collection.aggregate([ { $match: { status: "active" } }, { $group: { _id: "$category", count: { $sum: 1 } } } ]).hint({ status: 1 });
In the example above, the hint()
method specifies the index to use for the aggregation pipeline.
2. Utilize Aggregation Pipeline Stages
The aggregation framework provides various stages that allow you to perform complex transformations and calculations on your data. By utilizing these stages effectively, you can optimize your MongoDB queries.
Here’s an example of using the $project
stage to include only specific fields in the query results:
db.collection.aggregate([ { $match: { status: "active" } }, { $project: { name: 1, age: 1 } } ]);
In the example above, the $project
stage includes only the name
and age
fields in the query results.
Optimizing Read/Write Operations in MongoDB
In this section, we will discuss techniques to optimize read and write operations in MongoDB for improved performance.
Related Article: How to Improve the Speed of MongoDB Queries
1. Use Bulk Write Operations
Bulk write operations allow you to perform multiple write operations in a single request, reducing network round trips and improving write performance.
Here’s an example of using bulk write operations to insert multiple documents:
const bulk = db.collection.initializeUnorderedBulkOp(); bulk.insert({ name: "John" }); bulk.insert({ name: "Jane" }); bulk.insert({ name: "Joe" }); bulk.execute();
In the example above, the initializeUnorderedBulkOp()
method initializes a new bulk write operation, and the execute()
method executes the bulk write operation.
2. Use Write Concerns
Write concerns allow you to control the acknowledgment behavior of MongoDB write operations. By setting appropriate write concerns, you can balance between write performance and data durability.
For example, you can use the { w: 0 }
write concern to perform unacknowledged writes, which can improve write performance at the cost of potential data loss in case of failures.
db.collection.insert({ name: "John" }, { w: 0 });
In the example above, the { w: 0 }
write concern is used to perform an unacknowledged write operation.
Leveraging Covered Queries in MongoDB for Improved Performance
Covered queries are queries that can be satisfied entirely using the index and do not need to access the actual documents. In this section, we will explore how to leverage covered queries for improved query performance in MongoDB.
Related Article: How to Run Geospatial Queries in Nodejs Loopback & MongoDB
1. Indexes and Projection
To create a covered query, you need to ensure that all the fields in the query and projection are covered by an index. By including only the necessary fields in the projection, you can further optimize the performance of covered queries.
Here’s an example of a covered query:
db.collection.find({ status: "active" }, { _id: 0, name: 1, age: 1 }).hint({ status: 1 });
In the example above, the index on the status
field is used to satisfy the query, and the projection includes only the name
and age
fields.
2. Avoid Sort Operations
Covered queries cannot use indexes for sorting operations. To leverage covered queries for improved performance, it’s important to avoid sorting operations whenever possible.
If you need to retrieve the data in a specific order, consider creating an appropriate index that matches the desired sort order.
Monitoring and Analyzing MongoDB Query Performance
Monitoring and analyzing MongoDB query performance is crucial for identifying bottlenecks and optimizing your queries. In this section, we will explore techniques to monitor and analyze MongoDB query performance.
Related Article: Declaring Variables in MongoDB Queries
1. Use the MongoDB Profiler
The MongoDB Profiler is a built-in tool that logs detailed information about the performance of MongoDB operations. By enabling the profiler, you can analyze the query execution time, the number of documents examined, and other important metrics.
To enable the profiler, you can use the following command in the MongoDB shell:
db.setProfilingLevel(2);
The profiler logs can be accessed using the system.profile
collection.
2. Utilize Database Profiling Tools
In addition to the built-in MongoDB Profiler, there are various third-party tools available for monitoring and analyzing MongoDB query performance. These tools provide more advanced features and visualizations to help you identify performance issues.
Some popular database profiling tools for MongoDB include MongoDB Compass, Datadog, and New Relic.