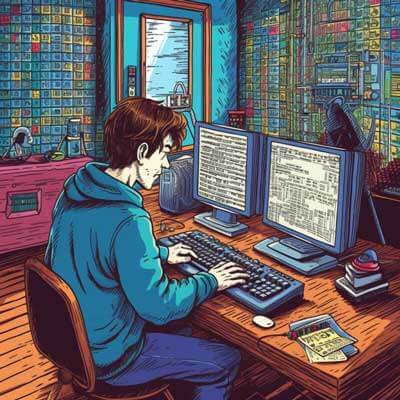
Table of Contents
In MongoDB, adding a field with a blank value in a query involves updating a document to include a new field with an empty value. This can be useful when you want to add a field to an existing document without assigning it a specific value right away. MongoDB allows you to easily add new fields to a document by using the $set
operator along with an empty value.
Here's an example of how to add a field with a blank value in a MongoDB query:
db.collection.updateOne( { _id: ObjectId("5f5e9e5c9dfb9c7d3e4e44d2") }, { $set: { newField: "" } } )
In the above example, we are using the updateOne
method to update a document in the collection
with the specified _id
. The $set
operator is used to add the newField
to the document and assign it an empty value.
It's important to note that when adding a field with a blank value, MongoDB treats the field as if it does not exist. This means that any queries or operations performed on the field will not include documents where the field has an empty value. If you want to include documents with a blank value in your queries, you will need to specifically check for the existence of the field in your query conditions.
Syntax for Adding a Field with a Blank Value in MongoDB
To add a field with a blank value in a MongoDB query, you can use the $set
operator along with an empty value. The syntax for adding a field with a blank value in MongoDB is as follows:
db.collection.updateOne( { filter }, { $set: { field: "" } } )
In the above syntax, db.collection.updateOne
is the method used to update a document in the specified collection. The filter
is used to identify the document to update, and the $set
operator is used to add the field
with an empty value.
Related Article: Declaring Variables in MongoDB Queries
Recommended Way to Set a Field to Null in MongoDB
In MongoDB, setting a field to null is a common approach when you want to indicate the absence of a value. However, it's important to note that MongoDB treats null values differently than empty values. When a field is set to null, MongoDB considers it as a valid value and includes it in queries and operations.
To set a field to null in MongoDB, you can use the $set
operator along with the value null. Here's an example:
db.collection.updateOne( { _id: ObjectId("5f5e9e5c9dfb9c7d3e4e44d2") }, { $set: { field: null } } )
In the above example, we are using the updateOne
method to update a document in the collection
with the specified _id
. The $set
operator is used to set the value of the field
to null.
It's important to note that when setting a field to null in MongoDB, the field will be considered as existing in the document. This means that queries or operations that check for the existence of the field will include documents where the field is set to null.
Adding an Empty Field to a MongoDB Document
To add an empty field to a MongoDB document, you can use the $set
operator along with an empty value. This allows you to add a new field to an existing document without assigning it a specific value right away.
Here's an example of how to add an empty field to a MongoDB document:
db.collection.updateOne( { _id: ObjectId("5f5e9e5c9dfb9c7d3e4e44d2") }, { $set: { newField: "" } } )
In the above example, we are using the updateOne
method to update a document in the collection
with the specified _id
. The $set
operator is used to add the newField
to the document and assign it an empty value.
It's important to note that when adding an empty field, MongoDB treats the field as if it does not exist. This means that any queries or operations performed on the field will not include documents where the field has an empty value. If you want to include documents with an empty field in your queries, you will need to specifically check for the existence of the field.
Considerations when Updating a Field to an Empty Value in MongoDB
When updating a field to an empty value in MongoDB, there are several considerations to keep in mind:
1. Field Existence: MongoDB treats fields with empty values as if they do not exist. This means that any queries or operations performed on the field will not include documents where the field has an empty value. If you want to include documents with an empty value, you will need to specifically check for the existence of the field and its value in your queries.
2. Null vs Empty Value: MongoDB treats null values and empty values differently. Null values are considered as valid values and are included in queries and operations. Empty values, on the other hand, are treated as if the field does not exist. Consider whether null or an empty value is more appropriate for your use case.
3. Data Consistency: When updating a field to an empty value, consider the impact on data consistency. If the field is used in other parts of your application or system, ensure that the empty value does not cause any inconsistencies or unexpected behavior.
4. Performance: Updating fields to empty values can have an impact on performance, especially if you have a large number of documents or frequently update the same field. Consider the performance implications and optimize your queries and updates accordingly.
Related Article: How to Query MongoDB by Time
Additional Resources
- What is MongoDB?