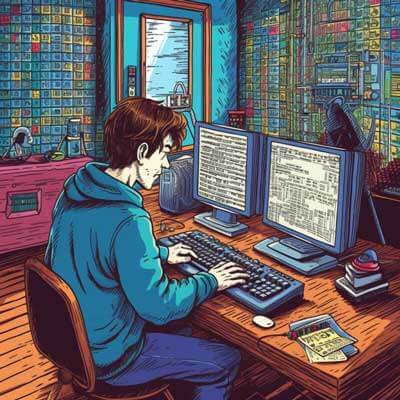
Table of Contents
How do callback functions work in MongoDB?
Callback functions play a crucial role in MongoDB search queries. When executing a search query, MongoDB allows developers to specify a callback function that will be invoked when the query is completed. This callback function allows developers to handle the results of the query and perform additional logic or operations based on those results.
In MongoDB, the callback function is typically provided as the last argument to the query method. Once the query is completed, the callback function is called with two arguments: an error object (if an error occurred) and the result of the query.
Here's an example of a MongoDB search query with a callback function:
db.collection('users').find({ age: { $gte: 18 } }, (error, result) => { if (error) { console.error('Error executing search query:', error); return; } console.log('Search query completed successfully. Result:', result);});
In this example, we are searching for all users with an age greater than or equal to 18. The callback function is responsible for handling any errors that may occur during the query execution and processing the result.
Related Article: How to Query MongoDB by Time
What is the purpose of chaining callbacks in a MongoDB search query?
Chaining callbacks in a MongoDB search query allows developers to perform multiple operations sequentially, one after another. This is particularly useful when the result of one operation is needed as input for the next operation.
For example, imagine a scenario where you need to find all users who have made a purchase in the last month and then send them a notification. You can achieve this by chaining multiple callbacks together.
Here's an example of chaining callbacks in a MongoDB search query:
db.collection('users').find({ lastPurchase: { $gte: lastMonth } }, (error, result) => { if (error) { console.error('Error executing search query:', error); return; } const userIds = result.map(user => user._id); db.collection('notifications').insertMany(userIds.map(userId => ({ userId, message: 'You have a new notification!', createdAt: new Date() })), (error, result) => { if (error) { console.error('Error executing insertMany query:', error); return; } console.log('InsertMany query completed successfully. Result:', result); });});
In this example, we first search for all users who made a purchase in the last month. Once we have the result, we extract the user IDs and use them to create notification documents. Finally, we insert the notification documents into the notifications
collection.
What are some alternatives to callback chaining in MongoDB?
While callback chaining is a common approach for executing multiple operations sequentially in MongoDB, there are also alternative approaches available.
One alternative is to use a library like async
or bluebird
, which provides utility functions for executing asynchronous operations in a more structured and readable way. These libraries offer functions like waterfall
, series
, and parallel
that allow you to define a sequence of operations and handle errors more easily.
Here's an example of using the async
library to chain operations in a MongoDB search query:
const async = require('async');async.waterfall([ function(callback) { db.collection('users').find({ age: { $gte: 18 } }, callback); }, function(result, callback) { const userIds = result.map(user => user._id); db.collection('notifications').insertMany(userIds.map(userId => ({ userId, message: 'You have a new notification!', createdAt: new Date() })), callback); }], function(error, result) { if (error) { console.error('Error executing search query:', error); return; } console.log('Search query and insertMany query completed successfully. Result:', result);});
In this example, we use the async.waterfall
function to define a sequence of operations. Each operation is defined as a separate function, and the result of one operation is passed as input to the next operation. The final callback function is called with the result of the last operation.
Another alternative is to use promises, which provide a more modern and concise syntax for handling asynchronous operations. MongoDB's official Node.js driver supports promises, allowing you to use promise-based APIs instead of callbacks.
How can I handle errors when chaining callbacks in MongoDB?
Handling errors properly is essential when chaining callbacks in MongoDB search queries. Errors can occur at any step of the chain, from the initial query execution to subsequent operations.
To handle errors, you can use the error parameter provided by the callback function. If an error occurs, the error parameter will be populated with an error object. You can then check if an error occurred and handle it accordingly.
Here's an example of error handling when chaining callbacks in a MongoDB search query:
db.collection('users').find({ age: { $gte: 18 } }, (error, result) => { if (error) { console.error('Error executing search query:', error); return; } const userIds = result.map(user => user._id); db.collection('notifications').insertMany(userIds.map(userId => ({ userId, message: 'You have a new notification!', createdAt: new Date() })), (error, result) => { if (error) { console.error('Error executing insertMany query:', error); return; } console.log('InsertMany query completed successfully. Result:', result); });});
In this example, we check for errors after each operation and log an error message if an error occurred. By logging the error and returning early, we ensure that subsequent operations are not executed if an error occurred.
Additionally, you can also use try-catch blocks to handle errors within each operation. This allows you to catch and handle specific errors within the callback chain.
Related Article: How to Use Range Queries in MongoDB
Why is callback hell a common issue with MongoDB search queries?
Callback hell is a common issue with MongoDB search queries due to the asynchronous nature of JavaScript and the need to chain multiple callbacks together.
When executing multiple operations sequentially, each operation typically requires the result of the previous operation. This leads to nested callback functions, also known as callback hell, where each callback is nested within another callback.
Here's an example of callback hell in a MongoDB search query:
db.collection('users').find({ age: { $gte: 18 } }, (error, result) => { if (error) { console.error('Error executing search query:', error); return; } const userIds = result.map(user => user._id); db.collection('notifications').insertMany(userIds.map(userId => ({ userId, message: 'You have a new notification!', createdAt: new Date() })), (error, result) => { if (error) { console.error('Error executing insertMany query:', error); return; } console.log('InsertMany query completed successfully. Result:', result); db.collection('logs').insertOne({ message: 'Notification sent' }, (error, result) => { if (error) { console.error('Error executing insertOne query:', error); return; } console.log('InsertOne query completed successfully. Result:', result); }); });});
In this example, each operation is nested within the callback of the previous operation, resulting in deeply nested code. This can make the code difficult to read, understand, and maintain.
Callback hell can also make error handling more complex. With each nested callback, error handling becomes more convoluted, leading to potential bugs and code that is hard to reason about.
What is the recommended approach for chaining callbacks in MongoDB?
To alleviate the issues caused by callback hell, there are several approaches you can take to chain callbacks in MongoDB search queries in a more readable and maintainable way.
One recommended approach is to use named functions instead of anonymous functions for your callbacks. By defining separate named functions for each callback, you can improve code readability and make it easier to reason about the flow of the code.
Here's an example of using named functions for chaining callbacks in a MongoDB search query:
function handleSearchQuery(error, result) { if (error) { console.error('Error executing search query:', error); return; } const userIds = result.map(user => user._id); db.collection('notifications').insertMany(userIds.map(userId => ({ userId, message: 'You have a new notification!', createdAt: new Date() })), handleInsertManyQuery);}function handleInsertManyQuery(error, result) { if (error) { console.error('Error executing insertMany query:', error); return; } console.log('InsertMany query completed successfully. Result:', result); db.collection('logs').insertOne({ message: 'Notification sent' }, handleInsertOneQuery);}function handleInsertOneQuery(error, result) { if (error) { console.error('Error executing insertOne query:', error); return; } console.log('InsertOne query completed successfully. Result:', result);}db.collection('users').find({ age: { $gte: 18 } }, handleSearchQuery);
In this example, each callback function is defined as a separate named function. This allows for a more linear and readable code structure. Additionally, error handling is simplified as each callback function only needs to handle its specific operation.
Another recommended approach is to use libraries or frameworks that provide a more structured and readable way to handle asynchronous operations, such as async
or bluebird
. These libraries offer functions like waterfall
, series
, and parallel
that allow you to define a sequence of operations in a more declarative manner.
Can I use promises instead of callbacks in MongoDB search queries?
Yes, you can use promises instead of callbacks in MongoDB search queries. The official Node.js MongoDB driver supports promises out of the box, allowing you to use promise-based APIs instead of callbacks.
To use promises with MongoDB, you can use the .then()
and .catch()
methods to handle the result or error of a query. Promises provide a more concise and readable syntax compared to traditional callback-based code.
Here's an example of using promises in a MongoDB search query:
db.collection('users').find({ age: { $gte: 18 } }) .then(result => { const userIds = result.map(user => user._id); return db.collection('notifications').insertMany(userIds.map(userId => ({ userId, message: 'You have a new notification!', createdAt: new Date() }))); }) .then(result => { console.log('InsertMany query completed successfully. Result:', result); return db.collection('logs').insertOne({ message: 'Notification sent' }); }) .then(result => { console.log('InsertOne query completed successfully. Result:', result); }) .catch(error => { console.error('Error executing search query or subsequent queries:', error); });
In this example, we chain the promises returned by the MongoDB queries using the .then()
method. Each .then()
block represents a subsequent operation that depends on the result of the previous operation. Errors are caught and handled using the .catch()
method.
Using promises with MongoDB search queries can lead to more readable and maintainable code, as the code flows in a linear fashion and error handling is centralized.
How does async/await syntax simplify callback chaining in MongoDB?
The async/await syntax is a modern addition to JavaScript that simplifies callback chaining in MongoDB search queries even further. It allows you to write asynchronous code that looks and behaves like synchronous code, making it easier to read, write, and reason about.
With async/await, you can write asynchronous code that appears to execute in a sequential and synchronous manner. It eliminates the need for explicit callbacks or promise chains, resulting in code that is more concise and easier to understand.
Here's an example of using async/await syntax to simplify callback chaining in a MongoDB search query:
async function executeSearchQuery() { try { const result = await db.collection('users').find({ age: { $gte: 18 } }).toArray(); const userIds = result.map(user => user._id); await db.collection('notifications').insertMany(userIds.map(userId => ({ userId, message: 'You have a new notification!', createdAt: new Date() }))); console.log('InsertMany query completed successfully.'); await db.collection('logs').insertOne({ message: 'Notification sent' }); console.log('InsertOne query completed successfully.'); } catch (error) { console.error('Error executing search query or subsequent queries:', error); }}executeSearchQuery();
In this example, the executeSearchQuery
function is defined as an async function. Within the function, we can use the await
keyword to pause the execution of the function until a promise is resolved or rejected. This allows us to write code that looks synchronous while still handling asynchronous operations.
The try-catch block is used to catch and handle any errors that may occur during the execution of the asynchronous code.
Using async/await syntax simplifies callback chaining in MongoDB search queries by providing a more intuitive and readable way to write asynchronous code.
Related Article: Crafting Query Operators in MongoDB
What are the advantages of using async/await over callback chaining in MongoDB?
Using async/await syntax in MongoDB search queries provides several advantages over traditional callback chaining:
1. Readability: Async/await code is more readable and easier to understand compared to deeply nested callback chains. The code flows in a linear and synchronous manner, making it easier to reason about.
2. Error handling: Async/await simplifies error handling by allowing you to use try-catch blocks to catch and handle errors. This eliminates the need for multiple error callbacks or promise chains, resulting in cleaner and more centralized error handling.
3. Synchronous-like syntax: Async/await allows you to write asynchronous code that resembles synchronous code. This makes it easier to write and maintain, especially for developers who are more familiar with synchronous programming.
4. Simplified control flow: Async/await simplifies control flow by eliminating the need for explicit callbacks or promise chains. The code can be written in a more sequential and natural way, improving code clarity and maintainability.
5. Integration with existing code: Async/await can be easily integrated with existing callback-based code by wrapping the callback-based functions with promise-based equivalents using the util.promisify
function.
Overall, async/await provides a more modern and concise syntax for handling asynchronous operations in MongoDB search queries, leading to code that is easier to read, write, and maintain.
Is there a specific order in which callbacks are executed in a MongoDB search query?
In general, the order in which callbacks are executed in a MongoDB search query is determined by the order of the operations and the completion of each operation.
When executing a MongoDB search query with a callback function, the callback is invoked once the query is completed. However, the order in which multiple callbacks are executed depends on the order in which the underlying operations are completed.
For example, consider a MongoDB search query that includes a find
operation and an insertMany
operation. The order in which the callbacks for these operations are executed depends on the completion order of the corresponding operations.
Here's an example that demonstrates the order of callback execution in a MongoDB search query:
db.collection('users').find({ age: { $gte: 18 } }, (error, result) => { if (error) { console.error('Error executing search query:', error); return; } console.log('Search query completed successfully. Result:', result); db.collection('notifications').insertMany(userIds.map(userId => ({ userId, message: 'You have a new notification!', createdAt: new Date() })), (error, result) => { if (error) { console.error('Error executing insertMany query:', error); return; } console.log('InsertMany query completed successfully. Result:', result); });});
In this example, the callback for the find
operation is executed first, followed by the callback for the insertMany
operation. This is because the find
operation is typically faster than the insertMany
operation, and the callback is invoked once the operation is completed.
It's important to note that the order of callback execution is not guaranteed if the operations are dependent on external factors or if they are executed in parallel. If the order of execution is crucial, you should ensure that the operations are performed in a deterministic and controlled manner.
Additional Resources
- Handling MongoDB Query Results with Promises