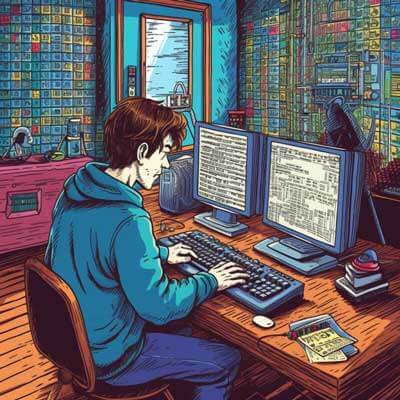
Table of Contents
Syntax for querying MongoDB by time
MongoDB provides a flexible and useful querying mechanism that allows you to query documents based on various criteria, including time. When querying MongoDB by time, you can use the $lt
(less than), $gt
(greater than), $lte
(less than or equal to), and $gte
(greater than or equal to) operators to specify the time range you are interested in.
The basic syntax for querying MongoDB by time is as follows:
db.collection.find({ field: { $operator: value } })
- db.collection
refers to the name of the collection you want to query.
- field
is the name of the field in the documents that holds the time value.
- $operator
is one of the time comparison operators ($lt
, $gt
, $lte
, $gte
).
- value
is the time value you want to compare against.
Here's an example that demonstrates the syntax for querying MongoDB by time:
db.orders.find({ created_at: { $gte: ISODate("2022-01-01T00:00:00Z") } })
This query will retrieve all documents from the orders
collection where the created_at
field is greater than or equal to January 1, 2022.
Related Article: Tutorial: MongoDB Aggregate Query Analysis
Querying MongoDB for documents within a specific time range
To query MongoDB for documents within a specific time range, you can combine the $gte
(greater than or equal to) and $lte
(less than or equal to) operators. This allows you to specify both the start and end time of the range you want to query.
Here's an example that demonstrates how to query MongoDB for documents within a specific time range:
db.orders.find({ created_at: { $gte: ISODate("2022-01-01T00:00:00Z"), $lte: ISODate("2022-01-31T23:59:59Z") } })
This query will retrieve all documents from the orders
collection where the created_at
field is greater than or equal to January 1, 2022, and less than or equal to January 31, 2022.
Examples of time-based queries in MongoDB
Let's explore some examples of time-based queries in MongoDB to further illustrate the concepts discussed.
Example 1: Querying MongoDB for documents created after a specific date
Suppose we have a collection named logs
that contains log entries with a timestamp
field indicating the creation time. We want to retrieve all log entries created after January 1, 2022.
db.logs.find({ timestamp: { $gt: ISODate("2022-01-01T00:00:00Z") } })
This query will return all documents from the logs
collection where the timestamp
field is greater than January 1, 2022.
Example 2: Querying MongoDB by timestamp
In some cases, you may have a timestamp field in your documents instead of a date field. To query MongoDB by timestamp, you can use the same comparison operators as before, but this time applied to the timestamp field.
Suppose we have a collection named events
that contains event documents with a timestamp
field indicating the event's occurrence time. We want to retrieve all events that occurred after a specific timestamp.
db.events.find({ timestamp: { $gt: 1640995200000 } })
This query will return all documents from the events
collection where the timestamp
field is greater than 1640995200000 (which represents January 1, 2022, in milliseconds since the Unix epoch).
Filtering MongoDB documents by time
In addition to querying MongoDB for documents within a specific time range, you can also filter documents based on time-related conditions. This allows you to narrow down your results further and retrieve only the documents that meet certain criteria.
To filter MongoDB documents by time, you can use the $expr
operator combined with the $lt
(less than) or $gt
(greater than) operators. The $expr
operator allows you to use aggregation expressions within your query.
Here's an example that demonstrates how to filter MongoDB documents by time:
db.orders.find({ $expr: { $lt: ["$created_at", ISODate("2022-01-01T00:00:00Z")] } })
This query will retrieve all documents from the orders
collection where the created_at
field is less than January 1, 2022.
Related Article: Exploring MongoDB: Does it Load Documents When Querying?
Using MongoDB's datetime functions for querying time periods
MongoDB provides a set of datetime functions that you can use to query for specific time periods. These functions allow you to manipulate and compare date and time values in your queries, making it easier to work with time-based data.
Here are some of the datetime functions available in MongoDB:
- $year
: Extracts the year from a date.
- $month
: Extracts the month from a date.
- $dayOfMonth
: Extracts the day of the month from a date.
- $hour
: Extracts the hour from a date.
- $minute
: Extracts the minute from a date.
- $second
: Extracts the second from a date.
You can use these functions in combination with the comparison operators to query MongoDB for documents within specific time periods.
Example: Querying MongoDB for documents created between two dates
Suppose we have a collection named logs
that contains log entries with a timestamp
field indicating the creation time. We want to retrieve all log entries created between January 1, 2022, and January 31, 2022.
db.logs.find({ $and: [ { timestamp: { $gte: ISODate("2022-01-01T00:00:00Z") } }, { timestamp: { $lte: ISODate("2022-01-31T23:59:59Z") } } ] })
This query uses the $and
operator to combine two conditions: one for the start date and one for the end date. It will return all documents from the logs
collection where the timestamp
field is greater than or equal to January 1, 2022, and less than or equal to January 31, 2022.
Best practices for querying MongoDB for documents within a specific date range
When querying MongoDB for documents within a specific date range, it's important to consider some best practices to ensure efficient and effective querying.
1. Index the date field: Indexing the date field you are querying on can significantly improve query performance. Create an index on the date field to allow MongoDB to quickly locate and retrieve the relevant documents.
db.collection.createIndex({ dateField: 1 })
2. Use the appropriate comparison operator: Choose the appropriate comparison operator ($lt
, $gt
, $lte
, $gte
) based on the specific time range you want to query. This helps MongoDB optimize the query execution and retrieve the desired results efficiently.
3. Leverage query optimization techniques: MongoDB provides various query optimization techniques, such as query plan caching and index intersection, to improve query performance. Familiarize yourself with these techniques and apply them when necessary.
4. Avoid unnecessary data retrieval: Only retrieve the fields you need in the query results. Limit the fields returned by using the projection parameter ({ field: 1 }
) to avoid unnecessary data transfer and improve query performance.
5. Consider data sharding: If your MongoDB deployment is distributed across multiple shards, consider how the time-based queries will distribute the load across the shards. Design your sharding strategy accordingly to achieve optimal performance.
Limitations and performance considerations when querying MongoDB by time
When querying MongoDB by time, there are some limitations and performance considerations to keep in mind.
1. Index size: Indexing the date field can increase the size of the index, especially if you have a large number of documents. This can impact the overall storage requirements and query performance.
2. Index selection: Choosing the right index is crucial for efficient time-based queries. Consider the specific query patterns and the data distribution when selecting the index type (e.g., ascending vs. descending) to ensure optimal performance.
3. Shard key selection: If you are using sharding in your MongoDB deployment, selecting an appropriate shard key is important. The shard key should distribute the data evenly across the shards to avoid hotspots and ensure efficient query routing.
4. Query complexity: Complex time-based queries involving multiple conditions or aggregation operations can impact query performance. Keep the queries as simple as possible and leverage MongoDB's aggregation framework when necessary to optimize performance.
5. Data distribution: If your data is skewed towards specific time ranges, it can impact query performance. Consider data distribution and partitioning strategies to evenly distribute the data across shards and ensure balanced query execution.
Querying MongoDB with time filters
Time filters allow you to query MongoDB for documents based on specific time-related conditions. You can use various operators and functions to define the time filters and retrieve the desired results.
Here's an example that demonstrates how to query MongoDB with time filters:
db.orders.find({ created_at: { $gte: ISODate("2022-01-01T00:00:00Z"), $lte: ISODate("2022-01-31T23:59:59Z") }, status: "completed" })
This query retrieves all documents from the orders
collection where the created_at
field is within the specified time range (January 1, 2022, to January 31, 2022) and the status
field is set to "completed".