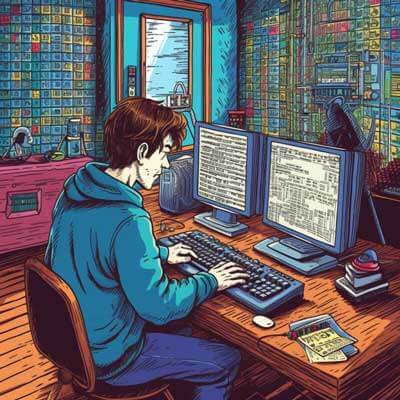
Table of Contents
Query Operators in MongoDB
MongoDB is a popular NoSQL database that allows developers to store and retrieve data in a flexible and scalable manner. One of the key features of MongoDB is its useful query operators, which enable developers to filter, manipulate, and sort data in a variety of ways.
Query operators in MongoDB are used to perform operations on documents stored in a collection. These operators allow developers to specify conditions for filtering data, perform complex aggregations, and update documents in a precise manner. By utilizing query operators, developers can retrieve the exact data they need from the database, saving time and effort.
MongoDB provides a wide range of query operators that cater to different use cases. Some of the commonly used query operators include:
- Comparison Operators: These operators are used to compare values and find documents that match specific conditions. Examples of comparison operators include $eq (equals), $ne (not equals), $gt (greater than), $lt (less than), $gte (greater than or equals), and $lte (less than or equals).
- Logical Operators: Logical operators allow developers to combine multiple conditions and perform logical operations on them. The commonly used logical operators in MongoDB are $and, $or, and $not.
- Element Operators: Element operators are used to query documents based on the presence or absence of fields. The $exists operator is commonly used to check if a field exists or not.
- Array Operators: MongoDB provides a set of operators to query arrays and perform operations on array elements. Some of the array operators include $in, $all, $elemMatch, and $size.
- Regular Expression Operators: Regular expression operators allow developers to perform pattern matching on string fields. The $regex operator is commonly used for regular expression matching.
Related Article: MongoDB Queries Tutorial
Using Query Operators to Filter Data
One of the primary use cases of query operators in MongoDB is to filter data based on specific conditions. By using query operators, developers can construct queries that retrieve only the documents that satisfy certain criteria.
Let's consider an example where we have a collection called "users" that stores information about users in a web application. Each document in the "users" collection has fields like "name", "age", and "email". We can use query operators to filter the users based on their age or email.
Here's an example query that uses the $gt operator to retrieve users who are older than 30:
db.users.find({ age: { $gt: 30 } })
This query will return all the users whose age is greater than 30. The $gt operator compares the value of the "age" field with the specified value (30 in this case) and retrieves the matching documents.
Similarly, we can use other query operators like $eq, $ne, $lt, $gte, and $lte to filter data based on different conditions. For example, to retrieve users with a specific email, we can use the $eq operator:
db.users.find({ email: { $eq: "example@example.com" } })
This query will return all the users whose email is "example@example.com".
Understanding MongoDB Query Syntax
MongoDB query syntax follows a JSON-like structure, where queries are expressed as JSON objects. The basic syntax for a MongoDB query is as follows:
db.collection.find(query, projection)
- The "db.collection.find()" method is used to perform a query on a specific collection. The "query" parameter specifies the conditions for filtering the documents, and the "projection" parameter specifies the fields to be included or excluded from the result.
- The "query" parameter is a JSON object that contains the conditions for filtering the documents. It can include query operators to specify complex conditions.
- The "projection" parameter is also a JSON object that specifies the fields to be included or excluded from the result. By default, all fields are returned if no projection is specified.
Here's an example that demonstrates the MongoDB query syntax:
db.users.find({ age: { $gt: 30 } }, { name: 1, email: 1 })
This query will return the names and emails of users who are older than 30. The "name: 1" and "email: 1" in the projection parameter specify that only the "name" and "email" fields should be included in the result.
Querying Specific Fields in MongoDB
In MongoDB, it is possible to query specific fields of a document instead of retrieving the entire document. This can be useful when you only need a subset of the fields and want to optimize the query performance.
To query specific fields in MongoDB, you can use the projection parameter in the "db.collection.find()" method. The projection parameter is a JSON object that specifies the fields to be included or excluded from the result.
Here's an example that demonstrates how to query specific fields in MongoDB:
db.users.find({}, { name: 1, email: 1 })
This query will return the names and emails of all users in the collection. The first parameter of the "db.users.find()" method is an empty object, which means all documents in the collection will be retrieved. The second parameter specifies that only the "name" and "email" fields should be included in the result.
If you want to exclude specific fields from the result, you can use the projection parameter with the value 0. For example, to exclude the "age" field from the result, you can modify the query as follows:
db.users.find({}, { age: 0 })
This query will return all the fields except for the "age" field.
Related Article: Supabase vs MongoDB: A Feature-by-Feature Comparison
Exploring Different Types of Query Filters
MongoDB provides a range of query filters that can be used to perform various types of queries on documents. These filters allow developers to query documents based on different criteria, such as comparison, logical operations, element presence, and array operations.
Let's explore some of the different types of query filters in MongoDB:
- Comparison Filters: Comparison filters are used to compare values and find documents that match specific conditions. Some of the commonly used comparison filters include $eq, $ne, $gt, $lt, $gte, and $lte.
Example:
db.users.find({ age: { $gt: 30 } })
This query will retrieve all the users whose age is greater than 30.
- Logical Filters: Logical filters allow developers to combine multiple conditions and perform logical operations on them. The $and, $or, and $not operators are commonly used for logical filtering.
Example:
db.users.find({ $and: [{ age: { $gte: 30 } }, { age: { $lte: 40 } }] })
This query will retrieve all the users whose age is between 30 and 40.
- Element Filters: Element filters are used to query documents based on the presence or absence of fields. The $exists operator is commonly used for element filtering.
Example:
db.users.find({ email: { $exists: true } })
This query will retrieve all the users who have an email field.
- Array Filters: Array filters allow developers to perform operations on array elements and query documents based on array conditions. Some of the commonly used array filters include $in, $all, $elemMatch, and $size.
Example:
db.users.find({ hobbies: { $in: ["reading", "writing"] } })
This query will retrieve all the users who have hobbies that include either "reading" or "writing".
Combining Multiple Query Operators in MongoDB
In MongoDB, it is possible to combine multiple query operators to create complex and precise queries. By using logical operators like $and and $or, developers can construct queries that satisfy multiple conditions.
Let's consider an example where we have a collection called "products" that stores information about products in an e-commerce application. Each document in the "products" collection has fields like "name", "category", and "price". We can use multiple query operators to retrieve products that match specific criteria.
Here's an example query that combines the $and and $or operators to retrieve products that are either in the "Electronics" category or have a price greater than 1000:
db.products.find({ $or: [{ category: "Electronics" }, { price: { $gt: 1000 } }] })
This query will return all the products that are either in the "Electronics" category or have a price greater than 1000.
Sorting Query Results in MongoDB
In MongoDB, it is possible to sort query results based on one or more fields. Sorting query results can be useful when you want to retrieve data in a specific order, such as ascending or descending order.
To sort query results in MongoDB, you can use the "sort()" method in combination with the "db.collection.find()" method. The "sort()" method takes a JSON object as a parameter, where each field specifies the sorting order for the corresponding field.
Here's an example that demonstrates how to sort query results in MongoDB:
db.products.find().sort({ price: 1 })
This query will retrieve all the products from the "products" collection and sort them in ascending order based on the "price" field.
If you want to sort query results in descending order, you can use -1 as the value for the field in the sort parameter. For example:
db.products.find().sort({ price: -1 })
This query will retrieve all the products from the "products" collection and sort them in descending order based on the "price" field.
The Purpose of Query Projection in MongoDB
Query projection in MongoDB is used to specify which fields should be included or excluded from the result of a query. By using query projection, developers can optimize query performance by retrieving only the necessary fields instead of retrieving the entire document.
In MongoDB, the projection parameter in the "db.collection.find()" method is used to specify the fields to be included or excluded from the result. The projection parameter is a JSON object where each field is specified with a value of 1 or 0.
If a field is specified with a value of 1, it will be included in the result. If a field is specified with a value of 0, it will be excluded from the result.
Here's an example that demonstrates the purpose of query projection in MongoDB:
db.users.find({}, { name: 1, email: 1 })
This query will retrieve all the users from the "users" collection and include only the "name" and "email" fields in the result.
Related Article: Exploring MongoDB: Does it Load Documents When Querying?
Case Sensitivity of Query Operators in MongoDB
Query operators in MongoDB are case-sensitive by default. This means that when performing queries, MongoDB differentiates between uppercase and lowercase letters.
For example, consider a collection called "users" that stores information about users in a web application. Each document in the "users" collection has a "name" field. If we want to retrieve users with a specific name, we need to provide the exact case-sensitive value in the query.
Here's an example query that demonstrates the case sensitivity of query operators in MongoDB:
db.users.find({ name: "John" })
This query will retrieve all the users whose name is "John" exactly. If there are users with names like "john" or "JOHN", they will not be included in the result.
To perform case-insensitive queries in MongoDB, we can use the $regex operator with the "i" option. The "i" option makes the regular expression case-insensitive.
Here's an example query that performs a case-insensitive query using the $regex operator:
db.users.find({ name: { $regex: /john/i } })
This query will retrieve all the users whose name contains "john" in any case, such as "John", "john", "JOHN", etc.
Using Regular Expressions in MongoDB Query Operators
MongoDB query operators support regular expressions, which allow developers to perform pattern matching on string fields. Regular expressions provide a useful way to search for data based on complex patterns.
In MongoDB, the $regex operator is used to perform regular expression matching in query operators. The $regex operator takes a regular expression as a parameter and matches the string fields against that regular expression.
Here's an example that demonstrates how to use regular expressions in MongoDB query operators:
db.users.find({ name: { $regex: /john/i } })
This query will retrieve all the users whose name contains "john" in any case, such as "John", "john", "JOHN", etc. The "/john/i" is the regular expression that matches any string containing "john" in any case.
Regular expressions can be used with other query operators as well. For example, we can use the $regex operator in combination with the $ne operator to retrieve users whose name does not contain "john":
db.users.find({ name: { $regex: /john/i, $ne: "John Doe" } })
This query will retrieve all the users whose name contains "john" but is not equal to "John Doe".
Additional Resources
- MongoDB Query Operators