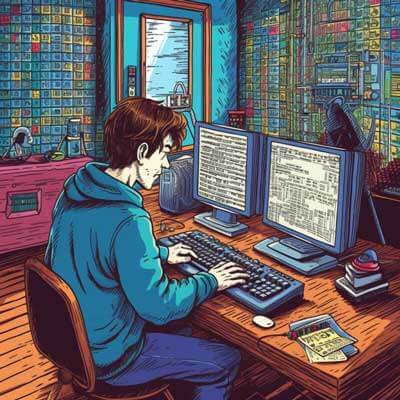
Table of Contents
In MongoDB, variables can be used in queries to store and manipulate values. Variables allow for dynamic and flexible queries by allowing you to reuse values and perform calculations within the query itself. This can be particularly useful when working with complex data structures or when you need to perform operations on multiple documents at once.
To use variables in MongoDB queries, you need to declare them using the appropriate syntax and then reference them within your query. The syntax for declaring variables in MongoDB queries is straightforward and follows a specific format.
Syntax for Declaring Variables in MongoDB Queries
To declare a variable in a query, you use the $let
operator. The $let
operator takes an array of variables, where each variable is defined using the vars
keyword followed by a document with the variable name as the key and the value as the corresponding expression.
The syntax for declaring variables in MongoDB queries using the $let
operator is as follows:
{ $let: { vars: { variable1: expression1, variable2: expression2, ... }, in: expression }}
- vars
: This is an object that contains the variables and their corresponding expressions. Each variable is defined using the variableName: expression
format.
- in
: This is the expression that uses the declared variables. It can reference the variables defined in the vars
object.
Related Article: How to Query MongoDB by Time
Declaring and Using Variables in MongoDB Queries
Let's say we have a collection called users
that contains documents with the following structure:
{ "_id" : ObjectId("60a4371b7b23c42d9c4c2a2a"), "name" : "John Doe", "age" : 30, "salary" : 50000 }
We want to find all users whose salary is above a certain threshold. To achieve this, we can declare a variable to store the threshold value and then use it in the query.
Here's an example of how to declare and use a variable in a MongoDB query:
db.users.aggregate([ { $let: { vars: { threshold: 40000 }, in: { $match: { salary: { $gt: "$$threshold" } } } } } ])
In this example, we use the $let
operator to declare a variable called threshold
with a value of 40000. We then use this variable in the $match
stage of the aggregation pipeline to filter the documents based on the salary
field.
Assigning Values to Variables in MongoDB Queries
Variables in MongoDB queries can be assigned values using various expressions, including constants, fields from the document being processed, and the results of other expressions.
Let's consider the following example where we want to find all users whose age is more than twice their salary:
db.users.aggregate([ { $let: { vars: { age: "$age", salary: "$salary" }, in: { $match: { $expr: { $gt: [ "$$age", { $multiply: [ 2, "$$salary" ] } ] } } } } } ])
In this example, we declare two variables age
and salary
and assign them the values of the age
and salary
fields from the document being processed, respectively. We then use these variables in the $match
stage of the aggregation pipeline to filter the documents based on the condition that the age
is greater than twice the salary
.
Advantages of Using Variables in MongoDB Queries
Using variables in MongoDB queries offers several advantages:
1. Reusability: Variables allow you to store values that can be reused within the query, reducing repetition and making the code more concise and maintainable.
2. Flexibility: Variables enable you to perform calculations and manipulations within the query itself, providing more flexibility and control over the data processing.
3. Readability: By using variables, you can give meaningful names to values, making the query more readable and easier to understand.
4. Dynamic queries: Variables allow you to create dynamic queries that adapt to different scenarios or input parameters. This can be particularly useful when building APIs or user interfaces where the query parameters can vary.
Related Article: Exploring MongoDB: Does it Load Documents When Querying?
Limitations of Declaring Variables in MongoDB Queries
While variables in MongoDB queries offer flexibility and convenience, there are some limitations to be aware of:
1. Scope: Variables declared within a query are only accessible within that query. They cannot be used outside of the query or in subsequent stages of the aggregation pipeline.
2. Expressions: The expressions used to assign values to variables are subject to the same limitations and restrictions as any other MongoDB expressions. For example, some operations may not be supported or may have specific syntax requirements.
3. Performance: The use of variables in queries can impact performance, especially if the variables are used in complex or computationally intensive operations. It is important to consider the performance implications when using variables in your queries.
Passing Variables into MongoDB Queries
In addition to declaring variables directly within a query, MongoDB also provides the ability to pass variables from an external source, such as a programming language or application, into the query.
To pass variables into a MongoDB query, you can use variable substitution techniques provided by the MongoDB driver or client library that you are using. The specific method for passing variables may vary depending on the programming language or framework you are using.
Here's an example in JavaScript using the MongoDB Node.js driver:
const threshold = 40000; db.users.aggregate([ { $match: { salary: { $gt: threshold } } } ])
In this example, we declare a variable threshold
and assign it a value of 40000. We then use this variable in the $match
stage of the aggregation pipeline to filter the documents based on the salary
field.
Case Sensitivity of Variables in MongoDB Queries
Variables in MongoDB queries are case-sensitive. This means that the variable names must match exactly, including the case, when referencing them in the query.
For example, if you declare a variable called threshold
, you must reference it as $$threshold
in the query. Using a different case, such as $$Threshold
or $$THRESHOLD
, will result in an error.
Declaring Multiple Variables in a Single MongoDB Query
You can declare multiple variables in a single MongoDB query by including them in the vars
object of the $let
operator.
Here's an example:
db.users.aggregate([ { $let: { vars: { threshold: 40000, discount: 0.1 }, in: { $project: { name: 1, discountedSalary: { $multiply: [ "$salary", { $subtract: [ 1, "$$discount" ] } ] } } } } } ])
In this example, we declare two variables threshold
and discount
with values of 40000 and 0.1, respectively. We then use these variables in the $project
stage of the aggregation pipeline to calculate the discountedSalary
by multiplying the salary
field with the difference between 1 and the discount
variable.
Related Article: Using Multi-Indexes with MongoDB Queries
Types of Variables in MongoDB Queries
Variables in MongoDB queries can hold values of different types, including numbers, strings, booleans, and arrays.
Here's an example that demonstrates using variables of different types:
db.users.aggregate([ { $let: { vars: { name: "John", age: 30, isActive: true, tags: ["mongodb", "query", "variables"] }, in: { $match: { $and: [ { name: "$$name" }, { age: { $gt: "$$age" } }, { isActive: "$$isActive" }, { tags: { $all: "$$tags" } } ] } } } } ])
In this example, we declare variables of different types, including a string variable name
, a number variable age
, a boolean variable isActive
, and an array variable tags
. We then use these variables in the $match
stage of the aggregation pipeline to filter the documents based on various conditions.