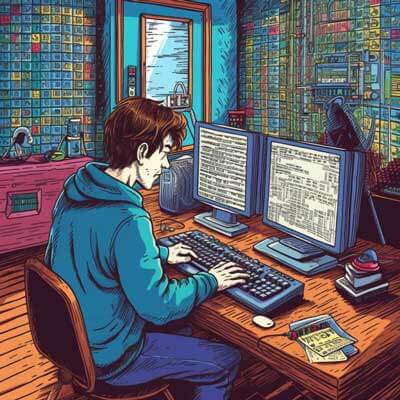
- Prerequisites
- Connecting Python with MongoDB Collections
- Advantages of Using Python with MongoDB
- Querying MongoDB Collections with Python
- Find All Documents
- Find Documents by Query Criteria
- Limiting Results
- Inserting Data into MongoDB Collections
- Inserting a Single Document
- Inserting Multiple Documents
- Updating Multiple Documents in a MongoDB Collection
Prerequisites
Before diving into using Python with MongoDB, make sure you have the following prerequisites:
1. Python installed on your machine. You can download the latest version of Python from the official website: https://www.python.org/downloads/.
2. The PyMongo library installed. You can install it by running the following command in your terminal or command prompt:
pip install pymongo
Now that we have all the necessary prerequisites in place let’s move on to connecting Python with MongoDB collections.
Related Article: Exploring MongoDB: Does it Load Documents When Querying?
Connecting Python with MongoDB Collections
To begin working with MongoDB collections in Python, we first need to establish a connection between our Python application and the MongoDB server.
The connection process involves specifying the server’s host address and port number. By default, a local instance of MongoDB runs on localhost:27017.
Let’s take a look at an example code snippet that demonstrates how to connect Python with MongoDB using PyMongo:
import pymongo # Establish connection client = pymongo.MongoClient("mongodb://localhost:27017/") # Access a specific database db = client["mydatabase"] # Access a specific collection within the database collection = db["mycollection"]
In the above code, we import the PyMongo library and establish a connection to the MongoDB server running on localhost. We then access a specific database called “mydatabase” and a collection named “mycollection” within that database.
It’s important to note that connecting to a remote MongoDB server requires specifying the appropriate host address and port number. Additionally, authentication credentials may be necessary for accessing secured databases.
Advantages of Using Python with MongoDB
Combining the power of Python with MongoDB offers several advantages for developers. Let’s explore some of these benefits:
1. Flexibility: MongoDB’s flexible schema allows developers to store and retrieve complex data structures without having to define an explicit schema upfront. This flexibility is particularly advantageous when dealing with evolving data models.
2. Pythonic Syntax: Python’s simple and expressive syntax makes it easy to work with MongoDB collections. The intuitive nature of Python allows developers to write clean and readable code when interacting with MongoDB.
3. Rich Ecosystem: With its vast collection of libraries and frameworks, Python provides extensive support for working with different data formats, including JSON, which aligns well with MongoDB’s document-based nature.
4. Data Analysis Capabilities: Python has become synonymous with data analysis and machine learning, thanks to libraries such as NumPy, Pandas, and scikit-learn. By combining these useful tools with MongoDB’s native aggregation framework, developers can perform advanced analytics on large datasets efficiently.
5. Scalability: Both Python and MongoDB are designed for scalability. As your application grows in size and complexity, you can easily scale your MongoDB cluster horizontally by adding more servers, while Python’s multiprocessing and distributed computing libraries enable efficient parallel processing.
These are just a few of the advantages that come with using Python alongside MongoDB. As we delve deeper into this tutorial, you will discover even more ways in which these technologies complement each other.
Querying MongoDB Collections with Python
Querying collections is one of the fundamental operations when working with a database. In the context of MongoDB, querying involves retrieving documents that match certain criteria from a collection.
In Python, we can use PyMongo’s query methods to perform various types of queries on MongoDB collections. Let’s explore some common query operations with code examples:
Related Article: How to Add a Field with a Blank Value in MongoDB
Find All Documents
To retrieve all documents from a collection, we can use the find()
method without any parameters. Here’s an example:
result = collection.find() for document in result: print(document)
In the above code snippet, we call the find()
method on our collection object without passing any arguments. This returns a cursor pointing to all documents in the collection. We then iterate over the cursor and print each document.
Find Documents by Query Criteria
To retrieve documents that match specific query criteria, we can pass a filter parameter to the find()
method. The filter parameter is a dictionary containing key-value pairs representing the search criteria. Here’s an example:
query = { "name": "John" } result = collection.find(query) for document in result: print(document)
In this example, we define a query dictionary with the key “name” and value “John”. The find()
method uses this query to retrieve all documents where the name field matches “John”.
Limiting Results
Sometimes it may be necessary to limit the number of results returned by a query. We can achieve this by using the limit()
method. Here’s an example:
result = collection.find().limit(5) for document in result: print(document)
In this code snippet, we use the limit()
method to restrict the number of documents returned to 5.
These are just a few examples of how to query MongoDB collections using Python and PyMongo. The PyMongo library provides many more methods and options for querying collections, allowing developers to perform complex searches efficiently.
Related Article: How to Use Range Queries in MongoDB
Inserting Data into MongoDB Collections
Inserting data into MongoDB collections is a common operation when working with databases. In Python, we can use PyMongo’s insert_one()
and insert_many()
methods to insert data into MongoDB collections.
Inserting a Single Document
To insert a single document into a collection, we can use the insert_one()
method. Here’s an example:
document = { "name": "John", "age": 25 } result = collection.insert_one(document) print(result.inserted_id)
In this code snippet, we define a document as a dictionary with key-value pairs representing the fields and values of the document. We then call the insert_one()
method on our collection object, passing in the document as an argument. The method returns an object that contains information about the insertion process, including the inserted document’s ID.
Inserting Multiple Documents
To insert multiple documents into a collection at once, we can use the insert_many()
method. Here’s an example:
documents = [ { "name": "John", "age": 25 }, { "name": "Jane", "age": 30 }, { "name": "Mike", "age": 35 } ] result = collection.insert_many(documents) print(result.inserted_ids)
In this code snippet, we define a list of documents, where each document is represented as a dictionary. We then call the insert_many()
method on our collection object, passing in the list of documents as an argument. The method returns an object that contains information about the insertion process, including the inserted documents’ IDs.
These are just basic examples of how to insert data into MongoDB collections using Python and PyMongo. The PyMongo library provides additional methods and options for handling more complex scenarios, such as bulk inserts and document validation.
Related Article: Crafting Query Operators in MongoDB
Updating Multiple Documents in a MongoDB Collection
Updating multiple documents within a MongoDB collection is a common task when working with databases. In Python, we can use PyMongo’s update_many()
method to update multiple documents that match certain criteria.
Let’s take a look at an example that demonstrates how to update documents in a MongoDB collection using Python:
query = { "name": "John" } new_values = { "$set": { "age": 30 } } result = collection.update_many(query, new_values) print(result.modified_count)
In this code snippet, we define a query dictionary with the key “name” and value “John”. We also define a new_values dictionary with the “$set” operator and the field-value pair we want to update. The update_many()
method then applies these changes to all documents where the name field matches “John”. The method returns an object that contains information about the update process, including the number of modified documents.
It’s important to note that MongoDB provides various operators for different types of updates. The “$set” operator used in this example sets or updates specific fields within the document. You can explore other operators such as “$inc”, “$push”, or “$addToSet” to perform different types of updates.
These are just basic examples of how to update multiple documents in a MongoDB collection using Python and PyMongo. The PyMongo library provides additional methods and options for handling more complex scenarios, such as updating specific fields within nested documents or performing conditional updates.