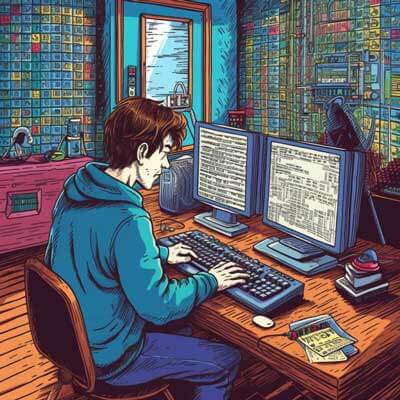
- Query Syntax
- Query Operators
- Query Performance
- Query Optimization
- Query Examples
- Query Projection
- Query Regular Expressions
- Query Sorting
- Query Limit
- Writing Complex Queries in MongoDB
- Common Query Operators in MongoDB
- Improving Performance of MongoDB Queries
- Query Projection in MongoDB
- Regular Expressions in MongoDB Queries
- Sorting Results of MongoDB Queries
- Limiting Results in MongoDB Queries
- Best Practices for Writing Efficient MongoDB Queries
- Real-World Examples of MongoDB Queries
- Limitations and Constraints in MongoDB Queries
MongoDB is a popular document-oriented NoSQL database that provides flexible and scalable data storage solutions. One of the key advantages of MongoDB is its ability to handle large volumes of data while still maintaining high performance. In this article, we will explore the modifiability of MongoDB queries and how they can be optimized for better performance.
Query Syntax
The syntax for querying data in MongoDB is flexible and intuitive. MongoDB uses a JSON-like syntax called the MongoDB Query Language (MQL) to define queries. MQL allows you to specify the criteria for selecting documents from a collection based on various conditions.
Here’s an example of a simple query in MongoDB:
db.collection.find({ field: value })
In this example, db.collection.find()
is the method used to query documents in a collection. The { field: value }
parameter specifies the condition for selecting documents. For instance, if you have a collection called users
and you want to find all documents where the age
field is equal to 25, you can use the following query:
db.users.find({ age: 25 })
Related Article: Tutorial: Using Python to Interact with MongoDB Collections
Query Operators
MongoDB provides a wide range of query operators that allow you to perform complex queries and retrieve specific documents from a collection. These operators can be used to define conditions for matching documents, perform logical operations, and more.
Here are some commonly used query operators in MongoDB:
– Comparison Operators: $eq
, $ne
, $gt
, $gte
, $lt
, $lte
, $in
, $nin
– Logical Operators: $and
, $or
, $not
, $nor
– Element Operators: $exists
, $type
– Array Operators: $all
, $elemMatch
, $size
– Evaluation Operators: $expr
, $jsonSchema
Let’s look at an example that uses the $in
operator to find documents where the status
field is either “active” or “inactive”:
db.users.find({ status: { $in: ["active", "inactive"] } })
Query Performance
Query performance is a critical aspect when working with large datasets in MongoDB. Efficient queries can significantly impact the overall performance of your application. MongoDB provides several features and techniques to optimize query performance.
One important consideration is the indexing of fields used in queries. Indexes help MongoDB locate and retrieve data more efficiently. By creating indexes on frequently queried fields, you can significantly improve query performance.
Here’s an example of creating an index on the name
field of a collection called users
:
db.users.createIndex({ name: 1 })
The 1
in the createIndex
method specifies an ascending index. You can also use -1
for a descending index. Once the index is created, MongoDB will use it to speed up queries that involve the name
field.
Query Optimization
In addition to indexing, MongoDB provides several techniques to optimize query performance. These include query profiling, query hints, and query plan analysis.
Query profiling allows you to analyze the execution time and resource usage of a query. By enabling profiling, you can identify slow queries and optimize them for better performance. Here’s an example of enabling query profiling:
db.setProfilingLevel(1)
Query hints allow you to provide hints to the query optimizer on how to execute a query. You can specify which index to use or force a specific execution plan. Here’s an example of using a query hint to enforce the use of an index:
db.users.find({ age: { $gt: 25 } }).hint({ age: 1 })
Query plan analysis allows you to examine and analyze the execution plan chosen by the query optimizer. You can use the explain()
method to retrieve the execution plan for a query and identify any potential bottlenecks or areas for optimization.
Related Article: Exploring MongoDB: Does it Load Documents When Querying?
Query Examples
Let’s explore some more examples of MongoDB queries to understand how they can be used in practice.
Example 1: Find all documents where the age
field is greater than 30:
db.users.find({ age: { $gt: 30 } })
Example 2: Find all documents where the name
field starts with the letter “J”:
db.users.find({ name: /^J/ })
Query Projection
Query projection allows you to specify which fields to include or exclude from the result set of a query. This can be useful when you only need specific fields and want to reduce the size of the returned documents.
Here’s an example of including only the name
and email
fields in the result set:
db.users.find({}, { name: 1, email: 1, _id: 0 })
In this example, the second parameter of the find()
method specifies the projection. The 1
indicates that the field should be included, while the 0
indicates exclusion.
Query Regular Expressions
MongoDB supports regular expressions in queries, allowing you to perform pattern matching on string fields. Regular expressions are useful when you need to find documents based on specific patterns or patterns that cannot be easily expressed using other query operators.
Here’s an example of using a regular expression to find documents where the name
field starts with the letter “A”:
db.users.find({ name: /^A/ })
In this example, the ^
character denotes the start of the string, and the A
character is the pattern to match.
Related Article: How to Add a Field with a Blank Value in MongoDB
Query Sorting
Sorting is another important aspect of querying in MongoDB. You can specify the order in which the documents should be returned based on one or more fields.
Here’s an example of sorting documents in ascending order based on the age
field:
db.users.find().sort({ age: 1 })
In this example, the sort()
method is used to specify the sorting order. The 1
indicates ascending order, while -1
would indicate descending order.
Query Limit
The limit()
method allows you to specify the maximum number of documents to return from a query. This can be useful when you only need a subset of the result set.
Here’s an example of limiting the result to the first 10 documents:
db.users.find().limit(10)
In this example, the limit()
method is used to restrict the result set to 10 documents.
Writing Complex Queries in MongoDB
MongoDB provides useful features and operators that allow you to write complex queries to meet your specific requirements. By combining multiple operators, you can create sophisticated queries that retrieve precise results.
Here’s an example of a complex query that combines multiple operators:
db.users.find({ $and: [ { age: { $gte: 25 } }, { $or: [ { status: "active" }, { status: "inactive" } ] } ] })
In this example, the query retrieves documents where the age
field is greater than or equal to 25 and the status
field is either “active” or “inactive”.
Related Article: How to Use Range Queries in MongoDB
Common Query Operators in MongoDB
MongoDB provides a wide range of query operators that can be used to perform various operations on documents. Here are some commonly used query operators:
– $eq
: Matches values that are equal to a specified value.
– $ne
: Matches values that are not equal to a specified value.
– $gt
: Matches values that are greater than a specified value.
– $gte
: Matches values that are greater than or equal to a specified value.
– $lt
: Matches values that are less than a specified value.
– $lte
: Matches values that are less than or equal to a specified value.
– $in
: Matches any of the values specified in an array.
– $nin
: Matches none of the values specified in an array.
– $and
: Matches all the specified conditions.
– $or
: Matches any of the specified conditions.
– $not
: Inverts the effect of a query expression.
– $exists
: Matches documents that have the specified field.
– $type
: Matches documents that have the specified BSON type.
– $all
: Matches arrays that contain all the specified elements.
– $elemMatch
: Matches arrays that contain at least one element that matches all the specified conditions.
– $size
: Matches arrays that have the specified number of elements.
– $expr
: Allows the use of aggregation expressions within the query language.
– $jsonSchema
: Validates documents against the given JSON Schema.
Improving Performance of MongoDB Queries
To improve the performance of MongoDB queries, consider the following best practices:
1. Use indexes: Properly indexing your collections can significantly improve query performance. Analyze your query patterns and create indexes on the fields commonly used in queries.
2. Limit the result set: Use the limit()
method to restrict the number of documents returned by a query. This can reduce the amount of data transferred and improve query response times.
3. Use query projection: Specify only the fields you need in the result set using query projection. This can reduce the size of the returned documents and improve query performance.
4. Avoid unnecessary sorting: If you don’t need the documents in a specific order, avoid sorting them. Sorting can be an expensive operation, especially on large datasets.
5. Optimize query patterns: Analyze your query patterns and optimize them for better performance. Avoid using complex operators or nested queries unless necessary.
Query Projection in MongoDB
Query projection allows you to specify which fields to include or exclude from the result set of a query. This can be useful when you only need specific fields and want to reduce the size of the returned documents.
Here’s an example of including only the name
and email
fields in the result set:
db.users.find({}, { name: 1, email: 1, _id: 0 })
In this example, the second parameter of the find()
method specifies the projection. The 1
indicates that the field should be included, while the 0
indicates exclusion.
Related Article: Crafting Query Operators in MongoDB
Regular Expressions in MongoDB Queries
MongoDB supports regular expressions in queries, allowing you to perform pattern matching on string fields. Regular expressions are useful when you need to find documents based on specific patterns or patterns that cannot be easily expressed using other query operators.
Here’s an example of using a regular expression to find documents where the name
field starts with the letter “A”:
db.users.find({ name: /^A/ })
In this example, the ^
character denotes the start of the string, and the A
character is the pattern to match.
Sorting Results of MongoDB Queries
Sorting is another important aspect of querying in MongoDB. You can specify the order in which the documents should be returned based on one or more fields.
Here’s an example of sorting documents in ascending order based on the age
field:
db.users.find().sort({ age: 1 })
In this example, the sort()
method is used to specify the sorting order. The 1
indicates ascending order, while -1
would indicate descending order.
Limiting Results in MongoDB Queries
The limit()
method allows you to specify the maximum number of documents to return from a query. This can be useful when you only need a subset of the result set.
Here’s an example of limiting the result to the first 10 documents:
db.users.find().limit(10)
In this example, the limit()
method is used to restrict the result set to 10 documents.
Related Article: Using Multi-Indexes with MongoDB Queries
Best Practices for Writing Efficient MongoDB Queries
To ensure efficient MongoDB queries, consider the following best practices:
1. Design your schema appropriately: Understand your data and query patterns to design an efficient schema. Normalize or denormalize your data based on the specific requirements of your application.
2. Use proper indexing: Identify the fields that are frequently used in queries and create indexes on those fields. Use compound indexes when necessary.
3. Take advantage of query projection: Only retrieve the fields you need in the result set. Avoid unnecessary data transfer and reduce the size of the returned documents.
4. Optimize query patterns: Analyze your query patterns and optimize them for better performance. Avoid using complex operators or nested queries unless necessary.
5. Monitor and analyze query performance: Use tools like the MongoDB profiler and explain() method to analyze the execution plans of your queries. Identify slow queries and optimize them for better performance.
Real-World Examples of MongoDB Queries
Let’s take a look at some real-world examples of MongoDB queries to see how they can be applied in practice.
Example 1: Find all active users who have made a purchase in the last 30 days:
db.users.find({ $and: [ { status: "active" }, { last_purchase_date: { $gte: new Date("2022-01-01") } } ] })
In this example, the query retrieves documents where the status
field is “active” and the last_purchase_date
field is greater than or equal to January 1, 2022.
Example 2: Find all documents where the tags
array contains both “mongodb” and “query”:
db.articles.find({ tags: { $all: ["mongodb", "query"] } })
In this example, the query retrieves documents where the tags
array contains both “mongodb” and “query”.
Limitations and Constraints in MongoDB Queries
While MongoDB provides a flexible and useful querying system, there are some limitations and constraints to be aware of:
1. Lack of joins: Unlike relational databases, MongoDB does not support joins. You need to denormalize your data or perform multiple queries to achieve the equivalent functionality.
2. Limited transaction support: MongoDB supports multi-document transactions, but they are not available in all configurations. Transactions are only supported on replica sets and sharded clusters in certain configurations.
3. Inflexible schema changes: Changing the schema of a collection in MongoDB can be challenging, especially when dealing with large datasets. It requires careful planning and migration strategies to avoid data inconsistencies.
4. Aggregation pipeline limitations: While the aggregation pipeline in MongoDB allows for complex data transformations, it has some limitations in terms of memory usage and performance. Large datasets or complex pipelines may require additional optimization.
5. Inefficient queries without proper indexing: Without proper indexing, MongoDB queries can be slow, especially on large datasets. It is important to understand the query patterns and create appropriate indexes to optimize query performance.