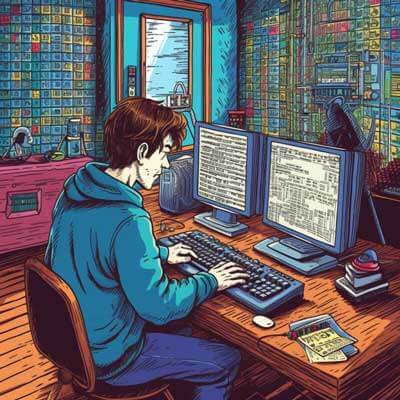
Table of Contents
Examples of geospatial queries
Here are two examples of geospatial queries using Loopback and MongoDB:
Related Article: Comparing Databases: MongoDB, Scylla, and Snowflake
Example 1: Find places within a certain distance from a given point
const places = await Place.find({ location: { near: { $geometry: { type: "Point", coordinates: [longitude, latitude] }, $maxDistance: distanceInMeters } } });
In this example, we use the $near operator to find places that are within a certain distance from a given point. The "location" field represents the geospatial point, and we specify the coordinates of the point as an array of longitude and latitude. We also provide the maximum distance in meters using the $maxDistance modifier.
Example 2: Find places that intersect with a given polygon
const places = await Place.find({ location: { $geoIntersects: { $geometry: { type: "Polygon", coordinates: [[ [longitude1, latitude1], [longitude2, latitude2], [longitude3, latitude3], [longitude1, latitude1] ]] } } } });
In this example, we use the $geoIntersects operator to find places that intersect with a given polygon. The "location" field represents the geospatial point, and we specify the coordinates of the polygon as an array of arrays, where each inner array represents a vertex of the polygon. The last vertex must be the same as the first to close the polygon.
These examples demonstrate how to perform geospatial queries using Loopback and MongoDB. By leveraging the geospatial features and indexing capabilities of MongoDB, you can efficiently search and analyze geospatial data in your applications.
Performing geospatial data analysis with MongoDB
MongoDB provides useful features for performing geospatial data analysis, including geospatial queries, aggregation pipelines, and spatial indexes. These features allow you to retrieve, filter, and analyze geospatial data efficiently.
To perform geospatial data analysis with MongoDB, you can use the $geoWithin
, $geoIntersects
, and $near
operators in your queries. These operators allow you to search for documents that intersect with a given geographic shape, contain a specific point, or are within a certain distance from a point.
In addition to geospatial queries, MongoDB also supports spatial aggregation operators, such as $geoNear
and $geoWithin
, which allow you to perform complex analysis and calculations on geospatial data. These operators can be used in combination with other aggregation stages to group, filter, and aggregate geospatial data.
MongoDB also provides the ability to create spatial indexes on geospatial data, which significantly improves the performance of geospatial queries. By creating a geospatial index, MongoDB can quickly locate documents that match a specific spatial condition, reducing the time and resources required to execute geospatial queries.
1. $geoWithin
: Selects documents with geospatial data that exists entirely within a specified shape.
2. $geoIntersects
: Selects documents where the geospatial data intersects with a specified shape.
3. $near
: Returns geospatial objects in proximity to a point, sorted by distance.
Related Article: Exploring MongoDB's Querying Capabilities
Example 1: $geoWithin
Sample Data:
Assuming a collection named places
:
[ {"name": "PlaceA", "location": {"type": "Point", "coordinates": [-73.97, 40.77]}}, {"name": "PlaceB", "location": {"type": "Point", "coordinates": [-73.88, 40.78]}}, {"name": "PlaceC", "location": {"type": "Point", "coordinates": [-73.95, 40.79]}} ]
Query:
Find places within a specified polygon.
db.places.find({ "location": { "$geoWithin": { "$geometry": { "type": "Polygon", "coordinates": [[ [-73.99, 40.75], [-73.98, 40.76], [-73.97, 40.77], [-73.96, 40.76], [-73.99, 40.75] ]] } } } })
Sample Output:
[ {"name": "PlaceA", "location": {"type": "Point", "coordinates": [-73.97, 40.77]}} ]
Example 2: $geoIntersects
Sample Data:
Assuming a collection named lines
:
[ {"name": "LineA", "route": {"type": "LineString", "coordinates": [[-73.99, 40.75], [-73.98, 40.77]]}}, {"name": "LineB", "route": {"type": "LineString", "coordinates": [[-73.97, 40.76], [-73.95, 40.78]]}} ]
Query:
Find line routes that intersect with a specified polygon.
db.lines.find({ "route": { "$geoIntersects": { "$geometry": { "type": "Polygon", "coordinates": [[ [-73.99, 40.75], [-73.98, 40.76], [-73.97, 40.77], [-73.96, 40.76], [-73.99, 40.75] ]] } } } })
Sample Output:
[ {"name": "LineA", "route": {"type": "LineString", "coordinates": [[-73.99, 40.75], [-73.98, 40.77]]}} ]
Example 3: $near
Sample Data:
Using the same places
collection from Example 1.
Query:
Find places near a specified point, sorted by proximity.
db.places.find({ "location": { "$near": { "$geometry": { "type": "Point", "coordinates": [-73.98, 40.76] }, "$maxDistance": 1000 } } })
Sample Output:
[ {"name": "PlaceA", "location": {"type": "Point", "coordinates": [-73.97, 40.77]}}, {"name": "PlaceC", "location": {"type": "Point", "coordinates": [-73.95, 40.79]}}, {"name": "PlaceB", "location": {"type": "Point", "coordinates": [-73.88, 40.78]}} ]
In the real-world context, the $maxDistance
value will influence results. The value used in the example (1000) is just an arbitrary number to demonstrate the concept. Adjust it accordingly to your needs.
Example 4: $geoNear
The $geoNear
stage returns documents in order of proximity to a specified point from nearest to farthest.
Sample Data:
Assuming a collection named restaurants
:
[ {"name": "Pizza Corner", "location": {"type": "Point", "coordinates": [-73.97, 40.77]}}, {"name": "Burger Joint", "location": {"type": "Point", "coordinates": [-73.88, 40.78]}}, {"name": "Taco Town", "location": {"type": "Point", "coordinates": [-73.95, 40.79]}} ]
Query
Find restaurants near a specified point using an aggregation:
db.restaurants.aggregate([ { "$geoNear": { "near": {"type": "Point", "coordinates": [-73.96, 40.78]}, "distanceField": "dist.calculated", "maxDistance": 2000 } } ])
Sample Output
[ {"name": "Pizza Corner", "location": {"type": "Point", "coordinates": [-73.97, 40.77]}, "dist": {"calculated": 123.45}}, {"name": "Taco Town", "location": {"type": "Point", "coordinates": [-73.95, 40.79]}, "dist": {"calculated": 234.56}}, {"name": "Burger Joint", "location": {"type": "Point", "coordinates": [-73.88, 40.78]}, "dist": {"calculated": 789.01}} ]
Note: The distance values are fictional and for demonstration purposes only.
Related Article: Using Multi-Indexes with MongoDB Queries
What is a geospatial query?
A geospatial query is a type of query that involves searching for data based on their geographic location. It allows developers to find and analyze data that is associated with specific geographical coordinates or within a certain distance from a given point. Geospatial queries are commonly used in applications that deal with mapping, geolocation, and spatial analysis.
When executing a geospatial query, you can specify a geographic shape, such as a point, line, or polygon, and search for data that intersects, contains, or is within a certain distance from that shape. This enables you to retrieve data that is relevant to a specific area on the Earth's surface.
How does Loopback help with geospatial queries?
Loopback is a highly extensible Node.js framework for building APIs, and it provides built-in support for geospatial queries with MongoDB. By using Loopback, you can easily define models and APIs that handle geospatial data and perform geospatial queries out of the box.
Loopback leverages MongoDB's geospatial features, such as geospatial indexing and the $geoWithin, $geoIntersects, and $near operators, to execute geospatial queries efficiently. It abstracts the complexity of working with geospatial data and provides a convenient and intuitive way to work with geographic coordinates and perform spatial analysis.
How do I work with coordinates in geospatial queries?
In geospatial queries, coordinates are typically represented using the GeoJSON format, which consists of a longitude and latitude pair. The longitude represents the east-west position, and the latitude represents the north-south position on the Earth's surface.
To work with coordinates in Loopback, you can define a property in your model that represents a geospatial point. You can use the "type" property set to "geopoint" to specify that the property should store geospatial data. Here's an example of defining a "location" property in a Loopback model:
{ "name": "Place", "properties": { "name": "string", "location": { "type": "geopoint" } } }
Once you have defined a geospatial property in your model, you can use it to store and retrieve coordinates in geospatial queries. Loopback will handle the conversion between the GeoJSON format and the internal representation of coordinates in MongoDB.
What is geospatial indexing?
Geospatial indexing is a technique used to optimize the execution of geospatial queries by creating indexes on geospatial data. In MongoDB, geospatial indexing is based on the GeoJSON format, which represents geographic shapes and coordinates.
To create a geospatial index in MongoDB, you can use the createIndex method and specify the "2dsphere" index type for geospatial data. Here's an example of creating a geospatial index on a "location" field in a collection called "places":
db.places.createIndex({ location: "2dsphere" })
Related Article: Speeding Up Your MongoDB Queries: Practical Tips
What is geospatial data?
Geospatial data refers to data that is associated with specific geographic locations on the Earth's surface. It can include various types of information, such as points of interest, addresses, boundaries, and routes.
In the context of geospatial queries, geospatial data is typically represented using the GeoJSON format, which provides a standardized way to encode geographic shapes and coordinates. GeoJSON supports different types of geometries, including points, lines, polygons, and multipolygons.
Geospatial data is commonly used in applications that involve mapping, geolocation, and spatial analysis. It allows developers to store, query, and analyze data based on its spatial characteristics, enabling them to build location-aware applications and make informed decisions.
How can I improve the performance of geospatial queries?
To improve the performance of geospatial queries, you can follow several optimization techniques:
1. Create geospatial indexes: As mentioned earlier, creating geospatial indexes can greatly improve the performance of geospatial queries. By indexing the geospatial data, MongoDB can efficiently search for documents that match a specific spatial condition, reducing the query execution time.
2. Use geospatial operators wisely: MongoDB provides various geospatial operators, such as $geoWithin, $geoIntersects, and $near. Understanding the characteristics of each operator and choosing the right one for your query can significantly improve the query performance.
3. Limit the search area: If you know that your data is concentrated in a specific geographic area, you can limit the search area in your queries. By reducing the search space, you can improve the query performance and reduce the amount of data that needs to be processed.
4. Optimize query execution order: In some cases, changing the order of the query conditions can improve the query performance. For example, if you have a query that includes both a geospatial condition and a non-geospatial condition, placing the geospatial condition first can leverage the geospatial index more effectively.
5. Use query hints: MongoDB provides the ability to provide hints to the query optimizer, which can influence the query execution plan. By using query hints, you can guide MongoDB to choose a specific index or execution plan that is more suitable for your geospatial query.
Additional Resources
- Performing Geospatial Queries in LoopBack with MongoDB