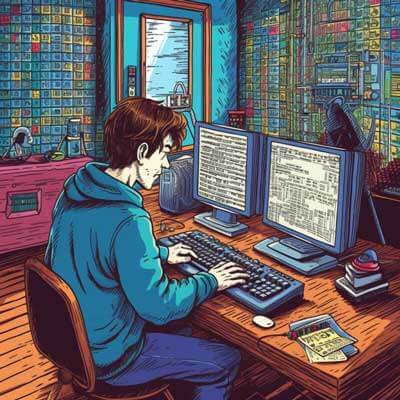
Table of Contents
Index Intersection in MongoDB
In MongoDB, index intersection refers to the ability for a query to utilize multiple indexes to optimize its execution. This feature allows MongoDB to combine multiple indexes and use them together to retrieve the required data efficiently.
Consider a scenario where you have a collection of documents representing users, and you want to find all the users with a specific age and a particular email domain. In this case, you can create separate indexes on the "age" and "email" fields. MongoDB can then intersect these indexes to fetch the documents that match both criteria.
To demonstrate index intersection, let's create a collection called "users" and insert some sample documents:
db.users.insertMany([ { name: "John", age: 25, email: "john@example.com" }, { name: "Jane", age: 30, email: "jane@example.com" }, { name: "Alice", age: 25, email: "alice@example.com" }, { name: "Bob", age: 35, email: "bob@example.com" } ]);
Now, let's create separate indexes on the "age" and "email" fields:
db.users.createIndex({ age: 1 }); db.users.createIndex({ email: 1 });
With these indexes in place, we can use them together in a query to find users with a specific age and email domain:
db.users.find({ age: 25, email: { $regex: /@example.com$/ } }).explain("executionStats");
The explain() method with the "executionStats" option will display the execution plan for the query, including the indexes used. In the output, you will see that MongoDB is able to intersect the indexes on "age" and "email" to optimize the query execution.
Related Article: How to Run Geospatial Queries in Nodejs Loopback & MongoDB
Compound Indexes in MongoDB
Compound indexes, also known as composite indexes, are indexes that include multiple fields. They allow queries to efficiently retrieve data based on multiple criteria by combining multiple fields into a single index.
To illustrate the use of compound indexes, let's consider a collection of documents representing products. Each document has fields for "category" and "price", and we want to query for products in a specific category with a price range.
First, let's create the "products" collection and insert some sample documents:
db.products.insertMany([ { name: "Product 1", category: "Electronics", price: 100 }, { name: "Product 2", category: "Electronics", price: 200 }, { name: "Product 3", category: "Clothing", price: 50 }, { name: "Product 4", category: "Clothing", price: 150 } ]);
Now, we can create a compound index on the "category" and "price" fields:
db.products.createIndex({ category: 1, price: 1 });
This index allows efficient querying for products in a specific category with a price range. For example, to find all electronics products with a price between 100 and 200, we can execute the following query:
db.products.find({ category: "Electronics", price: { $gte: 100, $lte: 200 } }).explain("executionStats");
The explain() method with the "executionStats" option will show that MongoDB is able to utilize the compound index to optimize the query execution.
Multi-key Indexes in MongoDB
In MongoDB, multi-key indexes are used to index arrays. They allow efficient querying and sorting based on array values.
To demonstrate the use of multi-key indexes, let's consider a collection of documents representing articles. Each article document has a "tags" field that contains an array of tags associated with the article.
First, let's create the "articles" collection and insert some sample documents:
db.articles.insertMany([ { title: "Article 1", tags: ["mongodb", "database"] }, { title: "Article 2", tags: ["mongodb", "query"] }, { title: "Article 3", tags: ["indexing", "performance"] }, { title: "Article 4", tags: ["query", "performance"] } ]);
Now, we can create a multi-key index on the "tags" field:
db.articles.createIndex({ tags: 1 });
This index allows efficient querying and sorting based on the values in the "tags" array. For example, to find all articles with the tag "mongodb", we can execute the following query:
db.articles.find({ tags: "mongodb" }).explain("executionStats");
The explain() method with the "executionStats" option will show that MongoDB is able to utilize the multi-key index to optimize the query execution.
Index Selection in MongoDB Queries
MongoDB uses the query optimizer to select the most efficient index for a given query. The query optimizer evaluates the available indexes and chooses the best index based on various factors such as index key order, index size, and selectivity.
To understand the index selection process, let's consider a scenario where we have a collection of documents representing orders. Each order document has fields for "customer_id" and "order_date", and we want to find all orders for a specific customer within a date range.
First, let's create the "orders" collection and insert some sample documents:
db.orders.insertMany([ { customer_id: 1, order_date: ISODate("2022-01-01") }, { customer_id: 1, order_date: ISODate("2022-01-05") }, { customer_id: 2, order_date: ISODate("2022-01-02") }, { customer_id: 2, order_date: ISODate("2022-01-06") } ]);
Now, we can create separate indexes on the "customer_id" and "order_date" fields:
db.orders.createIndex({ customer_id: 1 }); db.orders.createIndex({ order_date: 1 });
When executing a query to find orders for a specific customer within a date range, MongoDB's query optimizer will evaluate the available indexes and select the most efficient one. For example, to find all orders for customer 1 between January 1st and January 5th, we can execute the following query:
db.orders.find({ customer_id: 1, order_date: { $gte: ISODate("2022-01-01"), $lte: ISODate("2022-01-05") } }).explain("executionStats");
The explain() method with the "executionStats" option will show the execution plan for the query, including the selected index. In the output, you will see that MongoDB is able to choose the index on "customer_id" and "order_date" to optimize the query execution.
Related Article: How to Add a Field with a Blank Value in MongoDB
Index Merging in MongoDB
In MongoDB, index merging refers to the ability to merge multiple indexes to satisfy a query. This feature allows MongoDB to combine the benefits of multiple indexes and optimize query execution.
To illustrate index merging, let's consider a scenario where we have a collection of documents representing products. Each product document has fields for "category" and "price", and we want to find all products in multiple categories with a specific price range.
First, let's create the "products" collection and insert some sample documents:
db.products.insertMany([ { name: "Product 1", category: "Electronics", price: 100 }, { name: "Product 2", category: "Electronics", price: 200 }, { name: "Product 3", category: "Clothing", price: 50 }, { name: "Product 4", category: "Clothing", price: 150 } ]);
Now, we can create separate indexes on the "category" and "price" fields:
db.products.createIndex({ category: 1 }); db.products.createIndex({ price: 1 });
When executing a query to find products in multiple categories with a specific price range, MongoDB's query optimizer will evaluate the available indexes and merge them if necessary. For example, to find all products in the "Electronics" and "Clothing" categories with a price between 100 and 200, we can execute the following query:
db.products.find({ category: { $in: ["Electronics", "Clothing"] }, price: { $gte: 100, $lte: 200 } }).explain("executionStats");
The explain() method with the "executionStats" option will show the execution plan for the query, including the merged index. In the output, you will see that MongoDB is able to merge the indexes on "category" and "price" to optimize the query execution.
Query Planner in MongoDB
The query planner in MongoDB is responsible for generating and evaluating different query execution plans based on the available indexes and query predicates. It analyzes the query and index statistics to select the most efficient plan for query execution.
To understand the query planner, let's consider a scenario where we have a collection of documents representing products. Each product document has fields for "category" and "price", and we want to find all products in a specific category with a price greater than a certain threshold.
First, let's create the "products" collection and insert some sample documents:
db.products.insertMany([ { name: "Product 1", category: "Electronics", price: 100 }, { name: "Product 2", category: "Electronics", price: 200 }, { name: "Product 3", category: "Clothing", price: 50 }, { name: "Product 4", category: "Clothing", price: 150 } ]);
Now, we can create an index on the "category" field:
db.products.createIndex({ category: 1 });
When executing a query to find products in a specific category with a price greater than a certain threshold, the query planner will generate and evaluate different execution plans. For example, to find all products in the "Electronics" category with a price greater than 150, we can execute the following query:
db.products.find({ category: "Electronics", price: { $gt: 150 } }).explain("executionStats");
The explain() method with the "executionStats" option will show the execution plan for the query. In the output, you will see that the query planner evaluates different plans and selects the most efficient one based on the available index and query predicates.
Query Optimization in MongoDB
Query optimization in MongoDB involves optimizing the execution of queries to improve their performance. MongoDB provides various techniques and features to optimize queries, including index usage, query planner, and execution plan analysis.
To optimize a query in MongoDB, you can follow these steps:
1. Analyze the query: Understand the query requirements and the fields involved in the query predicates.
2. Create appropriate indexes: Create indexes on the fields involved in the query predicates to improve query performance. Consider using compound indexes for queries with multiple criteria.
3. Use explain() method: Use the explain() method with the "executionStats" option to view the execution plan for the query. Analyze the execution plan to understand how MongoDB is executing the query and whether it is utilizing the indexes effectively.
4. Optimize index selection: If MongoDB is not selecting the desired index, you can use index hints or modify the query predicates to guide the query optimizer towards the desired index.
5. Monitor and analyze query performance: Continuously monitor the performance of queries and analyze the execution plans to identify potential bottlenecks or areas for optimization. Consider using the MongoDB profiler or performance monitoring tools to gather data and insights.
Query Execution Plans in MongoDB
Query execution plans in MongoDB provide detailed information about how a query is executed, including the indexes used, the number of documents examined, and the execution time.
To view the execution plan of a query in MongoDB, you can use the explain() method with the "executionStats" option. This method returns a document that provides insights into the query execution.
The execution plan document includes various fields, such as:
- "executionSuccess": Indicates whether the query execution was successful.
- "nReturned": The number of documents returned by the query.
- "totalKeysExamined": The total number of index keys examined during the query execution.
- "totalDocsExamined": The total number of documents examined during the query execution.
- "executionTimeMillis": The execution time of the query in milliseconds.
For example, let's consider a scenario where we have a collection of documents representing orders. Each order document has fields for "customer_id" and "order_date", and we want to find all orders for a specific customer within a date range.
First, let's create the "orders" collection and insert some sample documents:
db.orders.insertMany([ { customer_id: 1, order_date: ISODate("2022-01-01") }, { customer_id: 1, order_date: ISODate("2022-01-05") }, { customer_id: 2, order_date: ISODate("2022-01-02") }, { customer_id: 2, order_date: ISODate("2022-01-06") } ]);
Now, we can create separate indexes on the "customer_id" and "order_date" fields:
db.orders.createIndex({ customer_id: 1 }); db.orders.createIndex({ order_date: 1 });
To view the execution plan of a query, we can execute the following query with the explain() method:
db.orders.find({ customer_id: 1, order_date: { $gte: ISODate("2022-01-01"), $lte: ISODate("2022-01-05") } }).explain("executionStats");
The output will include detailed information about the query execution, such as the indexes used, the number of documents examined, and the execution time. Analyzing the execution plan can help identify potential bottlenecks or areas for query optimization.
Related Article: Comparing Databases: MongoDB, Scylla, and Snowflake
Index Utilization in MongoDB
Index utilization in MongoDB refers to the effective use of indexes to optimize query performance. MongoDB utilizes indexes by selecting the most efficient index for a given query and using it to retrieve the required data.
To ensure effective index utilization in MongoDB, consider the following best practices:
1. Create appropriate indexes: Analyze the query patterns and create indexes on the fields involved in the query predicates. Use compound indexes for queries with multiple criteria.
2. Monitor index usage: Monitor the usage of indexes by enabling the MongoDB profiler or using performance monitoring tools. Identify queries that are not utilizing indexes effectively and optimize them.
3. Analyze execution plans: Use the explain() method with the "executionStats" option to view the execution plans for queries. Analyze the execution plans to understand how MongoDB is utilizing indexes and identify areas for optimization.
4. Optimize query predicates: Modify query predicates to guide the query optimizer towards the desired index. Consider using index hints to explicitly specify the index to be used.
5. Regularly update indexes: If the data distribution or query patterns change over time, reevaluate and update the indexes accordingly. Regularly monitor the performance of queries and make necessary adjustments to the indexes.
MongoDB Query Performance with Multiple Indexes
MongoDB query performance with multiple indexes can be improved by utilizing index intersection, compound indexes, and index merging.
Index intersection allows MongoDB to combine multiple indexes to optimize query execution. By creating separate indexes on different fields and using them together in a query, MongoDB can efficiently retrieve the required data. This approach is useful when queries involve multiple criteria that are indexed separately. For example, finding all users with a specific age and email domain can utilize the indexes on the "age" and "email" fields.
Compound indexes, also known as composite indexes, combine multiple fields into a single index. They allow efficient querying based on multiple criteria. By creating a compound index on the fields involved in a query, MongoDB can optimize query execution by utilizing the compound index. For example, finding all products in a specific category with a price range can utilize a compound index on the "category" and "price" fields.
Index merging in MongoDB allows the merging of multiple indexes to satisfy a query. When a query involves multiple criteria that are indexed separately, MongoDB can merge the indexes to optimize query execution. For example, finding all products in multiple categories with a specific price range can utilize the indexes on the "category" and "price" fields.
To ensure optimal query performance with multiple indexes in MongoDB, consider the following best practices:
1. Analyze query patterns: Understand the query patterns and the fields involved in the query predicates. Identify queries that involve multiple criteria and create appropriate indexes to optimize their execution.
2. Use compound indexes: Create compound indexes on fields involved in queries with multiple criteria. Consider the order of fields in the compound index based on query selectivity.
3. Monitor index usage: Regularly monitor the usage of indexes by enabling the MongoDB profiler or using performance monitoring tools. Identify queries that are not utilizing indexes effectively and optimize them.
4. Analyze execution plans: Use the explain() method with the "executionStats" option to view the execution plans for queries. Analyze the execution plans to understand how MongoDB is utilizing indexes and identify areas for optimization.
Viewing the Execution Plan of a MongoDB Query
To view the execution plan of a MongoDB query, you can use the explain() method with the "executionStats" option. This method provides detailed information about how a query is executed, including the indexes used, the number of documents examined, and the execution time.
To demonstrate the viewing of the execution plan, let's consider a scenario where we have a collection of documents representing orders. Each order document has fields for "customer_id" and "order_date", and we want to find all orders for a specific customer within a date range.
First, let's create the "orders" collection and insert some sample documents:
db.orders.insertMany([ { customer_id: 1, order_date: ISODate("2022-01-01") }, { customer_id: 1, order_date: ISODate("2022-01-05") }, { customer_id: 2, order_date: ISODate("2022-01-02") }, { customer_id: 2, order_date: ISODate("2022-01-06") } ]);
Now, we can create separate indexes on the "customer_id" and "order_date" fields:
db.orders.createIndex({ customer_id: 1 }); db.orders.createIndex({ order_date: 1 });
To view the execution plan of a query, we can execute the following query with the explain() method:
db.orders.find({ customer_id: 1, order_date: { $gte: ISODate("2022-01-01"), $lte: ISODate("2022-01-05") } }).explain("executionStats");
The output will include detailed information about the query execution, such as the indexes used, the number of documents examined, and the execution time. Analyzing the execution plan can help identify potential bottlenecks or areas for query optimization.
Utilizing Indexes for Query Optimization in MongoDB
In MongoDB, indexes play a crucial role in query optimization. By creating appropriate indexes and utilizing them effectively, you can significantly improve query performance.
To utilize indexes for query optimization in MongoDB, consider the following best practices:
1. Analyze query patterns: Understand the query patterns and the fields involved in the query predicates. Identify frequently executed queries and create indexes on the fields involved in the query predicates.
2. Choose the right index type: MongoDB supports various index types, such as single-field indexes, compound indexes, and multi-key indexes. Choose the appropriate index type based on the query requirements.
3. Create compound indexes: For queries with multiple criteria, create compound indexes on the fields involved in the query. Consider the order of fields in the compound index based on query selectivity.
4. Use index hints: If MongoDB is not selecting the desired index, you can use index hints to explicitly specify the index to be used. Index hints can guide the query optimizer towards the desired index.
5. Regularly monitor index usage: Enable the MongoDB profiler or use performance monitoring tools to monitor the usage of indexes. Identify queries that are not utilizing indexes effectively and optimize them.
6. Analyze execution plans: Use the explain() method with the "executionStats" option to view the execution plans for queries. Analyze the execution plans to understand how MongoDB is utilizing indexes and identify areas for optimization.