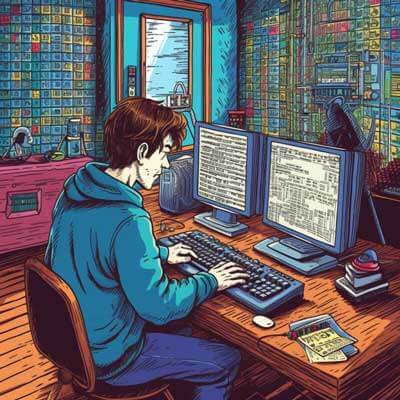
Table of Contents
Is Data Fetched Immediately When Querying in MongoDB?
When querying data in MongoDB, the data is not fetched immediately. MongoDB employs a lazy loading mechanism, which means that the data is loaded into memory only when it is required for further processing.
MongoDB's lazy loading mechanism offers several benefits, including reduced memory usage and improved query performance. By loading data into memory only when it is needed, MongoDB minimizes the memory footprint and optimizes resource utilization.
The lazy loading mechanism in MongoDB works as follows:
1. Query Parsing: MongoDB parses the query and determines the query plan, which is a strategy for executing the query efficiently. The query plan takes into account factors such as indexes, data distribution, and available resources.
2. Query Execution: Once the query plan is determined, MongoDB executes the query by retrieving the necessary data from disk or memory. However, the data is not loaded into memory immediately.
3. Document Loading: During the query execution process, MongoDB loads the required data into memory only when it is needed for further processing. This allows MongoDB to optimize memory usage and improve query performance by reducing the memory footprint.
4. Data Processing: Once the data is loaded into memory, MongoDB performs operations such as filtering, sorting, and aggregating the data. The data is processed as needed, minimizing the memory usage and improving the query performance.
Related Article: Comparing Databases: MongoDB, Scylla, and Snowflake
Example 1: Lazy Loading of Documents
Suppose we have a MongoDB deployment where the data is stored on disk. When executing a query, MongoDB does not fetch all the documents immediately. Instead, MongoDB loads the documents into memory only when they are required for further processing.
// Query to find all documents in the "users" collection db.users.find({})
In this example, MongoDB does not load all the documents from the "users" collection into memory immediately. The documents are loaded into memory only when they are needed for further processing, such as filtering or projecting specific fields.
Example 2: Lazy Loading of Data for Aggregation
MongoDB's lazy loading mechanism is particularly beneficial when performing aggregations on large datasets. MongoDB loads the data into memory in chunks, only retrieving the necessary data for each stage of the aggregation pipeline.
Suppose we have a collection called "orders" that stores information about customer orders. Each document in the "orders" collection has fields such as orderNumber
, customerName
, and totalAmount
. We want to calculate the total amount of all orders.
// Aggregation pipeline to calculate the total amount of all orders db.orders.aggregate([ { $group: { _id: null, totalAmount: { $sum: "$totalAmount" } } } ])
In this example, MongoDB's lazy loading mechanism ensures that only the necessary data is loaded into memory for each stage of the aggregation pipeline. The data is loaded in chunks, minimizing the memory footprint and improving the aggregation performance.
MongoDB's lazy loading mechanism optimizes resource utilization and query performance, making it a useful choice for data-intensive applications.
What Happens Behind the Scenes When Executing a MongoDB Query?
When executing a query in MongoDB, a series of operations take place behind the scenes to retrieve and process the data efficiently. MongoDB's query execution process involves multiple stages, optimizations, and mechanisms to ensure optimal performance and resource utilization.
The steps that happen behind the scenes when executing a MongoDB query can be summarized as follows:
1. Query Parsing: MongoDB parses the query and determines the query plan, which is a strategy for executing the query efficiently. The query plan takes into account factors such as indexes, data distribution, and available resources.
2. Query Optimization: MongoDB's query optimizer analyzes the query plan and selects the most efficient query plan based on factors such as indexes, data distribution, and available resources. The query optimizer aims to minimize disk I/O operations and maximize in-memory processing.
3. Document Scanning: Once the query plan is determined, MongoDB scans the documents in the collection that match the query criteria. This involves accessing the data from disk or memory and loading it into memory for further processing.
4. Document Filtering: MongoDB applies any filtering conditions specified in the query to the scanned documents. This helps narrow down the result set to only include the documents that match the query criteria.
5. Document Projection: If the query specifies specific fields to include or exclude, MongoDB applies the projection stage to the filtered documents. This helps optimize the memory usage by only loading and processing the required fields.
6. Document Sorting: If the query specifies a sort order, MongoDB performs the sorting operation on the filtered documents. This ensures that the final result set is returned in the specified order.
7. Document Limiting: If the query specifies a limit on the number of documents to return, MongoDB applies the limit stage to the sorted documents. This helps reduce the memory usage and improves the query performance by returning only the required number of documents.
8. Result Set Generation: Finally, MongoDB generates the result set based on the filtered, projected, sorted, and limited documents. The result set is then returned to the client for further processing or display.
Behind the scenes, MongoDB's query execution process involves a combination of memory mapping, in-memory caching, index usage, and query optimization techniques. These mechanisms work together to ensure efficient query execution and optimal resource utilization.
Related Article: How to Query MongoDB by Time
Example 1: Query Execution Process with Filtering and Projection
Suppose we have a collection called "products" that stores information about different products. Each document in the "products" collection has fields such as name
, price
, and category
. We want to retrieve all products in the "electronics" category with a price greater than $100.
// Query to find all products in the "electronics" category with a price greater than $100 db.products.find({ category: "electronics", price: { $gt: 100 } })
In this example, MongoDB's query execution process involves the following steps:
1. Query Parsing: MongoDB parses the query and determines the query plan.
2. Query Optimization: MongoDB's query optimizer analyzes the query plan and selects the most efficient query plan.
3. Document Scanning: MongoDB scans the documents in the "products" collection.
4. Document Filtering: MongoDB applies the filtering conditions to the scanned documents.
5. Document Projection: MongoDB applies the projection stage to the filtered documents.
6. Result Set Generation: MongoDB generates the final result set based on the filtered documents and returns it to the client.
Example 2: Query Execution Process with Index Usage
MongoDB's index usage is an important factor in optimizing query execution and data retrieval. Indexes allow MongoDB to efficiently locate and retrieve the documents that match the query criteria.
Suppose we have a collection called "users" that stores information about users. Each document in the "users" collection has fields such as name
, age
, and city
. We want to retrieve all users who are older than 30 and live in the city of "New York".
// Query to find all users who are older than 30 and live in the city of "New York" db.users.find({ age: { $gt: 30 }, city: "New York" })
In this example, MongoDB's query execution process involves the following steps:
1. Query Parsing: MongoDB parses the query and determines the query plan.
2. Query Optimization: MongoDB's query optimizer analyzes the query plan and selects the most efficient query plan.
3. Document Scanning: MongoDB scans the documents in the "users" collection.
4. Document Filtering: MongoDB applies the filtering conditions to the scanned documents.
5. Result Set Generation: MongoDB generates the final result set based on the filtered documents and returns it to the client.
If there is an index on the age
field, MongoDB can utilize the index to efficiently locate the documents that match the age criteria (age: { $gt: 30 }
). This reduces the number of documents that need to be scanned and loaded into memory during the query execution process, improving efficiency and performance.
MongoDB's query execution process ensures optimal performance and resource utilization, making it a useful choice for handling large datasets and complex queries.
Does MongoDB Fetch All Documents Matching a Query Condition?
When querying documents in MongoDB, the query execution process involves retrieving documents that match the query condition. However, MongoDB does not necessarily fetch all documents matching the query condition at once.
MongoDB retrieves documents in batches, also known as "chunks," during the query execution process. By fetching documents in chunks, MongoDB can efficiently utilize system resources, optimize memory usage, and improve query performance.
The size of the document chunks fetched by MongoDB depends on various factors, including the available system resources, query complexity, and the size of the documents. MongoDB dynamically adjusts the chunk size based on these factors to achieve optimal performance.
Fetching documents in chunks allows MongoDB to process and return query results incrementally, reducing the memory footprint and improving the overall query performance.
Example 1: Querying Documents with a Large Result Set
Suppose we have a collection called "users" that stores information about users. Each document in the "users" collection has fields such as name
, age
, and city
. We want to retrieve all users who are older than 30.
// Query to find all users who are older than 30 db.users.find({ age: { $gt: 30 } })
In this example, if the "users" collection contains a large number of documents that match the query condition (age: { $gt: 30 }
), MongoDB will fetch the documents in chunks. This allows MongoDB to efficiently process and return the query results, minimizing the memory usage and improving the query performance.
Related Article: MongoDB Queries Tutorial
Example 2: Querying Documents with a Complex Query Condition
MongoDB can efficiently fetch documents that match complex query conditions by utilizing indexes and optimizing the query execution process. MongoDB dynamically adjusts the document chunk size based on the available system resources and the complexity of the query condition.
Suppose we have a collection called "products" that stores information about different products. Each document in the "products" collection has fields such as name
, price
, and category
. We want to retrieve all products in the "electronics" category with a price greater than $100.
// Query to find all products in the "electronics" category with a price greater than $100 db.products.find({ category: "electronics", price: { $gt: 100 } })
In this example, MongoDB will fetch the documents that match the query condition (category: "electronics"
and price: { $gt: 100 }
) in chunks. The size of the document chunks fetched by MongoDB dynamically adjusts based on the available system resources and the complexity of the query condition. This ensures efficient document retrieval and optimal query performance.
How MongoDB Handles Document Loading During Querying
When handling document loading during querying, MongoDB employs efficient mechanisms to ensure optimal performance and resource utilization. MongoDB uses a combination of in-memory caching, disk-based storage, and query optimization techniques to handle document loading effectively.
MongoDB's document loading process can be summarized as follows:
1. Memory Mapping: MongoDB uses memory mapping to map the data files stored on disk to memory. This allows MongoDB to efficiently access the data without the need for explicit disk I/O operations.
2. WiredTiger Storage Engine: MongoDB's default storage engine, WiredTiger, employs various techniques to optimize document loading. WiredTiger uses a combination of in-memory caching, compression, and multi-threaded access to maximize performance and minimize resource usage.
3. Query Optimization: MongoDB's query optimizer analyzes the query and determines the most efficient query plan based on factors such as indexes, data distribution, and available resources. The query optimizer selects a query plan that minimizes disk I/O and maximizes in-memory processing.
4. Index Usage: MongoDB utilizes indexes to efficiently locate and retrieve the documents that match the query criteria. By leveraging indexes, MongoDB can minimize the number of documents that need to be loaded into memory, improving query performance.
5. Working Set: MongoDB maintains a working set, which is a subset of the data that is frequently accessed by queries. By keeping the working set in memory, MongoDB reduces the need for disk I/O operations during query execution, resulting in faster document loading.
Example 1: Memory Mapping and In-Memory Caching
Suppose we have a MongoDB deployment where the data is stored on disk. When executing a query, MongoDB uses memory mapping to map the data files stored on disk to memory. This allows MongoDB to efficiently access the data without the need for explicit disk I/O operations.
Once the data is mapped to memory, MongoDB utilizes in-memory caching to store frequently accessed data in memory. This improves query performance by reducing the need for disk I/O operations during document loading.
// Query to find all documents in the "users" collection db.users.find({})
In this example, MongoDB maps the data files of the "users" collection to memory and utilizes in-memory caching to store frequently accessed documents. This allows MongoDB to load the documents efficiently during the query execution process.
Example 2: Query Optimization and Index Usage
MongoDB's query optimizer plays a crucial role in optimizing query execution and document loading. The query optimizer analyzes the query and determines the most efficient query plan based on factors such as indexes, data distribution, and available resources.
Suppose we have a collection called "products" that stores information about different products. Each document in the "products" collection has fields such as name
, price
, and category
. We want to retrieve all products in the "electronics" category with a price greater than $100.
// Query to find products in the "electronics" category with a price greater than $100 db.products.find({ category: "electronics", price: { $gt: 100 } })
In this example, MongoDB's query optimizer analyzes the query and determines the most efficient query plan. If there is an index on the category
and price
fields, MongoDB can utilize the indexes to efficiently locate and retrieve the documents that match the query criteria. This reduces the number of documents that need to be loaded into memory during the query execution process.
MongoDB's query optimizer and index usage techniques ensure that document loading during querying is handled efficiently, resulting in improved performance and resource utilization.