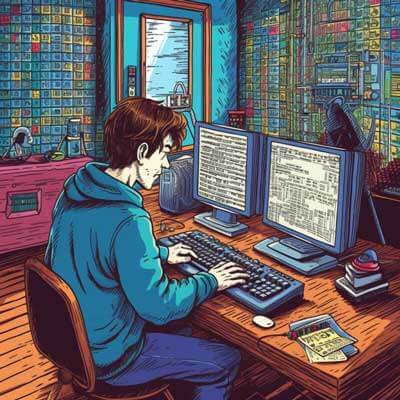
Table of Contents
Intro
When working with MongoDB, it is important to evaluate the performance of your queries to ensure optimal execution time. Evaluating query performance involves analyzing factors such as query speed, latency, and execution time. In this section, we will discuss how to evaluate MongoDB query performance and provide examples of code snippets to demonstrate the process.
To evaluate the performance of a MongoDB query, you can use the explain() method, which provides detailed information about the query execution plan. The explain() method returns a document that includes details such as the query plan, index usage, and execution statistics. Let's consider an example where we have a collection called "users" and we want to find all the users with a specific age:
db.users.find({ age: 25 }).explain()
The explain() method will return a document that provides information about the query execution plan. This includes details such as the number of documents scanned, the number of documents returned, and the execution time. By analyzing this information, you can identify potential bottlenecks and areas for optimization.
Related Article: Comparing Databases: MongoDB, Scylla, and Snowflake
Example 1: Evaluating Query Performance
Let's consider a scenario where we have a collection called "products" and we want to find all the products with a price greater than $50. We can evaluate the performance of this query using the explain() method:
db.products.find({ price: { $gt: 50 } }).explain()
The explain() method will provide information about the query execution plan. This includes details such as the number of documents scanned, the number of documents returned, and the execution time. By analyzing this information, we can determine if the query is performing efficiently or if there is room for improvement.
Example 2: Analyzing Query Performance
Let's consider another scenario where we have a collection called "orders" and we want to find all the orders placed by a specific customer. We can analyze the performance of this query using the explain() method:
db.orders.find({ customer_id: "abc123" }).explain()
The explain() method will provide information about the query execution plan. This includes details such as the number of documents scanned, the number of documents returned, and the execution time. By analyzing this information, we can gain insights into the performance of the query and identify potential areas for optimization.
Analyzing MongoDB Query Speed
Analyzing the speed of MongoDB queries is essential for optimizing query performance and improving the overall efficiency of your application. In this section, we will discuss how to analyze the speed of MongoDB queries and provide examples of code snippets to demonstrate the process.
To analyze the speed of a MongoDB query, you can use the explain() method along with the executionStats flag. This provides detailed information about the query execution, including the execution time. Let's consider an example where we have a collection called "users" and we want to find all the users with a specific age:
db.users.find({ age: 25 }).explain("executionStats")
The explain() method with the executionStats flag will return a document that includes details such as the execution time, the number of documents scanned, and the number of documents returned. By analyzing this information, you can determine the speed of the query and identify potential areas for optimization.
Related Article: MongoDB Queries Tutorial
Example 1: Analyzing Query Speed
Let's consider a scenario where we have a collection called "products" and we want to find all the products with a price greater than $50. We can analyze the speed of this query using the explain() method with the executionStats flag:
db.products.find({ price: { $gt: 50 } }).explain("executionStats")
The explain() method with the executionStats flag will provide information about the query execution, including the execution time, the number of documents scanned, and the number of documents returned. By analyzing this information, we can determine the speed of the query and identify potential areas for optimization.
Example 2: Optimizing Query Speed
Let's consider another scenario where we have a collection called "orders" and we want to find all the orders placed by a specific customer. We can optimize the speed of this query by using an index on the "customer_id" field:
db.orders.createIndex({ customer_id: 1 })
db.orders.find({ customer_id: "abc123" }).explain("executionStats")
Analyzing the speed of MongoDB queries is crucial for optimizing query performance. By using the explain() method with the executionStats flag, you can gain insights into the execution time, the number of documents scanned, and the number of documents returned. This information can help you identify bottlenecks, optimize query speed, and improve the overall efficiency of your MongoDB queries.
Optimizing MongoDB Query Execution Time
Optimizing the execution time of MongoDB queries is essential for improving the performance of your application. In this section, we will discuss various techniques for optimizing MongoDB query execution time and provide examples of code snippets to demonstrate the process.
One way to optimize the execution time of MongoDB queries is to use indexes. Indexes help MongoDB locate documents faster by creating a data structure that allows for efficient lookup based on the indexed fields. By creating appropriate indexes on the fields used in your queries, you can significantly improve query execution time.
Example 1: Creating Indexes
Let's consider a scenario where we have a collection called "products" and we frequently query products based on their price. To optimize the execution time of these queries, we can create an index on the "price" field:
db.products.createIndex({ price: 1 })
Related Article: How to Use Range Queries in MongoDB
Example 2: Analyzing Query Performance
Once you have created indexes on the relevant fields, you can analyze the performance of your queries using the explain() method. This provides detailed information about the query execution plan, including the execution time. Let's consider an example where we want to find all the products with a price greater than $50:
db.products.find({ price: { $gt: 50 } }).explain()
The explain() method will return a document that includes details such as the number of documents scanned, the number of documents returned, and the execution time. By analyzing this information, you can identify potential areas for optimization and fine-tune your indexes to improve query execution time.
Optimizing the execution time of MongoDB queries is crucial for improving the performance of your application. By creating appropriate indexes and analyzing query performance, you can significantly reduce query execution time and enhance the overall efficiency of your MongoDB queries.
MongoDB Query Latency
Understanding the latency of MongoDB queries is essential for optimizing query performance and ensuring a responsive application. In this section, we will discuss how to measure and understand MongoDB query latency and provide examples of code snippets to demonstrate the process.
MongoDB query latency refers to the time it takes for a query to be executed and for the results to be returned. Latency can be affected by various factors, including network latency, disk I/O, and query complexity. By measuring and understanding query latency, you can identify potential bottlenecks and optimize the performance of your queries.
To measure the latency of a MongoDB query, you can use the explain() method along with the executionStats flag. This provides detailed information about the query execution, including the execution time. Let's consider an example where we have a collection called "users" and we want to find all the users with a specific age:
db.users.find({ age: 25 }).explain("executionStats")
The explain() method with the executionStats flag will return a document that includes details such as the execution time, the number of documents scanned, and the number of documents returned. By analyzing this information, you can determine the latency of the query and identify potential areas for optimization.
Example 1: Measuring Query Latency
Let's consider a scenario where we have a collection called "products" and we want to find all the products with a price greater than $50. We can measure the latency of this query using the explain() method with the executionStats flag:
db.products.find({ price: { $gt: 50 } }).explain("executionStats")
The explain() method with the executionStats flag will provide information about the query execution, including the execution time, the number of documents scanned, and the number of documents returned. By analyzing this information, we can determine the latency of the query and identify potential areas for optimization.
Example 2: Analyzing Query Latency
Let's consider another scenario where we have a collection called "orders" and we want to find all the orders placed by a specific customer. We can analyze the latency of this query using the explain() method with the executionStats flag:
db.orders.find({ customer_id: "abc123" }).explain("executionStats")
The explain() method with the executionStats flag will provide information about the query execution, including the execution time, the number of documents scanned, and the number of documents returned. By analyzing this information, we can gain insights into the latency of the query and identify potential areas for optimization.
Understanding the latency of MongoDB queries is crucial for optimizing query performance and ensuring a responsive application. By measuring and analyzing query latency, you can identify potential bottlenecks, optimize query execution time, and improve the overall efficiency of your MongoDB queries.
Related Article: How to Run Geospatial Queries in Nodejs Loopback & MongoDB
Benchmarking MongoDB Query Performance
Benchmarking MongoDB query performance is a crucial step in evaluating the efficiency of your queries and identifying areas for optimization. In this section, we will discuss how to benchmark MongoDB query performance and provide examples of code snippets to demonstrate the process.
Benchmarking involves running a set of predefined queries and measuring their execution time. This allows you to compare the performance of different queries and identify potential bottlenecks. MongoDB provides a built-in tool called the MongoDB Performance Analyzer (MPA) that can be used for benchmarking queries.
Example 1: Using the MongoDB Performance Analyzer
The MongoDB Performance Analyzer (MPA) is a useful tool that can be used to benchmark MongoDB query performance. It allows you to define a set of queries and run them against your MongoDB database, providing detailed performance metrics for each query. To use MPA, you need to install it as a dependency:
npm install -g mongodb-perf-analyzer
Once installed, you can define your benchmark queries in a JSON file, specifying the collection, query, and any query options. Here's an example of a benchmark query file:
[ { "collection": "products", "query": { "price": { "$gt": 50 } }, "options": {} }, { "collection": "orders", "query": { "customer_id": "abc123" }, "options": {} } ]
You can then run the benchmark using the following command:
mpa benchmark -f benchmark-queries.json
MPA will execute each query in the benchmark file and provide detailed performance metrics, including execution time, latency, and throughput. By analyzing these metrics, you can identify potential areas for optimization and improve the overall performance of your MongoDB queries.
Example 2: Measuring Query Performance
In addition to using MPA, you can also measure the performance of individual queries using the explain() method. This provides detailed information about the query execution plan, including the execution time. Let's consider an example where we want to find all the products with a price greater than $50:
const startTime = new Date(); db.products.find({ price: { $gt: 50 } }).explain(); const endTime = new Date(); const executionTime = endTime - startTime; print("Execution time: " + executionTime + "ms");
Benchmarking MongoDB query performance is an important step in optimizing the efficiency of your queries. By using tools like the MongoDB Performance Analyzer (MPA) and measuring the execution time of individual queries, you can identify bottlenecks, optimize query performance, and improve the overall efficiency of your MongoDB queries.
Exploring MongoDB Query Indexing
Indexing is a useful technique that can significantly improve the performance of MongoDB queries. In this section, we will explore MongoDB query indexing and provide examples of code snippets to demonstrate the process.
MongoDB uses indexes to efficiently locate documents that match a query condition. Indexes are created on specific fields and allow MongoDB to quickly locate the documents that match the query condition, resulting in faster query execution time. By creating appropriate indexes, you can significantly improve the performance of your MongoDB queries.
Related Article: Supabase vs MongoDB: A Feature-by-Feature Comparison
Example 1: Creating Indexes
To create an index in MongoDB, you can use the createIndex() method. This method takes a parameter that specifies the fields to be indexed. Let's consider a scenario where we have a collection called "products" and we frequently query products based on their price. We can create an index on the "price" field using the following code snippet:
db.products.createIndex({ price: 1 })
Example 2: Analyzing Query Performance
Once you have created indexes on the relevant fields, you can analyze the performance of your queries using the explain() method. This provides detailed information about the query execution plan, including the index usage. Let's consider an example where we want to find all the products with a price greater than $50:
db.products.find({ price: { $gt: 50 } }).explain()
The explain() method will return a document that includes details such as the number of documents scanned, the number of documents returned, and the index usage. By analyzing this information, you can determine if the index is being utilized effectively and identify potential areas for optimization.
Exploring MongoDB query indexing is crucial for optimizing query performance. By creating appropriate indexes and analyzing query performance, you can significantly improve the execution time of your queries and enhance the overall efficiency of your MongoDB queries.
Implementing MongoDB Query Caching
Caching is a technique that can improve the performance of MongoDB queries by storing the results of frequently executed queries in memory. In this section, we will discuss how to implement MongoDB query caching and provide examples of code snippets to demonstrate the process.
MongoDB does not provide built-in query caching functionality. However, you can implement query caching in your application by using external caching systems such as Redis or Memcached. These caching systems can store the results of MongoDB queries in memory, allowing for faster retrieval and reducing the load on the MongoDB server.
Example 1: Implementing Query Caching with Redis
To implement query caching with Redis, you can use the Redis client library for your programming language of choice. Let's consider a scenario where we have a Node.js application that queries a collection called "products" frequently. We can implement query caching with Redis using the following code snippet:
const redis = require("redis"); const client = redis.createClient(); function getProductByPrice(price) { return new Promise((resolve, reject) => { const cacheKey = `product:${price}`; client.get(cacheKey, (error, result) => { if (error) { reject(error); } else if (result !== null) { resolve(JSON.parse(result)); } else { db.products.find({ price: price }).toArray((error, products) => { if (error) { reject(error); } else { client.set(cacheKey, JSON.stringify(products)); resolve(products); } }); } }); }); }
In this code snippet, we first check if the query result is stored in the Redis cache. If it is, we return the cached result. Otherwise, we execute the query against MongoDB, store the result in the cache, and return the result.
Related Article: How to Query MongoDB by Time
Example 2: Implementing Query Caching with Memcached
To implement query caching with Memcached, you can use the Memcached client library for your programming language of choice. Let's consider a scenario where we have a Java application that queries a collection called "products" frequently. We can implement query caching with Memcached using the following code snippet:
import net.spy.memcached.MemcachedClient; public class ProductRepository { private final MemcachedClient memcachedClient; public ProductRepository(MemcachedClient memcachedClient) { this.memcachedClient = memcachedClient; } public List<Product> getProductsByPrice(double price) { String cacheKey = "product:" + price; List<Product> products = memcachedClient.get(cacheKey); if (products != null) { return products; } products = db.products.find({ price: price }).toArray(); memcachedClient.set(cacheKey, products); return products; } }
In this code snippet, we first check if the query result is stored in the Memcached cache. If it is, we return the cached result. Otherwise, we execute the query against MongoDB, store the result in the cache, and return the result.
Implementing query caching with external caching systems such as Redis or Memcached can significantly improve the performance of MongoDB queries. By storing the results of frequently executed queries in memory, you can reduce the load on the MongoDB server and provide faster response times for your application.