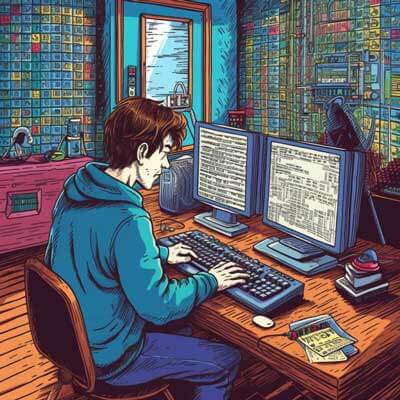
- The Index -1
- Exploring the $hour Function
- Using the Average Function
- Troubleshooting the ‘Could Not Be Cloned’ Error
- Performing Distinct Aggregation
- Exporting MongoDB Data to Amazon S3
- Retrieving Column Names in MongoDB
- Achieving High Availability
- Creating Materialized Views
- Handling the ‘NotWritablePrimary’ Error
- Replacing All Occurrences in MongoDB
- Removing Duplicate Records in MongoDB Query
- Renaming a Database in MongoDB
- Checking if Field Exists with Aggregation in MongoDB
- Grouping Nested Objects with Aggregation in MongoDB
- Sorting Aggregated Data in MongoDB
The Index -1
In MongoDB, indexes play a crucial role in improving query performance by allowing the database to quickly locate and retrieve the data requested by a query. One commonly used index option is the “-1” option, which indicates a descending index.
A descending index is useful when you frequently perform queries that require sorting in descending order or when you need to optimize range queries. By creating a descending index on a field, MongoDB can efficiently scan and retrieve the matching documents in descending order, reducing the sorting time required during query execution.
To create a descending index in MongoDB, you can use the createIndex()
method and specify the field along with the “-1” option:
db.collection.createIndex({ field: -1 })
Here’s an example that demonstrates how to create and use a descending index in MongoDB:
// Create an index on the "score" field in descending order db.students.createIndex({ score: -1 }) // Query documents sorted by score in descending order db.students.find().sort({ score: -1 })
For more information on MongoDB indexes and how they can be used effectively, refer to the official documentation: https://docs.mongodb.com/manual/indexes/
Related Article: Tutorial: Using Python to Interact with MongoDB Collections
Exploring the $hour Function
In MongoDB, the $hour
operator is part of the aggregation framework and allows you to extract the hour component from a date or timestamp field. This can be useful when analyzing data based on specific time intervals or when performing time-based aggregations.
The $hour
operator can be used within the $project
stage of an aggregation pipeline to extract the hour component. Here’s an example that demonstrates how to use the $hour
operator:
db.collection.aggregate([ { $project: { hour: { $hour: "$timestamp" } } } ])
In this example, the $hour
operator is applied to the “timestamp” field, extracting the hour component and assigning it to a new field called “hour”.
It’s important to note that the $hour
operator returns values in the range of 0-23, representing hours from midnight to 11 PM. If your timestamp field is in a different timezone or you need to adjust for daylight saving time, consider using additional operators or functions to handle the timezone conversions.
For more information on MongoDB’s aggregation framework and available operators, refer to the official documentation: https://docs.mongodb.com/manual/aggregation/
Using the Average Function
In MongoDB, the aggregate()
method provides useful aggregation capabilities for performing calculations and transformations on your data. One commonly used aggregation function is average
, which calculates the average value of a numeric field across multiple documents.
To calculate the average using MongoDB’s aggregation framework, you can use the $group
stage along with the $avg
operator:
db.collection.aggregate([ { $group: { _id: null, averageField: { $avg: "$numericField" } } } ])
In this example, we’re using $group
to group all documents into a single group (specified as _id: null
) and calculate the average of the “numericField” across all documents. The result is stored in a new field called “averageField”.
You can also calculate the average within each group by specifying a different _id
value in the $group
stage. For example, if you want to calculate the average based on a category field, you can modify the pipeline as follows:
db.collection.aggregate([ { $group: { _id: "$category", averageField: { $avg: "$numericField" } } } ])
In this case, MongoDB will group documents based on their “category” field and calculate the average of “numericField” within each category.
For more information on MongoDB’s aggregation framework and available functions, refer to the official documentation: https://docs.mongodb.com/manual/aggregation/
Troubleshooting the ‘Could Not Be Cloned’ Error
The ‘Could Not Be Cloned’ error in MongoDB typically occurs when attempting to clone or copy a database that is actively being written to or has open transactions. This error indicates that MongoDB cannot create a consistent copy of the database due to ongoing write operations.
To troubleshoot and resolve this error, follow these steps:
1. Check for ongoing write operations or open transactions: Use the db.currentOp()
command to view all current operations in MongoDB. Look for any active write operations or long-running transactions that might be preventing database cloning.
2. Stop ongoing write operations or close transactions: If you identify any active write operations or open transactions, try to stop or complete them before attempting to clone the database again. Use the appropriate commands or operations to stop or close transactions based on your application’s requirements.
3. Check storage availability: Ensure that you have enough disk space available on your MongoDB server to accommodate the cloned database. Insufficient disk space can also cause the ‘Could Not Be Cloned’ error.
4. Restart MongoDB: If the above steps do not resolve the issue, try restarting your MongoDB server. This can help clear any internal state that might be causing conflicts during database cloning.
5. Perform a repair operation: If none of the above steps work, you can try running a repair operation on the source database before attempting to clone it. The repair operation can help resolve any inconsistencies or corruption that might be preventing successful cloning.
It’s important to note that cloning a live production database should be done with caution and during periods of low activity to minimize potential data inconsistencies or disruptions.
For more information on troubleshooting MongoDB errors and performing database operations, refer to the official documentation: https://docs.mongodb.com/manual/tutorial/
Related Article: Exploring MongoDB: Does it Load Documents When Querying?
Performing Distinct Aggregation
You can perform distinct aggregations to retrieve unique values from a specific field in a collection. This is useful when you want to obtain a list of distinct values for further analysis or reporting purposes.
To perform a distinct aggregation in MongoDB, you can use the $group
stage along with the $addToSet
operator:
db.collection.aggregate([ { $group: { _id: "$field", distinctValues: { $addToSet: "$field" } } } ])
In this example, we’re using $group
to group documents based on their “field” value and create an array of distinct values using $addToSet
. The result is stored in a new field called “distinctValues”.
You can also combine multiple fields in the $group
stage to create unique combinations of values. For example:
db.collection.aggregate([ { $group: { _id: { field1: "$field1", field2: "$field2" }, distinctValues: { $addToSet: { field1: "$field1", field2: "$field2" } } } } ])
In this case, MongoDB will group documents based on the combination of “field1” and “field2”, creating an array of distinct combinations.
For more information on MongoDB’s aggregation framework and available operators, refer to the official documentation: https://docs.mongodb.com/manual/aggregation/
Exporting MongoDB Data to Amazon S3
Dumping MongoDB data to Amazon S3 allows you to create backups or transfer your data to an external storage system. By leveraging the capabilities of Amazon S3, you can benefit from its durability, scalability, and cost-effectiveness for storing your MongoDB backups.
To dump MongoDB data to Amazon S3, follow these steps:
1. Install the AWS Command Line Interface (CLI): If you haven’t already, install the AWS CLI on your machine. You can download it from the official AWS website and follow the installation instructions provided.
2. Configure AWS CLI credentials: Open a terminal or command prompt and run the aws configure
command. Enter your AWS access key ID, secret access key, default region name, and output format when prompted. This will authenticate your machine with your AWS account.
3. Dump MongoDB data to a local directory: Use the mongodump
command to create a backup of your MongoDB data. Specify the desired options such as the host, port, authentication credentials, and the output directory where the backup will be stored.
Example command:
mongodump --host <hostname> --port <port> --username <username> --password <password> --out <output_directory>
4. Sync the local backup directory with Amazon S3: After successfully creating a local backup, you can use the AWS CLI’s aws s3 sync
command to synchronize the contents of your local backup directory with an Amazon S3 bucket.
Example command:
aws s3 sync <local_directory> s3://<bucket_name>
5. Verify the data in Amazon S3: Access your Amazon S3 bucket through the AWS Management Console or programmatically to verify that the MongoDB data has been successfully uploaded.
Dumping MongoDB data to Amazon S3 provides an easy and efficient way to create backups or transfer your data to cloud storage. Remember to schedule regular backups and ensure that your backups are stored securely in accordance with your organization’s data protection policies.
For more information on using Amazon S3 with MongoDB and AWS CLI commands, refer to the official documentation:
– AWS CLI: https://docs.aws.amazon.com/cli/latest/userguide/install-cliv2.html
– MongoDB Backup with AWS S3: https://docs.mongodb.com/database-tools/mongodump/#backup-with-aws-s3
Retrieving Column Names in MongoDB
In MongoDB, collections are schemaless, which means that each document within a collection can have its own set of fields. Unlike traditional relational databases, MongoDB does not have predefined columns or a fixed schema.
However, if you need to retrieve the names of the fields (or “columns”) present in your MongoDB collection, you can use the distinct()
function in conjunction with the $objectToArray
operator:
db.collection.distinct("_id").map(doc => Object.keys(doc))
In this example, we’re using distinct("_id")
to obtain a list of all unique _id
values from the collection. Then, we use .map()
and Object.keys()
to extract the field names from each document.
Note that this approach relies on iterating through all documents in the collection, so it may not be suitable for large collections with millions of documents. In such cases, it’s recommended to utilize indexes and other performance optimization techniques to efficiently retrieve field names.
It’s important to remember that MongoDB’s flexible schema allows for dynamic changes to your data structure over time. As a result, different documents within the same collection can have different fields or variations in field names. Therefore, when retrieving column names in MongoDB, it’s essential to consider the dynamic nature of the data and handle potential variations.
For more information on working with collections and documents in MongoDB, refer to the official documentation: https://docs.mongodb.com/manual/core/collections/
Related Article: How to Add a Field with a Blank Value in MongoDB
Achieving High Availability
High availability is a critical requirement for any production database system. In MongoDB, you can achieve high availability by setting up a replica set—a group of MongoDB instances that maintain identical copies of your data.
To achieve high availability in MongoDB using replica sets, follow these steps:
1. Set up multiple MongoDB instances: Install and configure multiple MongoDB instances on separate machines or virtual servers. These instances will form the replica set.
2. Initialize the replica set: Connect to one of the MongoDB instances and run the rs.initiate()
command to initialize the replica set.
3. Add additional members to the replica set: Use the rs.add()
command to add more MongoDB instances as members of the replica set. Each member should have a unique hostname and port number.
4. Configure replica set options: Set appropriate configuration options for your replica set, such as choosing a primary, adjusting read preferences, enabling authentication, and defining voting configurations.
5. Monitor and maintain your replica set: Regularly monitor the health and status of your replica set using built-in tools like rs.status()
. Perform routine maintenance tasks such as backups, index optimization, and software upgrades.
For more information on setting up and managing replica sets in MongoDB, refer to the official documentation: https://docs.mongodb.com/manual/replication/
Creating Materialized Views
Materialized views in MongoDB provide a way to precompute and store the results of complex queries or aggregations. By creating materialized views, you can improve query performance and reduce the need for repeated calculations on large datasets.
To create a materialized view in MongoDB, follow these steps:
1. Define an aggregation pipeline: Identify the complex query or aggregation that you want to materialize into a view. This could involve multiple stages like filtering documents, grouping data, or performing calculations.
2. Create a new collection for the view: Use the db.createView()
method to specify the name of the view collection and the aggregation pipeline that defines its content.
Example command:
db.createView("viewCollection", "sourceCollection", [ { $match: { field: "value" } }, { $group: { _id: "$category", totalAmount: { $sum: "$amount" } } } ])
In this example, we’re creating a materialized view named “viewCollection” based on the “sourceCollection”. The aggregation pipeline filters documents based on a specific field value and then groups them by category while calculating the total amount.
3. Query the materialized view: Once the materialized view is created, you can query it like any other collection in MongoDB. The results will be returned much faster since they are precomputed and stored.
Materialized views can significantly improve query performance for complex operations. However, keep in mind that materialized views require additional storage space and need to be updated periodically to reflect changes in the source data. You can use triggers or scheduled jobs to refresh or rebuild materialized views as needed.
For more information on creating and managing materialized views in MongoDB, refer to the official documentation: https://docs.mongodb.com/manual/core/materialized-views/
Handling the ‘NotWritablePrimary’ Error
The ‘NotWritablePrimary’ error in MongoDB occurs when performing write operations on a secondary node of a replica set. By design, secondary nodes are read-only and cannot accept write operations directly.
To handle the ‘NotWritablePrimary’ error in MongoDB, follow these steps:
1. Identify your primary node: Connect to one of your replica set members using a MongoDB client and run the rs.isMaster()
command. Look for the ismaster
field to determine if the connected node is the primary.
2. Connect to the primary node: If you’re connected to a secondary node, switch your connection to the primary node of your replica set. You can find its hostname and port from the output of the rs.isMaster()
command.
3. Perform write operations on the primary: Once connected to the primary node, you can execute write operations like insert, update, or delete on your MongoDB collections without encountering the ‘NotWritablePrimary’ error.
It’s important to note that MongoDB provides read scaling by allowing read operations on secondary nodes. However, write operations should always be performed on the primary node to maintain data consistency and prevent conflicts.
For more information on replica sets and understanding MongoDB’s read and write behavior, refer to the official documentation: https://docs.mongodb.com/manual/replication/
Related Article: How to Use Range Queries in MongoDB
Replacing All Occurrences in MongoDB
In MongoDB, you can use update operations with specific modifiers to replace all occurrences of a value within an array or a field in multiple documents. This can be useful when you need to perform bulk updates or search-and-replace operations across your data.
To replace all occurrences in MongoDB, follow these steps:
1. Identify documents for replacement: Use a query operation like find()
or findOne()
to identify the documents that need replacement based on specific criteria.
2. Use $set
with $replaceAll
modifier: In an update operation, use $set
along with $replaceAll
modifier to replace all occurrences of a value within an array or field.
Example command:
db.collection.updateMany( { field: "value" }, { $set: { field: { $replaceAll: { input: "$field", find: "oldValue", replacement: "newValue" } } } } )
In this example, we’re using updateMany()
to update all documents where the “field” value is equal to “value”. The $set
operator with $replaceAll
modifier replaces all occurrences of “oldValue” with “newValue” within the “field”.
3. Verify the replacements: After executing the update operation, you can verify that the replacements were successful by inspecting the updated documents.
Replacing all occurrences in MongoDB provides an efficient way to update multiple documents at once without looping through each document individually. It’s important to carefully review and test your update operation before applying it to your production data.
For more information on updating documents and using modifiers in MongoDB, refer to the official documentation: https://docs.mongodb.com/manual/tutorial/update-documents/
Removing Duplicate Records in MongoDB Query
Removing duplicate records from a MongoDB query involves identifying duplicate criteria and using aggregation pipeline stages like $group
and $project
to filter out duplicate documents.
To remove duplicate records in a MongoDB query, follow these steps:
1. Identify duplicate criteria: Determine the fields or combination of fields that define duplicate records in your collection. This could be based on specific attributes or a unique identifier.
2. Use $group
stage for grouping: In an aggregation pipeline, use the $group
stage to group documents based on your duplicate criteria.
Example pipeline:
db.collection.aggregate([ { $group: { _id: { field1: "$field1", field2: "$field2" }, count: { $sum: 1 } } }, { $match: { count: { $gt : 1 } } } ])
In this example, we’re using $group
to group documents based on the combination of “field1” and “field2”. The $sum
operator is used to count the number of occurrences for each group. We then use $match
to filter groups with a count greater than 1, indicating duplicate records.
3. Use $project
stage for filtering: After identifying the duplicate groups, you can use the $project
stage to filter out unwanted documents and retain only the first occurrence or specific duplicates.
Example pipeline:
db.collection.aggregate([ { $group: { _id: { field1: "$field1", field2: "$field2" }, count: { $sum: 1 }, docs: { $push: "$$ROOT" } } }, { $match: { count: { $gt : 1 } } }, { $project: { firstOccurrence: { $arrayElemAt: [ "$docs", 0 ] } } } ])
In this example, we’re using $project
to extract only the first occurrence of each duplicate group by using the $arrayElemAt
operator and specifying an index of 0.
For more information on using aggregation pipelines in MongoDB, refer to the official documentation: https://docs.mongodb.com/manual/core/aggregation-pipeline/
Renaming a Database in MongoDB
Renaming a database in MongoDB involves creating a new database with the desired name and copying or moving data from the old database to the new one. MongoDB does not provide a built-in command to directly rename a database.
To rename a database in MongoDB, follow these steps:
1. Create a new database: Use the db.copyDatabase()
command or equivalent functionality in your MongoDB client to create a new database with the desired name.
Example command:
db.copyDatabase("oldDB", "newDB")
In this example, we’re using db.copyDatabase()
to create a new database named “newDB” based on an existing database named “oldDB”.
2. Copy or move data: Depending on your requirements, you can choose to copy or move data from the old database to the new one. This can be done using the db.collection.find()
and db.collection.insert()
methods or by exporting and importing data with tools like mongodump
and mongorestore
.
3. Verify data migration: After copying or moving the data, verify that it has been successfully migrated to the new database. Perform extensive testing and validation to ensure data integrity.
4. Update application configurations: Update any application configurations or connection strings that reference the old database name to use the new name instead.
5. Remove or archive old database: Once you have confirmed that all data has been migrated successfully, you can choose to remove or archive the old database based on your organization’s retention policies.
Renaming a database in MongoDB requires careful planning and execution to ensure data consistency and integrity throughout the process. It’s recommended to perform this operation during low activity periods and have proper backups available in case of any issues.
For more information on managing databases in MongoDB, refer to the official documentation: https://docs.mongodb.com/manual/administration/
Related Article: Crafting Query Operators in MongoDB
Checking if Field Exists with Aggregation in MongoDB
You can use aggregation pipelines to check if a field exists in documents within a collection. By utilizing the $project
, $match
, and $exists
operators, you can filter documents based on the presence or absence of a specific field.
To check if a field exists with aggregation in MongoDB, follow these steps:
1. Use $project
to include or exclude fields: In the initial stage of your aggregation pipeline, use $project
to include or exclude the field you want to check for existence.
Example pipeline stage:
{ $project: { hasField: { $cond: [{ $gt: [{ $type: "$field" }, "missing"] }, true, false] } } }
In this example, we’re using $project
to create a new field called “hasField” that evaluates whether the “field” exists or is missing. The $cond
operator is used in combination with $type
and comparison operators to determine the existence.
2. Use $match
to filter documents based on field existence: After creating the “hasField” field, you can use subsequent stages like $match
to filter documents based on its value.
Example pipeline stage:
{ $match: { hasField: true } }
In this example, we’re using $match
to filter out documents where “hasField” is true, indicating that the “field” exists.
For more information on using aggregation pipelines and operators in MongoDB, refer to the official documentation: https://docs.mongodb.com/manual/aggregation/
Grouping Nested Objects with Aggregation in MongoDB
In MongoDB, you can use the aggregation framework to group nested objects within documents based on specific criteria. By utilizing the $group
stage and dot notation, you can perform complex aggregations on nested fields and arrays.
To group nested objects with aggregation in MongoDB, follow these steps:
1. Use $unwind
to flatten nested arrays: If your nested objects are stored within arrays, use the $unwind
stage to expand the array elements into separate documents.
Example pipeline stage:
{ $unwind: "$nestedArrayField" }
In this example, we’re using $unwind
to flatten the “nestedArrayField” array.
2. Use dot notation for grouping: In the subsequent $group
stage, use dot notation to specify the path to the nested object or field you want to group by.
Example pipeline stage:
{ $group: { _id: "$nestedObjectField.nestedField", count: { $sum: 1 } } }
In this example, we’re using dot notation ($nestedObjectField.nestedField
) to access a field within a nested object and grouping documents by its value. The $sum
operator is used to calculate the count of occurrences.
For more information on using aggregation pipelines and operators in MongoDB, refer to the official documentation: https://docs.mongodb.com/manual/aggregation/
Sorting Aggregated Data in MongoDB
In MongoDB, you can sort aggregated data based on specific fields or criteria using the $sort
operator within an aggregation pipeline. By specifying a sort order and field(s), you can control how the aggregated data is presented in the final result.
To sort aggregated data in MongoDB, follow these steps:
1. Use $sort
within the $group
stage: Place the $sort
operator within the $group
stage of your aggregation pipeline to sort the grouped data based on specific fields or criteria.
Example pipeline stage:
{ $group: { _id: "$category", totalAmount: { $sum: "$amount" } } }, { $sort: { totalAmount: -1 } }
In this example, we’re using $group
to group documents by their “category” field and calculate the sum of their “amount” field. We then use $sort
to sort the grouped data in descending order based on the “totalAmount” field.
2. Use additional sorting stages if necessary: You can add multiple $sort
stages within your aggregation pipeline to perform more complex sorting operations based on different fields or criteria.
Example pipeline stage:
{ $group: { _id: "$category", totalAmount: { $sum: "$amount" }, averageRating: { $avg: "$rating" } } }, { $sort: { totalAmount: -1, averageRating: -1 } }
In this example, we’re sorting the grouped data first by “totalAmount” in descending order and then by “averageRating” in descending order.
For more information on using aggregation pipelines and operators in MongoDB, refer to the official documentation: https://docs.mongodb.com/manual/aggregation/