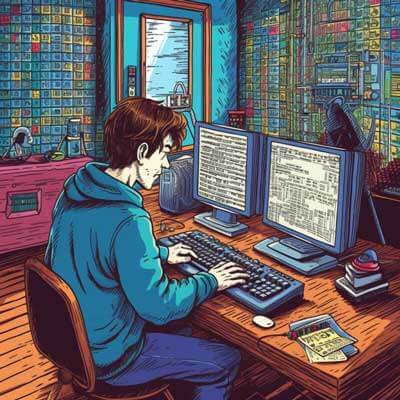
Table of Contents
Finding the Maximum Value in a MongoDB Query
The maximum value in a query can be found by using the $max
operator within an aggregation pipeline. The $max
operator returns the maximum value of the specified field across all the documents in the collection.
Here's an example of how to use the $max
operator:
db.collection.aggregate([ { $group: { _id: null, maxField: { $max: "$field" } } } ])
In this example, collection
is the name of the collection you are querying, and field
is the field you want to find the maximum value of. The $group
stage groups all the documents into a single group (with _id: null
), and the $max
operator calculates the maximum value of the specified field.
You can also specify additional fields to group by, if needed. For example, if you want to find the maximum value of a field for each category in a collection of products, you can modify the query as follows:
db.products.aggregate([ { $group: { _id: "$category", maxField: { $max: "$field" } } } ])
This will return the maximum value of the field for each category in the collection.
Related Article: How to Use Range Queries in MongoDB
Sorting Results in a MongoDB Query
When querying data in MongoDB, it is often necessary to sort the results based on a specific field or fields. Sorting allows you to arrange the documents in a specified order, such as ascending or descending. The sort()
method in MongoDB is used to perform this operation.
To sort the results in ascending order, you can use the following syntax:
db.collection.find().sort({ field: 1 })
Where collection
is the name of the collection you are querying and field
is the field you want to sort by. The value 1
indicates ascending order. For example, if you have a collection of books and you want to sort them by the publication year in ascending order, you can use the following query:
db.books.find().sort({ year: 1 })
To sort the results in descending order, you can use the value -1
instead:
db.collection.find().sort({ field: -1 })
Continuing with the previous example, if you want to sort the books by the publication year in descending order, you can use the following query:
db.books.find().sort({ year: -1 })
It's important to note that the sort()
method can also accept multiple fields for sorting. In this case, the documents will be sorted based on the first field specified, and if there are any ties, they will be sorted based on the second field, and so on.
Limiting the Number of Results in a MongoDB Query
In some cases, you may only be interested in retrieving a certain number of documents from a MongoDB query. The limit()
method allows you to specify the maximum number of documents to be returned.
The syntax for using the limit()
method is as follows:
db.collection.find().limit(number)
Where collection
is the name of the collection you are querying and number
is the maximum number of documents you want to retrieve.
For example, if you want to retrieve the first 5 books from a collection, you can use the following query:
db.books.find().limit(5)
This will return the first 5 documents in the collection.
It's important to note that the limit()
method should be used after any sorting or filtering operations, as it limits the number of documents returned by the query.
Using the $sort Operator in a MongoDB Query
In MongoDB, the $sort
operator is used to sort the documents within a pipeline. It is similar to the sort()
method used in regular queries, but it allows for more flexibility when combined with other aggregation stages.
Here's an example of how to use the $sort
operator:
db.collection.aggregate([ { $match: { field: { $gte: 0 } } }, { $sort: { field: -1 } } ])
In this example, collection
is the name of the collection you are querying, and field
is the field you want to sort by. The $match
stage is used to filter the documents based on a condition (in this case, field
should be greater than or equal to 0), and the $sort
stage is used to sort the filtered documents in descending order based on the field
value.
It's important to note that the $sort
stage should be placed after any $match
or other stages that filter or transform the documents, as it operates on the documents that have passed through the preceding stages.
Related Article: Exploring MongoDB: Does it Load Documents When Querying?
Using the $limit Operator in a MongoDB Query
In MongoDB, the $limit
operator is used to limit the number of documents returned by a pipeline. It is similar to the limit()
method used in regular queries, but it allows for more control when combined with other aggregation stages.
Here's an example of how to use the $limit
operator:
db.collection.aggregate([ { $sort: { field: -1 } }, { $limit: 10 } ])
In this example, collection
is the name of the collection you are querying, and field
is the field you want to sort by. The $sort
stage is used to sort the documents in descending order based on the field
value, and the $limit
stage is used to limit the result set to 10 documents.
It's important to note that the $limit
stage should be placed after any $sort
or other stages that modify the order or number of documents in the pipeline.
Performing Aggregation in MongoDB
Aggregation in MongoDB allows you to process and analyze data in a collection and return computed results. It is a useful feature that enables you to perform complex data transformations and calculations.
Aggregation pipelines in MongoDB consist of stages, each of which performs a specific operation on the input documents and passes the results to the next stage. Some common aggregation stages include $match
, $group
, $sort
, $limit
, and $project
.
Here's an example of how to perform aggregation in MongoDB:
db.collection.aggregate([ { $match: { field: { $gte: 0 } } }, { $group: { _id: "$category", count: { $sum: 1 } } }, { $sort: { count: -1 } }, { $limit: 5 } ])
In this example, collection
is the name of the collection you are querying, and field
is the field you want to filter by. The pipeline consists of four stages:
1. The $match
stage filters the documents based on a condition (in this case, field
should be greater than or equal to 0).
2. The $group
stage groups the documents by the category
field and calculates the count of documents in each group.
3. The $sort
stage sorts the groups in descending order based on the count.
4. The $limit
stage limits the result set to the top 5 groups.
Understanding the Difference Between find() and aggregate() in MongoDB
In MongoDB, the find()
method and the aggregate()
method are two different ways to query data, each with its own benefits and use cases.
The find()
method is used to query a collection and retrieve documents that match a specified set of criteria. It is straightforward to use and suitable for simple queries that don't require complex data transformations or calculations. The find()
method supports filtering, sorting, and limiting results, as well as various query operators.
Here's an example of how to use the find()
method:
db.collection.find({ field: { $gte: 0 } }).sort({ field: -1 }).limit(10)
In this example, collection
is the name of the collection you are querying, and field
is the field you want to filter by. The query filters the documents based on a condition (in this case, field
should be greater than or equal to 0), sorts the filtered documents in descending order based on the field
value, and limits the result set to 10 documents.
On the other hand, the aggregate()
method is used to perform complex data transformations and calculations on a collection. It allows you to use aggregation pipelines, which consist of multiple stages that process the input documents and produce the final result. Aggregation pipelines can include stages such as filtering, grouping, sorting, limiting, and more.
Here's an example of how to use the aggregate()
method:
db.collection.aggregate([ { $match: { field: { $gte: 0 } } }, { $group: { _id: "$category", count: { $sum: 1 } } }, { $sort: { count: -1 } }, { $limit: 5 } ])
In this example, collection
is the name of the collection you are querying, and the pipeline consists of four stages that filter, group, sort, and limit the documents based on various criteria.
The choice between using find()
and aggregate()
depends on the complexity of your query and the specific data transformations or calculations you need to perform. If your query involves simple filtering, sorting, and limiting, the find()
method is usually sufficient. However, if you need to perform more complex operations or aggregate data across multiple documents, the aggregate()
method provides more flexibility and power.
Finding the Greatest Value in a MongoDB Query
To find the greatest value in a MongoDB query, you can combine the $max
operator with the $group
stage in an aggregation pipeline. The $max
operator retrieves the maximum value of a specified field, and the $group
stage groups the documents and calculates the maximum value within each group.
Here's an example of how to find the greatest value in a MongoDB query:
db.collection.aggregate([ { $group: { _id: null, maxField: { $max: "$field" } } } ])
In this example, collection
is the name of the collection you are querying, and field
is the field you want to find the greatest value of. The $group
stage groups all the documents into a single group (with _id: null
), and the $max
operator calculates the maximum value of the specified field.
If you want to find the greatest value within each category in a collection, you can modify the query as follows:
db.collection.aggregate([ { $group: { _id: "$category", maxField: { $max: "$field" } } } ])
This will return the greatest value of the field within each category in the collection.
Related Article: Crafting Query Operators in MongoDB
Finding the Highest Value in a MongoDB Query
To find the highest value in a MongoDB query, you can combine the $sort
and $limit
stages in an aggregation pipeline. The $sort
stage sorts the documents in descending order based on a specified field, and the $limit
stage limits the result set to a specified number of documents.
Here's an example of how to find the highest value in a MongoDB query:
db.collection.aggregate([ { $sort: { field: -1 } }, { $limit: 1 } ])
In this example, collection
is the name of the collection you are querying, and field
is the field you want to find the highest value of. The $sort
stage sorts the documents in descending order based on the field
value, and the $limit
stage limits the result set to 1 document.
If you want to find the highest value within each category in a collection, you can modify the query as follows:
db.collection.aggregate([ { $sort: { category: 1, field: -1 } }, { $group: { _id: "$category", highestField: { $first: "$field" } } } ])
This will sort the documents first by the category
field in ascending order, and then by the field
field in descending order. The $group
stage groups the sorted documents by category
and retrieves the highest field
value within each group using the $first
operator.