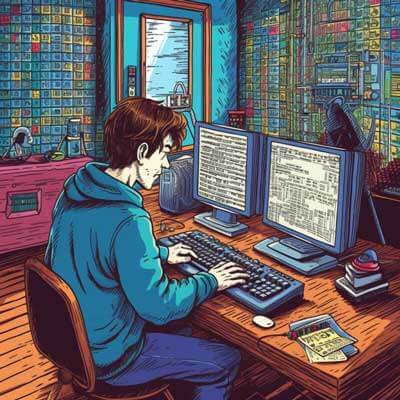
Table of Contents
Syntax for using the $ne operator in MongoDB
The $ne
operator in MongoDB is used to query for documents where a specific field is not equal to a specified value. It stands for "not equal" and is part of the query language in MongoDB. The syntax for using the $ne
operator is as follows:
{ field: { $ne: value } }
In this syntax, 'field' represents the name of the field you want to check for inequality, and 'value' represents the value that you want to compare against. The '$ne' operator is used as a key in a JSON object and its value is set to the desired comparison value.
Let's take a look at an example to see how this syntax works in practice.
Related Article: Using Multi-Indexes with MongoDB Queries
Example of using the $ne operator in a query
Suppose we have a collection called 'users' which contains documents representing different users. Each document has a 'name' field. We want to find all the users whose name is not equal to 'John'. We can use the $ne
operator to achieve this:
db.users.find({ name: { $ne: 'John' } })
This query will return all the documents where the 'name' field is not equal to 'John'. The result will include all the users with names like 'Alice', 'Bob', 'Sarah', etc.
Checking inequality in a MongoDB query
The $ne
operator allows us to check for inequality in a MongoDB query. It is particularly useful when we want to filter documents based on a field that is not equal to a specific value. By using the '$ne' operator, we can easily retrieve documents that do not match a certain value or condition.
Let's say we have a collection called 'products' which contains documents representing different products. Each document has a 'price' field. We want to find all the products that are not priced at $10. We can use the $ne
operator to achieve this:
db.products.find({ price: { $ne: 10 } })
This query will return all the documents where the 'price' field is not equal to 10. The result will include all the products with prices like $5, $7, $15, etc.
Alternative operators for checking inequality in MongoDB
In addition to the '$ne' operator, MongoDB provides other operators that can be used to check for inequality in a query. These operators include:
- $gt
(greater than): Matches values that are greater than a specified value.
- $gte
(greater than or equal to): Matches values that are greater than or equal to a specified value.
- $lt
(less than): Matches values that are less than a specified value.
- $lte
(less than or equal to): Matches values that are less than or equal to a specified value.
- $nin
(not in): Matches values that are not in a specified array of values.
These operators can be used in combination with the '$ne' operator or on their own, depending on the specific requirements of your query. The choice of operator depends on the specific inequality condition you want to check.
Let's take a look at an example that uses the '$gt' operator to find all the products with prices greater than $10:
db.products.find({ price: { $gt: 10 } })
This query will return all the documents where the 'price' field is greater than 10. The result will include all the products with prices like $15, $20, $25, etc.
Related Article: Executing Chained Callbacks in MongoDB Search Queries
Using the $ne operator with other operators
The $ne
operator can be used in combination with other operators in a MongoDB query to create more complex conditions. By combining operators, we can build queries that match documents based on multiple inequality conditions.
Let's say we have a collection called 'users' which contains documents representing different users. Each document has a 'age' field. We want to find all the users who are not between the ages of 18 and 30. We can use the $ne
operator in combination with the $lt
and $gt
operators to achieve this:
db.users.find({ age: { $ne: { $gt: 18, $lt: 30 } } })
This query will return all the documents where the 'age' field is not between 18 and 30. The result will include all the users with ages less than 18 or greater than 30.
Common use cases
The $ne
operator is commonly used in various scenarios where inequality needs to be checked. Some common use cases include:
1. Filtering documents based on a specific value: The $ne
operator allows you to exclude documents that have a specific value for a given field. For example, you can use it to find all the users who are not from a specific country.
db.users.find({ country: { $ne: 'USA' } })
2. Excluding documents with a null or missing value: The $ne
operator can be used to exclude documents that have a null or missing value for a given field. This can be useful when you want to filter out incomplete or invalid data.
db.users.find({ address: { $ne: null } })
3. Comparing against multiple values: The $ne
operator can also be used to compare a field against multiple values. This can be achieved by using the '$nin' operator, which stands for "not in". It allows you to specify an array of values to compare against.
db.users.find({ country: { $nin: ['USA', 'Canada'] } })
This query will return all the documents where the 'country' field is neither 'USA' nor 'Canada'.
How to check for not equal values in a MongoDB query
To check for not equal values in a MongoDB query, you can use the $ne
operator as described earlier. The syntax for using the $ne
operator is straightforward:
{ field: { $ne: value } }
In this syntax, 'field' represents the name of the field you want to check for inequality, and 'value' represents the value that you want to compare against. The $ne
operator is used as a key in a JSON object and its value is set to the desired comparison value.
Let's take a look at a more detailed example to see how this works. Suppose we have a collection called 'orders' which contains documents representing different orders. Each document has an 'amount' field. We want to find all the orders that have an amount not equal to $100. We can use the '$ne' operator to achieve this:
db.orders.find({ amount: { $ne: 100 } })
This query will return all the documents where the 'amount' field is not equal to 100. The result will include all the orders with amounts like $50, $75, $150, etc.
Understanding the behavior of the $ne operator
The $ne
operator performs a simple inequality comparison between the specified field and value. It returns documents where the field is not equal to the specified value.
Here are some key points to consider regarding its behavior:
1. Matching Documents: The '$ne' operator matches documents where the field is not equal to the specified value. It does not match documents where the field is missing or null.
2. Comparison Type: The '$ne' operator performs a simple comparison based on the type of the field and the value. It does not perform any type conversion or coercion. This means that the field and the value must be of the same type for an accurate comparison.
3. Index Usage: The '$ne' operator can use indexes efficiently when querying for inequality. However, it may not perform as well as equality queries. If you need to perform frequent inequality queries, it is recommended to create an index on the field you are querying against.
4. Performance Considerations: The performance of queries using the '$ne' operator can be affected by the number of documents in the collection, the size of the documents, and the complexity of the query. It is important to optimize your queries and ensure that your indexes are properly configured for efficient execution.
Related Article: MongoDB Essentials: Aggregation, Indexing and More
Tips and best practices
1. Use Indexes: If you frequently query for inequality using the '$ne' operator, consider creating an index on the field you are querying against. This can significantly improve the performance of your queries.
2. Avoid Large Result Sets: Be cautious when using the '$ne' operator with a large result set. If the number of documents matching the inequality condition is large, it can impact the performance of your queries and consume significant resources. Consider using other operators or additional filters to narrow down the result set.
3. Be Mindful of Type Mismatch: Ensure that the field and the value you are comparing are of the same type. MongoDB performs a strict comparison based on the type of the field and the value. If the types do not match, the query may not return the expected results.
4. Combine Operators for Complex Conditions: The '$ne' operator can be combined with other operators to create more complex conditions. Experiment with different combinations of operators to achieve the desired query behavior.
5. Test and Optimize: As with any query, it is important to test and optimize your queries to ensure optimal performance. Use the MongoDB explain() method to analyze the query execution plan and identify any potential performance issues.
Code snippet: Implementing a 'not equal' query in MongoDB
Here's a code snippet that demonstrates how to implement a 'not equal' query in MongoDB using the '$ne' operator:
// Connect to MongoDB const MongoClient = require('mongodb').MongoClient; const uri = 'mongodb://localhost:27017'; const client = new MongoClient(uri, { useNewUrlParser: true }); // Query for documents where the 'age' field is not equal to 30 client.connect(err => { if (err) throw err; const collection = client.db('mydb').collection('users'); collection.find({ age: { $ne: 30 } }).toArray((err, docs) => { if (err) throw err; console.log(docs); client.close(); }); });
In this code snippet, we first connect to the MongoDB server using the MongoClient class. Then, we retrieve the 'users' collection and use the find() method to query for documents where the 'age' field is not equal to 30. Finally, we use the toArray() method to retrieve the query results as an array and log them to the console.
Advanced features of the $ne operator
1. Using Regular Expressions: The '$ne' operator can be combined with regular expressions to perform pattern matching on string fields. This allows for more flexible querying based on inequality. For example, you can use a regular expression to find all documents where a field does not start with a specific letter.
db.users.find({ name: { $not: /^A/ } })
2. Combining Multiple Fields: The '$ne' operator can be used to compare multiple fields in a single query. This is useful when you want to find documents where multiple fields are not equal to specific values.
db.users.find({ $and: [ { age: { $ne: 30 } }, { country: { $ne: 'USA' } } ] })
3. Negating Conditions: The '$ne' operator can be negated using the '$not' operator. This allows you to find documents that do not match a specific inequality condition.
db.users.find({ age: { $not: { $ne: 30 } } })
Troubleshooting common issues when using the $ne operator
1. Incorrect Syntax: Double-check the syntax of your query to ensure that you are using the '$ne' operator correctly. Make sure that the operator is used as a key in a JSON object and that its value is set to the desired comparison value.
2. Type Mismatch: Ensure that the field and the value you are comparing are of the same type. MongoDB performs a strict comparison based on the type of the field and the value. If the types do not match, the query may not return the expected results.
3. Indexing: If your query using the '$ne' operator is slow, check if you have an index on the field you are querying against. Without an index, MongoDB may need to perform a collection scan, which can impact performance. Consider creating an index to improve query performance.
4. Large Result Sets: Be cautious when using the '$ne' operator with a large result set. If the number of documents matching the inequality condition is large, it can impact the performance of your queries and consume significant resources. Consider using other operators or additional filters to narrow down the result set.
5. Query Optimization: If your query using the '$ne' operator is still slow, consider optimizing your query by using other operators or restructuring your data model. Analyze the query execution plan using the explain() method to identify any potential performance issues.