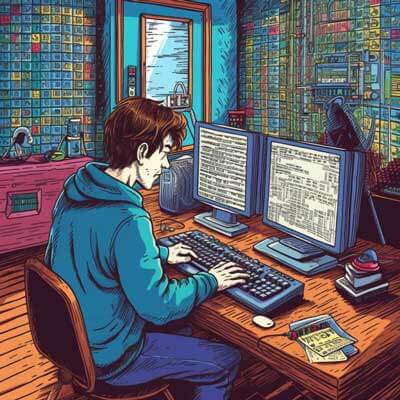
- Introduction to Map Function
- Syntax and Parameters of Map Function
- Working with Single Iterable: Map Function
- Working with Multiple Iterables: Map Function
- Use Case 1: Data Transformation with Map
- Use Case 2: Data Filtering with Map
- Use Case 3: Applying Functions to Elements with Map
- Best Practice 1: Using Lambdas with Map
- Best Practice 2: Handling Empty Iterables with Map
- Real World Example 1: Map Function in Data Analysis
- Real World Example 2: Map Function in Web Scraping
- Performance Consideration 1: Map vs For Loops
- Performance Consideration 2: Map vs List Comprehension
- Advanced Technique 1: Combining Map with Reduce
- Advanced Technique 2: Map Function with Custom Functions
- Code Snippet Idea 1: Mapping Functions to Strings
- Code Snippet Idea 2: Mapping Functions to Lists
- Code Snippet Idea 3: Mapping Functions to Dictionaries
- Code Snippet Idea 4: Mapping Functions to Sets
- Code Snippet Idea 5: Mapping Functions to Tuples
- Error Handling 1: Handling Type Errors with Map
- Error Handling 2: Handling Value Errors with Map
- Error Handling 3: Handling Index Errors with Map
Introduction to Map Function
The map()
function in Python is a built-in function that is used to apply a given function to all the items in an iterable and return an iterator with the results. It takes in two or more arguments: the function to apply and one or more iterables. The function is applied to each corresponding element of the iterables, and the results are returned as an iterator, which can be converted to a list or tuple if desired.
Related Article: How To Limit Floats To Two Decimal Points In Python
Syntax and Parameters of Map Function
The syntax of the map()
function is as follows:
map(function, iterable1, iterable2, ...)
The function
parameter is the function to be applied to the elements of the iterables. It can be a built-in function, a user-defined function, or a lambda function. The iterable1
, iterable2
, etc. parameters are the iterables on which the function will be applied.
Here is an example of using the map()
function:
numbers = [1, 2, 3, 4, 5] squared_numbers = map(lambda x: x**2, numbers) print(list(squared_numbers))
This will output:
[1, 4, 9, 16, 25]
Working with Single Iterable: Map Function
The map()
function can be used with a single iterable. In this case, the function will be applied to each element of the iterable. Here is an example:
names = ["Alice", "Bob", "Charlie"] greetings = map(lambda name: "Hello, " + name, names) print(list(greetings))
This will output:
['Hello, Alice', 'Hello, Bob', 'Hello, Charlie']
Another example is applying a mathematical function to a list of numbers:
numbers = [1, 2, 3, 4, 5] squared_numbers = map(lambda x: x**2, numbers) print(list(squared_numbers))
This will output:
[1, 4, 9, 16, 25]
Working with Multiple Iterables: Map Function
The map()
function can also be used with multiple iterables. In this case, the function should take as many arguments as there are iterables. Here is an example:
numbers = [1, 2, 3, 4, 5] multipliers = [2, 3, 4, 5, 6] products = map(lambda x, y: x * y, numbers, multipliers) print(list(products))
This will output:
[2, 6, 12, 20, 30]
Note that the function takes two arguments, x
and y
, which correspond to the elements of the two iterables, numbers
and multipliers
, respectively.
Related Article: How To Rename A File With Python
Use Case 1: Data Transformation with Map
One common use case of the map()
function is to transform data by applying a function to each element. For example, suppose we have a list of temperatures in Celsius and we want to convert them to Fahrenheit:
celsius_temperatures = [20, 25, 30, 35, 40] fahrenheit_temperatures = map(lambda c: (c * 9/5) + 32, celsius_temperatures) print(list(fahrenheit_temperatures))
This will output:
[68.0, 77.0, 86.0, 95.0, 104.0]
The lambda function in this example takes a Celsius temperature, converts it to Fahrenheit using the formula (c * 9/5) + 32
, and returns the result.
Another example is converting a list of strings to uppercase:
names = ["alice", "bob", "charlie"] uppercased_names = map(str.upper, names) print(list(uppercased_names))
This will output:
['ALICE', 'BOB', 'CHARLIE']
Use Case 2: Data Filtering with Map
The map()
function can also be used to filter data by applying a function that returns either True
or False
. Only the elements for which the function returns True
will be included in the result. Here is an example:
numbers = [1, 2, 3, 4, 5] even_numbers = map(lambda x: x if x % 2 == 0 else None, numbers) print(list(even_numbers))
This will output:
[None, 2, None, 4, None]
In this example, the lambda function returns None
for odd numbers and the number itself for even numbers. The None
values are filtered out, resulting in a list of even numbers.
Another example is filtering out empty strings from a list:
names = ["Alice", "", "Bob", "Charlie", ""] non_empty_names = map(lambda name: name if name else None, names) print(list(non_empty_names))
This will output:
['Alice', None, 'Bob', 'Charlie', None]
Use Case 3: Applying Functions to Elements with Map
The map()
function can be used to apply any function to each element of an iterable. This allows for various types of operations, such as calculating the length of strings in a list:
names = ["Alice", "Bob", "Charlie"] name_lengths = map(len, names) print(list(name_lengths))
This will output:
[5, 3, 7]
In this example, the built-in len()
function is applied to each element of the names
list, resulting in a list of the lengths of the strings.
Another example is converting a list of integers to their absolute values:
numbers = [-1, 2, -3, 4, -5] absolute_values = map(abs, numbers) print(list(absolute_values))
This will output:
[1, 2, 3, 4, 5]
Related Article: How To Check If List Is Empty In Python
Best Practice 1: Using Lambdas with Map
Using lambda functions with the map()
function can be a convenient way to apply simple operations to elements. Lambdas are anonymous functions that can be defined in-place. They are often used in conjunction with the map()
function to provide concise and readable code. Here is an example:
numbers = [1, 2, 3, 4, 5] squared_numbers = map(lambda x: x**2, numbers) print(list(squared_numbers))
This will output:
[1, 4, 9, 16, 25]
In this example, the lambda function takes an input x
and returns its square. The map()
function applies this lambda function to each element of the numbers
list.
Lambdas can also be used for more complex operations. For example, suppose we have a list of numbers and we want to calculate their square roots:
import math numbers = [1, 4, 9, 16, 25] square_roots = map(lambda x: math.sqrt(x), numbers) print(list(square_roots))
This will output:
[1.0, 2.0, 3.0, 4.0, 5.0]
In this example, the lambda function takes an input x
and returns its square root using the math.sqrt()
function.
Best Practice 2: Handling Empty Iterables with Map
When working with the map()
function, it’s important to consider how it behaves when given empty iterables. If any of the iterables are empty, the map()
function will return an empty iterator. This can lead to unexpected behavior, especially if you are expecting a non-empty result. One way to handle this is to use the zip()
function to ensure that all iterables are of the same length. Here is an example:
numbers = [1, 2, 3, 4, 5] empty_iterable = [] result = map(lambda x, y: x + y, numbers, empty_iterable) print(list(result))
This will output:
[]
In this example, the empty_iterable
is empty, so the map()
function returns an empty iterator.
To handle this situation, we can use the zip()
function to ensure that all iterables have the same length:
numbers = [1, 2, 3, 4, 5] empty_iterable = [] result = map(lambda x, y: x + y, zip(numbers, empty_iterable)) print(list(result))
This will output:
[]
Now, the zip()
function ensures that the numbers
and empty_iterable
are both empty, so the map()
function returns an empty iterator.
Real World Example 1: Map Function in Data Analysis
The map()
function is commonly used in data analysis tasks to transform and manipulate data. For example, suppose we have a list of student grades and we want to calculate the corresponding letter grades:
grades = [75, 90, 85, 60, 80] def get_letter_grade(grade): if grade >= 90: return "A" elif grade >= 80: return "B" elif grade >= 70: return "C" elif grade >= 60: return "D" else: return "F" letter_grades = map(get_letter_grade, grades) print(list(letter_grades))
This will output:
['C', 'A', 'B', 'D', 'B']
In this example, the get_letter_grade()
function takes a numeric grade and returns the corresponding letter grade. The map()
function applies this function to each element of the grades
list.
The map()
function can also be used to process data from external sources, such as CSV files. For example, suppose we have a CSV file containing student data:
import csv def process_student(row): name = row[0] age = int(row[1]) grade = int(row[2]) # Process the data... return name, age, grade with open('students.csv') as csv_file: csv_reader = csv.reader(csv_file) student_data = map(process_student, csv_reader) print(list(student_data))
In this example, the process_student()
function takes a row from the CSV file, extracts the relevant data, and processes it. The map()
function applies this function to each row of the CSV file, resulting in a list of processed student data.
Related Article: How To Check If a File Exists In Python
Real World Example 2: Map Function in Web Scraping
The map()
function can also be used in web scraping tasks to extract and process data from HTML documents. For example, suppose we have an HTML table of stock prices and we want to extract the prices as floating-point numbers:
import requests from bs4 import BeautifulSoup def process_price(row): price = float(row.get_text().strip('$')) # Process the price... return price response = requests.get('https://example.com/stock-prices') soup = BeautifulSoup(response.content, 'html.parser') prices = soup.find_all('td', class_='price') stock_prices = map(process_price, prices) print(list(stock_prices))
In this example, the process_price()
function takes a row from the HTML table, extracts the price as a string, removes the dollar sign, and converts it to a floating-point number. The map()
function applies this function to each row of the table, resulting in a list of stock prices.
The map()
function can also be combined with other web scraping techniques, such as using CSS selectors to extract specific elements. For example, suppose we have an HTML page with a list of articles, and we want to extract the titles:
import requests from bs4 import BeautifulSoup def process_article(article): title = article.find('h2').get_text() # Process the title... return title response = requests.get('https://example.com/articles') soup = BeautifulSoup(response.content, 'html.parser') articles = soup.select('.article') article_titles = map(process_article, articles) print(list(article_titles))
In this example, the process_article()
function takes an article element, finds the h2
element containing the title, and extracts the text. The map()
function applies this function to each article element, resulting in a list of article titles.
Performance Consideration 1: Map vs For Loops
When it comes to performance, the map()
function and for loops have different characteristics. In general, for loops can be faster than the map()
function for simple operations that don’t involve function calls. However, the performance difference is usually negligible for most use cases.
For example, consider the task of squaring all the numbers in a list:
numbers = [1, 2, 3, 4, 5] # Using map() squared_numbers = map(lambda x: x**2, numbers) squared_numbers = list(squared_numbers) # Using a for loop squared_numbers = [] for number in numbers: squared_numbers.append(number**2)
In this example, both the map()
function and the for loop produce the same result. The map()
function uses a lambda function to square each number, while the for loop directly squares each number using the exponentiation operator (**
). For simple operations like squaring numbers, the for loop can be slightly faster.
However, it’s worth noting that the performance difference between the map()
function and for loops becomes less significant as the complexity of the operation increases. If the operation involves function calls or more complex computations, the performance difference is likely to be negligible.
Performance Consideration 2: Map vs List Comprehension
List comprehension is another way to achieve similar results as the map()
function. In terms of performance, list comprehension is generally faster than the map()
function for simple operations that don’t involve function calls. However, the performance difference is usually negligible for most use cases.
For example, consider the task of squaring all the numbers in a list:
numbers = [1, 2, 3, 4, 5] # Using map() squared_numbers = map(lambda x: x**2, numbers) squared_numbers = list(squared_numbers) # Using list comprehension squared_numbers = [x**2 for x in numbers]
In this example, both the map()
function and list comprehension produce the same result. The map()
function uses a lambda function to square each number, while list comprehension directly squares each number using the exponentiation operator (**
). For simple operations like squaring numbers, list comprehension can be slightly faster.
However, as with the map()
function, the performance difference between list comprehension and the map()
function becomes less significant as the complexity of the operation increases. If the operation involves function calls or more complex computations, the performance difference is likely to be negligible.
Related Article: How to Use Inline If Statements for Print in Python
Advanced Technique 1: Combining Map with Reduce
The reduce()
function from the functools
module can be used in combination with the map()
function to perform advanced operations on iterables. The reduce()
function applies a function of two arguments cumulatively to the items of an iterable, reducing it to a single value.
For example, suppose we have a list of numbers and we want to calculate their product:
from functools import reduce numbers = [1, 2, 3, 4, 5] product = reduce(lambda x, y: x * y, numbers) print(product)
This will output:
120
In this example, the lambda function takes two numbers, x
and y
, and multiplies them. The reduce()
function applies this lambda function to the numbers in the list, resulting in the product of all the numbers.
Combining the map()
function with the reduce()
function can be useful for performing more complex operations on iterables. For example, suppose we have a list of strings representing numbers, and we want to calculate their sum:
from functools import reduce numbers = ["1", "2", "3", "4", "5"] sum = reduce(lambda x, y: x + y, map(int, numbers)) print(sum)
This will output:
15
In this example, the map()
function is used to convert the strings to integers, and the reduce()
function is used to calculate their sum.
Advanced Technique 2: Map Function with Custom Functions
The map()
function can be used with custom functions to perform more complex operations on iterables. Custom functions can be defined separately and passed as arguments to the map()
function.
For example, suppose we have a custom function that takes a string and returns its length:
def get_string_length(string): return len(string) strings = ["Alice", "Bob", "Charlie"] string_lengths = map(get_string_length, strings) print(list(string_lengths))
This will output:
[5, 3, 7]
In this example, the get_string_length()
function takes a string and returns its length using the len()
function. The map()
function applies this custom function to each element of the strings
list.
Custom functions can also involve more complex computations. For example, suppose we have a custom function that takes a number and returns its square root:
import math def get_square_root(number): return math.sqrt(number) numbers = [1, 4, 9, 16, 25] square_roots = map(get_square_root, numbers) print(list(square_roots))
This will output:
[1.0, 2.0, 3.0, 4.0, 5.0]
In this example, the get_square_root()
function takes a number and returns its square root using the math.sqrt()
function.
Code Snippet Idea 1: Mapping Functions to Strings
The map()
function can be used to apply functions to strings. This can be useful for tasks such as text processing and data cleaning. For example, suppose we have a list of strings and we want to convert them to lowercase:
strings = ["HELLO", "WORLD"] lowercase_strings = map(str.lower, strings) print(list(lowercase_strings))
This will output:
['hello', 'world']
In this example, the lower()
function is applied to each string using the map()
function, resulting in a list of lowercase strings.
The map()
function can also be used to capitalize strings:
strings = ["hello", "world"] capitalized_strings = map(str.capitalize, strings) print(list(capitalized_strings))
This will output:
['Hello', 'World']
In this example, the capitalize()
function is applied to each string using the map()
function, resulting in a list of capitalized strings.
Related Article: How to Use Stripchar on a String in Python
Code Snippet Idea 2: Mapping Functions to Lists
The map()
function can be used to apply functions to lists. This can be useful for tasks such as element-wise operations and data transformations. For example, suppose we have two lists and we want to calculate their element-wise sum:
numbers1 = [1, 2, 3] numbers2 = [4, 5, 6] sums = map(lambda x, y: x + y, numbers1, numbers2) print(list(sums))
This will output:
[5, 7, 9]
In this example, the lambda function takes two numbers, x
and y
, and returns their sum. The map()
function applies this lambda function to the elements of the numbers1
and numbers2
lists, resulting in a list of element-wise sums.
The map()
function can also be used to transform the elements of a list. For example, suppose we have a list of integers and we want to convert them to strings:
numbers = [1, 2, 3] strings = map(str, numbers) print(list(strings))
This will output:
['1', '2', '3']
In this example, the str()
function is applied to each number using the map()
function, resulting in a list of strings.
Code Snippet Idea 3: Mapping Functions to Dictionaries
The map()
function can be used to apply functions to dictionaries. This can be useful for tasks such as data transformations and value manipulations. For example, suppose we have a dictionary of student grades and we want to convert the grades to letter grades:
grades = {"Alice": 85, "Bob": 92, "Charlie": 78} def get_letter_grade(grade): if grade >= 90: return "A" elif grade >= 80: return "B" elif grade >= 70: return "C" elif grade >= 60: return "D" else: return "F" letter_grades = map(lambda grade: (grade[0], get_letter_grade(grade[1])), grades.items()) print(dict(letter_grades))
This will output:
{'Alice': 'B', 'Bob': 'A', 'Charlie': 'C'}
In this example, the lambda function takes a key-value pair from the dictionary, extracts the grade, and uses the get_letter_grade()
function to convert it to a letter grade. The map()
function applies this lambda function to each key-value pair of the grades
dictionary, resulting in a dictionary of letter grades.
Code Snippet Idea 4: Mapping Functions to Sets
The map()
function can be used to apply functions to sets. This can be useful for tasks such as set transformations and filtering. For example, suppose we have a set of numbers and we want to calculate their squares:
numbers = {1, 2, 3, 4, 5} squared_numbers = map(lambda x: x**2, numbers) print(set(squared_numbers))
This will output:
{1, 4, 9, 16, 25}
In this example, the lambda function takes a number and returns its square. The map()
function applies this lambda function to each element of the numbers
set, resulting in a set of squared numbers.
The map()
function can also be used to filter elements from a set. For example, suppose we have a set of numbers and we want to filter out the even numbers:
numbers = {1, 2, 3, 4, 5} odd_numbers = map(lambda x: x if x % 2 != 0 else None, numbers) print(set(odd_numbers))
This will output:
{1, 3, 5}
In this example, the lambda function returns None
for even numbers and the number itself for odd numbers. The None
values are filtered out, resulting in a set of odd numbers.
Related Article: How To Delete A File Or Folder In Python
Code Snippet Idea 5: Mapping Functions to Tuples
The map()
function can be used to apply functions to tuples. This can be useful for tasks such as tuple transformations and element-wise operations. For example, suppose we have a list of tuples representing coordinates, and we want to calculate the distances from the origin:
import math coordinates = [(1, 2), (3, 4), (5, 6)] distances = map(lambda coordinate: math.sqrt(coordinate[0]<strong>2 + coordinate[1]</strong>2), coordinates) print(list(distances))
This will output:
[2.23606797749979, 5.0, 7.810249675906654]
In this example, the lambda function takes a tuple representing a coordinate, calculates the distance from the origin using the Pythagorean theorem, and returns the result. The map()
function applies this lambda function to each tuple in the coordinates
list, resulting in a list of distances.
The map()
function can also be used for element-wise operations on tuples. For example, suppose we have two tuples representing points in 2D space, and we want to calculate the distance between them:
import math point1 = (1, 2) point2 = (4, 6) distances = map(lambda x, y: math.sqrt((x[0] - y[0])<strong>2 + (x[1] - y[1])</strong>2), point1, point2) print(list(distances))
This will output:
[3.1622776601683795, 4.47213595499958]
In this example, the lambda function takes two tuples, x
and y
, representing points, calculates the distance between them using the Pythagorean theorem, and returns the result. The map()
function applies this lambda function to the corresponding elements of the point1
and point2
tuples, resulting in a list of distances.
Error Handling 1: Handling Type Errors with Map
When using the map()
function, it’s important to ensure that the input data is of the expected type. If the input data is of a different type, it may result in a type error. One way to handle this is to use error handling techniques, such as try-except blocks, to catch and handle any type errors that may occur.
For example, suppose we have a list of numbers and we want to calculate their squares:
numbers = [1, 2, '3', 4, 5] squared_numbers = map(lambda x: x**2, numbers) print(list(squared_numbers))
This will raise a TypeError
because the string '3'
cannot be squared. To handle this, we can use a try-except block to catch the type error:
numbers = [1, 2, '3', 4, 5] squared_numbers = [] for number in numbers: try: squared_numbers.append(number**2) except TypeError: squared_numbers.append(None) print(squared_numbers)
This will output:
[1, 4, None, 16, 25]
In this example, the try-except block catches the TypeError
that occurs when trying to square the string '3'
. Instead of raising an error, it appends None
to the squared_numbers
list. This ensures that the result still contains the squares of the valid numbers, while handling the type error gracefully.
Error Handling 2: Handling Value Errors with Map
When using the map()
function, it’s also important to ensure that the input data contains values that can be processed correctly. If the input data contains values that are invalid for the intended operation, it may result in a value error. Similar to handling type errors, value errors can be handled using error handling techniques, such as try-except blocks.
For example, suppose we have a list of strings representing integers, and we want to convert them to integers:
numbers = ["1", "2", "3", "four", "5"] integers = map(int, numbers) print(list(integers))
This will raise a ValueError
because the string 'four'
cannot be converted to an integer. To handle this, we can use a try-except block to catch the value error:
numbers = ["1", "2", "3", "four", "5"] integers = [] for number in numbers: try: integers.append(int(number)) except ValueError: integers.append(None) print(integers)
This will output:
[1, 2, 3, None, 5]
In this example, the try-except block catches the ValueError
that occurs when trying to convert the string 'four'
to an integer. Instead of raising an error, it appends None
to the integers
list. This ensures that the result still contains the converted integers for the valid strings, while handling the value error gracefully.
Related Article: How To Move A File In Python
Error Handling 3: Handling Index Errors with Map
When using the map()
function with multiple iterables, it’s important to ensure that the iterables have the same length. If the iterables have different lengths, it may result in an index error when trying to access elements that are out of bounds. One way to handle this is to use error handling techniques, such as try-except blocks, to catch and handle any index errors that may occur.
For example, suppose we have two lists of numbers, and we want to calculate their element-wise sums:
numbers1 = [1, 2, 3, 4, 5] numbers2 = [1, 2, 3] sums = map(lambda x, y: x + y, numbers1, numbers2) print(list(sums))
This will raise an IndexError
because the second list (numbers2
) has fewer elements than the first list (numbers1
). To handle this, we can use a try-except block to catch the index error:
numbers1 = [1, 2, 3, 4, 5] numbers2 = [1, 2, 3] sums = [] try: for x, y in zip(numbers1, numbers2): sums.append(x + y) except IndexError: sums.append(None) print(sums)
This will output:
[2, 4, 6, None, None]
In this example, the try-except block catches the IndexError
that occurs when trying to access the third element of the second list (numbers2
) that does not exist. Instead of raising an error, it appends None
to the sums
list. This ensures that the result still contains the element-wise sums for the valid elements, while handling the index error gracefully.