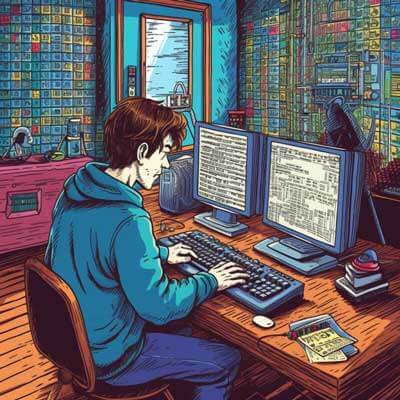
Table of Contents
To convert a Python dictionary to a dataframe, you can use the pandas library, which provides efficient and powerful data manipulation and analysis tools. Converting a dictionary to a dataframe is a common operation when working with data in Python, as it allows you to easily manipulate and analyze the data using pandas' extensive functionality. In this answer, we will explore two possible methods for converting a Python dictionary to a dataframe.
Method 1: Using the pandas.DataFrame.from_dict() Method
The pandas library provides the DataFrame.from_dict()
method, which allows you to create a dataframe from a dictionary. This method takes the dictionary as input and converts it into a dataframe, where the keys of the dictionary become the column names and the values become the corresponding column values.
Here is an example of how to use the DataFrame.from_dict()
method to convert a dictionary to a dataframe:
import pandas as pd # Example dictionary data = {'Name': ['John', 'Jane', 'Mike'], 'Age': [25, 30, 35], 'City': ['New York', 'Paris', 'London']} # Convert dictionary to dataframe df = pd.DataFrame.from_dict(data) # Print the dataframe print(df)
Output:
Name Age City 0 John 25 New York 1 Jane 30 Paris 2 Mike 35 London
In the above example, we create a dictionary data
with three keys ('Name', 'Age', 'City') and their corresponding values. We then pass this dictionary to the DataFrame.from_dict()
method, which converts it to a dataframe df
. Finally, we print the dataframe to see the result.
Related Article: Working with Numpy Concatenate
Method 2: Using the pandas.DataFrame() Constructor
Another way to convert a dictionary to a dataframe is by using the pandas DataFrame()
constructor. This constructor allows you to create a dataframe by passing a dictionary as input, where the keys of the dictionary become the column names and the values become the corresponding column values.
Here is an example of how to use the DataFrame()
constructor to convert a dictionary to a dataframe:
import pandas as pd # Example dictionary data = {'Name': ['John', 'Jane', 'Mike'], 'Age': [25, 30, 35], 'City': ['New York', 'Paris', 'London']} # Convert dictionary to dataframe df = pd.DataFrame(data) # Print the dataframe print(df)
Output:
Name Age City 0 John 25 New York 1 Jane 30 Paris 2 Mike 35 London
In the above example, we create a dictionary data
with three keys ('Name', 'Age', 'City') and their corresponding values. We then pass this dictionary to the DataFrame()
constructor, which converts it to a dataframe df
. Finally, we print the dataframe to see the result.
Why Convert a Python Dict to Dataframe?
The question of how to convert a Python dictionary to a dataframe arises in scenarios where you have data stored in the form of a dictionary and you want to perform various data manipulations and analyses using pandas. Converting the dictionary to a dataframe allows you to leverage the extensive functionality provided by pandas for data manipulation, analysis, and visualization.
Some potential reasons for wanting to convert a dictionary to a dataframe include:
1. Data preprocessing: Before performing data analysis or machine learning tasks, it is often necessary to preprocess the data. Converting a dictionary to a dataframe can be the first step in this preprocessing pipeline, as it allows you to easily manipulate and transform the data using pandas.
2. Data analysis: Once the data is in a dataframe, you can use pandas' powerful tools and functions to perform various data analysis tasks, such as aggregations, filtering, sorting, and grouping. This can help you gain insights and make data-driven decisions.
3. Data visualization: Pandas provides integration with popular data visualization libraries such as Matplotlib and Seaborn. By converting a dictionary to a dataframe, you can take advantage of these libraries to create visually appealing plots and charts to better understand your data.
Suggestions and Alternative Ideas
While the methods described above are the most straightforward ways to convert a Python dictionary to a dataframe using pandas, there are a few alternative ideas and suggestions to consider:
1. Nested dictionaries: If your dictionary contains nested dictionaries, you can use the orient
parameter of the DataFrame.from_dict()
method to control how the nested dictionaries are converted to a dataframe. By specifying the orient
parameter as 'index'
, 'columns'
, or 'values'
, you can choose the desired orientation of the resulting dataframe.
2. Additional parameters: The DataFrame.from_dict()
method and the DataFrame()
constructor both accept additional parameters that allow you to customize the conversion process. For example, you can specify the column names, index labels, and data types of the resulting dataframe by using the columns
, index
, and dtype
parameters, respectively.
3. Data cleaning and validation: Before converting a dictionary to a dataframe, it is important to ensure that the dictionary is properly formatted and that the data types of the values are consistent. You can use built-in Python functions or libraries like json
or schema
to validate and clean your data before converting it to a dataframe.
Related Article: How to Use Python Time Sleep
Best Practices
When converting a Python dictionary to a dataframe, it is good practice to follow these best practices:
1. Clean and validate the data: Before converting the dictionary to a dataframe, make sure that the data is properly formatted and validated. This includes checking for missing values, inconsistent data types, and any other data quality issues.
2. Provide meaningful column names: When converting a dictionary to a dataframe, the keys of the dictionary become the column names. It is important to choose descriptive column names that accurately represent the data contained in each column. This will make it easier to understand and work with the resulting dataframe.
3. Handle missing values appropriately: If your dictionary contains missing values, it is important to handle them appropriately. Pandas provides various functions for handling missing values, such as fillna()
and dropna()
. Choose the method that best suits your needs and the nature of your data.
4. Consider data types: Pandas automatically assigns data types to the columns in the dataframe based on the data contained in each column. However, it is often necessary to manually specify the data types to ensure that the dataframe is correctly interpreted. Use the dtype
parameter of the DataFrame.from_dict()
method or the DataFrame()
constructor to specify the desired data types.
5. Optimize performance: If you are working with large datasets, it is important to optimize the performance of your code. This can be achieved by avoiding unnecessary computations, using appropriate data types, and leveraging pandas' vectorized operations instead of iterating over the data.