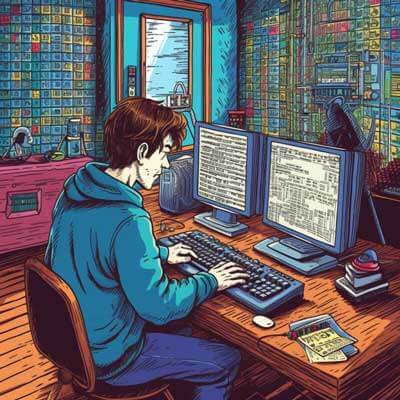
Table of Contents
Monitoring Tools for Python Django Development
Monitoring is a critical aspect of software development, allowing developers to detect and address issues that may arise in their applications. In Python Django development, there are several useful tools available for monitoring. Two popular choices are Sentry and New Relic.
Related Article: Python File Operations: How to Read, Write, Delete, Copy
Using Sentry for Error Monitoring
Sentry is an open-source error monitoring tool that helps developers track and fix errors in their applications. It provides real-time error tracking, allowing developers to identify and resolve issues quickly. Sentry has a rich set of features, including error grouping, event data, and integrations with popular development tools.
To use Sentry in a Python Django application, you need to install the sentry-sdk
package and configure it with your Sentry project's DSN (Data Source Name). The DSN is a unique identifier that links your application to your Sentry project.
Here's an example of how to configure Sentry in a Django project:
# settings.py import sentry_sdk from sentry_sdk.integrations.django import DjangoIntegration sentry_sdk.init( dsn="your-sentry-dsn", integrations=[DjangoIntegration()] )
With Sentry configured, you can now capture and report errors in your Django application. Sentry automatically captures unhandled exceptions and allows you to manually capture specific errors using the sentry_sdk.capture_exception()
function.
# views.py from django.http import HttpResponse import sentry_sdk def my_view(request): try: # Your code here pass except Exception as e: sentry_sdk.capture_exception(e) return HttpResponse("Hello, World!")
Sentry provides a comprehensive web interface that allows you to view and manage captured errors. You can track error occurrences, view stack traces, and even assign errors to team members for resolution.
Leveraging New Relic for Performance Monitoring
New Relic is a popular performance monitoring tool that helps developers identify and optimize performance bottlenecks in their applications. It provides real-time insights into application performance, allowing developers to monitor response times, database queries, and external service calls.
To use New Relic in a Python Django application, you need to install the newrelic
package and configure it with your New Relic license key.
Here's an example of how to configure New Relic in a Django project:
# wsgi.py import newrelic.agent newrelic.agent.initialize("your-newrelic-license-key") # Rest of the WSGI configuration
With New Relic configured, you can now monitor the performance of your Django application. New Relic automatically tracks transactions, database queries, and external service calls. It provides detailed performance metrics and allows you to set up alerts based on predefined thresholds.
New Relic also offers a comprehensive web interface that allows you to analyze performance data, generate reports, and visualize trends over time. This can help you identify and resolve performance issues before they impact your users.
Integrating SQLAlchemy with Django ORM
SQLAlchemy is a useful SQL toolkit and Object-Relational Mapping (ORM) library for Python. It provides a set of high-level API for interacting with databases, allowing developers to write database-agnostic code.
While Django comes with its own ORM, Django ORM, SQLAlchemy can be integrated with Django to leverage its advanced features. This integration allows developers to benefit from the flexibility and power of SQLAlchemy while still using the familiar Django ORM syntax.
To integrate SQLAlchemy with Django ORM, you need to install the sqlalchemy
package and configure it in your Django project's settings.
Here's an example of how to configure SQLAlchemy in a Django project:
# settings.py DATABASES = { 'default': { 'ENGINE': 'django.db.backends.postgresql', 'NAME': 'mydatabase', 'USER': 'mydatabaseuser', 'PASSWORD': 'mypassword', 'HOST': 'localhost', 'PORT': '5432', 'OPTIONS': { 'drivername': 'postgresql+psycopg2', } } } # Rest of the Django settings
With SQLAlchemy configured, you can now use it alongside Django ORM in your Django models. You can define SQLAlchemy models using the sqlalchemy.ext.declarative
module and then map them to Django models using the django.db.models
module.
Here's an example of how to define a SQLAlchemy model and map it to a Django model:
# models.py from sqlalchemy.ext.declarative import declarative_base from sqlalchemy import Column, Integer, String from django.db import models Base = declarative_base() class SQLAlchemyModel(Base): __tablename__ = 'mytable' id = Column(Integer, primary_key=True) name = Column(String) class DjangoModel(models.Model): name = models.CharField(max_length=255) class Meta: managed = False db_table = 'mytable'
With the integration in place, you can now use SQLAlchemy queries alongside Django ORM queries in your Django views. This allows you to take advantage of SQLAlchemy's useful querying capabilities while still benefiting from Django's convenience and features.
Related Article: How To Write Pandas Dataframe To CSV File
Benefits of Using SQLAlchemy alongside Django ORM
Integrating SQLAlchemy with Django ORM offers several benefits for Python Django development:
1. Flexibility: SQLAlchemy provides a more flexible and expressive API for working with databases. It allows developers to write complex queries and perform advanced database operations that may not be possible or straightforward with Django ORM alone.
2. Database Agnosticism: SQLAlchemy supports multiple database backends, allowing developers to write code that is not tied to a specific database. This makes it easier to switch between different databases or support multiple databases in the same application.
3. Advanced Features: SQLAlchemy offers a wide range of advanced features, including support for complex joins, subqueries, and custom SQL expressions. These features can be useful in scenarios where Django ORM falls short or cannot meet specific requirements.
4. Code Reusability: By integrating SQLAlchemy with Django ORM, developers can reuse existing SQLAlchemy code and libraries in their Django projects. This can save development time and effort, as well as leverage the extensive ecosystem of SQLAlchemy extensions and plugins.
Introduction to Celery for Asynchronous Tasks
In modern web applications, there are often tasks that need to be performed asynchronously, such as sending emails, processing large datasets, or performing time-consuming calculations. Performing these tasks synchronously can lead to slow response times and a poor user experience. Celery is a useful distributed task queue system that allows developers to handle asynchronous tasks efficiently.
Celery is built on a message broker such as RabbitMQ or Redis, which acts as a communication channel between the application and the Celery workers. The application pushes tasks to the message broker, and the Celery workers consume these tasks and execute them asynchronously.
To use Celery in a Python Django application, you need to install the celery
package and configure it with your chosen message broker.
Here's an example of how to configure Celery in a Django project:
# settings.py CELERY_BROKER_URL = 'redis://localhost:6379/0' CELERY_RESULT_BACKEND = 'redis://localhost:6379/0' CELERY_ACCEPT_CONTENT = ['application/json'] CELERY_TASK_SERIALIZER = 'json' CELERY_RESULT_SERIALIZER = 'json' # Rest of the Django settings
With Celery configured, you can now define and execute asynchronous tasks in your Django application. To define a Celery task, you need to create a Python function decorated with the @celery.task
decorator.
Here's an example of how to define and execute a Celery task:
# tasks.py from celery import shared_task @shared_task def send_email(to, subject, body): # Code to send email pass # views.py from .tasks import send_email def my_view(request): send_email.delay('user@example.com', 'Hello', 'Welcome to our website!') return HttpResponse("Task submitted successfully!")
In this example, the send_email
task is defined as a Celery task. It can be invoked asynchronously using the delay()
method, which adds the task to the message broker for processing by the Celery workers.
Celery provides a robust monitoring and management interface called Flower. It allows you to monitor task progress, view task history, and manage Celery workers through a web-based dashboard.
Utilizing Celery for Asynchronous Task Handling in Python Django
Celery provides several features and capabilities that make it a useful tool for handling asynchronous tasks in Python Django development:
1. Scalability: Celery is designed to handle large workloads and can scale horizontally by adding more Celery workers. This allows you to handle increasing amounts of asynchronous tasks as your application grows.
2. Task Prioritization: Celery allows you to assign priorities to tasks, ensuring that high-priority tasks are executed before lower-priority ones. This can be useful for managing time-sensitive tasks or tasks with different levels of importance.
3. Task Chaining: Celery supports task chaining, which allows you to define complex workflows by chaining multiple tasks together. This can be useful for breaking down complex tasks into smaller, manageable units, and executing them in a specific order.
4. Task Retry: Celery provides built-in support for task retrying, allowing you to automatically retry failed tasks. This can be helpful in scenarios where temporary failures occur, such as network issues or external service unavailability.
5. Task Result Handling: Celery allows you to retrieve the result of a completed task, either synchronously or asynchronously. This can be useful for obtaining the output of a task or performing follow-up actions based on the task result.
Code Snippet: Using Sentry for Error Monitoring in Django
# settings.py import sentry_sdk from sentry_sdk.integrations.django import DjangoIntegration sentry_sdk.init( dsn="your-sentry-dsn", integrations=[DjangoIntegration()] )
# views.py from django.http import HttpResponse import sentry_sdk def my_view(request): try: # Your code here pass except Exception as e: sentry_sdk.capture_exception(e) return HttpResponse("Hello, World!")
Related Article: How to Use Pandas Dataframe Apply in Python
Code Snippet: Configuring and Utilizing New Relic in Django
# wsgi.py import newrelic.agent newrelic.agent.initialize("your-newrelic-license-key") # Rest of the WSGI configuration
Code Snippet: Integrating SQLAlchemy with Django ORM
# settings.py DATABASES = { 'default': { 'ENGINE': 'django.db.backends.postgresql', 'NAME': 'mydatabase', 'USER': 'mydatabaseuser', 'PASSWORD': 'mypassword', 'HOST': 'localhost', 'PORT': '5432', 'OPTIONS': { 'drivername': 'postgresql+psycopg2', } } } # Rest of the Django settings
# models.py from sqlalchemy.ext.declarative import declarative_base from sqlalchemy import Column, Integer, String from django.db import models Base = declarative_base() class SQLAlchemyModel(Base): __tablename__ = 'mytable' id = Column(Integer, primary_key=True) name = Column(String) class DjangoModel(models.Model): name = models.CharField(max_length=255) class Meta: managed = False db_table = 'mytable'
Code Snippet: Leveraging Celery for Asynchronous Tasks in Django
# settings.py CELERY_BROKER_URL = 'redis://localhost:6379/0' CELERY_RESULT_BACKEND = 'redis://localhost:6379/0' CELERY_ACCEPT_CONTENT = ['application/json'] CELERY_TASK_SERIALIZER = 'json' CELERY_RESULT_SERIALIZER = 'json' # Rest of the Django settings
# tasks.py from celery import shared_task @shared_task def send_email(to, subject, body): # Code to send email pass # views.py from .tasks import send_email def my_view(request): send_email.delay('user@example.com', 'Hello', 'Welcome to our website!') return HttpResponse("Task submitted successfully!")