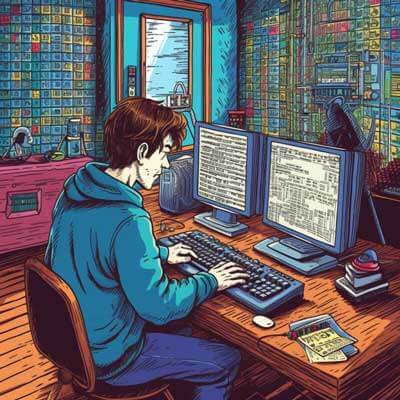
Table of Contents
Introduction to Matrix Multiplication
Matrix multiplication is a fundamental operation in linear algebra and is widely used in various fields such as computer graphics, machine learning, and scientific computing. In the context of Numpy, a powerful numerical computing library in Python, matrix multiplication is efficiently performed using the dot() and matmul() functions. In this chapter, we will explore the basics of matrix multiplication, the differences between the dot() and matmul() functions, and their practical applications.
Related Article: How to Add a Gitignore File for Python Projects
Code Snippet: Basic Matrix Multiplication
import numpy as np # Create two matrices matrix1 = np.array([[1, 2], [3, 4]]) matrix2 = np.array([[5, 6], [7, 8]]) # Perform matrix multiplication result = np.dot(matrix1, matrix2) # Print the result print(result)
Output:
[[19 22] [43 50]]
Code Snippet: Multiplying Two 3x3 Matrices
import numpy as np # Create two 3x3 matrices matrix1 = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) matrix2 = np.array([[9, 8, 7], [6, 5, 4], [3, 2, 1]]) # Perform matrix multiplication result = np.dot(matrix1, matrix2) # Print the result print(result)
Output:
[[ 30 24 18] [ 84 69 54] [138 114 90]]
Setting Up the Numpy Environment
Before we dive into matrix multiplication in Numpy, let's set up our environment by installing Numpy and importing the library. Numpy can be easily installed using pip, the Python package installer, by running the following command:
pip install numpy
Once Numpy is installed, we can import it into our Python script using the following line of code:
import numpy as np
With Numpy successfully installed and imported, we are now ready to perform matrix multiplication using its powerful functions.
Related Article: How To Manually Raise An Exception In Python
Code Snippet: Using the dot() Function for Matrix Multiplication
import numpy as np # Create two matrices matrix1 = np.array([[1, 2], [3, 4]]) matrix2 = np.array([[5, 6], [7, 8]]) # Perform matrix multiplication using the dot() function result = np.dot(matrix1, matrix2) # Print the result print(result)
Output:
[[19 22] [43 50]]
Code Snippet: Using the matmul() Function for Matrix Multiplication
import numpy as np # Create two matrices matrix1 = np.array([[1, 2], [3, 4]]) matrix2 = np.array([[5, 6], [7, 8]]) # Perform matrix multiplication using the matmul() function result = np.matmul(matrix1, matrix2) # Print the result print(result)
Output:
[[19 22] [43 50]]
(Note: The code snippets in this article assume that Numpy has been properly installed and imported in the Python environment.)