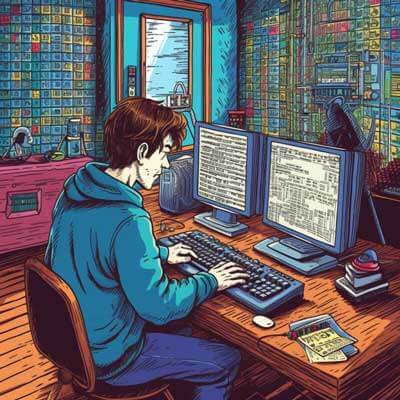
Table of Contents
To import files from different folders in Python, you can use the following methods:
Method 1: Adding the Folder to the System Path
One way to import files from a different folder is to add the folder to the system path. The system path is a list of directories where Python looks for modules to import. By adding the folder to the system path, you can import modules from that folder as if they were in the current directory.
Here's how you can add a folder to the system path:
import sys sys.path.append('/path/to/folder')
Replace /path/to/folder
with the actual path to the folder you want to import from.
After adding the folder to the system path, you can import modules from that folder using the import
statement:
import module_name
Replace module_name
with the name of the module you want to import.
Related Article: How to Use Python's isnumeric() Method
Method 2: Using the Relative Path
Another way to import files from a different folder is to use the relative path. The relative path specifies the location of the file relative to the current script or module.
For example, if you have the following folder structure:
project/ main.py folder/ module.py
To import module.py
from main.py
, you can use the following import statement:
from folder import module
This imports the module.py
file from the folder
folder.
If the file you want to import is in a parent folder, you can use the ..
notation to go up one level in the folder hierarchy. For example, if you have the following folder structure:
project/ main.py folder/ module.py utils/ helper.py
To import helper.py
from module.py
, you can use the following import statement:
from ..utils import helper
This imports the helper.py
file from the utils
folder, which is one level up from the folder
folder.
Best Practices
When importing files from different folders in Python, it is important to follow some best practices to ensure maintainability and readability of your code:
1. Use meaningful module and package names: Give your modules and packages descriptive names that accurately reflect their functionality. This will make it easier to understand and manage your imports.
2. Use absolute imports for top-level modules: When importing top-level modules, such as modules from the Python standard library or third-party packages, use absolute imports. This makes it clear where the module is coming from and avoids potential conflicts with local modules.
3. Use relative imports for modules within the same package: When importing modules within the same package, use relative imports. This makes it easier to move or rename the package without having to update all the import statements.
4. Avoid circular imports: Circular imports occur when two or more modules depend on each other, creating a loop. This can lead to unpredictable behavior and should be avoided. If you encounter a circular import, consider restructuring your code to remove the dependency.
5. Organize your project structure: Maintain a well-organized project structure with clear separation of concerns. Use packages and modules to group related functionality together. This will make it easier to locate and import files from different folders.
Alternative Ideas
While the methods described above are commonly used to import files from different folders in Python, there are alternative approaches you can consider based on your specific requirements:
1. Use a package manager: If you are working on a larger project with multiple dependencies, consider using a package manager like pipenv or poetry. These tools allow you to manage and install packages from different folders or repositories, simplifying the import process.
2. Use a virtual environment: Virtual environments provide isolated Python environments where you can install packages and manage dependencies. By creating a virtual environment for your project, you can avoid conflicts between different versions of packages and ensure consistent imports.
3. Use a build tool: If you are working on a project with complex build requirements, consider using a build tool like setuptools or PyInstaller. These tools allow you to package your Python code and its dependencies into a standalone executable or distributable format, making it easier to distribute and import files from different folders.