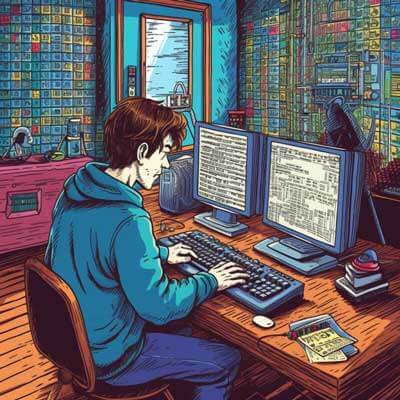
Table of Contents
A common issue encountered by Java developers is the "java.lang.ClassNotFoundException" error. This error indicates that the Java Virtual Machine (JVM) cannot find the class specified in the code during runtime. To resolve this error, you can follow the steps below:
1. Check the Classpath
The first step is to verify that the classpath is correctly set up. The classpath is a parameter that tells the JVM where to look for classes and packages. If the class you are trying to use is not in the classpath, the JVM will not be able to find it.
To check the classpath, you can use the following command in the command line:
java -classpath <main>
Replace with the directory path where your JAR files are located, and
<main>
with the name of your main class. If the classpath is incorrect, you can update it by adding the required JAR files or directories using the "-classpath" or "-cp" option.
Related Article: Spring Boot Integration with Payment, Communication Tools
2. Verify the Class Name and Package
Ensure that the class name and package in your code match the actual class name and package structure in your source code. The Java language is case-sensitive, so even a minor difference in the class name or package can result in a "ClassNotFoundException."
For example, if you have a class named "MyClass" in the package "com.example", make sure that your code references it correctly as "com.example.MyClass".
3. Check the Class Loader
The ClassLoader is responsible for loading classes at runtime. It follows a hierarchical structure and loads classes from different sources such as the bootstrap classpath, extension classpath, and application classpath.
If you are using a custom ClassLoader or have modified the default behavior, ensure that the ClassLoader is correctly configured and can find the required class.
4. Check the Deployment and Build Process
If you are working on a project that involves building and deploying a Java application, make sure that the class causing the "ClassNotFoundException" error is included in the build and deployment process.
For example, if you are using a build tool like Maven or Gradle, ensure that the required dependencies are correctly specified in the project configuration files (e.g., pom.xml for Maven). Also, ensure that the class is included in the final artifact (e.g., JAR file) that is deployed to the runtime environment.
Related Article: Tutorial: Best Practices for Java Singleton Design Pattern
5. Examine the Exception Stack Trace
When the "ClassNotFoundException" error occurs, the JVM provides a stack trace that can help identify the root cause of the error. Examine the stack trace to determine which class is causing the error and where it is being invoked in your code.
6. Check for Missing Dependencies
The "ClassNotFoundException" error can also occur if the required dependencies are missing. If your code depends on external libraries or modules, make sure that they are included in the classpath or bundled with your application.
For example, if you are using Maven as your build tool, check the project's dependencies in the pom.xml file and ensure that the required dependencies are correctly specified.
7. Verify the Java Version Compatibility
In some cases, the "ClassNotFoundException" error can occur due to a mismatch between the Java version used during compilation and the Java version used during runtime. If you are using a class or library that is not compatible with the Java version in your runtime environment, the JVM will not be able to find the class.
Ensure that the Java version used during compilation matches the Java version used during runtime. You can check the Java version by running the following command in the command line:
java -version
Make sure that the output matches the Java version you intend to use.
8. Consider Using a Build Tool
Using a build tool like Maven or Gradle can help manage dependencies and simplify the build process. These tools automatically handle the classpath and dependency resolution, reducing the chances of encountering a "ClassNotFoundException" error.
Consider integrating a build tool into your project to manage dependencies, build the project, and handle the classpath configuration.
Related Article: How to Compare Strings in Java
9. Seek Community Support
If you have tried all the above steps and are still unable to resolve the "ClassNotFoundException" error, consider seeking help from the Java community. Online forums, developer communities, and Q&A platforms like Stack Overflow can provide insights and solutions to specific issues.
When seeking community support, provide relevant details such as the complete error message, stack trace, code snippets, and any additional information that may help others understand and diagnose the problem.