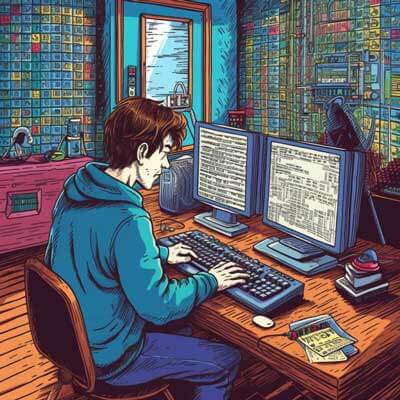
- Introduction to String and Array
- Examining String to Array Conversion Methods
- Using the split() Method
- Leveraging Regular Expressions
- Use Case: Parsing CSV Files into Array
- Code Snippet: Basic String to Array Conversion
- Code Snippet: Handling Edge Cases in Conversion
- Use Case: Splitting User Input into Tokens
- Code Snippet: Using split() with Regular Expressions
- Code Snippet: Using StringTokenizer Class
- Best Practice: Using the split() Method
- Best Practice: Leveraging Regular Expressions
- Real World Example: Text Processing in Data Analysis
- Code Snippet: Using split() with Regular Expressions
- Code Snippet: Using split() with Punctuation Characters
- Real World Example: Manipulating File Paths
- Code Snippet: Conversion with Custom Delimiter
- Code Snippet: Handling Edge Cases in Conversion
- Performance Consideration: split() vs. StringTokenizer
- Code Snippet: Using split()
- Code Snippet: Using StringTokenizer
- Performance Consideration: Impact on Memory Usage
- Code Snippet: Using split() with Large String
- Code Snippet: Using StringTokenizer with Large String
- Advanced Technique: Custom Delimiters with split()
- Code Snippet: Conversion with Custom Delimiter
- Code Snippet: Handling Escape Characters in Custom Delimiter
- Code Snippet: Conversion with Pattern and Matcher
- Error Handling: Dealing with Null Strings
- Code Snippet: Handling Null Strings
- Error Handling: Handling Invalid Delimiters
- Code Snippet: Handling Invalid Delimiter
Introduction to String and Array
In Java, a string is a sequence of characters, while an array is a data structure that stores a fixed-size sequence of elements of the same type. Converting a string to an array is a common operation in Java programming. This article will explore various methods and techniques for converting a string to an array in Java.
Related Article: How to Use the Xmx Option in Java
Examining String to Array Conversion Methods
There are multiple methods available in Java for converting a string to an array. Let’s explore two common approaches: using the split() method and leveraging regular expressions.
Using the split() Method
The split() method, available in the String class, allows us to split a string into an array of substrings based on a specified delimiter. Here’s an example:
String str = "apple,banana,orange"; String[] fruits = str.split(",");
In this example, the string “apple,banana,orange” is split into an array of strings using the comma (“,”) as the delimiter. The resulting array, fruits
, will contain three elements: “apple”, “banana”, and “orange”.
Leveraging Regular Expressions
Regular expressions provide a powerful way to match patterns in strings. We can use regular expressions to split a string into an array based on more complex patterns. Here’s an example:
String str = "Hello 123 World"; String[] tokens = str.split("\\s+");
In this example, the string “Hello 123 World” is split into an array of strings using one or more whitespace characters as the delimiter. The resulting array, tokens
, will contain three elements: “Hello”, “123”, and “World”.
Related Article: Can Two Java Threads Access the Same MySQL Session?
Use Case: Parsing CSV Files into Array
Parsing CSV (Comma-Separated Values) files is a common task in data processing. Let’s see how we can convert a CSV string into an array of values.
Code Snippet: Basic String to Array Conversion
String csv = "John,Doe,30"; String[] values = csv.split(",");
In this example, the CSV string “John,Doe,30” is split into an array of strings using the comma (“,”) as the delimiter. The resulting array, values
, will contain three elements: “John”, “Doe”, and “30”.
Code Snippet: Handling Edge Cases in Conversion
String csv = "John,Doe,,30"; String[] values = csv.split(",", -1);
In this example, the CSV string “John,Doe,,30” contains an empty value between the second and third commas. By specifying a negative limit in the split() method, we ensure that the empty value is preserved in the resulting array. The array values
will contain four elements: “John”, “Doe”, “”, and “30”.
Related Article: How to Implement Recursion in Java
Use Case: Splitting User Input into Tokens
Splitting user input into tokens is another common scenario. Let’s explore how we can convert a user-entered string into an array of tokens.
Code Snippet: Using split() with Regular Expressions
Scanner scanner = new Scanner(System.in); System.out.println("Enter a sentence:"); String sentence = scanner.nextLine(); String[] tokens = sentence.split("\\s+");
In this example, the user is prompted to enter a sentence. The split()
method with the regular expression “\\s+” is used to split the sentence into an array of tokens based on one or more whitespace characters. The resulting array, tokens
, will contain each word from the sentence as a separate element.
Code Snippet: Using StringTokenizer Class
import java.util.StringTokenizer; String sentence = "This is a sentence."; StringTokenizer tokenizer = new StringTokenizer(sentence); String[] tokens = new String[tokenizer.countTokens()]; int index = 0; while (tokenizer.hasMoreTokens()) { tokens[index++] = tokenizer.nextToken(); }
In this example, the StringTokenizer
class is used to split the sentence into tokens. The countTokens()
method is used to determine the number of tokens, and a string array is created with the same size. The nextToken()
method is called iteratively to extract each token and store it in the array.
Related Article: Java Adapter Design Pattern Tutorial
Best Practice: Using the split() Method
When converting a string to an array in Java, using the split() method is often the simplest and most straightforward approach. It allows us to split a string into an array of substrings based on a specified delimiter.
Best Practice: Leveraging Regular Expressions
Regular expressions provide a more flexible and powerful way to split strings into arrays. By defining complex patterns, we can split strings based on various criteria, such as whitespace, punctuation, or specific character sequences.
Real World Example: Text Processing in Data Analysis
In data analysis and text processing tasks, converting strings to arrays can be essential. Let’s consider a real-world example of how this conversion can be used in text analysis.
Related Article: How to Print a Hashmap in Java
Code Snippet: Using split() with Regular Expressions
String text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit."; String[] words = text.split("\\s+");
In this example, the string “Lorem ipsum dolor sit amet, consectetur adipiscing elit.” is split into an array of words using the split() method with the regular expression “\\s+”. The resulting array, words
, will contain each word from the text as a separate element.
Code Snippet: Using split() with Punctuation Characters
String text = "Hello! How are you?"; String[] sentences = text.split("[.!?]");
In this example, the string “Hello! How are you?” is split into an array of sentences using the split() method with the regular expression “[.!?]”. The resulting array, sentences
, will contain each sentence from the text as a separate element.
Real World Example: Manipulating File Paths
When working with file paths, converting strings to arrays can be useful for extracting directory names or specific components of the path. Let’s explore a real-world example.
Related Article: How to Manage Collections Without a SortedList in Java
Code Snippet: Conversion with Custom Delimiter
String filePath = "/home/user/documents/file.txt"; String[] pathComponents = filePath.split("/");
In this example, the file path “/home/user/documents/file.txt” is split into an array of path components using the slash (“/”) as the delimiter. The resulting array, pathComponents
, will contain each component of the file path as a separate element.
Code Snippet: Handling Edge Cases in Conversion
String filePath = "/home/user/documents/"; String[] pathComponents = filePath.split("/");
In this example, the file path “/home/user/documents/” ends with a slash (“/”) character. By using the split() method, we can still obtain the desired array of path components, even if the last component is an empty string.
Performance Consideration: split() vs. StringTokenizer
When it comes to performance considerations, the split() method and the StringTokenizer class have different characteristics. Let’s compare them.
Related Article: Java String Comparison: String vs StringBuffer vs StringBuilder
Code Snippet: Using split()
String text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit."; String[] words = text.split("\\s+");
In this example, the split() method is used to split the string into an array of words. The regular expression “\\s+” is used to split the text based on whitespace characters.
Code Snippet: Using StringTokenizer
String text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit."; StringTokenizer tokenizer = new StringTokenizer(text); String[] words = new String[tokenizer.countTokens()]; int index = 0; while (tokenizer.hasMoreTokens()) { words[index++] = tokenizer.nextToken(); }
In this example, the StringTokenizer class is used to split the string into tokens. The countTokens() method is used to determine the number of tokens, and a string array is created with the same size. The nextToken() method is called iteratively to extract each token and store it in the array.
Performance Consideration: Impact on Memory Usage
When converting strings to arrays, it’s important to consider the impact on memory usage, especially for large strings or when performing the conversion frequently. Let’s explore this aspect.
Related Article: How to Fix the Java NullPointerException
Code Snippet: Using split() with Large String
String largeString = "Lorem ipsum dolor sit amet, consectetur adipiscing elit."; String[] words = largeString.split("\\s+");
In this example, a large string is split into an array of words using the split() method. The regular expression “\\s+” is used to split the text based on whitespace characters.
Code Snippet: Using StringTokenizer with Large String
String largeString = "Lorem ipsum dolor sit amet, consectetur adipiscing elit."; StringTokenizer tokenizer = new StringTokenizer(largeString); String[] words = new String[tokenizer.countTokens()]; int index = 0; while (tokenizer.hasMoreTokens()) { words[index++] = tokenizer.nextToken(); }
In this example, a large string is split into tokens using the StringTokenizer class. The countTokens() method is used to determine the number of tokens, and a string array is created with the same size. The nextToken() method is called iteratively to extract each token and store it in the array.
Advanced Technique: Custom Delimiters with split()
In some cases, the split() method’s default behavior might not be sufficient. We can use regular expressions to define custom delimiters for splitting strings into arrays.
Related Article: How To Fix Java Certification Path Error
Code Snippet: Conversion with Custom Delimiter
String text = "Lorem ipsum dolor-sit_amet,consectetur.adipiscing-elit"; String[] tokens = text.split("[-_,.]");
In this example, the string “Lorem ipsum dolor-sit_amet,consectetur.adipiscing-elit” is split into an array of tokens using the regular expression “[-_,.]”, which matches any dash, underscore, comma, or period character. The resulting array, tokens
, will contain each token from the text as a separate element.
Code Snippet: Handling Escape Characters in Custom Delimiter
String text = "Lorem ipsum dolor\\-sit_amet,consectetur.adipiscing\\-elit"; String[] tokens = text.split("(?<!\\\\)[-_,.]");
In this example, the string “Lorem ipsum dolor\-sit_amet,consectetur.adipiscing\-elit” contains escape characters before the dash (“-“) in order to treat it as a literal character. The regular expression “(?Advanced Technique: Use of Pattern Class
The Pattern class in Java provides more advanced capabilities for working with regular expressions. We can use it in combination with the Matcher class to perform more complex string-to-array conversions.
Code Snippet: Conversion with Pattern and Matcher
import java.util.regex.Matcher; import java.util.regex.Pattern; String text = "Lorem ipsum dolor sit amet, consectetur adipiscing elit."; Pattern pattern = Pattern.compile("\\b\\w{5}\\b"); Matcher matcher = pattern.matcher(text); List<String> words = new ArrayList<>(); while (matcher.find()) { words.add(matcher.group()); } String[] wordArray = words.toArray(new String[0]);
In this example, the Pattern class is used to define a regular expression that matches words with exactly five characters. The Matcher class is used to find all matches of this pattern in the text. The matches are stored in an ArrayList, which is then converted into an array of strings using the toArray() method.
Related Article: How to Implement a Strategy Design Pattern in Java
Error Handling: Dealing with Null Strings
When working with string-to-array conversions, it’s important to handle null strings properly to avoid NullPointerExceptions. Let’s explore how to handle null strings in a safe manner.
Code Snippet: Handling Null Strings
String str = null; String[] array; if (str == null) { array = new String[0]; } else { array = str.split(","); }
In this example, the string str
is checked for nullity. If it is null, an empty array is created. Otherwise, the string is split into an array using the split() method.
Error Handling: Handling Invalid Delimiters
When converting a string to an array, it’s crucial to handle cases where the delimiter is not present or is invalid. Let’s explore how to handle such scenarios.
Code Snippet: Handling Invalid Delimiter
String str = "apple,banana,orange"; String delimiter = "."; String[] array; if (str.contains(delimiter)) { array = str.split(delimiter); } else { array = new String[]{str}; }
In this example, the string str
is checked for the presence of the delimiter “.”. If the delimiter is found, the string is split into an array using the split() method. Otherwise, the string is assigned as the only element of a new array.