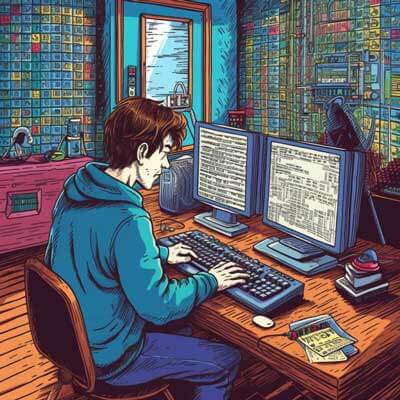
- Code Snippet – Loading Single Elements from Java Arrays
- Code Snippet – Loading Single Elements from Java Linked Lists
- Code Snippet – Loading Single Elements from Java Stacks
- Code Snippet – Loading Single Elements from Java Queues
- Code Snippet – Loading Single Elements from Java Hash Tables
- Code Snippet – Loading Single Elements from Java Trees
- Code Snippet – Loading Single Elements from Java Graphs
- Code Snippet – Loading Single Elements from Java Sets
- Code Snippet – Loading Single Elements from Java Maps
- How to Implement a Data Structure in Java
- Different Types of Data Structures in Java
- How to Load Data into a Java Array
- How to Load Data into a Java Linked List
- How to Load Data into a Java Stack
- How to Load Data into a Java Queue
- How to Load Data into a Java Hash Table
- How to Load Data into a Java Tree
- How to Load Data into a Java Graph
- How to Load Data into a Java Set
- How to Load Data into a Java Map
- Additional Resources
Java provides a wide range of data structures to store and manipulate data efficiently. These data structures include arrays, linked lists, stacks, queues, hash tables, trees, graphs, sets, and maps. In this article, we will explore how to load single elements from these Java data structures.
Code Snippet – Loading Single Elements from Java Arrays
Arrays are a fundamental data structure in Java that allows you to store a fixed-size sequence of elements of the same type. To load a single element from a Java array, you can use the index of the element.
Here’s an example of loading a single element from a Java array:
int[] numbers = {1, 2, 3, 4, 5}; int element = numbers[2]; // Load the element at index 2 System.out.println(element); // Output: 3
In this example, we have an array of integers called numbers
. We use the index 2
to load the element at that position, which is 3
. We then print the loaded element, which outputs 3
.
You can also load single elements from arrays of other types, such as strings or objects, using the same approach.
String[] names = {"Alice", "Bob", "Charlie"}; String element = names[1]; // Load the element at index 1 System.out.println(element); // Output: Bob
In this example, we have an array of strings called names
. We use the index 1
to load the element at that position, which is "Bob"
. We then print the loaded element, which outputs "Bob"
.
Related Article: How to Convert List to Array in Java
Code Snippet – Loading Single Elements from Java Linked Lists
Linked lists are a dynamic data structure in Java that allows you to store a variable-size sequence of elements. Each element in a linked list is represented by a node, which contains the data and a reference to the next node.
To load a single element from a Java linked list, you can traverse the list starting from the head node until you reach the desired position.
Here’s an example of loading a single element from a Java linked list:
LinkedList names = new LinkedList(); names.add("Alice"); names.add("Bob"); names.add("Charlie"); String element = names.get(1); // Load the element at index 1 System.out.println(element); // Output: Bob
In this example, we create a linked list of strings called names
. We add three elements to the list: "Alice"
, "Bob"
, and "Charlie"
. We use the get()
method with index 1
to load the element at that position, which is "Bob"
. We then print the loaded element, which outputs "Bob"
.
You can also load single elements from linked lists of other types, such as integers or objects, using the same approach.
LinkedList numbers = new LinkedList(); numbers.add(1); numbers.add(2); numbers.add(3); int element = numbers.get(2); // Load the element at index 2 System.out.println(element); // Output: 3
In this example, we create a linked list of integers called numbers
. We add three elements to the list: 1
, 2
, and 3
. We use the get()
method with index 2
to load the element at that position, which is 3
. We then print the loaded element, which outputs 3
.
Code Snippet – Loading Single Elements from Java Stacks
Stacks are a data structure in Java that follows the Last-In-First-Out (LIFO) principle. Elements are added and removed from the top of the stack. To load a single element from a Java stack, you can use the peek()
method.
Here’s an example of loading a single element from a Java stack:
Stack names = new Stack(); names.push("Alice"); names.push("Bob"); names.push("Charlie"); String element = names.peek(); // Load the top element System.out.println(element); // Output: Charlie
In this example, we create a stack of strings called names
. We push three elements onto the stack: "Alice"
, "Bob"
, and "Charlie"
. We use the peek()
method to load the top element of the stack, which is "Charlie"
. We then print the loaded element, which outputs "Charlie"
.
You can also load single elements from stacks of other types, such as integers or objects, using the same approach.
Stack numbers = new Stack(); numbers.push(1); numbers.push(2); numbers.push(3); int element = numbers.peek(); // Load the top element System.out.println(element); // Output: 3
In this example, we create a stack of integers called numbers
. We push three elements onto the stack: 1
, 2
, and 3
. We use the peek()
method to load the top element of the stack, which is 3
. We then print the loaded element, which outputs 3
.
Code Snippet – Loading Single Elements from Java Queues
Queues are a data structure in Java that follows the First-In-First-Out (FIFO) principle. Elements are added at the rear and removed from the front of the queue. To load a single element from a Java queue, you can use the peek()
method.
Here’s an example of loading a single element from a Java queue:
Queue names = new LinkedList(); names.add("Alice"); names.add("Bob"); names.add("Charlie"); String element = names.peek(); // Load the front element System.out.println(element); // Output: Alice
In this example, we create a queue of strings called names
. We add three elements to the queue: "Alice"
, "Bob"
, and "Charlie"
. We use the peek()
method to load the front element of the queue, which is "Alice"
. We then print the loaded element, which outputs "Alice"
.
You can also load single elements from queues of other types, such as integers or objects, using the same approach.
Queue numbers = new LinkedList(); numbers.add(1); numbers.add(2); numbers.add(3); int element = numbers.peek(); // Load the front element System.out.println(element); // Output: 1
In this example, we create a queue of integers called numbers
. We add three elements to the queue: 1
, 2
, and 3
. We use the peek()
method to load the front element of the queue, which is 1
. We then print the loaded element, which outputs 1
.
Related Article: Tutorial: Sorted Data Structure Storage in Java
Code Snippet – Loading Single Elements from Java Hash Tables
Hash tables, also known as hash maps, are a data structure in Java that allows you to store key-value pairs. To load a single element from a Java hash table, you can use the get()
method with the key.
Here’s an example of loading a single element from a Java hash table:
HashMap ages = new HashMap(); ages.put("Alice", 25); ages.put("Bob", 30); ages.put("Charlie", 35); int element = ages.get("Bob"); // Load the element with key "Bob" System.out.println(element); // Output: 30
In this example, we create a hash table of strings as keys and integers as values called ages
. We put three key-value pairs into the hash table: "Alice" - 25
, "Bob" - 30
, and "Charlie" - 35
. We use the get()
method with the key "Bob"
to load the corresponding value, which is 30
. We then print the loaded element, which outputs 30
.
You can also load single elements from hash tables with keys and values of other types, using the same approach.
HashMap cities = new HashMap(); cities.put(1, "New York"); cities.put(2, "London"); cities.put(3, "Tokyo"); String element = cities.get(3); // Load the element with key 3 System.out.println(element); // Output: Tokyo
In this example, we create a hash table of integers as keys and strings as values called cities
. We put three key-value pairs into the hash table: 1 - "New York"
, 2 - "London"
, and 3 - "Tokyo"
. We use the get()
method with the key 3
to load the corresponding value, which is "Tokyo"
. We then print the loaded element, which outputs "Tokyo"
.
Code Snippet – Loading Single Elements from Java Trees
Trees are hierarchical data structures in Java that allow you to store elements in a hierarchical manner. To load a single element from a Java tree, you can use traversal algorithms such as depth-first search (DFS) or breadth-first search (BFS).
Here’s an example of loading a single element from a Java tree:
class TreeNode { int val; TreeNode left; TreeNode right; TreeNode(int val) { this.val = val; } } TreeNode root = new TreeNode(1); root.left = new TreeNode(2); root.right = new TreeNode(3); int element = root.val; // Load the element at the root System.out.println(element); // Output: 1
In this example, we create a tree with three nodes: a root node with value 1
, a left child node with value 2
, and a right child node with value 3
. We load the element at the root, which is 1
, and print it, resulting in the output 1
.
You can also load single elements from trees with more complex structures, using traversal algorithms such as DFS or BFS.
class TreeNode { int val; TreeNode left; TreeNode right; TreeNode(int val) { this.val = val; } } TreeNode root = new TreeNode(1); root.left = new TreeNode(2); root.right = new TreeNode(3); root.left.left = new TreeNode(4); root.left.right = new TreeNode(5); int element = root.left.right.val; // Load the element at root->left->right System.out.println(element); // Output: 5
In this example, we create a tree with five nodes. We load the element at the path root->left->right
, which is 5
, and print it, resulting in the output 5
.
Code Snippet – Loading Single Elements from Java Graphs
Graphs are a data structure in Java that represent a collection of nodes (vertices) and their connections (edges). To load a single element from a Java graph, you can use graph traversal algorithms such as depth-first search (DFS) or breadth-first search (BFS).
Here’s an example of loading a single element from a Java graph:
class Graph { int V; LinkedList[] adjList; Graph(int V) { this.V = V; adjList = new LinkedList[V]; for (int i = 0; i < V; i++) { adjList[i] = new LinkedList(); } } void addEdge(int src, int dest) { adjList[src].add(dest); } } Graph graph = new Graph(4); graph.addEdge(0, 1); graph.addEdge(0, 2); graph.addEdge(1, 2); graph.addEdge(2, 0); graph.addEdge(2, 3); graph.addEdge(3, 3); int element = graph.adjList[0].get(1); // Load the element at index 1 of the adjacency list of vertex 0 System.out.println(element); // Output: 2
In this example, we create a graph with four vertices and add six edges between them. We load the element at index 1
of the adjacency list of vertex 0
, which is 2
, and print it, resulting in the output 2
.
You can also load single elements from graphs with more complex structures, using graph traversal algorithms such as DFS or BFS.
class Graph { int V; LinkedList[] adjList; Graph(int V) { this.V = V; adjList = new LinkedList[V]; for (int i = 0; i < V; i++) { adjList[i] = new LinkedList(); } } void addEdge(int src, int dest) { adjList[src].add(dest); } } Graph graph = new Graph(5); graph.addEdge(0, 1); graph.addEdge(0, 2); graph.addEdge(1, 2); graph.addEdge(2, 0); graph.addEdge(2, 3); graph.addEdge(3, 3); graph.addEdge(4, 2); int element = graph.adjList[4].get(0); // Load the element at index 0 of the adjacency list of vertex 4 System.out.println(element); // Output: 2
In this example, we create a graph with five vertices and add seven edges between them. We load the element at index 0
of the adjacency list of vertex 4
, which is 2
, and print it, resulting in the output 2
.
Related Article: Tutorial: Enumeration Types and Data Structures in Java
Code Snippet – Loading Single Elements from Java Sets
Sets are a data structure in Java that allows you to store a collection of unique elements. To load a single element from a Java set, you can use the iterator()
method or the stream()
method.
Here’s an example of loading a single element from a Java set using the iterator()
method:
Set names = new HashSet(); names.add("Alice"); names.add("Bob"); names.add("Charlie"); Iterator iterator = names.iterator(); String element = iterator.next(); // Load the next element System.out.println(element); // Output: Alice
In this example, we create a set of strings called names
. We add three elements to the set: "Alice"
, "Bob"
, and "Charlie"
. We obtain an iterator from the set and use the next()
method to load the next element, which is "Alice"
. We then print the loaded element, which outputs "Alice"
.
You can also load single elements from sets of other types, such as integers or objects, using the same approach.
Set numbers = new HashSet(); numbers.add(1); numbers.add(2); numbers.add(3); Iterator iterator = numbers.iterator(); int element = iterator.next(); // Load the next element System.out.println(element); // Output: 1
In this example, we create a set of integers called numbers
. We add three elements to the set: 1
, 2
, and 3
. We obtain an iterator from the set and use the next()
method to load the next element, which is 1
. We then print the loaded element, which outputs 1
.
Alternatively, you can use the stream()
method to load a single element from a Java set.
Set names = new HashSet(); names.add("Alice"); names.add("Bob"); names.add("Charlie"); String element = names.stream().findFirst().orElse(null); // Load the first element System.out.println(element); // Output: Alice
In this example, we create a set of strings called names
. We add three elements to the set: "Alice"
, "Bob"
, and "Charlie"
. We use the stream()
method to obtain a stream of elements from the set, and then use the findFirst()
method to load the first element, which is "Alice"
. We then print the loaded element, which outputs "Alice"
.
Code Snippet – Loading Single Elements from Java Maps
Maps are a data structure in Java that allows you to store key-value pairs. To load a single element from a Java map, you can use the get()
method with the key.
Here’s an example of loading a single element from a Java map:
Map ages = new HashMap(); ages.put("Alice", 25); ages.put("Bob", 30); ages.put("Charlie", 35); int element = ages.get("Bob"); // Load the element with key "Bob" System.out.println(element); // Output: 30
In this example, we create a map with strings as keys and integers as values called ages
. We put three key-value pairs into the map: "Alice" - 25
, "Bob" - 30
, and "Charlie" - 35
. We use the get()
method with the key "Bob"
to load the corresponding value, which is 30
. We then print the loaded element, which outputs 30
.
You can also load single elements from maps with keys and values of other types, using the same approach.
Map cities = new HashMap(); cities.put(1, "New York"); cities.put(2, "London"); cities.put(3, "Tokyo"); String element = cities.get(3); // Load the element with key 3 System.out.println(element); // Output: Tokyo
In this example, we create a map with integers as keys and strings as values called cities
. We put three key-value pairs into the map: 1 - "New York"
, 2 - "London"
, and 3 - "Tokyo"
. We use the get()
method with the key 3
to load the corresponding value, which is "Tokyo"
. We then print the loaded element, which outputs "Tokyo"
.
How to Implement a Data Structure in Java
To implement a data structure in Java, you need to define a class or use one of the existing data structure classes provided by the Java API. Here are the steps to implement a data structure in Java:
1. Determine the requirements and functionality of the data structure you want to implement. Consider factors such as the type of data to be stored, the operations you want to perform on the data structure, and the expected performance characteristics.
2. Choose an appropriate data structure class from the Java API or design your own class if necessary. The Java API provides a wide range of data structure classes, including arrays, linked lists, stacks, queues, hash tables, trees, graphs, sets, and maps.
3. Define the necessary data fields and methods for your data structure class. Data fields represent the state or properties of the data structure, while methods define the behavior or operations that can be performed on the data structure.
4. Implement the methods of your data structure class according to the desired functionality. This may involve manipulating the data fields, performing calculations, or calling other methods.
5. Test your data structure implementation by creating instances of the class and invoking its methods with different inputs and scenarios. Verify that the data structure behaves as expected and produces the correct results.
6. Consider performance optimizations if necessary. Depending on the complexity and size of your data structure, you may need to optimize certain operations to ensure efficient data storage and retrieval.
7. Document your data structure implementation by providing clear and concise comments in the code. Explain the purpose, functionality, and usage of each data field and method to make it easier for other developers to understand and use your data structure.
Related Article: How to Initialize an ArrayList in One Line in Java
Different Types of Data Structures in Java
Java provides a variety of data structures that are designed to store and organize data in different ways. Each data structure has its own strengths and weaknesses, making it suitable for specific use cases. Here are some of the different types of data structures available in Java:
1. Arrays: Arrays are a fundamental data structure in Java that allow you to store a fixed-size sequence of elements of the same type. Arrays provide direct access to elements using an index.
2. Linked Lists: Linked lists are dynamic data structures in Java that allow you to store a variable-size sequence of elements. Each element in a linked list is represented by a node, which contains the data and a reference to the next node.
3. Stacks: Stacks are data structures in Java that follow the Last-In-First-Out (LIFO) principle. Elements are added and removed from the top of the stack.
4. Queues: Queues are data structures in Java that follow the First-In-First-Out (FIFO) principle. Elements are added at the rear and removed from the front of the queue.
5. Hash Tables: Hash tables, also known as hash maps, are data structures in Java that allow you to store key-value pairs. Hash tables use a hash function to map keys to their corresponding values.
6. Trees: Trees are hierarchical data structures in Java that allow you to store elements in a hierarchical manner. Each element in a tree is represented by a node, which may have one or more child nodes.
7. Graphs: Graphs are data structures in Java that represent a collection of nodes (vertices) and their connections (edges). Graphs can be used to model relationships between entities.
8. Sets: Sets are data structures in Java that allow you to store a collection of unique elements. Sets do not allow duplicate elements.
9. Maps: Maps are data structures in Java that allow you to store key-value pairs. Maps provide efficient lookup and retrieval of values based on their associated keys.
Each type of data structure has its own advantages and use cases. By understanding the characteristics and functionality of these data structures, you can choose the most appropriate one for your specific needs.
How to Load Data into a Java Array
Loading data into a Java array involves initializing the array and assigning values to its elements. Here’s how you can load data into a Java array:
1. Declare the array variable and specify its type and size. For example, to create an array of integers with a size of 5, use the following code:
int[] numbers = new int[5];
2. Assign values to the elements of the array using the assignment operator (=
). You can do this individually for each element, or use a loop to assign values to multiple elements. For example:
numbers[0] = 1; numbers[1] = 2; numbers[2] = 3; numbers[3] = 4; numbers[4] = 5;
Alternatively, you can initialize the array with values directly at the time of declaration. For example:
int[] numbers = {1, 2, 3, 4, 5};
3. Access and use the values stored in the array using the index of the element. For example, to print the value of the third element in the array, use the following code:
System.out.println(numbers[2]); // Output: 3
In this example, we create an array of integers called numbers
with a size of 5. We assign values to the elements of the array starting from index 0. We then access and print the value of the third element, which is 3.
You can load data of different types, such as strings or objects, into arrays using the same approach. Just make sure to specify the appropriate type when declaring the array and assign values of the corresponding type to its elements.
How to Load Data into a Java Linked List
Loading data into a Java linked list involves creating a linked list object and adding elements to it. Here’s how you can load data into a Java linked list:
1. Import the LinkedList
class from the java.util
package. This class provides the implementation of a linked list in Java.
import java.util.LinkedList;
2. Create an instance of the LinkedList
class, specifying the type of elements you want to store in the linked list. For example, to create a linked list of strings, use the following code:
LinkedList names = new LinkedList();
3. Use the add()
method to add elements to the linked list. You can add elements individually or in bulk using the addAll()
method. For example:
names.add("Alice"); names.add("Bob"); names.add("Charlie");
Alternatively, you can initialize the linked list with values directly at the time of declaration. For example:
LinkedList names = new LinkedList(Arrays.asList("Alice", "Bob", "Charlie"));
4. Access and use the values stored in the linked list using the get()
method with the index of the element. For example, to print the value of the second element in the linked list, use the following code:
System.out.println(names.get(1)); // Output: Bob
In this example, we create a linked list of strings called names
. We add three elements to the linked list using the add()
method. We then access and print the value of the second element using the get()
method, which outputs "Bob"
.
You can load data of different types, such as integers or objects, into linked lists using the same approach. Just make sure to specify the appropriate type when creating the linked list and add values of the corresponding type to it.
Related Article: How to Easily Print a Java Array
How to Load Data into a Java Stack
Loading data into a Java stack involves creating a stack object and pushing elements onto it. Here’s how you can load data into a Java stack:
1. Import the Stack
class from the java.util
package. This class provides the implementation of a stack in Java.
import java.util.Stack;
2. Create an instance of the Stack
class, specifying the type of elements you want to store in the stack. For example, to create a stack of integers, use the following code:
Stack numbers = new Stack();
3. Use the push()
method to add elements to the stack. For example:
numbers.push(1); numbers.push(2); numbers.push(3);
4. Access and use the values stored in the stack using the peek()
method, which retrieves the top element without removing it. For example, to print the value of the top element in the stack, use the following code:
System.out.println(numbers.peek()); // Output: 3
In this example, we create a stack of integers called numbers
. We push three elements onto the stack using the push()
method. We then access and print the value of the top element using the peek()
method, which outputs 3
.
You can load data of different types, such as strings or objects, into stacks using the same approach. Just make sure to specify the appropriate type when creating the stack and push values of the corresponding type onto it.
How to Load Data into a Java Queue
Loading data into a Java queue involves creating a queue object and adding elements to it. Here’s how you can load data into a Java queue:
1. Import the Queue
interface and choose one of its implementing classes, such as LinkedList
or ArrayDeque
, from the java.util
package. These classes provide the implementation of a queue in Java.
import java.util.Queue; import java.util.LinkedList;
2. Create an instance of the chosen queue class, specifying the type of elements you want to store in the queue. For example, to create a queue of strings, use the following code:
Queue names = new LinkedList();
3. Use the add()
or offer()
method to add elements to the queue. Both methods add an element to the rear of the queue. For example:
names.add("Alice"); names.add("Bob"); names.add("Charlie");
Alternatively, you can initialize the queue with values directly at the time of declaration. For example:
Queue names = new LinkedList(Arrays.asList("Alice", "Bob", "Charlie"));
4. Access and use the values stored in the queue using the peek()
method, which retrieves the front element without removing it. For example, to print the value of the front element in the queue, use the following code:
System.out.println(names.peek()); // Output: Alice
In this example, we create a queue of strings called names
. We add three elements to the queue using the add()
method. We then access and print the value of the front element using the peek()
method, which outputs "Alice"
.
You can load data of different types, such as integers or objects, into queues using the same approach. Just make sure to specify the appropriate type when creating the queue and add values of the corresponding type to it.
How to Load Data into a Java Hash Table
Loading data into a Java hash table involves creating a hash table object and putting key-value pairs into it. Here’s how you can load data into a Java hash table:
1. Import the HashMap
class from the java.util
package. This class provides the implementation of a hash table in Java.
import java.util.HashMap;
2. Create an instance of the HashMap
class, specifying the types of keys and values you want to store in the hash table. For example, to create a hash table with strings as keys and integers as values, use the following code:
HashMap ages = new HashMap();
3. Use the put()
method to add key-value pairs to the hash table. For example:
ages.put("Alice", 25); ages.put("Bob", 30); ages.put("Charlie", 35);
4. Access and use the values stored in the hash table using the get()
method with the key. For example, to print the value associated with the key "Bob"
, use the following code:
System.out.println(ages.get("Bob")); // Output: 30
In this example, we create a hash table with strings as keys and integers as values called ages
. We put three key-value pairs into the hash table using the put()
method. We then access and print the value associated with the key "Bob"
using the get()
method, which outputs 30
.
You can load data of different types, such as integers or objects, into hash tables using the same approach. Just make sure to specify the appropriate types when creating the hash table and put key-value pairs of the corresponding types into it.
Related Article: How to Sort a List of ArrayList in Java
How to Load Data into a Java Tree
Loading data into a Java tree involves creating a tree object and adding elements to it. Here’s how you can load data into a Java tree:
1. Define a class for the tree nodes. Each node should contain a value and references to its child nodes. For example:
class TreeNode { int val; TreeNode left; TreeNode right; TreeNode(int val) { this.val = val; } }
2. Create an instance of the TreeNode
class for the root node, specifying its value. For example, to create a tree with a root node containing the value 1
, use the following code:
TreeNode root = new TreeNode(1);
3. Create instances of the TreeNode
class for the child nodes and assign them to the appropriate references. For example, to add left and right child nodes with values 2
and 3
to the root node, use the following code:
root.left = new TreeNode(2); root.right = new TreeNode(3);
4. Access and use the values stored in the tree by traversing it using depth-first search (DFS) or breadth-first search (BFS) algorithms. For example, to print the value of the root node, use the following code:
System.out.println(root.val); // Output: 1
In this example, we create a tree with a root node containing the value 1
. We add left and right child nodes with values 2
and 3
to the root node. We then access and print the value of the root node, which outputs 1
.
You can load data into trees with more complex structures by adding additional child nodes to the existing nodes. Just make sure to assign the child nodes to the appropriate references to maintain the hierarchy of the tree.
How to Load Data into a Java Graph
Loading data into a Java graph involves creating a graph object and adding vertices and edges to it. Here’s how you can load data into a Java graph:
1. Define a class for the graph. The class should contain a number of vertices and an adjacency list to store the connections between vertices. For example:
class Graph { int V; // Number of vertices LinkedList[] adjList; // Adjacency list Graph(int V) { this.V = V; adjList = new LinkedList[V]; for (int i = 0; i < V; i++) { adjList[i] = new LinkedList(); } } void addEdge(int src, int dest) { adjList[src].add(dest); } }
2. Create an instance of the Graph
class, specifying the number of vertices in the graph. For example, to create a graph with four vertices, use the following code:
Graph graph = new Graph(4);
3. Use the addEdge()
method to add edges between vertices in the graph. For example, to add an edge between vertices 0
and 1
, use the following code:
graph.addEdge(0, 1);
4. Access and use the values stored in the graph by traversing it using graph traversal algorithms such as depth-first search (DFS) or breadth-first search (BFS). For example, to print the adjacency list of vertex 0
, use the following code:
System.out.println(graph.adjList[0]); // Output: [1]
In this example, we create a graph with four vertices using the Graph
class. We add an edge between vertices 0
and 1
using the addEdge()
method. We then access and print the adjacency list of vertex 0
, which outputs [1]
.
You can load data into graphs with more complex structures by adding additional edges between vertices. Just make sure to specify the source and destination vertices when adding edges to the graph.
How to Load Data into a Java Set
Loading data into a Java set involves creating a set object and adding elements to it. Here’s how you can load data into a Java set:
1. Import the Set
interface and choose one of its implementing classes, such as HashSet
or TreeSet
, from the java.util
package. These classes provide the implementation of a set in Java.
import java.util.Set; import java.util.HashSet;
2. Create an instance of the chosen set class, specifying the type of elements you want to store in the set. For example, to create a set of strings, use the following code:
Set names = new HashSet();
3. Use the add()
method to add elements to the set. For example:
names.add("Alice"); names.add("Bob"); names.add("Charlie");
Alternatively, you can initialize the set with values directly at the time of declaration. For example:
Set names = new HashSet(Arrays.asList("Alice", "Bob", "Charlie"));
4. Access and use the values stored in the set using iteration or stream operations. For example, to print all the elements in the set, use the following code:
for (String name : names) { System.out.println(name); }
In this example, we create a set of strings called names
. We add three elements to the set using the add()
method. We then iterate over the set using a for-each loop and print each element.
You can load data of different types, such as integers or objects, into sets using the same approach. Just make sure to specify the appropriate type when creating the set and add values of the corresponding type to it.
Related Article: How to Declare and Initialize a New Array in Java
How to Load Data into a Java Map
Loading data into a Java map involves creating a map object and putting key-value pairs into it. Here’s how you can load data into a Java map:
1. Import the Map
interface and choose one of its implementing classes, such as HashMap
or TreeMap
, from the java.util
package. These classes provide the implementation of a map in Java.
import java.util.Map; import java.util.HashMap;
2. Create an instance of the chosen map class, specifying the types of keys and values you want to store in the map. For example, to create a map with strings as keys and integers as values, use the following code:
Map ages = new HashMap();
3. Use the put()
method to add key-value pairs to the map. For example:
ages.put("Alice", 25); ages.put("Bob", 30); ages.put("Charlie", 35);
4. Access and use the values stored in the map using the get()
method with the key. For example, to print the value associated with the key "Bob"
, use the following code:
System.out.println(ages.get("Bob")); // Output: 30
In this example, we create a map with strings as keys and integers as values called ages
. We put three key-value pairs into the map using the put()
method. We then access and print the value associated with the key "Bob"
using the get()
method, which outputs 30
.
You can load data of different types, such as integers or objects, into maps using the same approach. Just make sure to specify the appropriate types when creating the map and put key-value pairs of the corresponding types into it.
Additional Resources
– Oracle Java Documentation – Data Structures
– GeeksforGeeks – Data Structures in Java
– Tutorialspoint – Java Data Structures