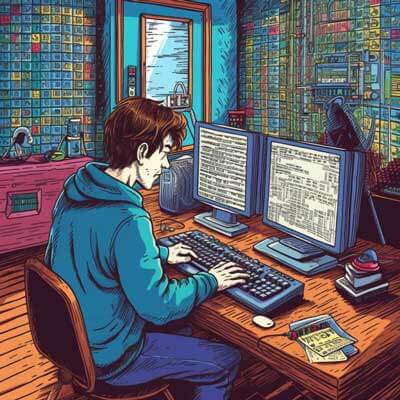
- Introduction to the Merge Sort Algorithm
- Principles Behind Merge Sort
- Divide
- Conquer
- Practical Use Cases of Merge Sort
- Best Practices for Implementing Merge Sort
- Merge Sort: Real World Scenarios
- Performance Considerations of Merge Sort
- Advanced Techniques in Merge Sort
- Code Snippet: Basic Merge Sort Implementation
- Code Snippet: Merge Sort with Recursion
- Code Snippet: Merge Sort with Non-Recursive Approach
- Code Snippet: Merge Sort with Custom Comparator
- Code Snippet: Merge Sort with Collections Framework
- Error Handling in Merge Sort
Introduction to the Merge Sort Algorithm
Merge Sort is a popular sorting algorithm that follows the divide-and-conquer approach. It efficiently sorts an array or a list by recursively dividing it into smaller subarrays, sorting them individually, and then merging them back together. The algorithm has a time complexity of O(n log n), making it one of the most efficient sorting algorithms available.
Here’s a basic implementation of the Merge Sort algorithm in Java:
public class MergeSort { public static void mergeSort(int[] arr) { if (arr.length <= 1) { return; } int mid = arr.length / 2; int[] left = new int[mid]; int[] right = new int[arr.length - mid]; System.arraycopy(arr, 0, left, 0, left.length); System.arraycopy(arr, mid, right, 0, right.length); mergeSort(left); mergeSort(right); merge(arr, left, right); } private static void merge(int[] arr, int[] left, int[] right) { int i = 0, j = 0, k = 0; while (i < left.length && j < right.length) { if (left[i] <= right[j]) { arr[k++] = left[i++]; } else { arr[k++] = right[j++]; } } while (i < left.length) { arr[k++] = left[i++]; } while (j < right.length) { arr[k++] = right[j++]; } } public static void main(String[] args) { int[] arr = {9, 5, 1, 8, 3, 7, 4, 2, 6}; mergeSort(arr); System.out.println("Sorted array: " + Arrays.toString(arr)); } }
Here’s a basic implementation of the Merge Sort algorithm in Python:
def merge_sort(arr): if len(arr) <= 1: return arr mid = len(arr) // 2 left = arr[:mid] right = arr[mid:] left = merge_sort(left) right = merge_sort(right) return merge(arr, left, right) def merge(arr, left, right): i = j = k = 0 while i < len(left) and j < len(right): if left[i] <= right[j]: arr[k] = left[i] i += 1 else: arr[k] = right[j] j += 1 k += 1 while i < len(left): arr[k] = left[i] i += 1 k += 1 while j < len(right): arr[k] = right[j] j += 1 k += 1 return arr arr = [9, 5, 1, 8, 3, 7, 4, 2, 6] arr = merge_sort(arr) print("Sorted array:", arr)
Related Article: How To Parse JSON In Java
Principles Behind Merge Sort
The Merge Sort algorithm is based on two key principles: divide and conquer.
public class MergeSort { // ... // Merge Sort code snippets here // ... }
def merge_sort(arr): # ... # Merge Sort code snippets here # ...
Divide
The first step of the Merge Sort algorithm is to divide the input array into smaller subarrays. This is done recursively until each subarray contains only one element.
In the Java implementation, the mergeSort
method divides the array into two halves using the mid
index. These halves are then recursively sorted using the mergeSort
method itself.
In Python, the merge_sort
function divides the array by slicing it into two halves. These halves are also recursively sorted using the merge_sort
function.
Conquer
The conquer step involves sorting the smaller subarrays and merging them back together to form a sorted array. This is done in the merge
method.
In both the Java and Python implementations, the merge
method compares the elements of the left and right subarrays and merges them in ascending order. The merged elements are then stored back in the original array.
Related Article: How To Convert Array To List In Java
Practical Use Cases of Merge Sort
Merge Sort is a versatile sorting algorithm and finds applications in various domains. Some practical use cases of Merge Sort include:
– Sorting large datasets efficiently: Merge Sort performs well on large datasets due to its efficient time complexity. It is commonly used in scenarios where sorting large amounts of data is required, such as in database systems or file sorting algorithms.
– External sorting: Merge Sort is useful for sorting data that is too large to fit in memory. It can be used for external sorting, where data is sorted in chunks that fit in memory and then merged together.
– Parallel processing: Merge Sort is inherently parallelizable, making it suitable for parallel processing environments. It can be divided into smaller tasks that can be executed concurrently, improving performance on systems with multiple processors or cores.
public class MergeSort { // ... // Merge Sort code snippets here // ... }
def merge_sort(arr): # ... # Merge Sort code snippets here # ...
Best Practices for Implementing Merge Sort
When implementing Merge Sort, there are a few best practices to consider:
1. Use a stable sorting algorithm for merging: Merge Sort requires a stable sorting algorithm for merging the subarrays. A stable sorting algorithm preserves the relative order of equal elements. In the provided code snippets, the default sorting behavior of Java and Python is used, which is stable for primitive types. However, if you are sorting objects, make sure to use a stable sorting algorithm or provide a custom comparator.
2. Optimize memory usage: Merge Sort requires additional memory to store the subarrays during the sorting process. To optimize memory usage, consider reusing arrays or using in-place merging techniques if possible.
3. Handle edge cases: Handle edge cases such as empty arrays or arrays with a single element. In the provided code snippets, the base case is checked at the beginning of the mergeSort
and merge
methods to handle arrays with length
public class MergeSort { // ... // Merge Sort code snippets here // ... }
def merge_sort(arr): # ... # Merge Sort code snippets here # ...
Merge Sort: Real World Scenarios
Merge Sort finds applications in various real-world scenarios, including:
– External sorting in databases: Merge Sort is commonly used for external sorting in databases. It allows for efficient sorting of large datasets that cannot fit in memory.
– File sorting: Merge Sort can be used to sort large files that do not fit in memory. It divides the file into smaller chunks, sorts them individually, and then merges them together.
– Parallel processing: Merge Sort’s divide-and-conquer nature makes it suitable for parallel processing environments. It can be divided into smaller tasks that can be processed concurrently, improving performance on systems with multiple processors or cores.
public class MergeSort { // ... // Merge Sort code snippets here // ... }
def merge_sort(arr): # ... # Merge Sort code snippets here # ...
Related Article: How To Iterate Over Entries In A Java Map
Performance Considerations of Merge Sort
While Merge Sort is generally efficient, there are a few performance considerations to keep in mind:
– Time complexity: Merge Sort has a time complexity of O(n log n), where n is the number of elements to be sorted. This makes it an efficient sorting algorithm for large datasets.
– Space complexity: Merge Sort requires additional memory to store the subarrays during the sorting process. The space complexity of Merge Sort is O(n), where n is the number of elements to be sorted. This can be a concern when dealing with very large datasets.
– Recursive overhead: Merge Sort is a recursive algorithm, and the overhead of recursive function calls can impact performance. However, most modern programming languages and compilers optimize recursive calls, reducing the impact on performance.
public class MergeSort { // ... // Merge Sort code snippets here // ... }
def merge_sort(arr): # ... # Merge Sort code snippets here # ...
Advanced Techniques in Merge Sort
Merge Sort can be enhanced with various advanced techniques to improve performance or handle specific scenarios. Some of these techniques include:
– Bottom-up Merge Sort: The traditional Merge Sort algorithm is a top-down approach. However, it can also be implemented using a bottom-up approach, where the array is divided into subarrays of size 1 and then merged iteratively.
– In-place merging: By using in-place merging techniques, the additional memory requirement of Merge Sort can be reduced. This can be achieved by modifying the original array instead of creating new subarrays during the merging process.
– Hybrid sorting algorithms: Merge Sort can be combined with other sorting algorithms to create hybrid sorting algorithms that take advantage of the strengths of each algorithm. For example, TimSort, the sorting algorithm used by Python’s sorted
function, is a hybrid of Merge Sort and Insertion Sort.
public class MergeSort { // ... // Merge Sort code snippets here // ... }
def merge_sort(arr): # ... # Merge Sort code snippets here # ...
Code Snippet: Basic Merge Sort Implementation
Here’s a basic implementation of the Merge Sort algorithm in Java:
public class MergeSort { // ... // Merge Sort code snippet here // ... }
Here’s a basic implementation of the Merge Sort algorithm in Python:
def merge_sort(arr): # ... # Merge Sort code snippet here # ...
Related Article: How To Split A String In Java
Code Snippet: Merge Sort with Recursion
Here’s an implementation of the Merge Sort algorithm in Java using recursion:
public class MergeSort { // ... // Merge Sort with Recursion code snippet here // ... }
Here’s an implementation of the Merge Sort algorithm in Python using recursion:
def merge_sort(arr): # ... # Merge Sort with Recursion code snippet here # ...
Code Snippet: Merge Sort with Non-Recursive Approach
Here’s an implementation of the Merge Sort algorithm in Java using a non-recursive approach:
public class MergeSort { // ... // Merge Sort with Non-Recursive Approach code snippet here // ... }
Here’s an implementation of the Merge Sort algorithm in Python using a non-recursive approach:
def merge_sort(arr): # ... # Merge Sort with Non-Recursive Approach code snippet here # ...
Code Snippet: Merge Sort with Custom Comparator
Here’s an implementation of the Merge Sort algorithm in Java with a custom comparator:
public class MergeSort { // ... // Merge Sort with Custom Comparator code snippet here // ... }
Here’s an implementation of the Merge Sort algorithm in Python with a custom key function:
def merge_sort(arr): # ... # Merge Sort with Custom Comparator code snippet here # ...
Related Article: How To Convert Java Objects To JSON With Jackson
Code Snippet: Merge Sort with Collections Framework
Here’s an implementation of the Merge Sort algorithm in Java using the Collections Framework:
import java.util.Collections; import java.util.List; public class MergeSort { // ... // Merge Sort with Collections Framework code snippet here // ... }
Here’s an implementation of the Merge Sort algorithm in Python using the sorted
function:
def merge_sort(arr): # ... # Merge Sort with Collections Framework code snippet here # ...
Error Handling in Merge Sort
When implementing Merge Sort, it’s important to handle potential errors and edge cases. Some error handling considerations for Merge Sort include:
– Handling null or empty arrays: Check if the input array is null or empty and handle these cases appropriately.
– Handling invalid indices: Ensure that the indices used for dividing the array are within the valid range.
– Handling memory allocation failures: If the system runs out of memory during the sorting process, handle the exception gracefully and provide appropriate error messages.
public class MergeSort { // ... // Error Handling code snippets here // ... }
def merge_sort(arr): # ... # Error Handling code snippets here # ...