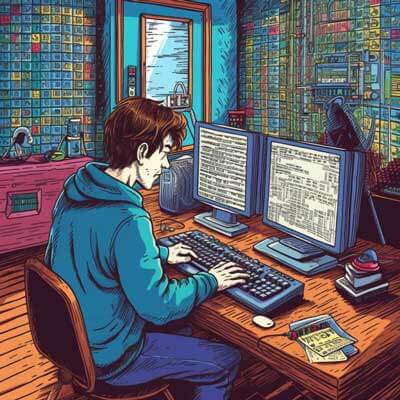
- Introduction to Interface
- Defining an Interface
- Code Snippet: Defining an Interface
- Implementing an Interface
- Code Snippet: Implementing an Interface
- Interface Variables
- Code Snippet: Using Interface Variables
- Interface Methods
- Code Snippet: Implementing Interface Methods
- Nested Interfaces
- Code Snippet: Using Nested Interfaces
- Functional Interfaces
- Code Snippet: Using Functional Interfaces
- Default Methods in Interfaces
- Code Snippet: Using Default Methods in Interface
- Static Methods in Interfaces
- Code Snippet: Using Static Methods in Interface
- Use Case: Interface for Sorting
- Code Snippet: Implementing Comparable Interface
- Use Case: Interface for Event Handling
- Best Practice: Avoiding Redundant Interface Methods
- Best Practice: Naming Conventions for Interfaces
- Performance: Interface versus Abstract Class
- Performance: Runtime Polymorphism with Interfaces
- Advanced Technique: Multiple Inheritance with Interfaces
- Advanced Technique: Adapter Pattern with Interfaces
- Error Handling: Exceptions in Interface Methods
- Code Snippet: Using Exceptions in Interface Methods
Introduction to Interface
In Java, an interface is a blueprint of a class that defines a set of methods that the implementing class must implement. It allows for the abstraction of behavior, allowing different classes to provide their own implementation while maintaining a common contract.
Interfaces are defined using the interface
keyword and can contain method signatures, constant variables, and nested types. They provide a way to achieve multiple inheritance-like behavior since a class can implement multiple interfaces.
Here’s an example of an interface declaration in Java:
public interface MyInterface { void doSomething(); int calculate(int a, int b); }
Related Article: How To Parse JSON In Java
Defining an Interface
To define an interface in Java, you use the interface
keyword followed by the interface name. Inside the interface, you can declare method signatures and constant variables.
Here’s an example of an interface declaration:
public interface MyInterface { void doSomething(); int calculate(int a, int b); }
In the above example, MyInterface
declares two methods: doSomething()
and calculate()
. These methods are not implemented in the interface but only provide a contract that implementing classes must fulfill.
Code Snippet: Defining an Interface
public interface MyInterface { void doSomething(); int calculate(int a, int b); }
Implementing an Interface
To implement an interface in Java, you use the implements
keyword followed by the interface name(s) that you want to implement. The implementing class must provide an implementation for all the methods declared in the interface.
Here’s an example of a class implementing an interface:
public class MyClass implements MyInterface { @Override public void doSomething() { // Implementation for doSomething() method } @Override public int calculate(int a, int b) { // Implementation for calculate() method return a + b; } }
In the above example, the class MyClass
implements the MyInterface
interface. It provides the implementation for the doSomething()
and calculate()
methods as required by the interface.
Related Article: How To Convert Array To List In Java
Code Snippet: Implementing an Interface
public class MyClass implements MyInterface { @Override public void doSomething() { // Implementation for doSomething() method } @Override public int calculate(int a, int b) { // Implementation for calculate() method return a + b; } }
Interface Variables
In Java, interfaces can also declare constant variables, which are implicitly public, static, and final. These variables can be accessed using the interface name followed by the variable name.
Here’s an example of an interface with a constant variable:
public interface MyInterface { int MAX_VALUE = 100; }
In the above example, the interface MyInterface
declares a constant variable MAX_VALUE
with a value of 100.
Code Snippet: Using Interface Variables
public class MyClass { public static void main(String[] args) { System.out.println(MyInterface.MAX_VALUE); // Output: 100 } }
Related Article: How To Iterate Over Entries In A Java Map
Interface Methods
Interface methods represent the behavior that implementing classes must define. These methods are declared in the interface without an implementation and must be implemented by the classes that implement the interface.
Here’s an example of an interface with a method:
public interface MyInterface { void doSomething(); }
In the above example, the interface MyInterface
declares a method doSomething()
. Any class implementing this interface must provide an implementation for this method.
Code Snippet: Implementing Interface Methods
public class MyClass implements MyInterface { @Override public void doSomething() { // Implementation for doSomething() method } }
Nested Interfaces
In Java, interfaces can also be nested within other interfaces or classes. A nested interface is implicitly static, and its name must be qualified with the outer interface or class name.
Here’s an example of a nested interface:
public interface MyInterface { void doSomething(); interface NestedInterface { void doNestedSomething(); } }
In the above example, the interface MyInterface
contains a nested interface NestedInterface
with its own set of methods. The nested interface can be accessed using the qualified name MyInterface.NestedInterface
.
Related Article: How To Split A String In Java
Code Snippet: Using Nested Interfaces
public class MyClass implements MyInterface.NestedInterface { @Override public void doNestedSomething() { // Implementation for doNestedSomething() method } }
Functional Interfaces
Functional interfaces are interfaces that contain only one abstract method. They are often used with lambda expressions and method references for functional programming in Java.
Here’s an example of a functional interface:
@FunctionalInterface public interface MyInterface { void doSomething(); }
In the above example, the @FunctionalInterface
annotation is used to indicate that the interface is intended to be used as a functional interface. It helps enforce the single abstract method requirement.
Code Snippet: Using Functional Interfaces
public class MyClass { public static void main(String[] args) { MyInterface myInterface = () -> { // Implementation for doSomething() method }; myInterface.doSomething(); } }
Related Article: How To Convert Java Objects To JSON With Jackson
Default Methods in Interfaces
Default methods were introduced in Java 8 to provide a way to add new functionality to existing interfaces without breaking backward compatibility. A default method is a method with an implementation in the interface itself.
Here’s an example of a default method in an interface:
public interface MyInterface { void doSomething(); default void doSomethingElse() { // Default implementation for doSomethingElse() method } }
In the above example, the interface MyInterface
declares a default method doSomethingElse()
. Implementing classes can choose to override this default implementation if needed.
Code Snippet: Using Default Methods in Interface
public class MyClass implements MyInterface { @Override public void doSomething() { // Implementation for doSomething() method } @Override public void doSomethingElse() { // Custom implementation for doSomethingElse() method } }
Static Methods in Interfaces
Static methods in interfaces were introduced in Java 8 to provide utility methods that can be called directly on the interface without the need for an implementing class instance.
Here’s an example of a static method in an interface:
public interface MyInterface { void doSomething(); static void doStaticSomething() { // Implementation for doStaticSomething() method } }
In the above example, the interface MyInterface
declares a static method doStaticSomething()
. The static method can be called using the interface name, like MyInterface.doStaticSomething()
.
Related Article: Storing Contact Information in Java Data Structures
Code Snippet: Using Static Methods in Interface
public class MyClass { public static void main(String[] args) { MyInterface.doStaticSomething(); } }
Use Case: Interface for Sorting
Interfaces are commonly used to define contracts for sorting operations. For example, the Comparable
interface is used to provide a natural ordering for objects.
Here’s an example of using the Comparable
interface to sort objects:
public class MyClass implements Comparable<MyClass> { private int value; public MyClass(int value) { this.value = value; } public int getValue() { return value; } @Override public int compareTo(MyClass other) { return Integer.compare(this.value, other.value); } }
In the above example, the class MyClass
implements the Comparable
interface, which requires the implementation of the compareTo()
method. This method defines the natural ordering of MyClass
objects based on their value
property.
Code Snippet: Implementing Comparable Interface
public class MyClass implements Comparable<MyClass> { private int value; public MyClass(int value) { this.value = value; } public int getValue() { return value; } @Override public int compareTo(MyClass other) { return Integer.compare(this.value, other.value); } }
Related Article: How to Convert JSON String to Java Object
Use Case: Interface for Event Handling
Interfaces are also commonly used for event handling in graphical user interfaces (GUI). For example, the ActionListener
interface is used to handle button clicks.
Here’s an example of using the ActionListener
interface for event handling:
import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.JButton; public class MyButton { private JButton button; public MyButton() { button = new JButton("Click Me"); button.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { // Handle button click event } }); } }
In the above example, the MyButton
class creates a button and attaches an anonymous class implementing the ActionListener
interface to handle the button click event.
Best Practice: Avoiding Redundant Interface Methods
When designing interfaces, it is important to avoid redundant methods that serve no purpose or have duplicate functionality. Redundant methods can lead to confusion and code duplication.
For example, consider an interface that declares two methods with similar functionality:
public interface MyInterface { void doSomething(); void doSomethingElse(); // Redundant method with similar functionality as doSomething() void doAnotherThing(); }
In the above example, the doAnotherThing()
method is redundant because it has similar functionality as the doSomething()
method. It is best to remove such redundant methods to keep the interface clean and focused.
Best Practice: Naming Conventions for Interfaces
When naming interfaces in Java, it is common to use nouns or noun phrases that describe the abstraction or concept represented by the interface. Interface names should be clear, concise, and follow the camel case naming convention.
For example, consider an interface representing a database connection:
public interface DatabaseConnection { void connect(); void disconnect(); }
In the above example, the interface name DatabaseConnection
clearly represents the concept of a database connection.
Related Article: How to Retrieve Current Date and Time in Java
Performance: Interface versus Abstract Class
When it comes to performance, there is generally no significant difference between using an interface or an abstract class in Java. Both interfaces and abstract classes can be used to define contracts and provide common behavior.
The choice between interfaces and abstract classes should be based on the design requirements and the relationship between the classes. Interfaces are more suitable when defining behavior contracts for unrelated classes or achieving multiple inheritance-like behavior. Abstract classes are more suitable when providing a base implementation and sharing common code among related classes.
Performance: Runtime Polymorphism with Interfaces
One of the key benefits of interfaces in Java is the ability to achieve runtime polymorphism. Runtime polymorphism allows different classes that implement the same interface to be treated interchangeably, providing flexibility and extensibility in the code.
Here’s an example of runtime polymorphism with interfaces:
public interface Animal { void makeSound(); } public class Dog implements Animal { @Override public void makeSound() { System.out.println("Woof!"); } } public class Cat implements Animal { @Override public void makeSound() { System.out.println("Meow!"); } } public class Main { public static void main(String[] args) { Animal dog = new Dog(); Animal cat = new Cat(); dog.makeSound(); // Output: Woof! cat.makeSound(); // Output: Meow! } }
In the above example, the Animal
interface defines the makeSound()
method. The Dog
and Cat
classes implement the Animal
interface and provide their own implementation for the makeSound()
method. At runtime, the dog
and cat
objects can be treated as Animal
objects and their respective makeSound()
methods can be called.
Advanced Technique: Multiple Inheritance with Interfaces
Interfaces in Java allow for achieving multiple inheritance-like behavior since a class can implement multiple interfaces. This allows a class to inherit behavior from multiple sources, providing flexibility in code design.
Here’s an example of multiple inheritance-like behavior with interfaces:
public interface InterfaceA { void methodA(); } public interface InterfaceB { void methodB(); } public class MyClass implements InterfaceA, InterfaceB { @Override public void methodA() { // Implementation for methodA() from InterfaceA } @Override public void methodB() { // Implementation for methodB() from InterfaceB } }
In the above example, the class MyClass
implements both InterfaceA
and InterfaceB
, inheriting the behavior defined by both interfaces.
Related Article: How to Reverse a String in Java
Advanced Technique: Adapter Pattern with Interfaces
The adapter pattern is a design pattern that allows objects with incompatible interfaces to work together. Interfaces in Java can be used as the basis for implementing the adapter pattern, providing a way to adapt one interface to another.
Here’s an example of using the adapter pattern with interfaces:
public interface MediaPlayer { void play(String filename); } public interface MediaAdapter { void play(String filename); } public class AudioPlayer implements MediaPlayer { private MediaAdapter adapter; @Override public void play(String filename) { if (filename.endsWith(".mp3")) { // Play MP3 file directly } else if (filename.endsWith(".vlc") || filename.endsWith(".mp4")) { // Use adapter to play VLC or MP4 file adapter = new MediaAdapter(); adapter.play(filename); } else { System.out.println("Invalid media format: " + filename); } } } public class MediaAdapter implements MediaPlayer { private VideoPlayer videoPlayer; @Override public void play(String filename) { if (filename.endsWith(".vlc")) { videoPlayer = new VLCPlayer(); videoPlayer.playVideo(filename); } else if (filename.endsWith(".mp4")) { videoPlayer = new MP4Player(); videoPlayer.playVideo(filename); } else { System.out.println("Invalid media format: " + filename); } } } public interface VideoPlayer { void playVideo(String filename); } public class VLCPlayer implements VideoPlayer { @Override public void playVideo(String filename) { // Play VLC video } } public class MP4Player implements VideoPlayer { @Override public void playVideo(String filename) { // Play MP4 video } }
In the above example, the MediaPlayer
interface declares the play()
method. The AudioPlayer
class implements the MediaPlayer
interface and uses an adapter (MediaAdapter
) to play VLC or MP4 files by adapting the MediaPlayer
interface to the VideoPlayer
interface.
Error Handling: Exceptions in Interface Methods
Interface methods in Java can declare checked exceptions in their method signature. A checked exception is an exception that must be caught or declared to be thrown by the caller.
Here’s an example of an interface method with a checked exception:
public interface MyInterface { void doSomething() throws IOException; }
In the above example, the doSomething()
method in the MyInterface
interface declares that it can throw an IOException
. Any class implementing this interface must handle or declare the exception.
Code Snippet: Using Exceptions in Interface Methods
public class MyClass implements MyInterface { @Override public void doSomething() throws IOException { // Implementation for doSomething() method } }
In the above example, the MyClass
class implements the MyInterface
interface and provides an implementation for the doSomething()
method. Since the method can throw an IOException
, the implementing class must handle or declare the exception accordingly.