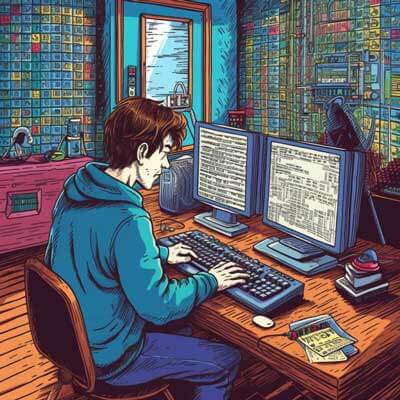
- Intro: Calculating Factorial
- Finding the Maximum Number
- Reversing a String
- Checking if a Number is Prime
- Sorting an Array
- Finding the Fibonacci Sequence
- Converting Strings to Integers
- Generating Random Numbers
- Checking if a String is Palindrome
- Getting the Current Date and Time
- Removing Duplicates from an Array
- Finding the Length of a String
- Checking if Two Strings are Anagrams
- Calculating the Power of a Number
- Reversing an Array
- Finding the Minimum Number
- Converting Integers to Strings
- Calculating the Average of an Array
- Checking if an Array is Sorted
- Converting Strings to Doubles
- Searching for an Element in an Array
- Best Practices for Using Java Code Snippets
- 1. Use Descriptive Variable and Method Names
- 2. Follow Coding Conventions
- 3. Use Comments Sparingly
- 4. Handle Exceptions Properly
- 5. Use Meaningful Class and Method Modifiers
- 6. Test Code Snippets
- Optimizing Java Code Snippets for Everyday Problems
- 1. Use Efficient Data Structures
- 2. Minimize Memory Usage
- 3. Avoid Unnecessary Operations
- 4. Use Efficient Algorithms
- 5. Avoid Unnecessary String Concatenation
- Libraries and Frameworks for Java Code Snippets
- 1. Apache Commons
- 2. Guava
- 3. JUnit
- 4. Spring Framework
- 5. Jackson
- Avoiding Common Mistakes with Java Code Snippets
- 1. Not Handling Exceptions Properly
- 2. Using Inefficient Data Structures or Algorithms
- 3. Not Testing Code Snippets
- 4. Ignoring Coding Conventions
- Resources and Tutorials for Learning Java Code Snippets
- 1. Oracle Java Tutorials
- 2. Baeldung
- 3. Java Code Geeks
- 4. Stack Overflow
- External Sources
Intro: Calculating Factorial
The factorial of a non-negative integer n is denoted by n! and is the product of all positive integers less than or equal to n.
Here’s a Java code snippet that calculates the factorial of a given number:
public class FactorialCalculator { public static int factorial(int num) { if (num == 0 || num == 1) { return 1; } else { return num * factorial(num - 1); } } public static void main(String[] args) { int number = 5; int result = factorial(number); System.out.println("The factorial of " + number + " is: " + result); } }
This code snippet defines a FactorialCalculator
class with a factorial
method that takes an integer as input and recursively calculates its factorial. The main
method demonstrates the usage of the factorial
method by calculating and printing the factorial of the number 5.
Related Article: Critical Algorithms and Data Structures in Java
Finding the Maximum Number
You can iterate over the array elements and keep track of the maximum number found so far.
Here’s a Java code snippet that finds the maximum number in an array:
public class MaximumNumberFinder { public static int findMaximum(int[] numbers) { if (numbers.length == 0) { throw new IllegalArgumentException("Array cannot be empty"); } int max = numbers[0]; for (int i = 1; i < numbers.length; i++) { if (numbers[i] > max) { max = numbers[i]; } } return max; } public static void main(String[] args) { int[] numbers = { 5, 2, 8, 3, 1 }; int maximum = findMaximum(numbers); System.out.println("The maximum number in the array is: " + maximum); } }
This code snippet defines a MaximumNumberFinder
class with a findMaximum
method that takes an array of integers as input and iterates over the elements to find the maximum number. The main
method demonstrates the usage of the findMaximum
method by finding and printing the maximum number in the array { 5, 2, 8, 3, 1 }.
Reversing a String
There are multiple approaches to solve this problem, such as using a StringBuilder or converting the string to a character array.
Here’s a Java code snippet that reverses a given string:
public class StringReverser { public static String reverseString(String str) { StringBuilder reversed = new StringBuilder(str); return reversed.reverse().toString(); } public static void main(String[] args) { String original = "Hello, World!"; String reversed = reverseString(original); System.out.println("The reversed string is: " + reversed); } }
This code snippet defines a StringReverser
class with a reverseString
method that takes a string as input and uses a StringBuilder
to reverse it. The main
method demonstrates the usage of the reverseString
method by reversing and printing the string “Hello, World!”.
Checking if a Number is Prime
A prime number is a natural number greater than 1 that has no positive divisors other than 1 and itself.
Here’s a Java code snippet that checks if a given number is prime:
public class PrimeNumberChecker { public static boolean isPrime(int num) { if (num <= 1) { return false; } for (int i = 2; i <= Math.sqrt(num); i++) { if (num % i == 0) { return false; } } return true; } public static void main(String[] args) { int number = 17; boolean isPrime = isPrime(number); System.out.println(number + " is prime? " + isPrime); } }
This code snippet defines a PrimeNumberChecker
class with an isPrime
method that takes an integer as input and checks if it is prime. The method uses a loop to iterate over the numbers from 2 to the square root of the input number and checks if any of them divide the number evenly. If a divisor is found, the number is not prime. The main
method demonstrates the usage of the isPrime
method by checking and printing whether the number 17 is prime.
Sorting an Array
There are various sorting algorithms available, such as bubble sort, selection sort, insertion sort, merge sort, and quicksort.
Here’s a Java code snippet that sorts an array in ascending order using the bubble sort algorithm:
public class ArraySorter { public static void bubbleSort(int[] array) { int n = array.length; for (int i = 0; i < n - 1; i++) { for (int j = 0; j < n - i - 1; j++) { if (array[j] > array[j + 1]) { int temp = array[j]; array[j] = array[j + 1]; array[j + 1] = temp; } } } } public static void main(String[] args) { int[] array = { 5, 2, 8, 3, 1 }; bubbleSort(array); System.out.println("The sorted array is: " + Arrays.toString(array)); } }
This code snippet defines an ArraySorter
class with a bubbleSort
method that takes an array of integers as input and sorts it in ascending order using the bubble sort algorithm. The main
method demonstrates the usage of the bubbleSort
method by sorting and printing the array { 5, 2, 8, 3, 1 }.
Finding the Fibonacci Sequence
The Fibonacci sequence is a series of numbers in which each number is the sum of the two preceding ones, usually starting with 0 and 1.
Here’s a Java code snippet that generates the Fibonacci sequence up to a given number of terms:
public class FibonacciSequenceGenerator { public static void generateFibonacci(int numTerms) { int firstTerm = 0; int secondTerm = 1; System.out.print(firstTerm + " " + secondTerm); for (int i = 2; i < numTerms; i++) { int nextTerm = firstTerm + secondTerm; System.out.print(" " + nextTerm); firstTerm = secondTerm; secondTerm = nextTerm; } } public static void main(String[] args) { int numTerms = 10; generateFibonacci(numTerms); } }
This code snippet defines a FibonacciSequenceGenerator
class with a generateFibonacci
method that takes the number of terms as input and generates the Fibonacci sequence up to that number of terms. The method uses a loop to calculate and print each term in the sequence. The main
method demonstrates the usage of the generateFibonacci
method by generating and printing the Fibonacci sequence with 10 terms.
Converting Strings to Integers
This is often needed when dealing with user input or parsing data from external sources.
Here’s a Java code snippet that converts a string to an integer:
public class StringToIntConverter { public static int convertStringToInt(String str) { try { return Integer.parseInt(str); } catch (NumberFormatException e) { throw new IllegalArgumentException("Invalid integer format"); } } public static void main(String[] args) { String str = "123"; int num = convertStringToInt(str); System.out.println("The converted integer is: " + num); } }
This code snippet defines a StringToIntConverter
class with a convertStringToInt
method that takes a string as input and uses the parseInt
method from the Integer
class to convert it to an integer. The method also handles the case where the string is not a valid integer format by throwing an IllegalArgumentException
. The main
method demonstrates the usage of the convertStringToInt
method by converting and printing the string “123” as an integer.
Generating Random Numbers
Generating random numbers is a common requirement in Java programming. The java.util.Random
class provides methods for generating random numbers of different types.
Here’s a Java code snippet that generates a random number between a given range:
import java.util.Random; public class RandomNumberGenerator { public static int generateRandomNumber(int min, int max) { Random random = new Random(); return random.nextInt(max - min + 1) + min; } public static void main(String[] args) { int min = 1; int max = 100; int randomNumber = generateRandomNumber(min, max); System.out.println("The generated random number is: " + randomNumber); } }
This code snippet imports the java.util.Random
class and defines a RandomNumberGenerator
class with a generateRandomNumber
method that takes a minimum and maximum value and uses the nextInt
method of the Random
class to generate a random number within the specified range. The main
method demonstrates the usage of the generateRandomNumber
method by generating and printing a random number between 1 and 100.
Checking if a String is Palindrome
A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward.
Here’s a Java code snippet that checks if a given string is a palindrome:
public class PalindromeChecker { public static boolean isPalindrome(String str) { String reversed = new StringBuilder(str).reverse().toString(); return str.equals(reversed); } public static void main(String[] args) { String word = "racecar"; boolean isPalindrome = isPalindrome(word); System.out.println(word + " is a palindrome? " + isPalindrome); } }
This code snippet defines a PalindromeChecker
class with an isPalindrome
method that takes a string as input and uses a StringBuilder
to reverse the string. The method then compares the original string with the reversed string to check if they are equal. The main
method demonstrates the usage of the isPalindrome
method by checking and printing whether the word “racecar” is a palindrome.
Getting the Current Date and Time
Getting the current date and time is a common requirement in Java programming. The java.util.Date
and java.util.Calendar
classes provide methods for working with dates and times.
Here’s a Java code snippet that gets the current date and time:
import java.util.Date; public class CurrentDateTimeGetter { public static void main(String[] args) { Date currentDate = new Date(); System.out.println("Current date and time: " + currentDate); } }
This code snippet imports the java.util.Date
class and defines a CurrentDateTimeGetter
class with a main
method that creates a new Date
object to represent the current date and time. The main
method then prints the current date and time using the toString
method of the Date
class.
Removing Duplicates from an Array
There are various approaches to solve this problem, such as using a Set
or creating a new array without the duplicates.
Here’s a Java code snippet that removes duplicates from an array:
import java.util.Arrays; import java.util.LinkedHashSet; public class ArrayDuplicateRemover { public static int[] removeDuplicates(int[] array) { LinkedHashSet<Integer> set = new LinkedHashSet<>(); for (int num : array) { set.add(num); } int[] result = new int[set.size()]; int i = 0; for (int num : set) { result[i++] = num; } return result; } public static void main(String[] args) { int[] array = { 1, 2, 3, 1, 4, 2, 5 }; int[] uniqueArray = removeDuplicates(array); System.out.println("Array with duplicates: " + Arrays.toString(array)); System.out.println("Array without duplicates: " + Arrays.toString(uniqueArray)); } }
This code snippet imports the java.util.Arrays
and java.util.LinkedHashSet
classes and defines an ArrayDuplicateRemover
class with a removeDuplicates
method that takes an array of integers as input. The method uses a LinkedHashSet
to store unique elements from the array while preserving the order. It then creates a new array with the unique elements and returns it. The main
method demonstrates the usage of the removeDuplicates
method by removing duplicates from an array and printing both the original and the resulting array.
Finding the Length of a String
Finding the length of a string is a common operation in Java programming. The length
method of the String
class can be used to determine the number of characters in a string.
Here’s a Java code snippet that finds the length of a string:
public class StringLengthFinder { public static int findLength(String str) { return str.length(); } public static void main(String[] args) { String str = "Hello, World!"; int length = findLength(str); System.out.println("The length of the string is: " + length); } }
This code snippet defines a StringLengthFinder
class with a findLength
method that takes a string as input and uses the length
method of the String
class to find its length. The main
method demonstrates the usage of the findLength
method by finding and printing the length of the string “Hello, World!”.
Checking if Two Strings are Anagrams
An anagram is a word or phrase formed by rearranging the letters of another word or phrase.
Here’s a Java code snippet that checks if two strings are anagrams:
import java.util.Arrays; public class AnagramChecker { public static boolean areAnagrams(String str1, String str2) { char[] charArray1 = str1.toLowerCase().toCharArray(); char[] charArray2 = str2.toLowerCase().toCharArray(); Arrays.sort(charArray1); Arrays.sort(charArray2); return Arrays.equals(charArray1, charArray2); } public static void main(String[] args) { String word1 = "listen"; String word2 = "silent"; boolean areAnagrams = areAnagrams(word1, word2); System.out.println(word1 + " and " + word2 + " are anagrams? " + areAnagrams); } }
This code snippet imports the java.util.Arrays
class and defines an AnagramChecker
class with an areAnagrams
method that takes two strings as input. The method converts the strings to lowercase and then converts them to character arrays. It then sorts the character arrays and uses the equals
method of the Arrays
class to check if they are equal. The main
method demonstrates the usage of the areAnagrams
method by checking and printing whether the words “listen” and “silent” are anagrams.
Calculating the Power of a Number
The power of a number represents how many times the number is multiplied by itself.
Here’s a Java code snippet that calculates the power of a given number:
public class PowerCalculator { public static double calculatePower(double base, int exponent) { if (exponent == 0) { return 1; } else if (exponent > 0) { double result = 1; for (int i = 0; i < exponent; i++) { result *= base; } return result; } else { double result = 1; for (int i = 0; i > exponent; i--) { result /= base; } return result; } } public static void main(String[] args) { double base = 2; int exponent = 3; double result = calculatePower(base, exponent); System.out.println(base + " raised to the power of " + exponent + " is: " + result); } }
This code snippet defines a PowerCalculator
class with a calculatePower
method that takes a base number and an exponent as input and calculates the result of raising the base to the power of the exponent. The method uses a loop to perform the multiplication or division based on the sign of the exponent. The main
method demonstrates the usage of the calculatePower
method by calculating and printing the result of raising the number 2 to the power of 3.
Reversing an Array
There are multiple approaches to solve this problem, such as using two pointers or creating a new array.
Here’s a Java code snippet that reverses an array:
import java.util.Arrays; public class ArrayReverser { public static void reverseArray(int[] array) { int left = 0; int right = array.length - 1; while (left < right) { int temp = array[left]; array[left] = array[right]; array[right] = temp; left++; right--; } } public static void main(String[] args) { int[] array = { 1, 2, 3, 4, 5 }; System.out.println("Array before reversing: " + Arrays.toString(array)); reverseArray(array); System.out.println("Array after reversing: " + Arrays.toString(array)); } }
This code snippet imports the java.util.Arrays
class and defines an ArrayReverser
class with a reverseArray
method that takes an array of integers as input and reverses its elements using two pointers. The main
method demonstrates the usage of the reverseArray
method by reversing and printing an array { 1, 2, 3, 4, 5 }.
Finding the Minimum Number
You can iterate over the array elements and keep track of the minimum number found so far.
Here’s a Java code snippet that finds the minimum number in an array:
public class MinimumNumberFinder { public static int findMinimum(int[] numbers) { if (numbers.length == 0) { throw new IllegalArgumentException("Array cannot be empty"); } int min = numbers[0]; for (int i = 1; i < numbers.length; i++) { if (numbers[i] < min) { min = numbers[i]; } } return min; } public static void main(String[] args) { int[] numbers = { 5, 2, 8, 3, 1 }; int minimum = findMinimum(numbers); System.out.println("The minimum number in the array is: " + minimum); } }
This code snippet defines a MinimumNumberFinder
class with a findMinimum
method that takes an array of integers as input and iterates over the elements to find the minimum number. The main
method demonstrates the usage of the findMinimum
method by finding and printing the minimum number in the array { 5, 2, 8, 3, 1 }.
Converting Integers to Strings
This is often needed when concatenating numbers with strings or when formatting output.
Here’s a Java code snippet that converts an integer to a string:
public class IntToStringConverter { public static String convertIntToString(int num) { return String.valueOf(num); } public static void main(String[] args) { int num = 123; String str = convertIntToString(num); System.out.println("The converted string is: " + str); } }
This code snippet defines an IntToStringConverter
class with a convertIntToString
method that takes an integer as input and uses the valueOf
method of the String
class to convert it to a string. The main
method demonstrates the usage of the convertIntToString
method by converting and printing the integer 123 as a string.
Calculating the Average of an Array
You can iterate over the array elements and calculate the sum, then divide it by the number of elements.
Here’s a Java code snippet that calculates the average of an array:
public class ArrayAverageCalculator { public static double calculateAverage(int[] array) { if (array.length == 0) { return 0; } int sum = 0; for (int num : array) { sum += num; } return (double) sum / array.length; } public static void main(String[] args) { int[] array = { 5, 2, 8, 3, 1 }; double average = calculateAverage(array); System.out.println("The average of the array is: " + average); } }
This code snippet defines an ArrayAverageCalculator
class with a calculateAverage
method that takes an array of integers as input and calculates its average by summing all the elements and dividing by the number of elements. The main
method demonstrates the usage of the calculateAverage
method by calculating and printing the average of the array { 5, 2, 8, 3, 1 }.
Checking if an Array is Sorted
You can iterate over the array elements and compare adjacent elements to determine if they are in the correct order.
Here’s a Java code snippet that checks if an array is sorted in ascending order:
public class ArraySortChecker { public static boolean isSorted(int[] array) { for (int i = 1; i < array.length; i++) { if (array[i] < array[i - 1]) { return false; } } return true; } public static void main(String[] args) { int[] array = { 1, 2, 3, 4, 5 }; boolean isSorted = isSorted(array); System.out.println("Is the array sorted? " + isSorted); } }
This code snippet defines an ArraySortChecker
class with an isSorted
method that takes an array of integers as input and iterates over the elements to check if they are in ascending order. The main
method demonstrates the usage of the isSorted
method by checking and printing whether the array { 1, 2, 3, 4, 5 } is sorted.
Converting Strings to Doubles
This is often needed when dealing with user input or parsing data from external sources.
Here’s a Java code snippet that converts a string to a double:
public class StringToDoubleConverter { public static double convertStringToDouble(String str) { try { return Double.parseDouble(str); } catch (NumberFormatException e) { throw new IllegalArgumentException("Invalid double format"); } } public static void main(String[] args) { String str = "3.14"; double num = convertStringToDouble(str); System.out.println("The converted double is: " + num); } }
This code snippet defines a StringToDoubleConverter
class with a convertStringToDouble
method that takes a string as input and uses the parseDouble
method of the Double
class to convert it to a double. The method also handles the case where the string is not a valid double format by throwing an IllegalArgumentException
. The main
method demonstrates the usage of the convertStringToDouble
method by converting and printing the string “3.14” as a double.
Searching for an Element in an Array
You can iterate over the array elements and compare each element with the target element.
Here’s a Java code snippet that searches for an element in an array:
public class ArraySearcher { public static boolean searchElement(int[] array, int target) { for (int num : array) { if (num == target) { return true; } } return false; } public static void main(String[] args) { int[] array = { 5, 2, 8, 3, 1 }; int target = 8; boolean found = searchElement(array, target); System.out.println("Is " + target + " present in the array? " + found); } }
This code snippet defines an ArraySearcher
class with a searchElement
method that takes an array of integers and a target number as input. The method iterates over the elements of the array and checks if any of them match the target number. If a match is found, it returns true; otherwise, it returns false. The main
method demonstrates the usage of the searchElement
method by searching for the number 8 in the array { 5, 2, 8, 3, 1 }.
Best Practices for Using Java Code Snippets
When using Java code snippets, it’s important to follow best practices to ensure code readability, maintainability, and performance. Here are some best practices for using Java code snippets:
1. Use Descriptive Variable and Method Names
Using meaningful variable and method names is essential for code readability. Avoid using single-letter variable names or generic names like temp
or result
. Instead, use descriptive names that accurately represent the purpose of the variable or method.
Example:
// Bad int x = 5; // Good int numberOfStudents = 5;
2. Follow Coding Conventions
Following coding conventions improves code consistency and makes it easier for other developers to read and understand your code. Use proper indentations, consistent naming conventions (e.g., camel case for variables and methods, uppercase for constants), and adhere to the Java naming conventions.
Example:
// Bad public static void calculateaverage(int[] numbers) { // code here } // Good public static void calculateAverage(int[] numbers) { // code here }
3. Use Comments Sparingly
Comments should be used to explain complex logic or provide additional information that is not obvious from the code itself. Avoid unnecessary or redundant comments, as they can clutter the code and make it harder to read.
Example:
// Bad int x = 5; // initialize x to 5 // Good int numberOfStudents = 5;
4. Handle Exceptions Properly
When dealing with exceptions, it’s important to handle them properly to avoid unexpected program behavior or crashes. Use try-catch blocks to catch and handle exceptions, or throw exceptions when appropriate.
Example:
// Bad public static int divide(int a, int b) { return a / b; } // Good public static int divide(int a, int b) { try { return a / b; } catch (ArithmeticException e) { throw new IllegalArgumentException("Cannot divide by zero"); } }
5. Use Meaningful Class and Method Modifiers
Using appropriate class and method modifiers helps improve code clarity and maintainability. Use public
, private
, and protected
modifiers to control the visibility of classes and methods, and use final
and static
modifiers when appropriate.
Example:
// Bad class MyClass { // code here } // Good public final class MyClass { // code here }
6. Test Code Snippets
Before using code snippets in production, it’s important to thoroughly test them to ensure they work as intended and handle edge cases correctly. Write unit tests to cover different scenarios and validate the expected behavior of the code.
Example:
@Test public void testFindMaximum() { int[] numbers = { 5, 2, 8, 3, 1 }; assertEquals(8, MaximumNumberFinder.findMaximum(numbers)); }
Optimizing Java Code Snippets for Everyday Problems
Optimizing Java code snippets can improve performance and efficiency, especially for code that is executed frequently or deals with large datasets. Here are some techniques to optimize Java code snippets for everyday problems:
1. Use Efficient Data Structures
Choosing the right data structure can significantly impact the performance of your code. For example, use ArrayList
instead of LinkedList
when frequent random access is required, and use HashMap
instead of ArrayList
when efficient lookup is needed.
Example:
// Bad LinkedList<Integer> list = new LinkedList<>(); list.add(1); list.add(2); list.add(3); // Good ArrayList<Integer> list = new ArrayList<>(); list.add(1); list.add(2); list.add(3);
2. Minimize Memory Usage
Optimizing memory usage can improve the performance of your code, especially when dealing with large datasets. Avoid creating unnecessary objects or using excessive memory, and release resources as soon as they are no longer needed.
Example:
// Bad for (int i = 0; i < 1000000; i++) { String str = "Value: " + i; // code here } // Good for (int i = 0; i < 1000000; i++) { StringBuilder sb = new StringBuilder(); sb.append("Value: ").append(i); String str = sb.toString(); // code here }
3. Avoid Unnecessary Operations
Avoid performing unnecessary operations or calculations that can be avoided. Simplify your code by removing redundant statements or computations.
Example:
// Bad for (int i = 0; i < array.length; i++) { int num = array[i]; if (num > 0) { positiveCount++; } else if (num < 0) { negativeCount++; } else { zeroCount++; } } // Good for (int num : array) { if (num > 0) { positiveCount++; } else if (num < 0) { negativeCount++; } else { zeroCount++; } }
4. Use Efficient Algorithms
Choosing efficient algorithms can significantly impact the performance of your code. For example, use quicksort instead of bubblesort for sorting large arrays, and use binary search instead of linear search for finding elements in sorted arrays.
Example:
// Bad for (int i = 0; i < array.length; i++) { if (array[i] == target) { return i; } } // Good int low = 0; int high = array.length - 1; while (low <= high) { int mid = (low + high) / 2; if (array[mid] == target) { return mid; } else if (array[mid] < target) { low = mid + 1; } else { high = mid - 1; } }
5. Avoid Unnecessary String Concatenation
String concatenation can be expensive, especially when performed repeatedly in loops. Use StringBuilder
or StringBuffer
instead of concatenating strings using the +
operator.
Example:
// Bad String result = ""; for (String str : strings) { result += str; } // Good StringBuilder sb = new StringBuilder(); for (String str : strings) { sb.append(str); } String result = sb.toString();
Libraries and Frameworks for Java Code Snippets
Java has a rich ecosystem of libraries and frameworks that provide ready-to-use code snippets for various purposes. Here are some popular libraries and frameworks for Java:
1. Apache Commons
Apache Commons is a collection of reusable Java components that provide implementations of common utility functions, data structures, and algorithms. It includes libraries for string manipulation, file handling, math operations, and more.
Link: Apache Commons
2. Guava
Guava is a set of core libraries for Java that provide useful utility classes and data structures not found in the standard JDK. It includes libraries for collections, caching, functional programming, concurrency, and more.
Link: Guava
3. JUnit
JUnit is a unit testing framework for Java that provides a simple and efficient way to write and run tests for your code. It includes annotations, assertions, and test runners to facilitate the testing process.
Link: JUnit
4. Spring Framework
Spring Framework is a comprehensive framework for Java that provides support for building enterprise-grade applications. It includes libraries for dependency injection, MVC web development, data access, security, and more.
Link: Spring Framework
5. Jackson
Jackson is a high-performance JSON processing library for Java that provides methods for reading and writing JSON data. It includes annotations and converters for easy serialization and deserialization of Java objects.
Link: Jackson
Avoiding Common Mistakes with Java Code Snippets
When using Java code snippets, it’s important to avoid common mistakes that can lead to errors or inefficient code. Here are some common mistakes to avoid:
1. Not Handling Exceptions Properly
Failing to handle exceptions can result in unexpected program behavior or crashes. Always handle exceptions by using try-catch blocks or throwing them when appropriate.
Example:
// Bad public static int divide(int a, int b) { return a / b; // May throw ArithmeticException } // Good public static int divide(int a, int b) { try { return a / b; } catch (ArithmeticException e) { throw new IllegalArgumentException("Cannot divide by zero"); } }
2. Using Inefficient Data Structures or Algorithms
Choosing inefficient data structures or algorithms can lead to poor performance. Always choose the appropriate data structure or algorithm for the problem at hand.
Example:
// Bad LinkedList<Integer> list = new LinkedList<>(); list.add(1); list.add(2); list.add(3); // Good ArrayList<Integer> list = new ArrayList<>(); list.add(1); list.add(2); list.add(3);
3. Not Testing Code Snippets
Failing to test code snippets can result in bugs or unexpected behavior. Always write unit tests to cover different scenarios and validate the expected behavior of your code.
Example:
@Test public void testFindMaximum() { int[] numbers = { 5, 2, 8, 3, 1 }; assertEquals(8, MaximumNumberFinder.findMaximum(numbers)); }
4. Ignoring Coding Conventions
Ignoring coding conventions can make your code harder to read and maintain. Always follow coding conventions, such as proper indentations, consistent naming conventions, and class/method modifiers.
Example:
// Bad public static void calculateaverage(int[] numbers) { // code here } // Good public static void calculateAverage(int[] numbers) { // code here }
Resources and Tutorials for Learning Java Code Snippets
Learning Java code snippets requires practice and familiarity with the Java programming language. Here are some resources and tutorials to help you learn Java code snippets:
1. Oracle Java Tutorials
The official Oracle Java Tutorials provide comprehensive documentation and examples for learning Java programming. It covers various topics, including basic syntax, data types, control flow, arrays, classes, and more.
Link: Oracle Java Tutorials
2. Baeldung
Baeldung is a popular Java programming blog that provides in-depth tutorials, articles, and code snippets on various Java topics. It covers topics ranging from basic Java concepts to advanced frameworks and libraries.
Link: Baeldung
3. Java Code Geeks
Java Code Geeks is a community-driven platform that offers a wide range of Java tutorials, articles, and code snippets. It covers Java topics such as core Java, JEE, frameworks, tools, and more.
Link: Java Code Geeks
4. Stack Overflow
Stack Overflow is a question-and-answer platform for programmers. It contains a vast collection of Java-related questions and answers, which can help you learn from real-world scenarios and challenges faced by other developers.
Link: Stack Overflow
External Sources
Throughout this article, we have referred to various external sources for additional information and resources. Here are the links to those sources: