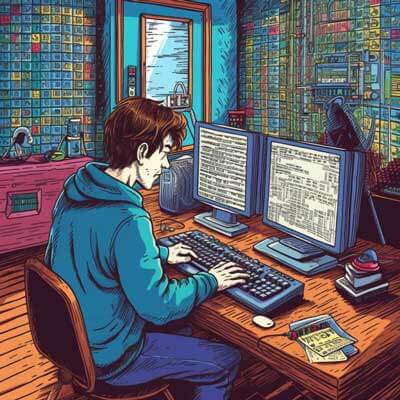
- The Importance of Data Structures in Software Engineering
- Example 1: Array
- Example 2: Linked List
- Understanding Trees and Their Role in Data Structures
- Example 1: Binary Tree
- Example 2: Trie
- The Trie Data Structure: An Overview
- Example 1: Trie Structure
- Example 2: Trie Search
- How Tries Enable Efficient String Search
- Example 1: Trie Search Process
- Example 2: Trie Search Complexity
- Understanding the Inner Workings of a Trie Data Structure
- Example: TrieNode Implementation
- Example: Trie Implementation
- Advantages of Using a Trie Data Structure
- Exploring Limitations and Drawbacks of Tries
- Implementing a Trie Data Structure in Java
- Examining Java Libraries/Frameworks for Trie Implementation
- Real-World Applications of Trie Data Structures
- Java’s Built-In Data Structures and Their Alternatives to Tries
- Additional Resources
The Importance of Data Structures in Software Engineering
Data structures are an essential component of software engineering. They provide a way to organize and store data efficiently, enabling faster access, retrieval, and manipulation of information. Choosing the right data structure for a particular problem can significantly impact the performance and scalability of a software solution.
In software engineering, data structures are used to represent and organize different types of data, such as numbers, text, and complex objects. They allow programmers to perform operations like searching, sorting, and modifying data in an efficient manner. Data structures are at the core of algorithm design and play a crucial role in solving various computational problems.
Related Article: How to Convert List to Array in Java
Example 1: Array
Arrays are a fundamental data structure in Java that store a fixed-size sequence of elements of the same type. They provide constant-time access to individual elements, making them suitable for scenarios where random access is required. Here’s an example of creating and accessing elements in an array:
int[] numbers = new int[5]; numbers[0] = 1; numbers[1] = 2; numbers[2] = 3; numbers[3] = 4; numbers[4] = 5; System.out.println(numbers[2]); // Output: 3
Example 2: Linked List
Linked lists are another commonly used data structure that consists of a sequence of nodes, where each node contains a value and a reference to the next node. Linked lists allow for dynamic memory allocation and efficient insertion and deletion operations. Here’s an example of creating and traversing a linked list:
class Node { int value; Node next; public Node(int value) { this.value = value; } } Node head = new Node(1); Node second = new Node(2); Node third = new Node(3); head.next = second; second.next = third; Node current = head; while (current != null) { System.out.println(current.value); current = current.next; }
Understanding different data structures and their characteristics is crucial for designing efficient software solutions. In the next section, we will explore trees, a type of data structure commonly used in combination with other data structures.
Understanding Trees and Their Role in Data Structures
Trees are hierarchical data structures that consist of nodes connected by edges. Each tree has a root node, which is the topmost node, and can have zero or more child nodes. Trees are widely used in data structures to represent relationships between elements and enable efficient searching, sorting, and traversal operations.
Related Article: Tutorial: Sorted Data Structure Storage in Java
Example 1: Binary Tree
A binary tree is a type of tree where each node has at most two child nodes, referred to as the left child and the right child. Binary trees are commonly used in search algorithms like binary search and can be efficiently traversed using techniques like in-order, pre-order, and post-order traversal. Here’s an example of creating and traversing a binary tree:
class TreeNode { int value; TreeNode left; TreeNode right; public TreeNode(int value) { this.value = value; } } TreeNode root = new TreeNode(1); root.left = new TreeNode(2); root.right = new TreeNode(3); root.left.left = new TreeNode(4); root.left.right = new TreeNode(5); // In-order traversal void inOrderTraversal(TreeNode node) { if (node != null) { inOrderTraversal(node.left); System.out.println(node.value); inOrderTraversal(node.right); } } inOrderTraversal(root);
Example 2: Trie
A trie, also known as a prefix tree, is a specialized tree data structure that is particularly useful for efficient string search operations. It is commonly used in applications like autocomplete, spell checkers, and IP routing tables. In the next section, we will explore the trie data structure in more detail.
The Trie Data Structure: An Overview
The trie data structure is a tree-like structure that stores a set of strings. Unlike binary trees, which store elements based on their values, tries store elements based on their characters. Each node in a trie represents a character, and the edges represent the relationship between the characters.
The root node of a trie is typically an empty node, and each child node represents a character. As we traverse down the trie, the characters form a path from the root to a leaf node. The leaf nodes represent the end of a string.
One of the key advantages of tries is that they allow for efficient string search operations. By traversing the trie based on the characters of a string, we can quickly determine whether the string exists in the trie or not.
Related Article: Tutorial: Enumeration Types and Data Structures in Java
Example 1: Trie Structure
Let’s consider the following words: “cat”, “car”, “cart”, “dog”, and “dot”. We can represent these words using a trie data structure as follows:
root / \ c d / \ \ a o o / \ \ \ t r t g
In this example, the letters form a path from the root to the leaf nodes, representing the words stored in the trie.
Example 2: Trie Search
Suppose we want to search for the word “cart” in the trie. We can traverse the trie based on the characters of the word and check if the path exists in the trie. Here’s an example of searching for the word “cart” in the trie:
class TrieNode { Map children; boolean isEndOfWord; public TrieNode() { children = new HashMap(); isEndOfWord = false; } } class Trie { TrieNode root; public Trie() { root = new TrieNode(); } public void insert(String word) { TrieNode current = root; for (char c : word.toCharArray()) { current.children.putIfAbsent(c, new TrieNode()); current = current.children.get(c); } current.isEndOfWord = true; } public boolean search(String word) { TrieNode current = root; for (char c : word.toCharArray()) { if (!current.children.containsKey(c)) { return false; } current = current.children.get(c); } return current.isEndOfWord; } } Trie trie = new Trie(); trie.insert("cat"); trie.insert("car"); trie.insert("cart"); trie.insert("dog"); trie.insert("dot"); System.out.println(trie.search("cart")); // Output: true System.out.println(trie.search("dog")); // Output: true System.out.println(trie.search("carp")); // Output: false
In the next section, we will dive deeper into the inner workings of a trie data structure and understand how it enables efficient string search operations.
How Tries Enable Efficient String Search
Tries enable efficient string search operations by exploiting the structure of the trie to narrow down the search space. When searching for a string in a trie, we traverse the trie based on the characters of the string, eliminating large portions of the trie that are not relevant to the search.
During the search, if we encounter a character that does not exist in the trie, we can conclude that the string does not exist in the trie and terminate the search early. This early termination is what makes trie searches efficient, especially for large sets of strings.
Related Article: How to Initialize an ArrayList in One Line in Java
Example 1: Trie Search Process
Let’s consider the trie we created in the previous example:
root / \ c d / \ \ a o o / \ \ \ t r t g
Suppose we want to search for the word “carp” in the trie. We start at the root node and traverse down the trie based on the characters of the word. At each step, we check if the character exists in the current node’s children. If it does not, we can conclude that the word does not exist in the trie. Here’s the search process for the word “carp”:
– Start at the root node.
– Traverse to the “c” node.
– Traverse to the “a” node.
– Traverse to the “r” node.
– The “p” node does not exist in the children of the “r” node, so the word “carp” does not exist in the trie.
This search process allows us to quickly determine whether a string exists in the trie or not, without having to check every single string stored in the trie.
Example 2: Trie Search Complexity
The time complexity of searching for a string in a trie is O(m), where m is the length of the string. This is because, in the worst case, we may need to traverse the entire length of the string to determine its existence in the trie.
However, the actual time complexity can be much lower in practice, as trie searches can terminate early if a character does not exist in the trie. This makes trie searches highly efficient for large sets of strings, especially when the strings share common prefixes.
In the next section, we will explore the inner workings of a trie data structure and understand how it is implemented in Java.
Understanding the Inner Workings of a Trie Data Structure
The inner workings of a trie data structure involve the representation of nodes and the operations performed on the trie, such as insertion and search. In Java, a trie can be implemented using a class and a nested class to represent the trie nodes.
Related Article: How to Easily Print a Java Array
Example: TrieNode Implementation
class TrieNode { Map children; boolean isEndOfWord; public TrieNode() { children = new HashMap(); isEndOfWord = false; } }
In this example, the TrieNode
class represents a node in the trie. It contains a Map
called children
, which maps characters to their corresponding child nodes. The isEndOfWord
flag indicates whether the current node represents the end of a word.
Example: Trie Implementation
class Trie { TrieNode root; public Trie() { root = new TrieNode(); } public void insert(String word) { TrieNode current = root; for (char c : word.toCharArray()) { current.children.putIfAbsent(c, new TrieNode()); current = current.children.get(c); } current.isEndOfWord = true; } public boolean search(String word) { TrieNode current = root; for (char c : word.toCharArray()) { if (!current.children.containsKey(c)) { return false; } current = current.children.get(c); } return current.isEndOfWord; } }
In this example, the Trie
class represents the trie data structure. It contains a root
node, which is the topmost node of the trie. The insert
method is used to insert a word into the trie, while the search
method is used to search for a word in the trie.
The insert
method iterates over the characters of the word and traverses down the trie, creating new nodes if necessary. The last node in the traversal is marked as the end of the word by setting its isEndOfWord
flag to true
.
The search
method follows a similar process, traversing down the trie based on the characters of the word. If a character does not exist in the current node’s children, the search terminates early and returns false
. Otherwise, the search continues until the end of the word, and the result is determined by the isEndOfWord
flag of the last node.
In the next section, we will explore the advantages of using a trie data structure in software engineering.
Advantages of Using a Trie Data Structure
Trie data structures offer several advantages over other data structures, making them suitable for various applications and scenarios. Some of the key advantages of using a trie include:
1. Efficient String Search: Tries enable efficient string search operations by exploiting the structure of the trie to narrow down the search space. This makes them particularly useful for applications like autocomplete, spell checkers, and IP routing tables.
2. Space Efficiency: Tries are space-efficient compared to other data structures like hash tables. They store strings by sharing common prefixes, reducing the overall memory footprint. This makes them suitable for scenarios where memory usage is a concern.
3. Prefix Matching: Tries allow for efficient prefix matching, where we can quickly find all strings that share a common prefix. This is useful in applications like searching for contacts in a phonebook or finding all words starting with a certain prefix.
4. Incremental String Insertion: Tries support incremental string insertion, allowing for dynamic updates without the need to rebuild the entire data structure. This makes them suitable for scenarios where the set of strings is continuously changing.
5. Flexibility: Tries can be easily extended to support additional operations like pattern matching, wildcard search, and longest prefix matching. This flexibility makes them adaptable to a wide range of applications and use cases.
In the next section, we will explore the limitations and drawbacks of using trie data structures.
Related Article: How to Sort a List of ArrayList in Java
Exploring Limitations and Drawbacks of Tries
While trie data structures offer several advantages, they also have some limitations and drawbacks that need to be considered when choosing a data structure for a particular problem. Some of the limitations and drawbacks of using tries include:
1. Memory Usage: Tries can consume a significant amount of memory compared to other data structures, especially for large sets of strings or when the strings have long common prefixes. This can be a concern in memory-constrained environments.
2. Time Complexity: Although trie searches have a time complexity of O(m), where m is the length of the string, the actual time complexity can be higher in practice due to the overhead of traversing the trie and accessing the children nodes.
3. Insertion and Deletion Complexity: Inserting or deleting a string from a trie can be more complex compared to other data structures like hash tables or balanced trees. Modifying the trie structure requires updating multiple nodes, which can be time-consuming.
4. Lack of Ordering: Tries do not inherently maintain any ordering of the stored strings. While this can be an advantage in some scenarios, it can also be a limitation when ordering is required, such as in dictionary-based applications.
5. Limited Use Cases: Tries are most suitable for scenarios where string search operations are the primary concern. They may not be the best choice for other types of data or operations, such as numerical data or range queries.
It is important to carefully consider the limitations and drawbacks of using tries before deciding to use them in a software solution. In the next section, we will explore how to implement a trie data structure in Java.
Implementing a Trie Data Structure in Java
Implementing a trie data structure in Java involves creating classes to represent the nodes of the trie and defining methods for inserting and searching strings. Here’s an example implementation of a trie in Java:
import java.util.HashMap; import java.util.Map; class TrieNode { Map children; boolean isEndOfWord; public TrieNode() { children = new HashMap(); isEndOfWord = false; } } class Trie { TrieNode root; public Trie() { root = new TrieNode(); } public void insert(String word) { TrieNode current = root; for (char c : word.toCharArray()) { current.children.putIfAbsent(c, new TrieNode()); current = current.children.get(c); } current.isEndOfWord = true; } public boolean search(String word) { TrieNode current = root; for (char c : word.toCharArray()) { if (!current.children.containsKey(c)) { return false; } current = current.children.get(c); } return current.isEndOfWord; } }
In this implementation, the TrieNode
class represents a node in the trie. It contains a Map
called children
to store the child nodes, and a boolean flag isEndOfWord
to indicate whether the current node represents the end of a word.
The Trie
class represents the trie data structure itself. It contains a root
node, which is the topmost node of the trie. The insert
method is used to insert a word into the trie, while the search
method is used to search for a word in the trie.
To use the trie, create an instance of the Trie
class and call the insert
method to insert words into the trie. You can then use the search
method to search for words in the trie.
In the next section, we will examine Java libraries and frameworks that provide trie implementation.
Examining Java Libraries/Frameworks for Trie Implementation
While Java does not provide a built-in trie data structure in its standard library, there are several third-party libraries and frameworks available that provide trie implementations. These libraries offer additional features, optimizations, and customization options beyond a basic trie implementation.
One popular library for trie implementation in Java is the Apache Commons Collections library. It provides the PatriciaTrie
class, which is an implementation of a compact prefix tree (trie). The PatriciaTrie
class offers efficient storage and retrieval of strings based on their prefixes, making it suitable for autocomplete and search applications.
Here’s an example of using the PatriciaTrie
class from the Apache Commons Collections library:
import org.apache.commons.collections4.trie.PatriciaTrie; PatriciaTrie trie = new PatriciaTrie(); trie.put("cat", 1); trie.put("car", 2); trie.put("cart", 3); trie.put("dog", 4); trie.put("dot", 5); System.out.println(trie.get("cart")); // Output: 3 System.out.println(trie.get("dog")); // Output: 4 System.out.println(trie.get("carp")); // Output: null
In this example, we create an instance of the PatriciaTrie
class and use the put
method to insert key-value pairs into the trie. We can then use the get
method to retrieve the value associated with a specific key.
The Apache Commons Collections library provides additional functionality like prefix search, range search, and key iteration, making it a versatile choice for trie-based applications.
Other Java libraries and frameworks that provide trie implementations include Google Guava’s Trie
class, the Eclipse Collections library, and the fastutil library. These libraries offer various features and optimizations, so choosing the right library depends on the specific requirements of your application.
In the next section, we will explore real-world applications of trie data structures.
Related Article: How to Declare and Initialize a New Array in Java
Real-World Applications of Trie Data Structures
Trie data structures find applications in various domains and scenarios where efficient string search operations are required. Some real-world applications of trie data structures include:
1. Autocomplete: Tries are commonly used in autocomplete systems to provide suggestions as users type. By storing a dictionary of words in a trie, the system can quickly search for and retrieve words that match the user’s input, providing real-time suggestions.
2. Spell Checkers: Tries are used in spell checkers to efficiently check the validity of words. By traversing a trie based on the characters of a word, spell checkers can determine whether a word is misspelled or not, and provide suggestions for correct spelling.
3. IP Routing: Tries are used in IP routing tables to efficiently determine the next hop for a given IP address. The trie structure allows for prefix matching, where the longest matching prefix determines the route to the destination.
4. Contact Search: Tries are used in contact search applications to quickly find contacts based on partial name matches. By storing contacts in a trie, the system can efficiently search for contacts that share a common prefix, such as searching for “Joh” to find all contacts starting with “Joh”.
5. Word Games: Tries are used in word games like Scrabble and Boggle to validate words played by players. By storing a dictionary of valid words in a trie, the game can quickly search for and verify the existence of a word, ensuring fair gameplay.
These are just a few examples of the many applications of trie data structures in real-world scenarios. The efficiency and flexibility of trie data structures make them a useful tool in software engineering.
In the next section, we will explore Java’s built-in data structures and their alternatives to tries.
Java’s Built-In Data Structures and Their Alternatives to Tries
Java provides several built-in data structures that can be used as alternatives to trie data structures, depending on the specific requirements of the application. Some of the commonly used built-in data structures and their alternatives to tries include:
1. HashMap: Java’s HashMap
is a widely used data structure for fast key-value lookups. It provides constant-time complexity for average and best-case scenarios and can be a good alternative to trie data structures for applications that require efficient search based on exact matches.
2. HashSet: Java’s HashSet
is a data structure that stores a set of unique elements. It provides constant-time complexity for average and best-case scenarios and can be used as an alternative to trie data structures when the goal is to check for the existence of a specific element.
3. TreeMap: Java’s TreeMap
is a data structure that stores key-value pairs in a sorted manner. It provides logarithmic time complexity for average and worst-case scenarios and can be used as an alternative to trie data structures when ordering of the keys is required.
4. PatriciaTrie (Apache Commons Collections): The PatriciaTrie
class from the Apache Commons Collections library provides a compact prefix tree implementation, which can be an alternative to trie data structures when memory efficiency is a concern.
5. Radix Tree: A radix tree, also known as a compacted trie or a prefix tree, is a data structure that stores strings based on their prefixes. It can be an alternative to trie data structures when space efficiency is a priority, as it shares common prefixes among strings.
Choosing the right data structure depends on factors like the specific requirements of the application, the nature of the data being stored, the expected search and retrieval patterns, and the available memory resources.
Additional Resources
– Wikipedia – Trie
– GeeksforGeeks – Trie Data Structure
– Baeldung – Understanding Tries in Java