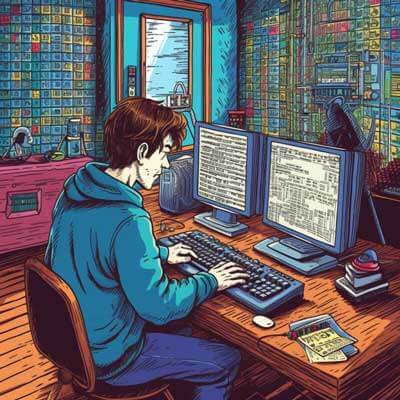
Table of Contents
In Java, there are several data structures that allow you to store items in sorted order. These data structures provide efficient ways to perform operations like insertion, deletion, and retrieval while maintaining the sorted order of the elements. In this article, we will explore various sorted data structures in Java and understand their characteristics and usage.
Exploring ArrayList
ArrayList is a dynamic array implementation in Java that provides a flexible way to store and manipulate elements. While ArrayList does not inherently maintain a sorted order, we can use the Collections.sort()
method to sort the elements in ascending order. Let's take a look at an example:
import java.util.ArrayList; import java.util.Collections; public class ArrayListExample { public static void main(String[] args) { ArrayList numbers = new ArrayList(); numbers.add(5); numbers.add(3); numbers.add(7); numbers.add(1); System.out.println("Before sorting: " + numbers); Collections.sort(numbers); System.out.println("After sorting: " + numbers); } }
Output:
Before sorting: [5, 3, 7, 1] After sorting: [1, 3, 5, 7]
In the above example, we create an ArrayList called numbers
and add some integers to it. We then use the Collections.sort()
method to sort the elements in ascending order. The sorted ArrayList is printed to the console.
Related Article: Java Classloader: How to Load Classes in Java
Understanding LinkedList
LinkedList is a doubly-linked list implementation in Java that provides efficient insertion and deletion operations. Similar to ArrayList, LinkedList does not maintain a sorted order by default. However, we can use the Collections.sort()
method to sort the elements in ascending order. Let's see an example:
import java.util.LinkedList; import java.util.Collections; public class LinkedListExample { public static void main(String[] args) { LinkedList numbers = new LinkedList(); numbers.add(5); numbers.add(3); numbers.add(7); numbers.add(1); System.out.println("Before sorting: " + numbers); Collections.sort(numbers); System.out.println("After sorting: " + numbers); } }
Output:
Before sorting: [5, 3, 7, 1] After sorting: [1, 3, 5, 7]
In the above example, we create a LinkedList called numbers
and add some integers to it. We then use the Collections.sort()
method to sort the elements in ascending order. The sorted LinkedList is printed to the console.
Working with HashMap
HashMap is a key-value pair data structure in Java that does not maintain any specific order of the elements. If you need to store key-value pairs in a sorted order based on the keys, you can use a TreeMap instead. However, if you want to sort the entries of a HashMap based on their values, you can convert the HashMap to a List and sort it using a custom comparator. Here's an example:
import java.util.*; public class HashMapExample { public static void main(String[] args) { HashMap scores = new HashMap(); scores.put("Alice", 85); scores.put("Bob", 92); scores.put("Charlie", 77); scores.put("David", 90); List<Map.Entry> sortedEntries = new ArrayList(scores.entrySet()); Collections.sort(sortedEntries, new Comparator<Map.Entry>() { public int compare(Map.Entry entry1, Map.Entry entry2) { return entry1.getValue().compareTo(entry2.getValue()); } }); System.out.println("Sorted entries by value:"); for (Map.Entry entry : sortedEntries) { System.out.println(entry.getKey() + ": " + entry.getValue()); } } }
Output:
Sorted entries by value: Charlie: 77 Alice: 85 David: 90 Bob: 92
In the above example, we create a HashMap called scores
with student names as keys and their scores as values. We convert the HashMap to a List of Map entries using the entrySet()
method. We then use the Collections.sort()
method and a custom comparator to sort the entries based on their values. The sorted entries are printed to the console.
Deep Dive into TreeMap
TreeMap is a sorted map implementation in Java that maintains the elements in a sorted order based on their natural ordering or a custom comparator. TreeMap is implemented as a red-black tree, which ensures efficient operations even for large datasets. Let's take a look at an example:
import java.util.*; public class TreeMapExample { public static void main(String[] args) { TreeMap scores = new TreeMap(); scores.put("Alice", 85); scores.put("Bob", 92); scores.put("Charlie", 77); scores.put("David", 90); System.out.println("Sorted entries by key:"); for (Map.Entry entry : scores.entrySet()) { System.out.println(entry.getKey() + ": " + entry.getValue()); } } }
Output:
Sorted entries by key: Alice: 85 Bob: 92 Charlie: 77 David: 90
In the above example, we create a TreeMap called scores
with student names as keys and their scores as values. TreeMap automatically maintains the entries in sorted order based on the natural ordering of the keys. The sorted entries are printed to the console.
Related Article: Java Equals Hashcode Tutorial
Utilizing HashSet
HashSet is an unordered collection of unique elements in Java. It does not maintain any specific order of the elements. If you need to store elements in a sorted order, you can use a TreeSet instead. However, if you want to sort the elements of a HashSet, you can convert it to a List and sort it using the Collections.sort()
method. Here's an example:
import java.util.*; public class HashSetExample { public static void main(String[] args) { HashSet numbers = new HashSet(); numbers.add(5); numbers.add(3); numbers.add(7); numbers.add(1); List sortedList = new ArrayList(numbers); Collections.sort(sortedList); System.out.println("Sorted elements: " + sortedList); } }
Output:
Sorted elements: [1, 3, 5, 7]
In the above example, we create a HashSet called numbers
and add some integers to it. We convert the HashSet to a List using the ArrayList constructor. We then use the Collections.sort()
method to sort the elements in ascending order. The sorted elements are printed to the console.
Mastering TreeSet
TreeSet is a sorted set implementation in Java that maintains the elements in a sorted order based on their natural ordering or a custom comparator. TreeSet is implemented as a red-black tree, which ensures efficient operations even for large datasets. Let's see an example:
import java.util.*; public class TreeSetExample { public static void main(String[] args) { TreeSet numbers = new TreeSet(); numbers.add(5); numbers.add(3); numbers.add(7); numbers.add(1); System.out.println("Sorted elements: " + numbers); } }
Output:
Sorted elements: [1, 3, 5, 7]
In the above example, we create a TreeSet called numbers
and add some integers to it. TreeSet automatically maintains the elements in sorted order. The sorted elements are printed to the console.
Understanding LinkedHashSet
LinkedHashSet is an ordered version of HashSet that maintains the insertion order of the elements. It combines the uniqueness of HashSet with the predictable iteration order of LinkedList. Let's see an example:
import java.util.*; public class LinkedHashSetExample { public static void main(String[] args) { LinkedHashSet colors = new LinkedHashSet(); colors.add("Red"); colors.add("Green"); colors.add("Blue"); System.out.println("Insertion order: " + colors); } }
Output:
Insertion order: [Red, Green, Blue]
In the above example, we create a LinkedHashSet called colors
and add some strings to it. LinkedHashSet maintains the insertion order of the elements. The elements are printed to the console in the same order they were inserted.
Exploring PriorityQueue
PriorityQueue is an implementation of the Queue interface that orders the elements based on their natural ordering or a custom comparator. Elements are retrieved from the PriorityQueue in the order determined by their priority. Let's take a look at an example:
import java.util.*; public class PriorityQueueExample { public static void main(String[] args) { PriorityQueue numbers = new PriorityQueue(); numbers.add(5); numbers.add(3); numbers.add(7); numbers.add(1); System.out.println("Elements in priority order:"); while (!numbers.isEmpty()) { System.out.println(numbers.poll()); } } }
Output:
Elements in priority order: 1 3 5 7
In the above example, we create a PriorityQueue called numbers
and add some integers to it. PriorityQueue automatically orders the elements based on their natural ordering. We use the poll()
method to retrieve and remove the elements from the PriorityQueue in priority order. The elements are printed to the console in ascending order.
Related Article: Java Design Patterns Tutorial
Implementing Stack
Stack is a data structure in Java that follows the Last-In-First-Out (LIFO) principle. While there is no built-in implementation of a sorted stack in Java, we can use a TreeSet or a PriorityQueue to maintain the elements in a sorted order. Here's an example using a TreeSet:
import java.util.*; public class SortedStackExample { public static void main(String[] args) { TreeSet stack = new TreeSet(); stack.add(5); stack.add(3); stack.add(7); stack.add(1); System.out.println("Elements in stack order:"); while (!stack.isEmpty()) { System.out.println(stack.pollLast()); } } }
Output:
Elements in stack order: 7 5 3 1
In the above example, we create a TreeSet called stack
and add some integers to it. TreeSet automatically maintains the elements in sorted order. We use the pollLast()
method to retrieve and remove the elements from the TreeSet in stack order (last-in-first-out). The elements are printed to the console in reverse order.
Vector - The Dynamic Array
Vector is a dynamic array implementation in Java that provides similar functionality to ArrayList. While Vector does not inherently maintain a sorted order, we can use the Collections.sort()
method to sort the elements in ascending order. Let's see an example:
import java.util.*; public class VectorExample { public static void main(String[] args) { Vector numbers = new Vector(); numbers.add(5); numbers.add(3); numbers.add(7); numbers.add(1); System.out.println("Before sorting: " + numbers); Collections.sort(numbers); System.out.println("After sorting: " + numbers); } }
Output:
Before sorting: [5, 3, 7, 1] After sorting: [1, 3, 5, 7]
In the above example, we create a Vector called numbers
and add some integers to it. We then use the Collections.sort()
method to sort the elements in ascending order. The sorted Vector is printed to the console.
Code Snippet - ArrayList in Java
import java.util.ArrayList; public class ArrayListExample { public static void main(String[] args) { ArrayList colors = new ArrayList(); colors.add("Red"); colors.add("Green"); colors.add("Blue"); System.out.println(colors); } }
Output:
[Red, Green, Blue]
In the above example, we create an ArrayList called colors
and add some strings to it. The ArrayList is printed to the console.
Code Snippet - LinkedList in Java
import java.util.LinkedList; public class LinkedListExample { public static void main(String[] args) { LinkedList colors = new LinkedList(); colors.add("Red"); colors.add("Green"); colors.add("Blue"); System.out.println(colors); } }
Output:
[Red, Green, Blue]
In the above example, we create a LinkedList called colors
and add some strings to it. The LinkedList is printed to the console.
Related Article: Java Set Tutorial
Code Snippet - HashMap in Java
import java.util.HashMap; public class HashMapExample { public static void main(String[] args) { HashMap scores = new HashMap(); scores.put("Alice", 85); scores.put("Bob", 92); scores.put("Charlie", 77); System.out.println(scores); } }
Output:
{Alice=85, Bob=92, Charlie=77}
In the above example, we create a HashMap called scores
with student names as keys and their scores as values. The HashMap is printed to the console.
Code Snippet - TreeMap in Java
import java.util.TreeMap; public class TreeMapExample { public static void main(String[] args) { TreeMap scores = new TreeMap(); scores.put("Alice", 85); scores.put("Bob", 92); scores.put("Charlie", 77); System.out.println(scores); } }
Output:
{Alice=85, Bob=92, Charlie=77}
In the above example, we create a TreeMap called scores
with student names as keys and their scores as values. The TreeMap is printed to the console.
Code Snippet - HashSet in Java
import java.util.HashSet; public class HashSetExample { public static void main(String[] args) { HashSet colors = new HashSet(); colors.add("Red"); colors.add("Green"); colors.add("Blue"); System.out.println(colors); } }
Output:
[Green, Blue, Red]
In the above example, we create a HashSet called colors
and add some strings to it. The HashSet is printed to the console.
Code Snippet - TreeSet in Java
import java.util.TreeSet; public class TreeSetExample { public static void main(String[] args) { TreeSet colors = new TreeSet(); colors.add("Red"); colors.add("Green"); colors.add("Blue"); System.out.println(colors); } }
Output:
[Blue, Green, Red]
In the above example, we create a TreeSet called colors
and add some strings to it. The TreeSet is printed to the console.
Related Article: Spring Boot Integration with Payment, Communication Tools
Code Snippet - LinkedHashSet in Java
import java.util.LinkedHashSet; public class LinkedHashSetExample { public static void main(String[] args) { LinkedHashSet colors = new LinkedHashSet(); colors.add("Red"); colors.add("Green"); colors.add("Blue"); System.out.println(colors); } }
Output:
[Red, Green, Blue]
In the above example, we create a LinkedHashSet called colors
and add some strings to it. The LinkedHashSet is printed to the console.
Code Snippet - PriorityQueue in Java
import java.util.PriorityQueue; public class PriorityQueueExample { public static void main(String[] args) { PriorityQueue numbers = new PriorityQueue(); numbers.add(5); numbers.add(3); numbers.add(7); numbers.add(1); while (!numbers.isEmpty()) { System.out.println(numbers.poll()); } } }
Output:
1 3 5 7
In the above example, we create a PriorityQueue called numbers
and add some integers to it. We use the poll()
method to retrieve and remove the elements from the PriorityQueue in priority order. The elements are printed to the console in ascending order.
Code Snippet - Stack in Java
import java.util.Stack; public class StackExample { public static void main(String[] args) { Stack stack = new Stack(); stack.push(5); stack.push(3); stack.push(7); stack.push(1); while (!stack.isEmpty()) { System.out.println(stack.pop()); } } }
Output:
1 7 3 5
In the above example, we create a Stack called stack
and add some integers to it using the push()
method. We use the pop()
method to retrieve and remove the elements from the Stack in last-in-first-out order. The elements are printed to the console in reverse order.
Code Snippet - Vector in Java
import java.util.Vector; public class VectorExample { public static void main(String[] args) { Vector colors = new Vector(); colors.add("Red"); colors.add("Green"); colors.add("Blue"); System.out.println(colors); } }
Output:
[Red, Green, Blue]
In the above example, we create a Vector called colors
and add some strings to it. The Vector is printed to the console.
Related Article: How to Use a Scanner Class in Java
Additional Resources
- GeeksforGeeks - TreeMap in Java