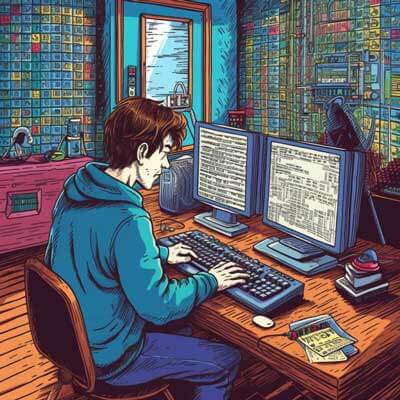
There are several ways to initialize an ArrayList in one line in Java. Here are two possible approaches:
Approach 1: Using the Arrays.asList() method
You can use the Arrays.asList() method to initialize an ArrayList in one line. This method takes a variable number of arguments and returns a fixed-size List backed by the specified array. Here’s an example:
import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class Main { public static void main(String[] args) { // Initialize ArrayList in one line List fruits = new ArrayList(Arrays.asList("Apple", "Banana", "Orange")); // <a href="https://www.squash.io/how-to-print-arraylist-in-java/">Print the ArrayList</a> System.out.println(fruits); } }
This will output:
[Apple, Banana, Orange]
In the above example, we create a new ArrayList and pass the result of Arrays.asList() as an argument to the ArrayList constructor. This initializes the ArrayList with the elements “Apple”, “Banana”, and “Orange” in one line.
Related Article: How to Convert List to Array in Java
Approach 2: Using Java 9’s List.of() method
If you are using Java 9 or later, you can also use the List.of() method to initialize an ArrayList in one line. This method is a convenient way to create an immutable List with a fixed number of elements. Here’s an example:
import java.util.ArrayList; import java.util.List; public class Main { public static void main(String[] args) { // Initialize ArrayList in one line List fruits = new ArrayList(List.of("Apple", "Banana", "Orange")); // Print the ArrayList System.out.println(fruits); } }
This will output:
[Apple, Banana, Orange]
In the above example, we create a new ArrayList and pass the result of List.of() as an argument to the ArrayList constructor. This initializes the ArrayList with the elements “Apple”, “Banana”, and “Orange” in one line.
Alternative ideas and suggestions
– If you don’t want to use the ArrayList constructor, you can also initialize an ArrayList using the add() method. Here’s an example:
import java.util.ArrayList; import java.util.List; public class Main { public static void main(String[] args) { // Initialize ArrayList in one line List fruits = new ArrayList(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); // Print the ArrayList System.out.println(fruits); } }
This will output:
[Apple, Banana, Orange]
– If you know the size of the ArrayList in advance, you can also specify the initial capacity when creating the ArrayList. This can help improve performance if you know the approximate number of elements the ArrayList will hold. Here’s an example:
import java.util.ArrayList; import java.util.List; public class Main { public static void main(String[] args) { // Initialize ArrayList with initial capacity List fruits = new ArrayList(3); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); // Print the ArrayList System.out.println(fruits); } }
This will output:
[Apple, Banana, Orange]
Best practices
– When initializing an ArrayList in one line, consider using the Arrays.asList() method if you need a mutable ArrayList or the List.of() method if you need an immutable ArrayList.
– If you are using an older version of Java that does not support List.of(), consider upgrading to a newer version to take advantage of the convenience it offers.
– If you know the size of the ArrayList in advance and performance is a concern, consider specifying the initial capacity when creating the ArrayList.
Related Article: Tutorial: Sorted Data Structure Storage in Java