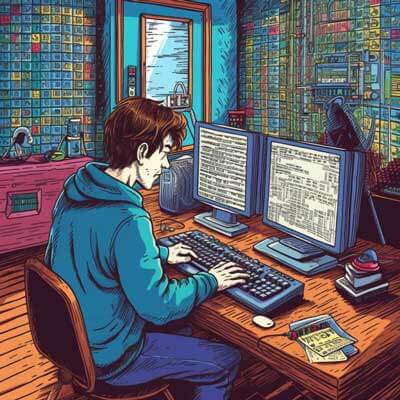
- Introduction to Command Line Arguments
- Basics of Command Line Arguments
- Command Line Arguments: Syntax and Structure
- Use Case 1: Command Line Argument Processing
- Real World Example 1: Using Command Line Arguments in Applications
- Best Practice 1: Validation of Command Line Inputs
- Performance Consideration 1: Efficient Use of Command Line Arguments
- Advanced Technique 1: Parsing Complex Command Line Arguments
- Code Snippet 1: Basic Command Line Argument Parsing
- Code Snippet 2: Validation of Command Line Inputs
- Code Snippet 3: Advanced Parsing of Complex Command Line Arguments
- Code Snippet 4: Using Command Line Arguments for Configuration Settings
- Code Snippet 5: Handling Errors in Command Line Argument Parsing
- Error Handling: Exception Handling and Reporting
- Use Case 2: Command Line Arguments for Configuration Settings
- Real World Example 2: Command Line Arguments in Large Scale Systems
- Best Practice 2: Using Descriptive and Consistent Argument Naming
- Performance Consideration 2: Impact of Command Line Arguments on Startup Time
- Advanced Technique 2: Using Command Line Arguments in Conjunction with Configuration Files
Introduction to Command Line Arguments
Command line arguments are a way to pass additional input parameters to a program when it is executed from the command line or terminal. They allow users to customize the behavior of a program without modifying its source code. In Java, command line arguments are passed as strings to the main method of the program’s entry point class.
Related Article: How To Parse JSON In Java
Basics of Command Line Arguments
To understand command line arguments in Java, let’s consider a simple example. Suppose we have a program that calculates the sum of two numbers. We can pass these numbers as command line arguments.
Here is a code snippet that demonstrates how to access command line arguments in Java:
public class CommandLineArgumentsExample { public static void main(String[] args) { // Check if command line arguments are provided if (args.length < 2) { System.out.println("Please provide two numbers as command line arguments."); return; } // Parse the command line arguments int num1 = Integer.parseInt(args[0]); int num2 = Integer.parseInt(args[1]); // Calculate the sum int sum = num1 + num2; // Print the result System.out.println("Sum: " + sum); } }
In this example, the program checks if two command line arguments are provided. If not, it displays an error message and exits. If two arguments are provided, it parses them as integers and calculates their sum.
To run this program with command line arguments, open a terminal or command prompt, navigate to the directory containing the Java file, and execute the following command:
java CommandLineArgumentsExample 10 20
This command will pass the numbers 10 and 20 as command line arguments to the program. The output will be:
Sum: 30
Command Line Arguments: Syntax and Structure
Command line arguments in Java are passed as an array of strings to the main method. The syntax for executing a Java program with command line arguments is as follows:
java ClassName arg1 arg2 ...
– java
: The command to execute the Java Virtual Machine (JVM).
– ClassName
: The name of the class containing the main method.
– arg1
, arg2
, …: The command line arguments to be passed to the program.
The command line arguments are accessed within the main method using the args
parameter, which is an array of strings. Each element of the args
array corresponds to a command line argument, starting from index 0.
For example, if we run a program named MyProgram
with three command line arguments:
java MyProgram arg1 arg2 arg3
The args
array within the main method will contain the following values:
– args[0]
: “arg1”
– args[1]
: “arg2”
– args[2]
: “arg3”
It’s important to note that command line arguments are always passed as strings. If you need to use them as other data types, such as integers or booleans, you will need to convert them explicitly.
Use Case 1: Command Line Argument Processing
Command line arguments are widely used in various scenarios. One common use case is to provide input parameters to configure the behavior of a program. For example, a command line argument can be used to specify a file path, a database connection string, or a configuration option.
Let’s consider an example where we have a program that reads a text file and counts the number of occurrences of a specific word. We can pass the file path and the word to search as command line arguments.
Here is a code snippet that demonstrates how to process command line arguments for this use case:
import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; public class WordCount { public static void main(String[] args) { // Check if command line arguments are provided if (args.length < 2) { System.out.println("Please provide the file path and the word to search as command line arguments."); return; } // Get the file path and the word to search from command line arguments String filePath = args[0]; String wordToSearch = args[1]; // Read the file and count the occurrences of the word int count = 0; try (BufferedReader reader = new BufferedReader(new FileReader(filePath))) { String line; while ((line = reader.readLine()) != null) { String[] words = line.split("\\s+"); for (String word : words) { if (word.equalsIgnoreCase(wordToSearch)) { count++; } } } } catch (IOException e) { System.out.println("Error reading the file: " + e.getMessage()); return; } // Print the result System.out.println("Occurrences of \"" + wordToSearch + "\": " + count); } }
In this example, the program checks if two command line arguments (file path and word to search) are provided. If not, it displays an error message and exits. If the arguments are provided, it reads the file specified by the file path and counts the occurrences of the word.
To run this program with command line arguments, execute the following command:
java WordCount input.txt hello
This command will pass the file path “input.txt” and the word “hello” as command line arguments to the program. The output will be the number of occurrences of the word “hello” in the file.
Related Article: How To Convert Array To List In Java
Real World Example 1: Using Command Line Arguments in Applications
Command line arguments are widely used in real-world applications for various purposes. One common use case is to configure the behavior of the application based on user input.
Let’s consider an example where we have a command line application that performs file operations, such as copying, moving, and deleting files. We can pass the operation type and the file paths as command line arguments.
Here is a code snippet that demonstrates how to use command line arguments in this real-world example:
import java.io.File; import java.io.IOException; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.StandardCopyOption; public class FileOperations { public static void main(String[] args) { // Check if command line arguments are provided if (args.length < 3) { System.out.println("Please provide the operation type (copy/move/delete) and the file paths as command line arguments."); return; } // Get the operation type and the file paths from command line arguments String operationType = args[0]; String[] filePaths = new String[args.length - 1]; System.arraycopy(args, 1, filePaths, 0, args.length - 1); // Perform the specified operation on the files switch (operationType) { case "copy": copyFiles(filePaths); break; case "move": moveFiles(filePaths); break; case "delete": deleteFiles(filePaths); break; default: System.out.println("Invalid operation type. Supported types are: copy, move, delete."); } } private static void copyFiles(String[] filePaths) { // Perform file copy operation // ... } private static void moveFiles(String[] filePaths) { // Perform file move operation // ... } private static void deleteFiles(String[] filePaths) { // Perform file delete operation // ... } }
In this example, the program checks if at least three command line arguments (operation type and file paths) are provided. If not, it displays an error message and exits. If the arguments are provided, it performs the specified file operation (copy, move, or delete) on the specified files.
To run this program with command line arguments, execute commands like the following:
java FileOperations copy file1.txt file2.txt file3.txt destinationFolder/ java FileOperations move file1.txt file2.txt file3.txt destinationFolder/ java FileOperations delete file1.txt file2.txt file3.txt
These commands will pass the operation type and the file paths as command line arguments to the program. The program will perform the specified file operation accordingly.
Best Practice 1: Validation of Command Line Inputs
When working with command line arguments, it’s important to validate the inputs to ensure they are correct and meet the expected requirements. Proper validation helps prevent errors and unexpected behavior.
Here is a code snippet that demonstrates how to validate command line inputs in Java:
public class CommandLineValidation { public static void main(String[] args) { // Check if command line arguments are provided if (args.length < 2) { System.out.println("Please provide two numbers as command line arguments."); return; } // Validate the format of the command line arguments try { int num1 = Integer.parseInt(args[0]); int num2 = Integer.parseInt(args[1]); } catch (NumberFormatException e) { System.out.println("Invalid number format. Please provide valid numbers as command line arguments."); return; } // Parse the command line arguments int num1 = Integer.parseInt(args[0]); int num2 = Integer.parseInt(args[1]); // Calculate the sum int sum = num1 + num2; // Print the result System.out.println("Sum: " + sum); } }
In this example, before parsing the command line arguments as integers, we validate the format of the arguments using a try-catch block. If the format is invalid (e.g., non-numeric values), an error message is displayed, and the program exits.
Validating command line inputs is essential to ensure that the program operates correctly and to provide meaningful error messages to users when invalid inputs are provided.
Performance Consideration 1: Efficient Use of Command Line Arguments
When using command line arguments, it’s important to consider performance implications, especially when dealing with large amounts of data or complex operations.
One performance consideration is the size of the command line arguments. Command line arguments are passed as strings, so if the arguments contain large amounts of data, it can consume significant memory. It’s recommended to keep the command line arguments concise and avoid passing unnecessary data.
Another consideration is the efficiency of processing command line arguments. If the program requires complex parsing or manipulation of the arguments, it’s important to optimize the algorithms and data structures used for processing. Inefficient processing can lead to slower execution times and decreased performance.
Here is an example that demonstrates efficient use of command line arguments:
public class EfficientArgumentsProcessing { public static void main(String[] args) { // Check if command line arguments are provided if (args.length < 2) { System.out.println("Please provide two numbers as command line arguments."); return; } // Parse the command line arguments directly without additional processing int num1 = Integer.parseInt(args[0]); int num2 = Integer.parseInt(args[1]); // Calculate the sum int sum = num1 + num2; // Print the result System.out.println("Sum: " + sum); } }
In this example, the program directly parses the command line arguments as integers without any additional processing or validation. This approach minimizes the processing overhead and improves performance.
By efficiently using command line arguments, you can optimize the performance of your Java programs and ensure they run smoothly even with large or complex inputs.
Related Article: How To Iterate Over Entries In A Java Map
Advanced Technique 1: Parsing Complex Command Line Arguments
In some cases, command line arguments may have complex structures that require advanced parsing techniques. For example, arguments may be in the form of key-value pairs, structured data, or nested structures.
To parse complex command line arguments, libraries such as Apache Commons CLI or JCommander can be used. These libraries provide convenient APIs for defining and parsing command line options and arguments.
Here is an example that demonstrates parsing complex command line arguments using Apache Commons CLI:
import org.apache.commons.cli.*; public class ComplexArgumentsParsing { public static void main(String[] args) { // Define the command line options Options options = new Options(); options.addOption("f", "file", true, "File path"); options.addOption("o", "output", true, "Output directory"); // Create the command line parser CommandLineParser parser = new DefaultParser(); try { // Parse the command line arguments CommandLine cmd = parser.parse(options, args); // Get the values of the options String filePath = cmd.getOptionValue("file"); String outputDir = cmd.getOptionValue("output"); // Process the values // ... } catch (ParseException e) { System.out.println("Error parsing command line arguments: " + e.getMessage()); } } }
In this example, we define two options: file
and output
. The file
option expects a file path as its value, and the output
option expects an output directory path. The Apache Commons CLI library handles the parsing of the command line arguments and provides convenient methods to retrieve the option values.
By using advanced techniques like this, you can handle complex command line arguments in a structured and efficient manner.
Code Snippet 1: Basic Command Line Argument Parsing
Here is a basic code snippet that demonstrates how to parse command line arguments in Java:
public class CommandLineArgumentsParsing { public static void main(String[] args) { // Check if command line arguments are provided if (args.length < 2) { System.out.println("Please provide two numbers as command line arguments."); return; } // Parse the command line arguments int num1 = Integer.parseInt(args[0]); int num2 = Integer.parseInt(args[1]); // Calculate the sum int sum = num1 + num2; // Print the result System.out.println("Sum: " + sum); } }
In this code snippet, we check if two command line arguments are provided. If not, an error message is displayed. If the arguments are provided, they are parsed as integers, and their sum is calculated and printed.
To run this code snippet with command line arguments, execute the following command:
java CommandLineArgumentsParsing 10 20
This command will pass the numbers 10 and 20 as command line arguments to the program, which will calculate their sum and print it.
Code Snippet 2: Validation of Command Line Inputs
Here is a code snippet that demonstrates how to validate command line inputs in Java:
public class CommandLineInputValidation { public static void main(String[] args) { // Check if command line arguments are provided if (args.length < 2) { System.out.println("Please provide two numbers as command line arguments."); return; } // Validate the format of the command line arguments try { int num1 = Integer.parseInt(args[0]); int num2 = Integer.parseInt(args[1]); } catch (NumberFormatException e) { System.out.println("Invalid number format. Please provide valid numbers as command line arguments."); return; } // Parse the command line arguments int num1 = Integer.parseInt(args[0]); int num2 = Integer.parseInt(args[1]); // Calculate the sum int sum = num1 + num2; // Print the result System.out.println("Sum: " + sum); } }
In this code snippet, we first check if two command line arguments are provided. If not, an error message is displayed. If the arguments are provided, we validate their format by attempting to parse them as integers. If the parsing fails (e.g., non-numeric values are provided), an error message is displayed.
Proper validation of command line inputs helps ensure that the program functions correctly and provides meaningful feedback to users when invalid inputs are provided.
Related Article: How To Split A String In Java
Code Snippet 3: Advanced Parsing of Complex Command Line Arguments
Here is a code snippet that demonstrates advanced parsing of complex command line arguments using Apache Commons CLI:
import org.apache.commons.cli.*; public class ComplexArgumentsParsing { public static void main(String[] args) { // Define the command line options Options options = new Options(); options.addOption("f", "file", true, "File path"); options.addOption("o", "output", true, "Output directory"); // Create the command line parser CommandLineParser parser = new DefaultParser(); try { // Parse the command line arguments CommandLine cmd = parser.parse(options, args); // Get the values of the options String filePath = cmd.getOptionValue("file"); String outputDir = cmd.getOptionValue("output"); // Process the values // ... } catch (ParseException e) { System.out.println("Error parsing command line arguments: " + e.getMessage()); } } }
In this code snippet, we define two options: file
and output
. The file
option expects a file path as its value, and the output
option expects an output directory path. The Apache Commons CLI library handles the parsing of the command line arguments and provides convenient methods to retrieve the option values.
By using advanced parsing techniques like this, you can handle complex command line arguments in a structured and efficient manner.
Code Snippet 4: Using Command Line Arguments for Configuration Settings
Here is a code snippet that demonstrates how to use command line arguments for configuration settings:
public class ConfigurationSettings { public static void main(String[] args) { // Default configuration values String serverUrl = "http://localhost:8080"; int timeout = 5000; // Process command line arguments to override default values for (int i = 0; i < args.length; i += 2) { String option = args[i]; String value = args[i + 1]; switch (option) { case "-url": serverUrl = value; break; case "-timeout": timeout = Integer.parseInt(value); break; default: System.out.println("Invalid option: " + option); return; } } // Use the configuration values System.out.println("Server URL: " + serverUrl); System.out.println("Timeout: " + timeout); } }
In this code snippet, we define default configuration values for a server URL and a timeout. The program processes the command line arguments to override the default values. The supported options are -url
for specifying the server URL and -timeout
for specifying the timeout value.
To run this code snippet with command line arguments, execute a command like the following:
java ConfigurationSettings -url http://example.com -timeout 10000
This command will override the default server URL with “http://example.com” and the default timeout with 10000. The program will then use these values for further processing.
Code Snippet 5: Handling Errors in Command Line Argument Parsing
Here is a code snippet that demonstrates handling errors in command line argument parsing:
public class ErrorHandling { public static void main(String[] args) { // Check if command line arguments are provided if (args.length < 2) { System.out.println("Please provide two numbers as command line arguments."); return; } try { // Parse the command line arguments int num1 = Integer.parseInt(args[0]); int num2 = Integer.parseInt(args[1]); // Calculate the sum int sum = num1 + num2; // Print the result System.out.println("Sum: " + sum); } catch (NumberFormatException e) { System.out.println("Invalid number format. Please provide valid numbers as command line arguments."); } } }
In this code snippet, we check if two command line arguments are provided. If not, an error message is displayed. If the arguments are provided, we attempt to parse them as integers. If the parsing fails (e.g., non-numeric values are provided), an error message is displayed.
By handling errors gracefully, we can provide meaningful feedback to users and ensure the program behaves as expected even in the presence of invalid inputs.
Related Article: How To Convert Java Objects To JSON With Jackson
Error Handling: Exception Handling and Reporting
When working with command line arguments, it’s important to handle exceptions and report errors appropriately. Exceptions can occur when parsing command line arguments, converting their values, or performing operations based on the arguments.
To handle exceptions and report errors effectively, you can use try-catch blocks and appropriate error messages. By providing informative error messages, you can help users understand the cause of the error and take corrective actions.
Here is an example that demonstrates exception handling and error reporting:
public class ExceptionHandling { public static void main(String[] args) { try { // Parse the command line arguments int num1 = Integer.parseInt(args[0]); int num2 = Integer.parseInt(args[1]); // Calculate the division double division = num1 / num2; // Print the result System.out.println("Division: " + division); } catch (ArrayIndexOutOfBoundsException e) { System.out.println("Please provide two numbers as command line arguments."); } catch (NumberFormatException e) { System.out.println("Invalid number format. Please provide valid numbers as command line arguments."); } catch (ArithmeticException e) { System.out.println("Division by zero is not allowed."); } } }
In this example, we attempt to parse the command line arguments as integers and calculate their division. We use separate catch blocks for different types of exceptions that may occur. Depending on the type of exception, an appropriate error message is displayed.
By handling exceptions and reporting errors effectively, you can ensure your Java programs using command line arguments are robust and provide a smooth user experience.
Use Case 2: Command Line Arguments for Configuration Settings
Another common use case for command line arguments is configuring the behavior of an application through runtime settings. Command line arguments can be used to specify various settings, such as logging levels, database connection details, or application-specific preferences.
Let’s consider an example where we have a multi-threaded application that performs parallel processing. We can pass the number of threads as a command line argument to configure the degree of parallelism.
Here is a code snippet that demonstrates using command line arguments for configuration settings:
public class ConfigurationSettings { public static void main(String[] args) { // Default configuration values int numThreads = 1; // Process command line arguments to override default values if (args.length > 0) { numThreads = Integer.parseInt(args[0]); } // Use the configuration value System.out.println("Number of Threads: " + numThreads); } }
In this example, we define a default value of 1 for the number of threads. If a command line argument is provided, it is parsed as an integer and used to override the default value. The number of threads is then used for further processing.
To run this program with a command line argument, execute a command like the following:
java ConfigurationSettings 4
This command will pass the number 4 as a command line argument to the program, which will use it as the number of threads for parallel processing.
Real World Example 2: Command Line Arguments in Large Scale Systems
Command line arguments are widely used in large scale systems and enterprise applications to configure various aspects of the system behavior. They provide flexibility and allow system administrators to customize the application without modifying the source code.
In a real-world example, let’s consider a distributed system that consists of multiple microservices. Each microservice can have its own set of command line arguments to configure its behavior, such as database connection details, service endpoints, logging levels, and more.
Here is a simplified code snippet that demonstrates the usage of command line arguments in a microservice:
public class Microservice { public static void main(String[] args) { // Default configuration values String databaseUrl = "jdbc:mysql://localhost:3306/mydb"; String serviceEndpoint = "http://localhost:8080"; String logLevel = "INFO"; // Process command line arguments to override default values for (int i = 0; i < args.length; i += 2) { String option = args[i]; String value = args[i + 1]; switch (option) { case "-dburl": databaseUrl = value; break; case "-endpoint": serviceEndpoint = value; break; case "-loglevel": logLevel = value; break; default: System.out.println("Invalid option: " + option); return; } } // Configure the microservice based on the values // ... } }
In this example, the microservice has three configuration options: -dburl
for specifying the database URL, -endpoint
for specifying the service endpoint, and -loglevel
for specifying the logging level. The program processes the command line arguments to override the default values.
By using command line arguments, large scale systems can be easily configured and customized based on specific requirements, making them more flexible and adaptable.
Related Article: Storing Contact Information in Java Data Structures
Best Practice 2: Using Descriptive and Consistent Argument Naming
When defining command line arguments, it’s important to use descriptive and consistent argument names. This helps users understand the purpose of each argument and reduces confusion.
Descriptive argument names should clearly indicate the purpose or meaning of the argument. For example, instead of using generic names like -a
, -b
, and -c
, it’s better to use names like -input
, -output
, and -config
that convey their intended use.
Consistent argument naming ensures that similar arguments across different programs or modules have consistent names. This makes it easier for users to remember and reuse these arguments across different contexts.
Here is an example that demonstrates the use of descriptive and consistent argument naming:
public class ArgumentNaming { public static void main(String[] args) { // Process command line arguments for (int i = 0; i < args.length; i += 2) { String option = args[i]; String value = args[i + 1]; switch (option) { case "-input": processInput(value); break; case "-output": processOutput(value); break; case "-config": processConfiguration(value); break; default: System.out.println("Invalid option: " + option); return; } } } private static void processInput(String input) { // Process the input // ... } private static void processOutput(String output) { // Process the output // ... } private static void processConfiguration(String config) { // Process the configuration // ... } }
In this example, we use descriptive argument names like -input
, -output
, and -config
to indicate the purpose of each argument. By using meaningful names, users can easily understand what each argument expects.
Performance Consideration 2: Impact of Command Line Arguments on Startup Time
When designing command line interfaces, it’s important to consider the impact of command line arguments on the startup time of your application. Excessive or unnecessary command line arguments can increase the time it takes for your application to start.
To minimize the impact on startup time, you should only include the necessary command line arguments that are essential for the operation of your application. Avoid including arguments that are rarely used or can be configured through other means.
Additionally, you can optimize the processing of command line arguments by using efficient algorithms and data structures. Complex argument parsing or validation logic can introduce unnecessary overhead and slow down the startup time.
By carefully considering the impact of command line arguments on startup time, you can ensure your applications start quickly and provide a seamless user experience.
Advanced Technique 2: Using Command Line Arguments in Conjunction with Configuration Files
In some cases, using command line arguments alone may not be sufficient to configure the behavior of your application, especially for complex or extensive configuration settings. In such cases, you can use command line arguments in conjunction with configuration files to provide a more flexible and scalable solution.
Here is an example that demonstrates using command line arguments in conjunction with a configuration file:
import java.io.FileInputStream; import java.io.IOException; import java.util.Properties; public class ConfigurationFiles { public static void main(String[] args) { // Default configuration values String databaseUrl = "jdbc:mysql://localhost:3306/mydb"; String serviceEndpoint = "http://localhost:8080"; String logLevel = "INFO"; // Process command line arguments to override default values if (args.length > 0) { for (int i = 0; i < args.length; i += 2) { String option = args[i]; String value = args[i + 1]; switch (option) { case "-dburl": databaseUrl = value; break; case "-endpoint": serviceEndpoint = value; break; case "-loglevel": logLevel = value; break; default: System.out.println("Invalid option: " + option); return; } } } // Load additional configuration from a file Properties config = new Properties(); try (FileInputStream inputStream = new FileInputStream("config.properties")) { config.load(inputStream); } catch (IOException e) { System.out.println("Error loading configuration file: " + e.getMessage()); return; } // Override the default values with the values from the configuration file if (config.containsKey("dburl")) { databaseUrl = config.getProperty("dburl"); } if (config.containsKey("endpoint")) { serviceEndpoint = config.getProperty("endpoint"); } if (config.containsKey("loglevel")) { logLevel = config.getProperty("loglevel"); } // Use the configuration values System.out.println("Database URL: " + databaseUrl); System.out.println("Service Endpoint: " + serviceEndpoint); System.out.println("Log Level: " + logLevel); } }
In this example, we first process the command line arguments to override the default configuration values. Then, we load additional configuration from a file named “config.properties” using the Properties
class. The values in the configuration file can further override the command line arguments.