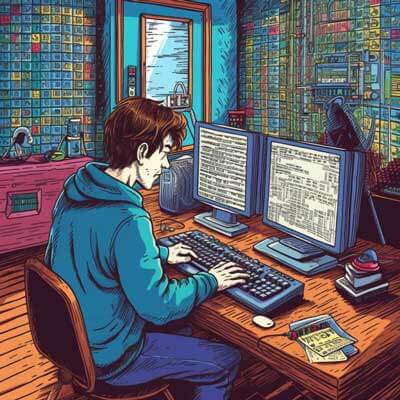
- Introduction to Serialization
- The Basics of Object Serialization
- Writing Serializable Classes
- The Role of serialVersionUID
- Customizing the Serialization Process
- Serialization and Inheritance
- Serialization and Aggregation
- Use Cases: Persisting User Sessions
- Use Cases: Storing Application Settings
- Use Cases: Implementing Undo Features
- Best Practices: Avoiding Serialization of Sensitive Data
- Best Practices: Considering Transient Keyword
- Best Practices: Using Externalizable Interface
- Real World Examples: Serialization in Distributed Systems
- Real World Examples: Serialization for Caching
- Performance Considerations: Impact on Memory
- Performance Considerations: Impact on Disk Space
- Performance Considerations: Impact on Network
- Advanced Techniques: Custom Serialization Formats
- Advanced Techniques: Versioning of Serialized Objects
- Code Snippet: Basic Serialization
- Code Snippet: Deserialization
- Code Snippet: Handling Serialization Errors
- Code Snippet: Customizing Serialization
- Code Snippet: Using Externalizable
- Error Handling: Dealing with Serialization Errors
- Error Handling: Managing Changes in Serializable Classes
Introduction to Serialization
Serialization is a fundamental concept in Java that allows objects to be converted into a stream of bytes. This stream of bytes can then be saved to a file, sent over a network, or stored in a database. Serialization is commonly used in scenarios where the state of an object needs to be persisted or transferred across different systems.
Serialization in Java works by converting the object’s state, including its instance variables, into a byte stream. This byte stream can then be reconstructed back into an object with the same state. The process of converting an object into a byte stream is called serialization, and the process of reconstructing an object from a byte stream is called deserialization.
Related Article: How To Parse JSON In Java
The Basics of Object Serialization
To serialize an object in Java, the class of the object must implement the Serializable interface. The Serializable interface acts as a marker interface, indicating that the objects of that class can be serialized.
Here’s an example of a simple class that implements the Serializable interface:
import java.io.Serializable; public class Person implements Serializable { private String name; private int age; public Person(String name, int age) { this.name = name; this.age = age; } // Getters and setters @Override public String toString() { return "Person{" + "name='" + name + '\'' + ", age=" + age + '}'; } }
In this example, the Person class has two instance variables: name and age. By implementing the Serializable interface, objects of the Person class can be serialized and deserialized.
Writing Serializable Classes
When a class implements the Serializable interface, all of its non-transient instance variables are automatically serialized. Transient variables are variables that should not be serialized and are marked with the transient keyword.
Here’s an example that demonstrates the serialization and deserialization of a Person object:
import java.io.*; public class SerializationExample { public static void main(String[] args) { Person person = new Person("John Doe", 30); // Serialization try (OutputStream fileOutputStream = new FileOutputStream("person.ser"); ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream)) { objectOutputStream.writeObject(person); System.out.println("Person object serialized successfully."); } catch (IOException e) { e.printStackTrace(); } // Deserialization try (InputStream fileInputStream = new FileInputStream("person.ser"); ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream)) { Person deserializedPerson = (Person) objectInputStream.readObject(); System.out.println("Deserialized Person object: " + deserializedPerson); } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } } }
In this example, a Person object is serialized and saved to a file named “person.ser”. Later, the object is deserialized from the file and printed to the console.
The Role of serialVersionUID
When a serialized object is deserialized, Java uses the serialVersionUID to verify that the version of the class used to serialize the object matches the version of the class used to deserialize the object. If the serialVersionUID of the serialized object does not match the serialVersionUID of the class being used for deserialization, an InvalidClassException is thrown.
By default, if a class does not explicitly declare a serialVersionUID, Java automatically generates one based on various aspects of the class, such as its name, fields, and methods. However, it is considered a best practice to explicitly declare a serialVersionUID to ensure compatibility between different versions of a class.
Here’s an example of a class with an explicitly declared serialVersionUID:
import java.io.Serializable; public class Person implements Serializable { private static final long serialVersionUID = 123456789L; // Rest of the class }
In this example, the serialVersionUID is set to 123456789L. If the class is modified in a way that affects its serialization compatibility, the serialVersionUID should be updated accordingly.
Related Article: How To Convert Array To List In Java
Customizing the Serialization Process
Java provides several mechanisms to customize the serialization process. Two common methods used to customize serialization are the writeObject() and readObject() methods.
The writeObject() method allows you to define custom serialization logic for a class. This method is automatically called during serialization and allows you to write additional fields or perform any necessary transformations before the object is serialized.
Here’s an example of a class that customizes the serialization process using the writeObject() method:
import java.io.IOException; import java.io.ObjectOutputStream; import java.io.Serializable; public class Person implements Serializable { private String name; private int age; // Constructor and other methods private void writeObject(ObjectOutputStream out) throws IOException { // Custom serialization logic out.writeObject(name); out.writeInt(age); } }
In this example, the writeObject() method is overridden to write the name and age fields directly to the ObjectOutputStream.
The readObject() method allows you to define custom deserialization logic for a class. This method is automatically called during deserialization and allows you to read additional fields or perform any necessary transformations after the object is deserialized.
Here’s an example of a class that customizes the deserialization process using the readObject() method:
import java.io.IOException; import java.io.ObjectInputStream; import java.io.Serializable; public class Person implements Serializable { private String name; private int age; // Constructor and other methods private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException { // Custom deserialization logic name = (String) in.readObject(); age = in.readInt(); } }
In this example, the readObject() method is overridden to read the name and age fields directly from the ObjectInputStream.
Serialization and Inheritance
When serializing an object that extends a superclass, both the superclass and subclass need to implement the Serializable interface. If the superclass is not serializable, a NotSerializableException will be thrown during serialization.
Here’s an example that demonstrates serialization and deserialization of a subclass:
import java.io.*; class Person implements Serializable { private String name; // Other fields and methods } class Employee extends Person { private String employeeId; // Other fields and methods } public class SerializationExample { public static void main(String[] args) { Employee employee = new Employee(); // Set employee fields // Serialization try (OutputStream fileOutputStream = new FileOutputStream("employee.ser"); ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream)) { objectOutputStream.writeObject(employee); System.out.println("Employee object serialized successfully."); } catch (IOException e) { e.printStackTrace(); } // Deserialization try (InputStream fileInputStream = new FileInputStream("employee.ser"); ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream)) { Employee deserializedEmployee = (Employee) objectInputStream.readObject(); System.out.println("Deserialized Employee object: " + deserializedEmployee); } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } } }
In this example, the Employee class extends the Person class, and both classes implement the Serializable interface. The Employee object is serialized and deserialized without any issues.
Serialization and Aggregation
When serializing an object that contains references to other objects (aggregation), those referenced objects must also be serializable. If a referenced object is not serializable, a NotSerializableException will be thrown during serialization.
Here’s an example that demonstrates serialization and deserialization of an object with aggregation:
import java.io.*; class Address implements Serializable { private String street; private String city; // Other fields and methods } class Person implements Serializable { private String name; private Address address; // Other fields and methods } public class SerializationExample { public static void main(String[] args) { Address address = new Address(); // Set address fields Person person = new Person(); person.setName("John Doe"); person.setAddress(address); // Serialization try (OutputStream fileOutputStream = new FileOutputStream("person.ser"); ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream)) { objectOutputStream.writeObject(person); System.out.println("Person object serialized successfully."); } catch (IOException e) { e.printStackTrace(); } // Deserialization try (InputStream fileInputStream = new FileInputStream("person.ser"); ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream)) { Person deserializedPerson = (Person) objectInputStream.readObject(); System.out.println("Deserialized Person object: " + deserializedPerson); } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } } }
In this example, the Person class has an Address object as an instance variable. Both the Person and Address classes implement the Serializable interface, allowing the object to be serialized and deserialized successfully.
Related Article: How To Iterate Over Entries In A Java Map
Use Cases: Persisting User Sessions
One common use case of serialization is persisting user sessions in web applications. By serializing the session objects, the application can store the session state in a database or file system, allowing the user to resume their session even after closing the browser.
Here’s an example of a simple web application that uses serialization to persist user sessions:
import javax.servlet.ServletException; import javax.servlet.http.*; import java.io.*; public class SessionExampleServlet extends HttpServlet { protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { HttpSession session = request.getSession(); session.setAttribute("username", "john_doe"); // Serialization try (OutputStream fileOutputStream = new FileOutputStream("session.ser"); ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream)) { objectOutputStream.writeObject(session); System.out.println("Session object serialized successfully."); } catch (IOException e) { e.printStackTrace(); } // Deserialization try (InputStream fileInputStream = new FileInputStream("session.ser"); ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream)) { HttpSession deserializedSession = (HttpSession) objectInputStream.readObject(); System.out.println("Deserialized Session object: " + deserializedSession); } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } // Rest of the servlet logic } }
In this example, the HttpSession object is serialized and saved to a file named “session.ser”. Later, the object is deserialized and printed to the console. This allows the web application to persist the user’s session state.
Use Cases: Storing Application Settings
Another common use case of serialization is storing application settings. By serializing the settings object, an application can save and load its configuration without the need for complex file parsing or database queries.
Here’s an example of a class that stores application settings and uses serialization to persist and load the settings:
import java.io.*; public class Settings implements Serializable { private int maxConnections; private String databaseUrl; // Other settings // Getters and setters public void saveToFile(String filename) { try (OutputStream fileOutputStream = new FileOutputStream(filename); ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream)) { objectOutputStream.writeObject(this); System.out.println("Settings object saved to file: " + filename); } catch (IOException e) { e.printStackTrace(); } } public static Settings loadFromFile(String filename) { Settings settings = null; try (InputStream fileInputStream = new FileInputStream(filename); ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream)) { settings = (Settings) objectInputStream.readObject(); System.out.println("Settings object loaded from file: " + filename); } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } return settings; } }
In this example, the Settings class has various application settings. The saveToFile() method allows the settings object to be serialized and saved to a file, while the loadFromFile() method allows the settings object to be deserialized from a file.
Use Cases: Implementing Undo Features
Serialization can also be used to implement undo features in applications. By serializing the state of an object before each operation, the application can restore the previous state by deserializing the object.
Here’s an example of a simple text editor that uses serialization to implement an undo feature:
import java.io.*; import java.util.*; class TextEditorState implements Serializable { private String text; private List<String> undoHistory; // Getters and setters public void saveState() { undoHistory.add(serialize()); } public void undo() { if (undoHistory.isEmpty()) { System.out.println("Nothing to undo."); return; } String lastState = undoHistory.remove(undoHistory.size() - 1); deserialize(lastState); System.out.println("Undo successful."); } private String serialize() { try (ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream(); ObjectOutputStream objectOutputStream = new ObjectOutputStream(byteArrayOutputStream)) { objectOutputStream.writeObject(this); return Base64.getEncoder().encodeToString(byteArrayOutputStream.toByteArray()); } catch (IOException e) { e.printStackTrace(); return null; } } private void deserialize(String serializedState) { byte[] bytes = Base64.getDecoder().decode(serializedState); try (ByteArrayInputStream byteArrayInputStream = new ByteArrayInputStream(bytes); ObjectInputStream objectInputStream = new ObjectInputStream(byteArrayInputStream)) { TextEditorState state = (TextEditorState) objectInputStream.readObject(); this.text = state.text; this.undoHistory = state.undoHistory; } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } } } public class TextEditor { private TextEditorState state; public TextEditor() { state = new TextEditorState(); state.setUndoHistory(new ArrayList<>()); } public void setText(String text) { state.setText(text); state.saveState(); } public void undo() { state.undo(); System.out.println("Current text: " + state.getText()); } }
In this example, the TextEditorState class represents the state of the text editor. The saveState() method serializes the state and adds it to the undo history. The undo() method removes the last serialized state from the undo history and restores the previous state by deserializing it.
The TextEditor class uses the TextEditorState to manage the text and undo operations. Each time the text is changed, the state is saved, allowing the user to undo their changes.
Related Article: How To Split A String In Java
Best Practices: Avoiding Serialization of Sensitive Data
When serializing objects, it’s important to consider the security implications. By default, all non-transient instance variables of a serializable class are serialized, including sensitive data such as passwords or private keys. To avoid serializing sensitive data, mark those variables as transient.
Here’s an example that demonstrates avoiding the serialization of sensitive data:
import java.io.Serializable; public class User implements Serializable { private String username; private transient String password; // Other fields and methods }
In this example, the password field is marked as transient, ensuring that it is not serialized when the User object is serialized.
Best Practices: Considering Transient Keyword
The transient keyword in Java is used to indicate that a field should not be serialized. Transient fields are excluded from the serialization process, and their values will not be saved or restored.
Here’s an example that demonstrates the use of the transient keyword:
import java.io.Serializable; public class Person implements Serializable { private String name; private transient int age; // Other fields and methods }
In this example, the age field is marked as transient, indicating that it should not be serialized. When a Person object is serialized and deserialized, the value of the age field will not be preserved.
Best Practices: Using Externalizable Interface
In addition to the Serializable interface, Java provides the Externalizable interface, which allows for more control over the serialization process. The Externalizable interface includes two methods: writeExternal() and readExternal(), which must be implemented to define the serialization and deserialization logic.
Here’s an example of a class that implements the Externalizable interface:
import java.io.*; public class Person implements Externalizable { private String name; private int age; // Constructor and other methods @Override public void writeExternal(ObjectOutput out) throws IOException { // Custom serialization logic out.writeObject(name); out.writeInt(age); } @Override public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException { // Custom deserialization logic name = (String) in.readObject(); age = in.readInt(); } }
In this example, the writeExternal() method is implemented to write the name and age fields to the ObjectOutput, and the readExternal() method is implemented to read the name and age fields from the ObjectInput.
Related Article: How To Convert Java Objects To JSON With Jackson
Real World Examples: Serialization in Distributed Systems
Serialization plays a crucial role in distributed systems, where objects need to be transmitted over the network or stored in databases. In distributed systems, objects are often serialized into a format such as JSON or XML before being transmitted, and then deserialized at the receiving end.
Here’s an example of serializing and deserializing objects in a distributed system:
import com.fasterxml.jackson.databind.ObjectMapper; import java.io.*; import java.net.HttpURLConnection; import java.net.URL; class Person { private String name; private int age; // Constructor and other methods public String toJson() throws IOException { ObjectMapper objectMapper = new ObjectMapper(); return objectMapper.writeValueAsString(this); } public static Person fromJson(String json) throws IOException { ObjectMapper objectMapper = new ObjectMapper(); return objectMapper.readValue(json, Person.class); } } public class Client { public static void main(String[] args) { Person person = new Person("John Doe", 30); // Serialization to JSON try { String json = person.toJson(); System.out.println("Person object serialized to JSON: " + json); // Send JSON over the network URL url = new URL("http://example.com/api"); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); connection.setRequestMethod("POST"); connection.setDoOutput(true); OutputStreamWriter writer = new OutputStreamWriter(connection.getOutputStream()); writer.write(json); writer.flush(); // Receive JSON from the network BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream())); String responseJson = reader.readLine(); System.out.println("Received JSON from the network: " + responseJson); // Deserialization from JSON Person responsePerson = Person.fromJson(responseJson); System.out.println("Deserialized Person object: " + responsePerson); } catch (IOException e) { e.printStackTrace(); } } }
In this example, the Person class is serialized to JSON using the Jackson library. The JSON representation of the object is then sent over the network using an HTTP POST request. At the receiving end, the JSON is deserialized back into a Person object.
Real World Examples: Serialization for Caching
Serialization is commonly used in caching systems to store and retrieve objects efficiently. By serializing objects to a binary format, caching systems can avoid the overhead of object creation and database queries.
Here’s an example of using serialization for caching:
import java.io.*; import java.util.HashMap; import java.util.Map; class CacheManager { private static final String CACHE_FILE = "cache.ser"; private Map<String, Object> cache; public CacheManager() { cache = loadCache(); } public void put(String key, Object value) { cache.put(key, value); saveCache(); } public Object get(String key) { return cache.get(key); } private void saveCache() { try (OutputStream fileOutputStream = new FileOutputStream(CACHE_FILE); ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream)) { objectOutputStream.writeObject(cache); System.out.println("Cache saved to file: " + CACHE_FILE); } catch (IOException e) { e.printStackTrace(); } } @SuppressWarnings("unchecked") private Map<String, Object> loadCache() { try (InputStream fileInputStream = new FileInputStream(CACHE_FILE); ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream)) { return (Map<String, Object>) objectInputStream.readObject(); } catch (IOException | ClassNotFoundException e) { return new HashMap<>(); } } } public class CacheExample { public static void main(String[] args) { CacheManager cacheManager = new CacheManager(); cacheManager.put("key1", "value1"); cacheManager.put("key2", "value2"); String value1 = (String) cacheManager.get("key1"); String value2 = (String) cacheManager.get("key2"); System.out.println("Value1: " + value1); System.out.println("Value2: " + value2); } }
In this example, the CacheManager class uses serialization to store and retrieve objects in a cache. The cache is saved to a file named “cache.ser” using serialization, allowing the cache to be restored across different runs of the application.
Performance Considerations: Impact on Memory
Serialization can have an impact on memory usage, especially when dealing with large objects or a large number of objects. When an object is serialized, a byte array representing the object is created in memory. This byte array can consume a significant amount of memory, especially for complex objects.
To mitigate the impact on memory, consider the following best practices:
– Avoid serializing unnecessary data. Only serialize the data that needs to be persisted or transmitted.
– Compress the serialized data if possible. Compression algorithms such as GZIP can reduce the size of the serialized data, resulting in lower memory usage.
– Consider using more memory-efficient serialization formats such as Protocol Buffers or Apache Avro.
Related Article: Storing Contact Information in Java Data Structures
Performance Considerations: Impact on Disk Space
When serializing objects to disk, the size of the serialized data can impact disk space usage. Large objects or a large number of objects can quickly consume a significant amount of disk space.
To optimize disk space usage when serializing objects, consider the following strategies:
– Compress the serialized data before storing it on disk. Compression algorithms such as GZIP or ZIP can significantly reduce the size of the serialized data.
– Use more compact serialization formats such as Protocol Buffers or Apache Avro.
– Store serialized data in a database or distributed file system that supports compression and deduplication.
Performance Considerations: Impact on Network
When transmitting serialized objects over a network, the size of the serialized data can impact network performance. Large objects or a high volume of objects can increase network latency and bandwidth usage.
To optimize network performance when transmitting serialized objects, consider the following strategies:
– Compress the serialized data before transmitting it over the network. Compression algorithms such as GZIP or LZ4 can significantly reduce the size of the serialized data, reducing network latency and bandwidth usage.
– Use more compact serialization formats such as Protocol Buffers or Apache Avro.
– Optimize the network protocol by reducing the number of round trips and minimizing the amount of data transmitted.
Advanced Techniques: Custom Serialization Formats
While Java’s built-in serialization mechanism is convenient, it may not always be the most efficient or flexible option. In some cases, it may be necessary to implement a custom serialization format.
Implementing a custom serialization format allows for more control over the serialized data structure, size, and compatibility between different versions of the serialized objects. However, it also requires more effort and may introduce additional complexity.
When designing a custom serialization format, consider the following:
– Define a compact binary representation that minimizes the size of the serialized data.
– Handle changes in the serialized object’s structure and versioning to ensure backward and forward compatibility.
– Implement custom serialization and deserialization logic to convert the object to and from the custom format.
Related Article: How to Convert JSON String to Java Object
Advanced Techniques: Versioning of Serialized Objects
Versioning of serialized objects is an important aspect of serialization in long-lived applications. As the structure of serialized objects evolves over time, it is crucial to maintain compatibility between different versions.
To version serialized objects, consider the following strategies:
– Assign a unique version identifier to each version of the serialized object.
– Implement custom serialization and deserialization logic to handle different versions of the object.
– Handle changes in the object’s structure, such as adding or removing fields, in a backward and forward compatible manner.
– Use the serialVersionUID field to control compatibility between different versions of the serialized object.
Code Snippet: Basic Serialization
Here’s an example of basic serialization in Java:
import java.io.*; public class SerializationExample { public static void main(String[] args) { Person person = new Person("John Doe", 30); // Serialization try (OutputStream fileOutputStream = new FileOutputStream("person.ser"); ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream)) { objectOutputStream.writeObject(person); System.out.println("Person object serialized successfully."); } catch (IOException e) { e.printStackTrace(); } // Deserialization try (InputStream fileInputStream = new FileInputStream("person.ser"); ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream)) { Person deserializedPerson = (Person) objectInputStream.readObject(); System.out.println("Deserialized Person object: " + deserializedPerson); } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } } }
In this example, a Person object is serialized and saved to a file named “person.ser”. Later, the object is deserialized from the file and printed to the console.
Code Snippet: Deserialization
Here’s an example of deserialization in Java:
import java.io.*; public class DeserializationExample { public static void main(String[] args) { try (InputStream fileInputStream = new FileInputStream("person.ser"); ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream)) { Person deserializedPerson = (Person) objectInputStream.readObject(); System.out.println("Deserialized Person object: " + deserializedPerson); } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } } }
In this example, a Person object is deserialized from a file named “person.ser” and printed to the console.
Related Article: How to Retrieve Current Date and Time in Java
Code Snippet: Handling Serialization Errors
Here’s an example of handling serialization errors in Java:
import java.io.*; public class SerializationExample { public static void main(String[] args) { Person person = new Person("John Doe", 30); // Serialization try (OutputStream fileOutputStream = new FileOutputStream("person.ser"); ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream)) { objectOutputStream.writeObject(person); System.out.println("Person object serialized successfully."); } catch (IOException e) { e.printStackTrace(); } // Deserialization try (InputStream fileInputStream = new FileInputStream("person.ser"); ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream)) { Person deserializedPerson = (Person) objectInputStream.readObject(); System.out.println("Deserialized Person object: " + deserializedPerson); } catch (InvalidClassException e) { System.out.println("InvalidClassException: Class version mismatch."); } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } } }
In this example, if a version mismatch occurs during deserialization, an InvalidClassException is caught and handled. Other exceptions, such as IOException and ClassNotFoundException, are also caught and printed to the console.
Code Snippet: Customizing Serialization
Here’s an example of customizing serialization in Java:
import java.io.*; public class Person implements Serializable { private String name; private int age; // Constructor and other methods private void writeObject(ObjectOutputStream out) throws IOException { // Custom serialization logic out.writeObject(name); out.writeInt(age); } private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException { // Custom deserialization logic name = (String) in.readObject(); age = in.readInt(); } }
In this example, the writeObject() and readObject() methods are implemented to define custom serialization and deserialization logic for the Person class. The name and age fields are serialized and deserialized using these custom methods.
Code Snippet: Using Externalizable
Here’s an example of using the Externalizable interface in Java:
import java.io.*; public class Person implements Externalizable { private String name; private int age; // Constructor and other methods @Override public void writeExternal(ObjectOutput out) throws IOException { // Custom serialization logic out.writeObject(name); out.writeInt(age); } @Override public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException { // Custom deserialization logic name = (String) in.readObject(); age = in.readInt(); } }
In this example, the Person class implements the Externalizable interface and overrides the writeExternal() and readExternal() methods to define custom serialization and deserialization logic. The name and age fields are serialized and deserialized using these custom methods.
Related Article: How to Reverse a String in Java
Error Handling: Dealing with Serialization Errors
When working with serialization, it’s important to handle errors that may occur during the serialization or deserialization process. Common errors include version mismatches, invalid class definitions, or incompatible serialization formats.
To deal with serialization errors, consider the following strategies:
– Catch and handle specific exceptions that may occur during serialization or deserialization, such as InvalidClassException or IOException.
– Provide meaningful error messages or logging to help diagnose and fix serialization errors.
– Test the serialization and deserialization process with different scenarios and edge cases to identify and fix potential issues.
Error Handling: Managing Changes in Serializable Classes
When modifying a class that is already serialized, it’s important to carefully manage the changes to ensure compatibility between different versions of the class. Incompatible changes can lead to serialization errors or data corruption.
To manage changes in serializable classes, consider the following strategies:
– Use the serialVersionUID field to control compatibility between different versions of the class. Increment the serialVersionUID when making incompatible changes.
– Handle version mismatches gracefully by providing backward and forward compatibility logic in custom serialization or deserialization methods.
– Avoid removing or renaming serialized fields to prevent data corruption when deserializing objects of older versions.
– Use the transient keyword to exclude fields that should not be serialized from the object’s state.
– Test the serialization and deserialization process after making changes to ensure compatibility with existing serialized objects.