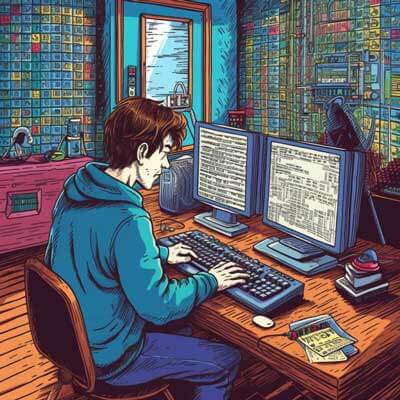
- Setting Up Java JDBC
- Example 1: Setting Up Java JDBC
- Establishing Java Database Connectivity
- Example 1: Establishing Java Database Connectivity
- Integrating MySQL with Java
- Example 1: Integrating MySQL with Java
- Using Java MySQL Driver
- Example 1: Using Java MySQL Driver
- Creating MySQL Connection in Java
- Example 1: Creating MySQL Connection in Java
- Performing CRUD Operations in Java MySQL
- Example 1: Performing CRUD Operations in Java MySQL
- Implementing Prepared Statements in Java MySQL
- Example 1: Implementing Prepared Statements in Java MySQL
- Configuring Java MySQL Connection Pool
- Example 1: Configuring Java MySQL Connection Pool
- Calling Stored Procedures in MySQL from Java
- Example 1: Calling Stored Procedures in MySQL from Java
- Connecting Java with MySQL Database
- Example 1: Connecting Java with MySQL Database
- Exploring MySQL Connector for Java
- Database Operations in Java using MySQL
- Example 1: Inserting Data into MySQL Database using Java
- Example 2: Retrieving Data from MySQL Database using Java
- Understanding Prepared Statements in Java MySQL
- Additional Resources
Setting Up Java JDBC
To connect Java with MySQL, we need to set up the Java Database Connectivity (JDBC) driver. JDBC is a Java API that allows Java programs to interact with databases. Here are the steps to set up JDBC:
1. Download the JDBC driver:
– Go to the MySQL website (https://dev.mysql.com/downloads/connector/j/) and download the MySQL Connector/J driver. Choose the appropriate version based on your MySQL server version and Java version.
2. Add the JDBC driver to your Java project:
– Extract the downloaded JDBC driver file.
– In your Java project, create a new folder called “lib” (if it doesn’t exist already) and copy the extracted JDBC driver file to this folder.
3. Configure your Java project to use the JDBC driver:
– In your Java IDE, right-click on your project and select “Properties” or “Build Path” (depending on your IDE).
– Navigate to the “Libraries” or “Dependencies” tab and click on “Add JARs” or “Add External JARs”.
– Select the JDBC driver JAR file from the “lib” folder and click “OK” to add it to your project’s classpath.
Related Article: Spring Boot Integration with Payment, Communication Tools
Example 1: Setting Up Java JDBC
Here’s an example of how to set up Java JDBC using Eclipse:
1. Download the JDBC driver:
– Go to the MySQL website (https://dev.mysql.com/downloads/connector/j/) and download the MySQL Connector/J driver. Choose the appropriate version based on your MySQL server version and Java version. Let’s assume the downloaded file is named “mysql-connector-java-8.0.26.jar”.
2. Add the JDBC driver to your Java project:
– Extract the downloaded JDBC driver file.
– In your Java project, create a new folder called “lib” if it doesn’t exist already. Copy the extracted “mysql-connector-java-8.0.26.jar” file to this folder.
3. Configure your Java project to use the JDBC driver in Eclipse:
– Right-click on your project in the “Package Explorer” and select “Properties”.
– In the Properties dialog, select “Java Build Path” from the left sidebar.
– Click on the “Libraries” tab.
– Click on the “Add JARs” button on the right side.
– Navigate to the “lib” folder within your project and select the “mysql-connector-java-8.0.26.jar” file.
– Click “OK” to add the JDBC driver to your project’s classpath.
With the JDBC driver set up, we can now proceed to establish the Java Database Connectivity.
Establishing Java Database Connectivity
To establish Java Database Connectivity (JDBC) with MySQL, we need to create a connection between our Java application and the MySQL database server. This connection allows us to execute SQL queries, retrieve results, and perform other database operations. Here are the steps to establish JDBC:
1. Import the necessary JDBC packages:
– In your Java class, import the necessary JDBC packages using the import
statement. The most commonly used packages are java.sql.*
and javax.sql.*
.
2. Load the JDBC driver:
– Before establishing a connection, we need to load the JDBC driver class using the Class.forName()
method. The driver class name depends on the specific JDBC driver you are using. For MySQL Connector/J, the driver class is com.mysql.cj.jdbc.Driver
.
3. Create a connection:
– Use the DriverManager.getConnection()
method to create a connection to the MySQL database. Pass the database URL, username, and password as parameters to this method. The database URL has the following format: jdbc:mysql://hostname:port/database_name
. Replace hostname
with the hostname or IP address of the MySQL server, port
with the port number (default is 3306), and database_name
with the name of the MySQL database.
4. Close the connection:
– After you are done with the database operations, make sure to close the connection using the connection.close()
method. This releases the database resources and ensures a clean shutdown.
Example 1: Establishing Java Database Connectivity
Here’s an example of how to establish JDBC with MySQL in Java:
import java.sql.*; public class JdbcExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try { // Load the JDBC driver Class.forName("com.mysql.cj.jdbc.Driver"); // Create a connection Connection connection = DriverManager.getConnection(url, username, password); // Perform database operations // Close the connection connection.close(); } catch (ClassNotFoundException | SQLException e) { e.printStackTrace(); } } }
In this example, we establish a JDBC connection to a MySQL database running on the local machine. Replace localhost
with the hostname or IP address of the MySQL server, 3306
with the port number, mydatabase
with the name of the database, root
with the MySQL username, and password
with the corresponding password.
Now that we have established the JDBC connection, let’s move on to integrating MySQL with Java.
Related Article: Java Spring Security Customizations & RESTful API Protection
Integrating MySQL with Java
Integrating MySQL with Java involves using the MySQL Connector/J driver to connect to a MySQL database, execute SQL queries, and perform database operations. Here are the steps to integrate MySQL with Java:
1. Import the necessary JDBC packages:
– In your Java class, import the necessary JDBC packages using the import
statement. The most commonly used packages are java.sql.*
and javax.sql.*
.
2. Load the JDBC driver:
– Before establishing a connection, we need to load the JDBC driver class using the Class.forName()
method. The driver class for MySQL Connector/J is com.mysql.cj.jdbc.Driver
.
3. Create a connection:
– Use the DriverManager.getConnection()
method to create a connection to the MySQL database. Pass the database URL, username, and password as parameters to this method. The database URL has the following format: jdbc:mysql://hostname:port/database_name
.
4. Execute SQL queries:
– Use the Connection.createStatement()
method to create a Statement
object. This object allows you to execute SQL queries and retrieve the results.
5. Process the results:
– Use the Statement.executeQuery()
method to execute a SELECT query and retrieve the results as a ResultSet
. Iterate over the ResultSet
to process each row of the result set.
6. Close the connection:
– After you are done with the database operations, make sure to close the connection using the connection.close()
method.
Example 1: Integrating MySQL with Java
Here’s an example of how to integrate MySQL with Java using JDBC:
import java.sql.*; public class JdbcExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try { // Load the JDBC driver Class.forName("com.mysql.cj.jdbc.Driver"); // Create a connection Connection connection = DriverManager.getConnection(url, username, password); // Create a statement Statement statement = connection.createStatement(); // Execute a query ResultSet resultSet = statement.executeQuery("SELECT * FROM users"); // Process the results while (resultSet.next()) { int id = resultSet.getInt("id"); String name = resultSet.getString("name"); String email = resultSet.getString("email"); System.out.println("ID: " + id + ", Name: " + name + ", Email: " + email); } // Close the connection connection.close(); } catch (ClassNotFoundException | SQLException e) { e.printStackTrace(); } } }
In this example, we integrate MySQL with Java by executing a SELECT query to retrieve all rows from the “users” table. Replace localhost
with the hostname or IP address of the MySQL server, 3306
with the port number, mydatabase
with the name of the database, root
with the MySQL username, and password
with the corresponding password.
With the integration of MySQL and Java established, we can now move on to using the Java MySQL driver for database operations.
Using Java MySQL Driver
The Java MySQL driver, also known as the MySQL Connector/J, is a JDBC driver that allows Java programs to interact with MySQL databases. It provides the necessary classes and methods to establish connections, execute SQL queries, and perform database operations. Here are the steps to use the Java MySQL driver:
1. Import the necessary JDBC packages:
– In your Java class, import the necessary JDBC packages using the import
statement. The most commonly used packages are java.sql.*
and javax.sql.*
.
2. Load the JDBC driver:
– Before establishing a connection, we need to load the JDBC driver class using the Class.forName()
method. The driver class for MySQL Connector/J is com.mysql.cj.jdbc.Driver
.
3. Create a connection:
– Use the DriverManager.getConnection()
method to create a connection to the MySQL database. Pass the database URL, username, and password as parameters to this method. The database URL has the following format: jdbc:mysql://hostname:port/database_name
.
4. Execute SQL queries:
– Use the Connection.createStatement()
method to create a Statement
object. This object allows you to execute SQL queries and retrieve the results.
5. Process the results:
– Use the Statement.executeQuery()
method to execute a SELECT query and retrieve the results as a ResultSet
. Iterate over the ResultSet
to process each row of the result set.
6. Close the connection:
– After you are done with the database operations, make sure to close the connection using the connection.close()
method.
Related Article: Tutorial on Integrating Redis with Spring Boot
Example 1: Using Java MySQL Driver
Here’s an example of how to use the Java MySQL driver in Java:
import java.sql.*; public class JdbcExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try { // Load the JDBC driver Class.forName("com.mysql.cj.jdbc.Driver"); // Create a connection Connection connection = DriverManager.getConnection(url, username, password); // Create a statement Statement statement = connection.createStatement(); // Execute a query ResultSet resultSet = statement.executeQuery("SELECT * FROM users"); // Process the results while (resultSet.next()) { int id = resultSet.getInt("id"); String name = resultSet.getString("name"); String email = resultSet.getString("email"); System.out.println("ID: " + id + ", Name: " + name + ", Email: " + email); } // Close the connection connection.close(); } catch (ClassNotFoundException | SQLException e) { e.printStackTrace(); } } }
In this example, we use the Java MySQL driver to execute a SELECT query and retrieve all rows from the “users” table. Replace localhost
with the hostname or IP address of the MySQL server, 3306
with the port number, mydatabase
with the name of the database, root
with the MySQL username, and password
with the corresponding password.
Now that we know how to use the Java MySQL driver, let’s move on to creating a MySQL connection in Java.
Creating MySQL Connection in Java
Creating a MySQL connection in Java involves using the MySQL Connector/J driver to establish a connection between your Java application and the MySQL database server. This connection allows you to execute SQL queries, retrieve results, and perform other database operations. Here are the steps to create a MySQL connection in Java:
1. Import the necessary JDBC packages:
– In your Java class, import the necessary JDBC packages using the import
statement. The most commonly used packages are java.sql.*
and javax.sql.*
.
2. Load the JDBC driver:
– Before establishing a connection, we need to load the JDBC driver class using the Class.forName()
method. The driver class for MySQL Connector/J is com.mysql.cj.jdbc.Driver
.
3. Create a connection:
– Use the DriverManager.getConnection()
method to create a connection to the MySQL database. Pass the database URL, username, and password as parameters to this method. The database URL has the following format: jdbc:mysql://hostname:port/database_name
.
4. Close the connection:
– After you are done with the database operations, make sure to close the connection using the connection.close()
method.
Example 1: Creating MySQL Connection in Java
Here’s an example of how to create a MySQL connection in Java:
import java.sql.*; public class JdbcExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try { // Load the JDBC driver Class.forName("com.mysql.cj.jdbc.Driver"); // Create a connection Connection connection = DriverManager.getConnection(url, username, password); // Close the connection connection.close(); } catch (ClassNotFoundException | SQLException e) { e.printStackTrace(); } } }
In this example, we create a MySQL connection in Java using the MySQL Connector/J driver. Replace localhost
with the hostname or IP address of the MySQL server, 3306
with the port number, mydatabase
with the name of the database, root
with the MySQL username, and password
with the corresponding password.
Now that we know how to create a MySQL connection in Java, let’s move on to performing CRUD operations in Java MySQL.
Related Article: Identifying the Version of Your MySQL-Connector-Java
Performing CRUD Operations in Java MySQL
CRUD (Create, Read, Update, Delete) operations are the basic database operations that allow you to create, retrieve, update, and delete data in a database. In Java MySQL, we can perform these operations using SQL queries and the Java MySQL driver. Here are the steps to perform CRUD operations in Java MySQL:
1. Import the necessary JDBC packages:
– In your Java class, import the necessary JDBC packages using the import
statement. The most commonly used packages are java.sql.*
and javax.sql.*
.
2. Load the JDBC driver:
– Before establishing a connection, we need to load the JDBC driver class using the Class.forName()
method. The driver class for MySQL Connector/J is com.mysql.cj.jdbc.Driver
.
3. Create a connection:
– Use the DriverManager.getConnection()
method to create a connection to the MySQL database. Pass the database URL, username, and password as parameters to this method. The database URL has the following format: jdbc:mysql://hostname:port/database_name
.
4. Perform CRUD operations:
– Use the appropriate SQL queries and the methods provided by the Java MySQL driver to perform CRUD operations. For example, use Statement.executeUpdate()
to execute an INSERT, UPDATE, or DELETE query, and use Statement.executeQuery()
to execute a SELECT query.
5. Close the connection:
– After you are done with the database operations, make sure to close the connection using the connection.close()
method.
Example 1: Performing CRUD Operations in Java MySQL
Here’s an example of how to perform CRUD operations in Java MySQL:
import java.sql.*; public class JdbcExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try { // Load the JDBC driver Class.forName("com.mysql.cj.jdbc.Driver"); // Create a connection Connection connection = DriverManager.getConnection(url, username, password); // Create a statement Statement statement = connection.createStatement(); // Insert data String insertQuery = "INSERT INTO users (name, email) VALUES ('John Doe', 'john@example.com')"; int rowsInserted = statement.executeUpdate(insertQuery); System.out.println(rowsInserted + " row(s) inserted."); // Update data String updateQuery = "UPDATE users SET email = 'johndoe@example.com' WHERE id = 1"; int rowsUpdated = statement.executeUpdate(updateQuery); System.out.println(rowsUpdated + " row(s) updated."); // Delete data String deleteQuery = "DELETE FROM users WHERE id = 1"; int rowsDeleted = statement.executeUpdate(deleteQuery); System.out.println(rowsDeleted + " row(s) deleted."); // Close the connection connection.close(); } catch (ClassNotFoundException | SQLException e) { e.printStackTrace(); } } }
In this example, we perform CRUD operations in Java MySQL using SQL queries and the Java MySQL driver. We insert a new user, update the email of an existing user, and delete a user from the “users” table. Replace localhost
with the hostname or IP address of the MySQL server, 3306
with the port number, mydatabase
with the name of the database, root
with the MySQL username, and password
with the corresponding password.
Now that we know how to perform CRUD operations in Java MySQL, let’s move on to implementing prepared statements.
Implementing Prepared Statements in Java MySQL
Prepared statements provide a way to execute parameterized SQL queries in Java MySQL. They allow you to write SQL queries with placeholders for parameters and then set the values for those parameters at runtime. This helps prevent SQL injection attacks and improves performance by reusing query execution plans. Here are the steps to implement prepared statements in Java MySQL:
1. Import the necessary JDBC packages:
– In your Java class, import the necessary JDBC packages using the import
statement. The most commonly used packages are java.sql.*
and javax.sql.*
.
2. Load the JDBC driver:
– Before establishing a connection, we need to load the JDBC driver class using the Class.forName()
method. The driver class for MySQL Connector/J is com.mysql.cj.jdbc.Driver
.
3. Create a connection:
– Use the DriverManager.getConnection()
method to create a connection to the MySQL database. Pass the database URL, username, and password as parameters to this method. The database URL has the following format: jdbc:mysql://hostname:port/database_name
.
4. Prepare the SQL statement:
– Use the Connection.prepareStatement()
method to create a prepared statement. Pass the SQL query as a parameter to this method. Use question marks (?
) as placeholders for parameters in the query.
5. Set parameter values:
– Use the PreparedStatement.setXXX()
methods to set the values for the parameters in the prepared statement. Replace XXX
with the appropriate data type of the parameter (e.g., Int
, String
, Date
, etc.). Use the index of the parameter to specify its position in the query.
6. Execute the prepared statement:
– Use the PreparedStatement.executeUpdate()
or PreparedStatement.executeQuery()
method to execute the prepared statement. These methods work similarly to their counterparts in regular statements.
7. Process the results:
– If the prepared statement is a SELECT query, use the ResultSet
object to retrieve and process the results.
8. Close the connection:
– After you are done with the database operations, make sure to close the connection using the connection.close()
method.
Related Article: Proper Placement of MySQL Connector JAR File in Java
Example 1: Implementing Prepared Statements in Java MySQL
Here’s an example of how to implement prepared statements in Java MySQL:
import java.sql.*; public class JdbcExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try { // Load the JDBC driver Class.forName("com.mysql.cj.jdbc.Driver"); // Create a connection Connection connection = DriverManager.getConnection(url, username, password); // Prepare the SQL statement String insertQuery = "INSERT INTO users (name, email) VALUES (?, ?)"; PreparedStatement preparedStatement = connection.prepareStatement(insertQuery); // Set parameter values preparedStatement.setString(1, "John Doe"); preparedStatement.setString(2, "john@example.com"); // Execute the prepared statement int rowsInserted = preparedStatement.executeUpdate(); System.out.println(rowsInserted + " row(s) inserted."); // Close the connection connection.close(); } catch (ClassNotFoundException | SQLException e) { e.printStackTrace(); } } }
In this example, we implement a prepared statement in Java MySQL to insert a new user into the “users” table. We use question marks (?
) as placeholders for the parameters in the SQL query and set their values using the setXXX()
methods of the PreparedStatement
object. Replace localhost
with the hostname or IP address of the MySQL server, 3306
with the port number, mydatabase
with the name of the database, root
with the MySQL username, and password
with the corresponding password.
Now that we know how to implement prepared statements in Java MySQL, let’s move on to configuring a Java MySQL connection pool.
Configuring Java MySQL Connection Pool
A connection pool is a cache of database connections maintained by the application server. It allows multiple clients to share a limited number of database connections, thus improving performance and scalability. To configure a Java MySQL connection pool, we need to use a connection pool implementation, such as Apache DBCP or HikariCP. Here are the steps to configure a Java MySQL connection pool using Apache DBCP:
1. Import the necessary JDBC and connection pool packages:
– In your Java class, import the necessary JDBC and connection pool packages using the import
statement. The most commonly used packages are java.sql.*
, javax.sql.*
, and the packages specific to your chosen connection pool implementation.
2. Load the JDBC driver:
– Before establishing a connection pool, we need to load the JDBC driver class using the Class.forName()
method. The driver class for MySQL Connector/J is com.mysql.cj.jdbc.Driver
.
3. Configure the connection pool properties:
– Use the properties specific to your chosen connection pool implementation to configure the connection pool. For example, in Apache DBCP, you can set properties such as the maximum number of connections, the connection timeout, the validation query, etc.
4. Create the connection pool:
– Use the classes and methods provided by your chosen connection pool implementation to create the connection pool. For example, in Apache DBCP, you can create a BasicDataSource
object and set its properties.
5. Get a connection from the connection pool:
– Use the DataSource.getConnection()
method to get a connection from the connection pool. This method returns a Connection
object that you can use to execute SQL queries and perform database operations.
6. Use the connection:
– Use the obtained connection to execute SQL queries, retrieve results, and perform other database operations.
7. Return the connection to the connection pool:
– After you are done with the database operations, make sure to close the connection using the connection.close()
method. This returns the connection to the connection pool for reuse by other clients.
Example 1: Configuring Java MySQL Connection Pool
Here’s an example of how to configure a Java MySQL connection pool using Apache DBCP:
import java.sql.*; import javax.sql.*; import org.apache.commons.dbcp2.BasicDataSource; public class JdbcExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try { // Load the JDBC driver Class.forName("com.mysql.cj.jdbc.Driver"); // Configure the connection pool properties BasicDataSource dataSource = new BasicDataSource(); dataSource.setUrl(url); dataSource.setUsername(username); dataSource.setPassword(password); dataSource.setMaxTotal(10); dataSource.setMinIdle(5); dataSource.setMaxIdle(10); // Get a connection from the connection pool Connection connection = dataSource.getConnection(); // Use the connection // Return the connection to the connection pool connection.close(); } catch (ClassNotFoundException | SQLException e) { e.printStackTrace(); } } }
In this example, we configure a Java MySQL connection pool using Apache DBCP. We set the MySQL database URL, username, and password, as well as other connection pool properties such as the maximum number of connections (setMaxTotal()
), the minimum number of idle connections (setMinIdle()
), and the maximum number of idle connections (setMaxIdle()
). Replace localhost
with the hostname or IP address of the MySQL server, 3306
with the port number, mydatabase
with the name of the database, root
with the MySQL username, and password
with the corresponding password.
Now that we know how to configure a Java MySQL connection pool, let’s move on to calling stored procedures in MySQL from Java.
Related Article: How to Read a JSON File in Java Using the Simple JSON Lib
Calling Stored Procedures in MySQL from Java
Stored procedures are precompiled database objects that contain one or more SQL statements. They allow you to encapsulate complex database operations and improve performance by reducing network traffic. To call stored procedures in MySQL from Java, we can use the Java MySQL driver and the CallableStatement
class. Here are the steps to call stored procedures in MySQL from Java:
1. Import the necessary JDBC packages:
– In your Java class, import the necessary JDBC packages using the import
statement. The most commonly used packages are java.sql.*
and javax.sql.*
.
2. Load the JDBC driver:
– Before calling a stored procedure, we need to load the JDBC driver class using the Class.forName()
method. The driver class for MySQL Connector/J is com.mysql.cj.jdbc.Driver
.
3. Create a connection:
– Use the DriverManager.getConnection()
method to create a connection to the MySQL database. Pass the database URL, username, and password as parameters to this method. The database URL has the following format: jdbc:mysql://hostname:port/database_name
.
4. Prepare the CallableStatement
:
– Use the Connection.prepareCall()
method to create a CallableStatement
object. Pass the SQL query that calls the stored procedure as a parameter to this method. Use question marks (?
) as placeholders for input and output parameters.
5. Set input parameters:
– Use the CallableStatement.setXXX()
methods to set the values for the input parameters of the stored procedure. Replace XXX
with the appropriate data type of the parameter (e.g., Int
, String
, Date
, etc.). Use the index of the parameter to specify its position in the stored procedure call.
6. Register output parameters:
– Use the CallableStatement.registerOutParameter()
method to register the output parameters of the stored procedure. Pass the index of the parameter and the SQL data type of the parameter as parameters to this method.
7. Execute the stored procedure:
– Use the CallableStatement.execute()
method to execute the stored procedure. This method returns a boolean value indicating whether the stored procedure executed successfully.
8. Retrieve output parameters:
– Use the CallableStatement.getXXX()
methods to retrieve the values of the output parameters. Replace XXX
with the appropriate data type of the parameter. Use the index of the parameter to specify its position in the stored procedure call.
9. Close the connection:
– After you are done with the database operations, make sure to close the connection using the connection.close()
method.
Example 1: Calling Stored Procedures in MySQL from Java
Here’s an example of how to call a stored procedure in MySQL from Java:
import java.sql.*; public class JdbcExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try { // Load the JDBC driver Class.forName("com.mysql.cj.jdbc.Driver"); // Create a connection Connection connection = DriverManager.getConnection(url, username, password); // Prepare the CallableStatement String callProcedure = "{CALL get_user_info(?, ?, ?)}"; CallableStatement callableStatement = connection.prepareCall(callProcedure); // Set input parameters callableStatement.setInt(1, 1); // Register output parameters callableStatement.registerOutParameter(2, Types.VARCHAR); callableStatement.registerOutParameter(3, Types.VARCHAR); // Execute the stored procedure boolean executed = callableStatement.execute(); // Retrieve output parameters if (executed) { String firstName = callableStatement.getString(2); String lastName = callableStatement.getString(3); System.out.println("First Name: " + firstName); System.out.println("Last Name: " + lastName); } // Close the connection connection.close(); } catch (ClassNotFoundException | SQLException e) { e.printStackTrace(); } } }
In this example, we call a stored procedure named “get_user_info” in MySQL from Java. The stored procedure takes an input parameter id
and returns the first name and last name of the user with that ID. We use the CallableStatement
object to set the input parameter, register the output parameters, execute the stored procedure, and retrieve the output parameters. Replace localhost
with the hostname or IP address of the MySQL server, 3306
with the port number, mydatabase
with the name of the database, root
with the MySQL username, and password
with the corresponding password.
Now that we know how to call stored procedures in MySQL from Java, let’s move on to connecting Java with a MySQL database.
Connecting Java with MySQL Database
To connect Java with a MySQL database, we need to use the Java MySQL driver (MySQL Connector/J). The driver allows Java programs to interact with MySQL databases by providing the necessary classes and methods for establishing connections, executing SQL queries, and performing database operations. Here are the steps to connect Java with a MySQL database:
1. Import the necessary JDBC packages:
– In your Java class, import the necessary JDBC packages using the import
statement. The most commonly used packages are java.sql.*
and javax.sql.*
.
2. Load the JDBC driver:
– Before establishing a connection, we need to load the JDBC driver class using the Class.forName()
method. The driver class for MySQL Connector/J is com.mysql.cj.jdbc.Driver
.
3. Create a connection:
– Use the DriverManager.getConnection()
method to create a connection to the MySQL database. Pass the database URL, username, and password as parameters to this method. The database URL has the following format: jdbc:mysql://hostname:port/database_name
.
4. Execute SQL queries:
– Use the Connection.createStatement()
method to create a Statement
object. This object allows you to execute SQL queries and retrieve the results.
5. Process the results:
– If the query is a SELECT query, use the Statement.executeQuery()
method to execute the query and retrieve the results as a ResultSet
. Iterate over the ResultSet
to process each row of the result set.
6. Close the connection:
– After you are done with the database operations, make sure to close the connection using the connection.close()
method.
Related Article: How to Use Spring Configuration Annotation
Example 1: Connecting Java with MySQL Database
Here’s an example of how to connect Java with a MySQL database:
import java.sql.*; public class JdbcExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try { // Load the JDBC driver Class.forName("com.mysql.cj.jdbc.Driver"); // Create a connection Connection connection = DriverManager.getConnection(url, username, password); // Create a statement Statement statement = connection.createStatement(); // Execute a query ResultSet resultSet = statement.executeQuery("SELECT * FROM users"); // Process the results while (resultSet.next()) { int id = resultSet.getInt("id"); String name = resultSet.getString("name"); String email = resultSet.getString("email"); System.out.println("ID: " + id + ", Name: " + name + ", Email: " + email); } // Close the connection connection.close(); } catch (ClassNotFoundException | SQLException e) { e.printStackTrace(); } } }
In this example, we connect Java with a MySQL database by establishing a JDBC connection using the MySQL Connector/J driver. We execute a SELECT query to retrieve all rows from the “users” table and process the results. Replace localhost
with the hostname or IP address of the MySQL server, 3306
with the port number, mydatabase
with the name of the database, root
with the MySQL username, and password
with the corresponding password.
Now that we know how to connect Java with a MySQL database, let’s move on to exploring the MySQL Connector for Java.
Exploring MySQL Connector for Java
The MySQL Connector for Java, also known as MySQL Connector/J, is a JDBC driver that allows Java applications to connect to MySQL databases. It provides the necessary classes and methods for establishing connections, executing SQL queries, and performing database operations. Here are some key features and benefits of the MySQL Connector for Java:
– Compatibility: The MySQL Connector for Java is compatible with the latest versions of MySQL and Java. It supports the JDBC API and is compliant with the Java Database Connectivity standard.
– Performance: The MySQL Connector for Java is optimized for performance. It uses advanced techniques such as caching, connection pooling, and statement caching to improve the performance of database operations.
– Security: The MySQL Connector for Java provides secure connections to MySQL databases. It supports SSL/TLS encryption and authentication mechanisms to protect sensitive data.
– Scalability: The MySQL Connector for Java supports connection pooling, which allows multiple clients to share a limited number of database connections. This improves scalability by reducing the overhead of creating and closing connections.
– Flexibility: The MySQL Connector for Java provides a wide range of configuration options and features. It supports various types of authentication, connection options, and database operations.
To use the MySQL Connector for Java, you need to download and install it from the official MySQL website. The connector is available as a JAR file, which you can add to your Java project’s classpath.
Database Operations in Java using MySQL
Performing database operations in Java using MySQL involves interacting with the MySQL database using the Java MySQL driver. The Java MySQL driver provides the necessary classes and methods to establish connections, execute SQL queries, and perform database operations. Here are the common database operations you can perform in Java using MySQL:
– Inserting data: Use the INSERT INTO
statement to insert data into a MySQL table. The values to be inserted can be provided as literals or variables.
– Retrieving data: Use the SELECT
statement to retrieve data from a MySQL table. You can specify the columns to retrieve and use conditions to filter the results.
– Updating data: Use the UPDATE
statement to update data in a MySQL table. You can specify the columns to update and use conditions to filter the rows to be updated.
– Deleting data: Use the DELETE FROM
statement to delete data from a MySQL table. You can use conditions to filter the rows to be deleted.
– Executing SQL queries: Use the Statement.execute()
method to execute SQL queries that don’t return a result set, such as CREATE TABLE
, ALTER TABLE
, or DROP TABLE
statements.
– Executing stored procedures: Use the CallableStatement
class to execute stored procedures in MySQL. Prepare the statement, set input parameters, register output parameters, and execute the stored procedure.
– Using prepared statements: Use prepared statements to execute parameterized SQL queries in MySQL. Prepare the statement, set parameter values, and execute the statement. Prepared statements help prevent SQL injection attacks and improve performance.
These are the basic database operations you can perform in Java using MySQL. Depending on your application’s requirements, you can combine these operations to implement more complex functionality.
Related Article: How To Set Xms And Xmx Parameters For Jvm
Example 1: Inserting Data into MySQL Database using Java
Here’s an example of how to insert data into a MySQL database using Java:
import java.sql.*; public class JdbcExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try { // Load the JDBC driver Class.forName("com.mysql.cj.jdbc.Driver"); // Create a connection Connection connection = DriverManager.getConnection(url, username, password); // Create a statement Statement statement = connection.createStatement(); // Insert data String insertQuery = "INSERT INTO users (name, email) VALUES ('John Doe', 'john@example.com')"; int rowsInserted = statement.executeUpdate(insertQuery); System.out.println(rowsInserted + " row(s) inserted."); // Close the connection connection.close(); } catch (ClassNotFoundException | SQLException e) { e.printStackTrace(); } } }
In this example, we insert a new user into the “users” table of a MySQL database. We create a connection, create a statement, and execute an INSERT INTO
statement to insert the data. Replace localhost
with the hostname or IP address of the MySQL server, 3306
with the port number, mydatabase
with the name of the database, root
with the MySQL username, and password
with the corresponding password.
Example 2: Retrieving Data from MySQL Database using Java
Here’s an example of how to retrieve data from a MySQL database using Java:
import java.sql.*; public class JdbcExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try { // Load the JDBC driver Class.forName("com.mysql.cj.jdbc.Driver"); // Create a connection Connection connection = DriverManager.getConnection(url, username, password); // Create a statement Statement statement = connection.createStatement(); // Execute a query ResultSet resultSet = statement.executeQuery("SELECT * FROM users"); // Process the results while (resultSet.next()) { int id = resultSet.getInt("id"); String name = resultSet.getString("name"); String email = resultSet.getString("email"); System.out.println("ID: " + id + ", Name: " + name + ", Email: " + email); } // Close the connection connection.close(); } catch (ClassNotFoundException | SQLException e) { e.printStackTrace(); } } }
In this example, we retrieve data from the “users” table of a MySQL database. We create a connection, create a statement, and execute a SELECT
query to retrieve the data. We then iterate over the ResultSet
object to process each row of the result set. Replace localhost
with the hostname or IP address of the MySQL server, 3306
with the port number, mydatabase
with the name of the database, root
with the MySQL username, and password
with the corresponding password.
These examples demonstrate how to perform basic database operations in Java using MySQL. With the Java MySQL driver, you have the flexibility to implement more complex functionality and interact with the MySQL database effectively.
Now that we have explored database operations in Java using MySQL, let’s move on to understanding prepared statements in Java MySQL.
Understanding Prepared Statements in Java MySQL
Prepared statements in Java MySQL are SQL statements that are precompiled by the database server and can be reused with different parameter values. They provide a way to execute parameterized SQL queries, which helps prevent SQL injection attacks and improves performance. Here’s how prepared statements work in Java MySQL:
1. Prepare the SQL statement:
– Use the Connection.prepareStatement()
method to create a prepared statement. Pass the SQL query as a parameter to this method. Use question marks (?
) as placeholders for parameters in the query.
2. Set parameter values:
– Use the PreparedStatement.setXXX()
methods to set the values for the parameters in the prepared statement. Replace XXX
with the appropriate data type of the parameter (e.g., Int
, String
, Date
, etc.). Use the index of the parameter to specify its position in the query.
3. Execute the prepared statement:
– Use the PreparedStatement.executeUpdate()
or PreparedStatement.executeQuery()
method to execute the prepared statement. These methods work similarly to their counterparts in regular statements.
4. Process the results:
– If the prepared statement is a SELECT query, use the ResultSet
object to retrieve and process the results.
Here’s an example of how to use prepared statements in Java MySQL:
import java.sql.*; public class JdbcExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try { // Load the JDBC driver Class.forName("com.mysql.cj.jdbc.Driver"); // Create a connection Connection connection = DriverManager.getConnection(url, username, password); // Prepare the SQL statement String insertQuery = "INSERT INTO users (name, email) VALUES (?, ?)"; PreparedStatement preparedStatement = connection.prepareStatement(insertQuery); // Set parameter values preparedStatement.setString(1, "John Doe"); preparedStatement.setString(2, "john@example.com"); // Execute the prepared statement int rowsInserted = preparedStatement.executeUpdate(); System.out.println(rowsInserted + " row(s) inserted."); // Close the connection connection.close(); } catch (ClassNotFoundException | SQLException e) { e.printStackTrace(); } } }
In this example, we use a prepared statement to insert a new user into the “users” table of a MySQL database. We prepare the SQL statement with placeholders for the parameters, set the parameter values using the setXXX()
methods of the PreparedStatement
object, and execute the statement using the executeUpdate()
method. Replace localhost
with the hostname or IP address of the MySQL server, 3306
with the port number, mydatabase
with the name of the database, root
with the MySQL username, and password
with the corresponding password.
Prepared statements in Java MySQL provide a secure and efficient way to execute parameterized SQL queries. They help prevent SQL injection attacks and improve performance by reusing query execution plans.
Related Article: PHP vs Java: A Practical Comparison