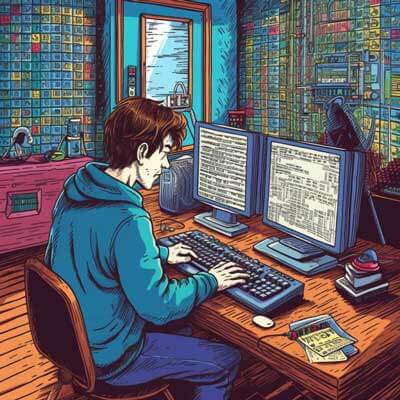
- Basics of @Configuration
- Code Snippet 1: Basic Usage of @Configuration
- Use Case 1: Using @Configuration for Dependency Injection
- Code Snippet 2: Defining Beans with @Configuration
- Use Case 2: Enabling Component Scanning with @Configuration
- Code Snippet 3: Component Scanning with @Configuration
- Use Case 3: Defining Bean Methods with @Configuration
- Code Snippet 4: Using @PropertySource with @Configuration
- Best Practice 1: Keeping Configuration Classes Package-Private
- Best Practice 2: Avoiding Cyclic References with @Configuration
- Real World Example 1: Building a Web Application with @Configuration
- Real World Example 2: Creating a Database Access Layer with @Configuration
- Performance Consideration 1: Overhead of @Configuration
- Performance Consideration 2: Impact of @Configuration on Startup Time
- Advanced Technique 1: Combining @Configuration with @Profile
- Advanced Technique 2: Using @PropertySource with @Configuration
- Code Snippet 5: Combining @Configuration with Other Annotations
- Error Handling: Common Issues with @Configuration
- Error Handling: Debugging @Configuration Issues
The Spring Framework provides various ways to configure and manage dependencies in a Java application. One of the popular approaches is through the use of annotations. Spring Configuration Annotations allow developers to define configuration classes that specify how beans should be created and wired together.
Configuration annotations provide a more concise and declarative way of configuring the Spring container compared to traditional XML configuration files. By using these annotations, developers can focus on writing code rather than dealing with cumbersome XML configurations.
In this article, we will explore the basics of Spring Configuration Annotation and delve into various use cases, best practices, real-world examples, and performance considerations associated with it.
Basics of @Configuration
The @Configuration
annotation is the core of Spring Configuration Annotation. It is used to mark a class as a configuration class, indicating that it contains bean definitions and other configuration-related methods. When the Spring container initializes, it identifies classes annotated with @Configuration
and processes them to create the necessary beans.
Let’s take a look at a basic example of using @Configuration
:
@Configuration public class AppConfig { // Bean definitions and other configuration methods go here }
In the above example, we have a class AppConfig
marked with @Configuration
. This class will be scanned by the Spring container during application startup.
Related Article: Spring Boot Integration with Payment, Communication Tools
Code Snippet 1: Basic Usage of @Configuration
@Configuration public class AppConfig { @Bean public UserService userService() { return new UserServiceImpl(); } }
In the above code snippet, we define a bean named userService
using the @Bean
annotation within the AppConfig
configuration class. The userService()
method is responsible for creating and configuring the bean instance.
Use Case 1: Using @Configuration for Dependency Injection
One of the primary use cases of @Configuration
is to enable dependency injection in Spring applications. By defining beans and their dependencies within a configuration class, the Spring container can automatically wire them together.
Let’s consider an example where we have a UserRepository
interface and its implementation UserRepositoryImpl
. We can define these beans in a configuration class as follows:
@Configuration public class AppConfig { @Bean public UserRepository userRepository() { return new UserRepositoryImpl(); } @Bean public UserService userService(UserRepository userRepository) { return new UserServiceImpl(userRepository); } }
In the above code snippet, we define two beans: userRepository
and userService
. The userService
bean has a dependency on the userRepository
bean, which is automatically injected by the Spring container.
Code Snippet 2: Defining Beans with @Configuration
@Configuration public class AppConfig { @Bean public UserRepository userRepository() { return new UserRepositoryImpl(); } @Bean public UserService userService(UserRepository userRepository) { return new UserServiceImpl(userRepository); } }
In the above code snippet, we have defined a UserRepository
bean and a UserService
bean. The userService()
method has a parameter of type UserRepository
, indicating the dependency relationship.
Related Article: Java Spring Security Customizations & RESTful API Protection
Use Case 2: Enabling Component Scanning with @Configuration
In addition to explicit bean definitions, @Configuration
also allows us to enable component scanning. Component scanning is a convenient way to automatically detect and register beans based on certain criteria, such as annotations or naming conventions.
To enable component scanning within a configuration class, we can use the @ComponentScan
annotation. This annotation tells the Spring container which packages to scan for annotated components.
Consider the following example:
@Configuration @ComponentScan("com.example") public class AppConfig { // Bean definitions and other configuration methods go here }
In the above code snippet, we have enabled component scanning for the package com.example
. The Spring container will scan this package and its subpackages for classes annotated with annotations like @Component
, @Service
, @Repository
, etc., and automatically register them as beans.
Code Snippet 3: Component Scanning with @Configuration
@Configuration @ComponentScan("com.example") public class AppConfig { // Bean definitions and other configuration methods go here }
In the above code snippet, we have enabled component scanning for the package com.example
.
Use Case 3: Defining Bean Methods with @Configuration
Another powerful feature of @Configuration
is the ability to define bean methods directly within the configuration class. These bean methods are annotated with @Bean
and provide a way to create and configure beans manually.
Let’s consider an example where we want to create a DataSource
bean:
@Configuration public class AppConfig { @Bean public DataSource dataSource() { BasicDataSource dataSource = new BasicDataSource(); dataSource.setUrl("jdbc:mysql://localhost:3306/mydb"); dataSource.setUsername("root"); dataSource.setPassword("password"); return dataSource; } }
In the above code snippet, we define a dataSource
bean using the @Bean
annotation. The dataSource()
method creates a BasicDataSource
instance, configures it with the necessary properties, and returns it as a bean.
Related Article: Tutorial on Integrating Redis with Spring Boot
Code Snippet 4: Using @PropertySource with @Configuration
@Configuration @PropertySource("classpath:application.properties") public class AppConfig { @Value("${database.url}") private String databaseUrl; @Value("${database.username}") private String databaseUsername; @Value("${database.password}") private String databasePassword; @Bean public DataSource dataSource() { BasicDataSource dataSource = new BasicDataSource(); dataSource.setUrl(databaseUrl); dataSource.setUsername(databaseUsername); dataSource.setPassword(databasePassword); return dataSource; } }
In the above code snippet, we use the @PropertySource
annotation to load properties from an external file (application.properties
). The properties are then injected into the dataSource()
method using @Value
.
Best Practice 1: Keeping Configuration Classes Package-Private
When working with @Configuration
classes, it is generally recommended to keep them package-private. This means that the configuration classes should not be directly accessible outside their package.
By keeping the configuration classes package-private, we can encapsulate the configuration logic and prevent accidental misuse by other classes. It also helps in maintaining a clean and modular codebase.
Best Practice 2: Avoiding Cyclic References with @Configuration
Cyclic references occur when two or more beans depend on each other directly or indirectly. This can lead to circular dependencies and runtime issues.
To avoid cyclic references, it is important to carefully design the dependencies between beans. In the context of @Configuration
, we should be cautious when using @Autowired
or constructor injection within the configuration class itself.
Consider the following example:
@Configuration public class AppConfig { @Autowired private UserService userService; // Other bean definitions and configuration methods }
In the above code snippet, we have a cyclic reference between the AppConfig
configuration class and the UserService
bean. This can lead to runtime errors and should be avoided.
Related Article: Identifying the Version of Your MySQL-Connector-Java
Real World Example 1: Building a Web Application with @Configuration
Let’s consider a real-world example of building a web application using @Configuration
. We will configure the necessary beans for a basic Spring MVC application.
@Configuration @EnableWebMvc @ComponentScan("com.example") public class AppConfig extends WebMvcConfigurerAdapter { @Override public void configureViewResolvers(ViewResolverRegistry registry) { registry.jsp("/WEB-INF/views/", ".jsp"); } @Override public void addResourceHandlers(ResourceHandlerRegistry registry) { registry.addResourceHandler("/static/**").addResourceLocations("/static/"); } @Bean public InternalResourceViewResolver viewResolver() { InternalResourceViewResolver viewResolver = new InternalResourceViewResolver(); viewResolver.setPrefix("/WEB-INF/views/"); viewResolver.setSuffix(".jsp"); return viewResolver; } }
In the above code snippet, we have defined a configuration class AppConfig
for a Spring MVC application. The class is annotated with @Configuration
and @EnableWebMvc
to enable MVC configuration.
Real World Example 2: Creating a Database Access Layer with @Configuration
Let’s consider another real-world example of creating a database access layer using @Configuration
. We will configure the necessary beans for connecting to a MySQL database.
@Configuration @PropertySource("classpath:application.properties") public class AppConfig { @Value("${database.url}") private String databaseUrl; @Value("${database.username}") private String databaseUsername; @Value("${database.password}") private String databasePassword; @Bean public DataSource dataSource() { BasicDataSource dataSource = new BasicDataSource(); dataSource.setUrl(databaseUrl); dataSource.setUsername(databaseUsername); dataSource.setPassword(databasePassword); return dataSource; } @Bean public JdbcTemplate jdbcTemplate(DataSource dataSource) { return new JdbcTemplate(dataSource); } }
In the above code snippet, we have defined a configuration class AppConfig
for a database access layer. The class is annotated with @Configuration
and @PropertySource
to load database properties from an external file. We create a DataSource
bean using the properties and use it to create a JdbcTemplate
bean.
Performance Consideration 1: Overhead of @Configuration
While @Configuration
provides great flexibility and ease of use, it is important to consider its potential impact on performance. The Spring container needs to process and initialize configuration classes, which can introduce some overhead.
In general, the overhead of @Configuration
is minimal and should not be a concern for most applications. However, it is advisable to avoid unnecessary configuration classes and keep them focused and concise. Also, consider the overall size and complexity of your application’s configuration.
Related Article: Proper Placement of MySQL Connector JAR File in Java
Performance Consideration 2: Impact of @Configuration on Startup Time
The number and complexity of @Configuration
classes can impact the startup time of your application. The Spring container needs to process and initialize these classes, which can take a significant amount of time, especially for large applications with numerous configuration classes.
To mitigate the impact on startup time, it is recommended to optimize your configuration classes. Avoid redundant configurations, minimize the number of configuration classes, and ensure that each configuration class is focused on a specific set of beans.
Advanced Technique 1: Combining @Configuration with @Profile
The @Profile
annotation allows you to define different sets of beans for different environments or scenarios. It can be used in conjunction with @Configuration
to conditionally activate or deactivate beans based on the active profiles.
Consider the following example:
@Configuration public class AppConfig { @Bean @Profile("development") public DataSource developmentDataSource() { // Configuration for development environment } @Bean @Profile("production") public DataSource productionDataSource() { // Configuration for production environment } }
In the above code snippet, we have defined two beans for different profiles: development
and production
. The appropriate bean will be activated based on the active profile during application startup.
Advanced Technique 2: Using @PropertySource with @Configuration
The @PropertySource
annotation allows you to load external properties files into your configuration class. It is often used in conjunction with @Configuration
to externalize configuration properties from the code.
Consider the following example:
@Configuration @PropertySource("classpath:application.properties") public class AppConfig { @Value("${database.url}") private String databaseUrl; @Value("${database.username}") private String databaseUsername; @Value("${database.password}") private String databasePassword; // Bean definitions and other configuration methods go here }
In the above code snippet, we have used @PropertySource
to load properties from application.properties
file. The properties are then injected into the configuration class using @Value
.
Related Article: How to Connect Java with MySQL
Code Snippet 5: Combining @Configuration with Other Annotations
@Configuration @EnableCaching @EnableAsync public class AppConfig { // Bean definitions and other configuration methods go here }
In the above code snippet, we have combined @Configuration
with other annotations like @EnableCaching
and @EnableAsync
. This allows us to enable additional features or functionalities in our application.
Error Handling: Common Issues with @Configuration
While using @Configuration
, it is important to be aware of common issues that may arise during development. Some of the common issues include cyclic references, misconfiguration of beans, missing or duplicate bean definitions, and incorrect property values.
To handle these issues, it is recommended to thoroughly test your configuration classes and use debugging tools to identify and resolve any issues. Additionally, referring to the Spring documentation and seeking help from the community can be beneficial.
Error Handling: Debugging @Configuration Issues
When encountering issues with @Configuration
, it can be helpful to enable debug logging for the Spring container. This will provide detailed information about the bean creation process, including any errors or misconfigurations.
To enable debug logging, you can add the following configuration to your application.properties
file:
logging.level.org.springframework.context.annotation.Configuration=DEBUG
With debug logging enabled, you can analyze the logs to identify the root cause of any issues and take appropriate actions to resolve them.
That concludes our in-depth exploration of Spring Configuration Annotation. We have covered the basics, various use cases, best practices, real-world examples, performance considerations, advanced techniques, and error handling. Spring Configuration Annotation provides a powerful and flexible way to configure your Spring applications, enabling you to write clean and modular code.